Socket APIs
|
SOCKET APIs Header file. More...
#include "wizchip_conf.h"
Go to the source code of this file.
Macros | |
#define | SOCKET uint8_t |
SOCKET type define for legacy driver. More... | |
#define | SOCK_OK 1 |
Result is OK about socket process. More... | |
#define | SOCK_BUSY 0 |
Socket is busy on processing the operation. Valid only Non-block IO Mode. More... | |
#define | SOCK_FATAL -1000 |
Result is fatal error about socket process. More... | |
#define | SOCK_ERROR 0 |
#define | SOCKERR_SOCKNUM (SOCK_ERROR - 1) |
Invalid socket number. More... | |
#define | SOCKERR_SOCKOPT (SOCK_ERROR - 2) |
Invalid socket option. More... | |
#define | SOCKERR_SOCKINIT (SOCK_ERROR - 3) |
Socket is not initialized or SIPR is Zero IP address when Sn_MR_TCP. More... | |
#define | SOCKERR_SOCKCLOSED (SOCK_ERROR - 4) |
Socket unexpectedly closed. More... | |
#define | SOCKERR_SOCKMODE (SOCK_ERROR - 5) |
Invalid socket mode for socket operation. More... | |
#define | SOCKERR_SOCKFLAG (SOCK_ERROR - 6) |
Invalid socket flag. More... | |
#define | SOCKERR_SOCKSTATUS (SOCK_ERROR - 7) |
Invalid socket status for socket operation. More... | |
#define | SOCKERR_ARG (SOCK_ERROR - 10) |
Invalid argument. More... | |
#define | SOCKERR_PORTZERO (SOCK_ERROR - 11) |
Port number is zero. More... | |
#define | SOCKERR_IPINVALID (SOCK_ERROR - 12) |
Invalid IP address. More... | |
#define | SOCKERR_TIMEOUT (SOCK_ERROR - 13) |
Timeout occurred. More... | |
#define | SOCKERR_DATALEN (SOCK_ERROR - 14) |
Data length is zero or greater than buffer max size. More... | |
#define | SOCKERR_BUFFER (SOCK_ERROR - 15) |
Socket buffer is not enough for data communication. More... | |
#define | SOCKFATAL_PACKLEN (SOCK_FATAL - 1) |
Invalid packet length. Fatal Error. More... | |
#define | SF_ETHER_OWN (Sn_MR_MFEN) |
In Sn_MR_MACRAW, Receive only the packet as broadcast, multicast and own packet. More... | |
#define | SF_IGMP_VER2 (Sn_MR_MC) |
In Sn_MR_UDP with SF_MULTI_ENABLE, Select IGMP version 2. More... | |
#define | SF_TCP_NODELAY (Sn_MR_ND) |
In Sn_MR_TCP, Use to nodelayed ack. More... | |
#define | SF_MULTI_ENABLE (Sn_MR_MULTI) |
In Sn_MR_UDP, Enable multicast mode. More... | |
#define | SF_BROAD_BLOCK (Sn_MR_BCASTB) |
In Sn_MR_UDP or Sn_MR_MACRAW, Block broadcast packet. Valid only in W5500. More... | |
#define | SF_MULTI_BLOCK (Sn_MR_MMB) |
In Sn_MR_MACRAW, Block multicast packet. Valid only in W5500. More... | |
#define | SF_IPv6_BLOCK (Sn_MR_MIP6B) |
In Sn_MR_MACRAW, Block IPv6 packet. Valid only in W5500. More... | |
#define | SF_UNI_BLOCK (Sn_MR_UCASTB) |
In Sn_MR_UDP with SF_MULTI_ENABLE. Valid only in W5500. More... | |
#define | SF_IO_NONBLOCK 0x01 |
Socket nonblock io mode. It used parameter in socket(). More... | |
#define | PACK_FIRST 0x80 |
In Non-TCP packet, It indicates to start receiving a packet. (When W5300, This flag can be applied) More... | |
#define | PACK_REMAINED 0x01 |
In Non-TCP packet, It indicates to remain a packet to be received. (When W5300, This flag can be applied) More... | |
#define | PACK_COMPLETED 0x00 |
In Non-TCP packet, It indicates to complete to receive a packet. (When W5300, This flag can be applied) More... | |
#define | PACK_FIFOBYTE 0x02 |
Valid only W5300, It indicate to have read already the Sn_RX_FIFOR. More... | |
#define | SOCK_IO_BLOCK 0 |
Socket Block IO Mode in setsockopt(). More... | |
#define | SOCK_IO_NONBLOCK 1 |
Socket Non-block IO Mode in setsockopt(). More... | |
Enumerations | |
enum | sockint_kind { SIK_CONNECTED = (1 << 0), SIK_DISCONNECTED = (1 << 1), SIK_RECEIVED = (1 << 2), SIK_TIMEOUT = (1 << 3), SIK_SENT = (1 << 4), SIK_ALL = 0x1F } |
The kind of Socket Interrupt. More... | |
enum | ctlsock_type { CS_SET_IOMODE, CS_GET_IOMODE, CS_GET_MAXTXBUF, CS_GET_MAXRXBUF, CS_CLR_INTERRUPT, CS_GET_INTERRUPT, CS_SET_INTMASK, CS_GET_INTMASK } |
The type of ctlsocket(). More... | |
enum | sockopt_type { SO_FLAG, SO_TTL, SO_TOS, SO_MSS, SO_DESTIP, SO_DESTPORT, SO_KEEPALIVESEND, SO_KEEPALIVEAUTO, SO_SENDBUF, SO_RECVBUF, SO_STATUS, SO_REMAINSIZE, SO_PACKINFO } |
The type of socket option in setsockopt() or getsockopt() More... | |
Functions | |
int8_t | socket (uint8_t sn, uint8_t protocol, uint16_t port, uint8_t flag) |
Open a socket. More... | |
int8_t | close (uint8_t sn) |
Close a socket. More... | |
int8_t | listen (uint8_t sn) |
Listen to a connection request from a client. More... | |
int8_t | connect (uint8_t sn, uint8_t *addr, uint16_t port) |
Try to connect a server. More... | |
int8_t | disconnect (uint8_t sn) |
Try to disconnect a connection socket. More... | |
int32_t | send (uint8_t sn, uint8_t *buf, uint16_t len) |
Send data to the connected peer in TCP socket. More... | |
int32_t | recv (uint8_t sn, uint8_t *buf, uint16_t len) |
Receive data from the connected peer. More... | |
int32_t | sendto (uint8_t sn, uint8_t *buf, uint16_t len, uint8_t *addr, uint16_t port) |
Sends datagram to the peer with destination IP address and port number passed as parameter. More... | |
int32_t | recvfrom (uint8_t sn, uint8_t *buf, uint16_t len, uint8_t *addr, uint16_t *port) |
Receive datagram of UDP or MACRAW. More... | |
int8_t | ctlsocket (uint8_t sn, ctlsock_type cstype, void *arg) |
Control socket. More... | |
int8_t | setsockopt (uint8_t sn, sockopt_type sotype, void *arg) |
set socket options More... | |
int8_t | getsockopt (uint8_t sn, sockopt_type sotype, void *arg) |
get socket options More... | |
Detailed Description
SOCKET APIs Header file.
SOCKET APIs like as berkeley socket api.
- Version
- 1.0.2
- Date
- 2013/10/21
- Revision history
- <2015/02/05> Notice The version history is not updated after this point. Download the latest version directly from GitHub. Please visit the our GitHub repository for ioLibrary. >> https://github.com/Wiznet/ioLibrary_Driver <2014/05/01> V1.0.2. Refer to M20140501
- Modify the comment : SO_REMAINED -> PACK_REMAINED
- Add the comment as zero byte udp data reception in getsockopt(). <2013/10/21> 1st Release
- Author
- MidnightCow
- Copyright
Copyright (c) 2013, WIZnet Co., LTD. All rights reserved.
Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
- Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution.
- Neither the name of the <ORGANIZATION> nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
Definition in file socket.h.
Macro Definition Documentation
#define SOCKET uint8_t |
#define SOCK_OK 1 |
Result is OK about socket process.
Definition at line 92 of file socket.h.
Referenced by close(), connect(), ctlsocket(), disconnect(), getsockopt(), listen(), and setsockopt().
#define SOCK_BUSY 0 |
Socket is busy on processing the operation. Valid only Non-block IO Mode.
Definition at line 93 of file socket.h.
Referenced by connect(), disconnect(), recv(), recvfrom(), send(), and sendto().
#define SOCK_FATAL -1000 |
#define SOCKERR_SOCKNUM (SOCK_ERROR - 1) |
#define SOCKERR_SOCKOPT (SOCK_ERROR - 2) |
Invalid socket option.
Definition at line 98 of file socket.h.
Referenced by getsockopt(), and setsockopt().
#define SOCKERR_SOCKINIT (SOCK_ERROR - 3) |
#define SOCKERR_SOCKCLOSED (SOCK_ERROR - 4) |
#define SOCKERR_SOCKMODE (SOCK_ERROR - 5) |
Invalid socket mode for socket operation.
Definition at line 101 of file socket.h.
Referenced by recvfrom(), sendto(), and socket().
#define SOCKERR_SOCKFLAG (SOCK_ERROR - 6) |
#define SOCKERR_SOCKSTATUS (SOCK_ERROR - 7) |
#define SOCKERR_ARG (SOCK_ERROR - 10) |
Invalid argument.
Definition at line 104 of file socket.h.
Referenced by ctlsocket(), and setsockopt().
#define SOCKERR_PORTZERO (SOCK_ERROR - 11) |
#define SOCKERR_IPINVALID (SOCK_ERROR - 12) |
#define SOCKERR_TIMEOUT (SOCK_ERROR - 13) |
Timeout occurred.
Definition at line 107 of file socket.h.
Referenced by connect(), disconnect(), send(), sendto(), and setsockopt().
#define SOCKERR_DATALEN (SOCK_ERROR - 14) |
#define SOCKERR_BUFFER (SOCK_ERROR - 15) |
#define SOCKFATAL_PACKLEN (SOCK_FATAL - 1) |
Invalid packet length. Fatal Error.
Definition at line 111 of file socket.h.
Referenced by recvfrom().
#define SF_ETHER_OWN (Sn_MR_MFEN) |
In Sn_MR_MACRAW, Receive only the packet as broadcast, multicast and own packet.
#define SF_IGMP_VER2 (Sn_MR_MC) |
In Sn_MR_UDP with SF_MULTI_ENABLE, Select IGMP version 2.
Definition at line 117 of file socket.h.
Referenced by socket().
#define SF_TCP_NODELAY (Sn_MR_ND) |
#define SF_MULTI_ENABLE (Sn_MR_MULTI) |
#define SF_BROAD_BLOCK (Sn_MR_BCASTB) |
In Sn_MR_UDP or Sn_MR_MACRAW, Block broadcast packet. Valid only in W5500.
#define SF_MULTI_BLOCK (Sn_MR_MMB) |
In Sn_MR_MACRAW, Block multicast packet. Valid only in W5500.
#define SF_IPv6_BLOCK (Sn_MR_MIP6B) |
In Sn_MR_MACRAW, Block IPv6 packet. Valid only in W5500.
#define SF_UNI_BLOCK (Sn_MR_UCASTB) |
In Sn_MR_UDP with SF_MULTI_ENABLE. Valid only in W5500.
Definition at line 125 of file socket.h.
Referenced by socket().
#define SF_IO_NONBLOCK 0x01 |
#define PACK_FIRST 0x80 |
In Non-TCP packet, It indicates to start receiving a packet. (When W5300, This flag can be applied)
Definition at line 138 of file socket.h.
Referenced by recv(), and recvfrom().
#define PACK_REMAINED 0x01 |
In Non-TCP packet, It indicates to remain a packet to be received. (When W5300, This flag can be applied)
Definition at line 139 of file socket.h.
Referenced by recv(), and recvfrom().
#define PACK_COMPLETED 0x00 |
In Non-TCP packet, It indicates to complete to receive a packet. (When W5300, This flag can be applied)
Definition at line 140 of file socket.h.
Referenced by recv(), recvfrom(), and socket().
#define PACK_FIFOBYTE 0x02 |
Valid only W5300, It indicate to have read already the Sn_RX_FIFOR.
Definition at line 142 of file socket.h.
Referenced by recv(), and recvfrom().
#define SOCK_IO_BLOCK 0 |
Socket Block IO Mode in setsockopt().
Definition at line 333 of file socket.h.
Referenced by ctlsocket().
#define SOCK_IO_NONBLOCK 1 |
Socket Non-block IO Mode in setsockopt().
Definition at line 334 of file socket.h.
Referenced by ctlsocket().
Generated on Wed May 4 2016 16:43:59 for Socket APIs by
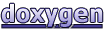