Socket APIs
|
socket.h
Go to the documentation of this file.
Set/Get keep-alive auto transmission timer in TCP mode, Not supported in W5100, W5200.
Definition: socket.h:391
Valid only in getsockopt. Get the socket status. Sn_SR, getSn_SR()
Definition: socket.h:396
int8_t ctlsocket(uint8_t sn, ctlsock_type cstype, void *arg)
Control socket.
Definition: socket.c:764
Valid only in getsockopt. Get the remained packet size in other then TCP mode.
Definition: socket.h:397
Valid only in setsockopt. Manually send keep-alive packet in TCP mode, Not supported in W5100...
Definition: socket.h:389
int8_t getsockopt(uint8_t sn, sockopt_type sotype, void *arg)
get socket options
Definition: socket.c:863
get the masked interrupt of socket. refer to sockint_kind, Not supported in W5100 ...
Definition: socket.h:371
int32_t recv(uint8_t sn, uint8_t *buf, uint16_t len)
Receive data from the connected peer.
Definition: socket.c:387
Valid only in getsockopt. Get the free data size of Socekt TX buffer. Sn_TX_FSR, getSn_TX_FSR() ...
Definition: socket.h:394
Set the destination Port number. Sn_DPORT ( setSn_DPORT(), getSn_DPORT() )
Definition: socket.h:387
Valid only in getsockopt(), For set flag of socket refer to flag in socket().
Definition: socket.h:382
int32_t sendto(uint8_t sn, uint8_t *buf, uint16_t len, uint8_t *addr, uint16_t port)
Sends datagram to the peer with destination IP address and port number passed as parameter.
Definition: socket.c:492
int8_t setsockopt(uint8_t sn, sockopt_type sotype, void *arg)
set socket options
Definition: socket.c:810
int8_t socket(uint8_t sn, uint8_t protocol, uint16_t port, uint8_t flag)
Open a socket.
Definition: socket.c:105
int32_t recvfrom(uint8_t sn, uint8_t *buf, uint16_t len, uint8_t *addr, uint16_t *port)
Receive datagram of UDP or MACRAW.
Definition: socket.c:588
Valid only in getsockopt. Get the packet information as PACK_FIRST, PACK_REMAINED, and PACK_COMPLETED in other then TCP mode.
Definition: socket.h:398
set the interrupt mask of socket with sockint_kind, Not supported in W5100
Definition: socket.h:370
Valid only in getsockopt. Get the received data size in socket RX buffer. Sn_RX_RSR, getSn_RX_RSR()
Definition: socket.h:395
int8_t connect(uint8_t sn, uint8_t *addr, uint16_t port)
Try to connect a server.
Definition: socket.c:259
WIZCHIP Config Header File.
int32_t send(uint8_t sn, uint8_t *buf, uint16_t len)
Send data to the connected peer in TCP socket.
Definition: socket.c:319
Set the destination IP address. Sn_DIPR ( setSn_DIPR(), getSn_DIPR() )
Definition: socket.h:386
Generated on Wed May 4 2016 16:43:58 for Socket APIs by
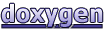