STSW-STLKT01
|
SensorTile_sd.c
Go to the documentation of this file.
208 uint8_t BSP_SD_ReadBlocks(uint32_t* p32Data, uint64_t Sector, uint16_t BlockSize, uint32_t NumberOfBlocks)
234 if (SD_IO_WriteCmd(SD_CMD_READ_SINGLE_BLOCK, Sector + offset, 0xFF, SD_RESPONSE_NO_ERROR) != HAL_OK)
287 uint8_t BSP_SD_WriteBlocks(uint32_t* p32Data, uint64_t Sector, uint16_t BlockSize, uint32_t NumberOfBlocks)
306 if (SD_IO_WriteCmd(SD_CMD_WRITE_SINGLE_BLOCK, Sector + offset, 0xFF, SD_RESPONSE_NO_ERROR) != HAL_OK)
387 /* Send CMD9 (CSD register) or CMD10(CSD register) and Wait for response in the R1 format (0x00 is no errors) */
714 if(SD_SendCmd(SD_CMD_APP_CMD, 0, 0xFF, 1) != MSD_OK) /* CMD55 because following command id SD Card Specific */
718 } while(SD_SendCmd(SD_CMD_SD_APP_OP_COND, 1UL << 30, 0xFF, SD_RESPONSE_NO_ERROR) != MSD_OK); /* ACMD41 */
760 /* Send CMD32 (Erase group start) and check if the SD acknowledged the erase command: R1 response (0x00: no errors) */
763 /* Send CMD33 (Erase group end) and Check if the SD acknowledged the erase command: R1 response (0x00: no errors) */
766 /* Send CMD38 (Erase) and Check if the SD acknowledged the erase command: R1 response (0x00: no errors) */
static uint8_t SD_GetCSDRegister(SD_CSD *Csd)
Read the CSD card register. Reading the contents of the CSD register in SPI mode is a simple read-blo...
Definition: SensorTile_sd.c:381
uint8_t BSP_SD_ReadBlocks(uint32_t *p32Data, uint64_t Sector, uint16_t BlockSize, uint32_t NumberOfBlocks)
Reads block(s) from a specified address in an SD card, in polling mode.
Definition: SensorTile_sd.c:208
void HAL_SPI_TxCpltCallback(SPI_HandleTypeDef *hspi)
TxRx Transfer completed callback.
Definition: SensorTile_sd.c:368
This file contains definitions for SensorTile_sd.c driver.
static uint8_t SD_GetCIDRegister(SD_CID *Cid)
Read the CID card register. Reading the contents of the CID register in SPI mode is a simple read-blo...
Definition: SensorTile_sd.c:499
uint8_t BSP_SD_Erase(uint32_t StartAddr, uint32_t EndAddr)
Erases the specified memory area of the given SD card.
Definition: SensorTile_sd.c:756
uint8_t BSP_SD_WriteBlocks(uint32_t *p32Data, uint64_t Sector, uint16_t BlockSize, uint32_t NumberOfBlocks)
Writes block(s) to a specified address in an SD card, in polling mode.
Definition: SensorTile_sd.c:287
#define SD_START_DATA_SINGLE_BLOCK_READ
Start Data tokens: Tokens (necessary because at nop/idle (and CS active) only 0xff is on the data/com...
Definition: SensorTile_sd.h:191
uint8_t SD_IO_WriteCmd_wResp(uint8_t Cmd, uint32_t Arg, uint8_t Crc)
Sends 5 bytes command to the SD card and get response.
Definition: SensorTile.c:1273
HAL_StatusTypeDef SD_IO_WriteCmd(uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response)
Sends 5 bytes command to the SD card and get response.
Definition: SensorTile.c:1312
uint8_t BSP_SD_GetCardInfo(SD_CardInfo *pCardInfo)
Returns information about specific card.
Definition: SensorTile_sd.c:185
void SD_IO_Init(void)
Initializes the SD Card and put it into StandBy State (Ready for data transfer).
Definition: SensorTile.c:1140
static uint8_t SD_SendCmd(uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response)
Send 5 bytes command to the SD card and get response.
Definition: SensorTile_sd.c:605
void SD_IO_Init_LS(void)
Initializes the SD Card and put it into StandBy State (Ready for data transfer). Low baundrate...
Definition: SensorTile.c:1167
static uint8_t SD_SendCmd_wResp(uint8_t Cmd, uint32_t Arg, uint8_t Crc)
Send 5 bytes command to the SD card and get response.
Definition: SensorTile_sd.c:592
HAL_StatusTypeDef SD_IO_WaitResponse(uint8_t Response)
Waits response from the SD card.
Definition: SensorTile.c:1347
void SD_IO_WriteDMA(uint8_t *pData, uint16_t Size)
Writes a block by DMA on the SD.
Definition: SensorTile.c:1237
Generated by
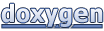