STSW-STLKT01
|
SensorTile_accelero.h
Go to the documentation of this file.
125 DrvStatusTypeDef BSP_ACCELERO_Get_Free_Fall_Detection_Status_Ext( void *handle, uint8_t *status );
126 DrvStatusTypeDef BSP_ACCELERO_Set_Free_Fall_Threshold_Ext( void *handle, LSM6DSM_ACC_GYRO_FF_THS_t thr );
141 DrvStatusTypeDef BSP_ACCELERO_Get_Wake_Up_Detection_Status_Ext( void *handle, uint8_t *status );
146 DrvStatusTypeDef BSP_ACCELERO_Get_Single_Tap_Detection_Status_Ext( void *handle, uint8_t *status );
149 DrvStatusTypeDef BSP_ACCELERO_Get_Double_Tap_Detection_Status_Ext( void *handle, uint8_t *status );
DrvStatusTypeDef BSP_ACCELERO_Get_Sensitivity(void *handle, float *sensitivity)
Get the accelerometer sensor sensitivity.
Definition: SensorTile_accelero.c:643
DrvStatusTypeDef BSP_ACCELERO_Get_ODR(void *handle, float *odr)
Get the accelerometer sensor output data rate.
Definition: SensorTile_accelero.c:683
DrvStatusTypeDef BSP_ACCELERO_Get_Instance(void *handle, uint8_t *instance)
Get the accelerometer sensor instance.
Definition: SensorTile_accelero.c:468
DrvStatusTypeDef BSP_ACCELERO_Get_6D_Orientation_ZL_Ext(void *handle, uint8_t *zl)
Get the 6D orientation ZL axis (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2717
DrvStatusTypeDef BSP_ACCELERO_Init(ACCELERO_ID_t id, void **handle)
Initialize an accelerometer sensor.
Definition: SensorTile_accelero.c:93
DrvStatusTypeDef BSP_ACCELERO_Get_6D_Orientation_YH_Ext(void *handle, uint8_t *yh)
Get the 6D orientation YH axis (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2666
DrvStatusTypeDef BSP_ACCELERO_Get_Step_Count_Ext(void *handle, uint16_t *step_count)
Get the step counter (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1420
DrvStatusTypeDef BSP_ACCELERO_Get_6D_Orientation_XH_Ext(void *handle, uint8_t *xh)
Get the 6D orientation XH axis (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2564
DrvStatusTypeDef BSP_ACCELERO_Get_Double_Tap_Detection_Status_Ext(void *handle, uint8_t *status)
Get the double tap detection status (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2137
DrvStatusTypeDef BSP_ACCELERO_IsInitialized(void *handle, uint8_t *status)
Check if the accelerometer sensor is initialized.
Definition: SensorTile_accelero.c:387
DrvStatusTypeDef BSP_ACCELERO_Get_Pedometer_Status_Ext(void *handle, uint8_t *status)
Get the pedometer status (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1369
DrvStatusTypeDef BSP_ACCELERO_Write_Reg(void *handle, uint8_t reg, uint8_t data)
Write the data to register.
Definition: SensorTile_accelero.c:1021
DrvStatusTypeDef BSP_ACCELERO_Get_AxesRaw(void *handle, SensorAxesRaw_t *value)
Get the accelerometer sensor raw axes.
Definition: SensorTile_accelero.c:605
DrvStatusTypeDef BSP_ACCELERO_Disable_Tilt_Detection_Ext(void *handle)
Disable the tilt detection (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1619
DrvStatusTypeDef BSP_ACCELERO_Get_Wake_Up_Detection_Status_Ext(void *handle, uint8_t *status)
Get the status of the wake up detection (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1807
DrvStatusTypeDef BSP_ACCELERO_Set_FS(void *handle, SensorFs_t fullScale)
Set the accelerometer sensor full scale.
Definition: SensorTile_accelero.c:831
DrvStatusTypeDef BSP_ACCELERO_Reset_Step_Counter_Ext(void *handle)
Reset of the step counter (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1470
DrvStatusTypeDef BSP_ACCELERO_Set_Free_Fall_Threshold_Ext(void *handle, LSM6DSM_ACC_GYRO_FF_THS_t thr)
Set the free fall detection threshold (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1232
DrvStatusTypeDef BSP_ACCELERO_Enable_Wake_Up_Detection_Ext(void *handle)
Enable the wake up detection (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1716
DrvStatusTypeDef BSP_ACCELERO_Set_Tap_Quiet_Time_Ext(void *handle, uint8_t time)
Set the tap quiet time window (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2279
DrvStatusTypeDef BSP_ACCELERO_Check_WhoAmI(void *handle)
Check the WHO_AM_I ID of the accelerometer sensor.
Definition: SensorTile_accelero.c:530
DrvStatusTypeDef BSP_ACCELERO_Enable_Single_Tap_Detection_Ext(void *handle)
Enable the single tap detection (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1904
DrvStatusTypeDef BSP_ACCELERO_Enable_Tilt_Detection_Ext(void *handle)
Enable the tilt detection (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1574
DrvStatusTypeDef BSP_ACCELERO_Enable_Pedometer_Ext(void *handle)
Enable the pedometer feature (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1278
DrvStatusTypeDef BSP_ACCELERO_Disable_6D_Orientation_Ext(void *handle)
Disable the 6D orientation detection (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2416
DrvStatusTypeDef BSP_ACCELERO_Read_Reg(void *handle, uint8_t reg, uint8_t *data)
Read the data from register.
Definition: SensorTile_accelero.c:979
DrvStatusTypeDef BSP_ACCELERO_Enable_Free_Fall_Detection_Ext(void *handle)
Enable the free fall detection (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1090
DrvStatusTypeDef BSP_ACCELERO_Get_Axes(void *handle, SensorAxes_t *acceleration)
Get the accelerometer sensor axes.
Definition: SensorTile_accelero.c:565
This file contains definitions for SensorTile.c file.
DrvStatusTypeDef BSP_ACCELERO_Disable_Wake_Up_Detection_Ext(void *handle)
Disable the wake up detection (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1761
DrvStatusTypeDef BSP_ACCELERO_Disable_Free_Fall_Detection_Ext(void *handle)
Disable the free fall detection (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1135
DrvStatusTypeDef BSP_ACCELERO_Get_Tilt_Detection_Status_Ext(void *handle, uint8_t *status)
Get the tilt detection status (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1665
DrvStatusTypeDef BSP_ACCELERO_Get_Free_Fall_Detection_Status_Ext(void *handle, uint8_t *status)
Get the status of the free fall detection (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1181
DrvStatusTypeDef BSP_ACCELERO_Get_6D_Orientation_XL_Ext(void *handle, uint8_t *xl)
Get the 6D orientation XL axis (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2513
DrvStatusTypeDef BSP_ACCELERO_Set_Axes_Status(void *handle, uint8_t *enable_xyz)
Set the enabled/disabled status of the accelerometer sensor axes.
Definition: SensorTile_accelero.c:939
DrvStatusTypeDef BSP_ACCELERO_Disable_Pedometer_Ext(void *handle)
Disable the pedometer feature (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1323
DrvStatusTypeDef BSP_ACCELERO_DeInit(void **handle)
Deinitialize accelerometer sensor.
Definition: SensorTile_accelero.c:282
DrvStatusTypeDef BSP_ACCELERO_Sensor_Enable(void *handle)
Enable accelerometer sensor.
Definition: SensorTile_accelero.c:319
DrvStatusTypeDef BSP_ACCELERO_IsCombo(void *handle, uint8_t *status)
Check if the accelerometer sensor is combo.
Definition: SensorTile_accelero.c:441
DrvStatusTypeDef BSP_ACCELERO_Set_ODR_Value(void *handle, float odr)
Set the accelerometer sensor output data rate.
Definition: SensorTile_accelero.c:757
DrvStatusTypeDef BSP_ACCELERO_Set_Pedometer_Threshold_Ext(void *handle, uint8_t thr)
Set the pedometer threshold (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1528
DrvStatusTypeDef BSP_ACCELERO_IsEnabled(void *handle, uint8_t *status)
Check if the accelerometer sensor is enabled.
Definition: SensorTile_accelero.c:414
DrvStatusTypeDef BSP_ACCELERO_Get_WhoAmI(void *handle, uint8_t *who_am_i)
Get the WHO_AM_I ID of the accelerometer sensor.
Definition: SensorTile_accelero.c:496
DrvStatusTypeDef BSP_ACCELERO_Get_6D_Orientation_YL_Ext(void *handle, uint8_t *yl)
Get the 6D orientation YL axis (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2615
DrvStatusTypeDef BSP_ACCELERO_Set_Tap_Threshold_Ext(void *handle, uint8_t thr)
Set the tap threshold (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2187
DrvStatusTypeDef BSP_ACCELERO_Get_6D_Orientation_Status_Ext(void *handle, uint8_t *status)
Get the status of the 6D orientation detection (available only for LSM6DS3 sensor) ...
Definition: SensorTile_accelero.c:2462
DrvStatusTypeDef BSP_ACCELERO_Get_FS(void *handle, float *fullScale)
Get the accelerometer sensor full scale.
Definition: SensorTile_accelero.c:791
DrvStatusTypeDef BSP_ACCELERO_Enable_6D_Orientation_Ext(void *handle)
Enable the 6D orientation detection (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2371
DrvStatusTypeDef BSP_ACCELERO_Set_FS_Value(void *handle, float fullScale)
Set the accelerometer sensor full scale.
Definition: SensorTile_accelero.c:865
DrvStatusTypeDef BSP_ACCELERO_Set_Tap_Shock_Time_Ext(void *handle, uint8_t time)
Set the tap shock time window (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2233
DrvStatusTypeDef BSP_ACCELERO_Get_Single_Tap_Detection_Status_Ext(void *handle, uint8_t *status)
Get the single tap detection status (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1995
DrvStatusTypeDef BSP_ACCELERO_Get_Axes_Status(void *handle, uint8_t *xyz_enabled)
Get the accelerometer sensor axes status.
Definition: SensorTile_accelero.c:899
DrvStatusTypeDef BSP_ACCELERO_Get_DRDY_Status(void *handle, uint8_t *status)
Get accelerometer data ready status.
Definition: SensorTile_accelero.c:1056
DrvStatusTypeDef BSP_ACCELERO_Get_6D_Orientation_ZH_Ext(void *handle, uint8_t *zh)
Get the 6D orientation ZH axis (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2768
DrvStatusTypeDef BSP_ACCELERO_Set_ODR(void *handle, SensorOdr_t odr)
Set the accelerometer sensor output data rate.
Definition: SensorTile_accelero.c:723
DrvStatusTypeDef BSP_ACCELERO_Sensor_Disable(void *handle)
Disable accelerometer sensor.
Definition: SensorTile_accelero.c:353
DrvStatusTypeDef BSP_ACCELERO_Disable_Double_Tap_Detection_Ext(void *handle)
Disable the double tap detection (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2091
DrvStatusTypeDef BSP_ACCELERO_Enable_Double_Tap_Detection_Ext(void *handle)
Enable the double tap detection (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2046
DrvStatusTypeDef BSP_ACCELERO_Disable_Single_Tap_Detection_Ext(void *handle)
Disable the single tap detection (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1949
DrvStatusTypeDef BSP_ACCELERO_Set_Tap_Duration_Time_Ext(void *handle, uint8_t time)
Set the tap duration of the time window (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:2325
DrvStatusTypeDef BSP_ACCELERO_Set_Wake_Up_Threshold_Ext(void *handle, uint8_t thr)
Set the wake up threshold (available only for LSM6DS3 sensor)
Definition: SensorTile_accelero.c:1858
Generated by
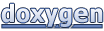