PxVehicleWheels Class Reference
[Vehicle]
Data structure with instanced dynamics data and configuration data of a vehicle with just wheels.
More...
#include <PxVehicleWheels.h>
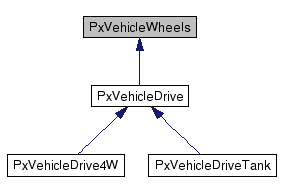
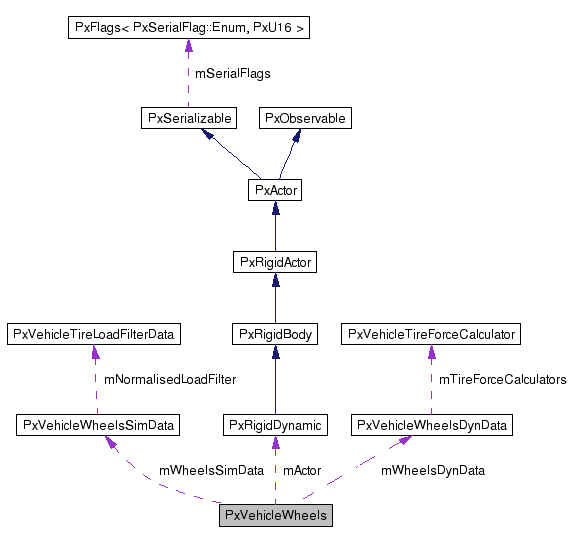
Public Member Functions | |
PX_FORCE_INLINE PxU32 | getVehicleType () const |
Return the type of vehicle. | |
PX_FORCE_INLINE PxRigidDynamic * | getRigidDynamicActor () |
Get non-const ptr to PxRigidDynamic instance that is the vehicle's physx representation. | |
PX_FORCE_INLINE const PxRigidDynamic * | getRigidDynamicActor () const |
Get non-const ptr to PxRigidDynamic instance that is the vehicle's physx representation. | |
void | setWheelShapeMapping (const PxU32 wheelId, const PxI32 shapeId) |
Set mapping between wheel id and position of corresponding wheel shape in the list of actor shapes. This mapping is used to pose the correct wheel shapes with the latest wheel rotation angle, steer angle, and suspension travel and allows arbitrary ordering of the wheel shapes in the actor's list of shapes. Use setWheelShapeMapping(i,-1) to register that there is no wheel shape corresponding to the ith wheel Set setWheelShapeMapping(i,k) to register that the ith wheel corresponds to the kth shape in the actor's list of shapes. Default value are provided in the setup functions. The default values correspond to setWheelShapeMapping(i,i) for all i<numWheels. | |
PxReal | computeForwardSpeed () const |
Compute the rigid body velocity component along the forward vector. | |
PxReal | computeSidewaysSpeed () const |
Compute the rigid body velocity component along the right vector. | |
bool | isInAir () const |
Test if all wheels are off the ground. | |
bool | isInAir (const PxU32 wheelId) const |
Test if a specific wheel is off the ground. | |
Public Attributes | |
PxVehicleWheelsSimData | mWheelsSimData |
Data describing the setup of all the wheels/suspensions/tires. | |
PxVehicleWheelsDynData | mWheelsDynData |
Data describing the dynamic state of all wheels/suspension/tires. | |
Protected Member Functions | |
void | setToRestState () |
Set all wheels to their rest state. | |
bool | isValid () const |
Test that all configuration and instanced dynamics data is valid. | |
void | free () |
Deallocate a PxVehicleWheels instance. | |
void | setup (PxPhysics *physics, PxRigidDynamic *vehActor, const PxVehicleWheelsSimData &wheelsData, const PxU32 numDrivenWheels, const PxU32 numNonDrivenWheels) |
Static Protected Member Functions | |
static PxU32 | computeByteSize (const PxU32 numWheels4) |
static PxU8 * | patchupPointers (PxVehicleWheels *veh, PxU8 *ptr, const PxU32 numWheels4, const PxU32 numWheels) |
Protected Attributes | |
PxRigidDynamic * | mActor |
PxU8 | mWheelShapeMap [PX_MAX_NUM_WHEELS] |
Mapping between wheel id and shape id. | |
PxU8 | mType |
Vehicle type (eVehicleDriveTypes). | |
Private Attributes | |
PxU8 | mOnConstraintReleaseCounter |
Count the number of constraint connectors that have hit their callback when deleting a vehicle. Can only delete the vehicle's memory when all constraint connectors have hit their callback. | |
PxU8 | mPad [2] |
PxU32 | mPad2 [1] |
Friends | |
class | PxVehicleUpdate |
class | PxVehicleConstraintShader |
Detailed Description
Data structure with instanced dynamics data and configuration data of a vehicle with just wheels.
- See also:
- PxVehicleDrive, PxVehicleDrive4W, PxVehicleDriveTank
Member Function Documentation
PxReal PxVehicleWheels::computeForwardSpeed | ( | ) | const |
Compute the rigid body velocity component along the forward vector.
PxReal PxVehicleWheels::computeSidewaysSpeed | ( | ) | const |
Compute the rigid body velocity component along the right vector.
void PxVehicleWheels::free | ( | ) | [protected] |
Deallocate a PxVehicleWheels instance.
Reimplemented in PxVehicleDrive, PxVehicleDrive4W, and PxVehicleDriveTank.
PX_FORCE_INLINE const PxRigidDynamic* PxVehicleWheels::getRigidDynamicActor | ( | ) | const [inline] |
Get non-const ptr to PxRigidDynamic instance that is the vehicle's physx representation.
PX_FORCE_INLINE PxRigidDynamic* PxVehicleWheels::getRigidDynamicActor | ( | ) | [inline] |
Get non-const ptr to PxRigidDynamic instance that is the vehicle's physx representation.
PX_FORCE_INLINE PxU32 PxVehicleWheels::getVehicleType | ( | ) | const [inline] |
Return the type of vehicle.
- See also:
bool PxVehicleWheels::isInAir | ( | const PxU32 | wheelId | ) | const |
Test if a specific wheel is off the ground.
bool PxVehicleWheels::isInAir | ( | ) | const |
Test if all wheels are off the ground.
bool PxVehicleWheels::isValid | ( | ) | const [protected] |
Test that all configuration and instanced dynamics data is valid.
Reimplemented in PxVehicleDrive, PxVehicleDrive4W, and PxVehicleDriveTank.
static PxU8* PxVehicleWheels::patchupPointers | ( | PxVehicleWheels * | veh, | |
PxU8 * | ptr, | |||
const PxU32 | numWheels4, | |||
const PxU32 | numWheels | |||
) | [static, protected] |
void PxVehicleWheels::setToRestState | ( | ) | [protected] |
Set all wheels to their rest state.
Reimplemented in PxVehicleDrive, PxVehicleDrive4W, and PxVehicleDriveTank.
void PxVehicleWheels::setup | ( | PxPhysics * | physics, | |
PxRigidDynamic * | vehActor, | |||
const PxVehicleWheelsSimData & | wheelsData, | |||
const PxU32 | numDrivenWheels, | |||
const PxU32 | numNonDrivenWheels | |||
) | [protected] |
Set mapping between wheel id and position of corresponding wheel shape in the list of actor shapes. This mapping is used to pose the correct wheel shapes with the latest wheel rotation angle, steer angle, and suspension travel and allows arbitrary ordering of the wheel shapes in the actor's list of shapes. Use setWheelShapeMapping(i,-1) to register that there is no wheel shape corresponding to the ith wheel Set setWheelShapeMapping(i,k) to register that the ith wheel corresponds to the kth shape in the actor's list of shapes. Default value are provided in the setup functions. The default values correspond to setWheelShapeMapping(i,i) for all i<numWheels.
Friends And Related Function Documentation
friend class PxVehicleConstraintShader [friend] |
friend class PxVehicleUpdate [friend] |
Reimplemented in PxVehicleDrive, PxVehicleDrive4W, and PxVehicleDriveTank.
Member Data Documentation
PxRigidDynamic* PxVehicleWheels::mActor [protected] |
Count the number of constraint connectors that have hit their callback when deleting a vehicle. Can only delete the vehicle's memory when all constraint connectors have hit their callback.
PxU8 PxVehicleWheels::mPad[2] [private] |
Reimplemented in PxVehicleDriveTank.
PxU32 PxVehicleWheels::mPad2[1] [private] |
PxU8 PxVehicleWheels::mType [protected] |
Vehicle type (eVehicleDriveTypes).
Data describing the dynamic state of all wheels/suspension/tires.
PxU8 PxVehicleWheels::mWheelShapeMap[PX_MAX_NUM_WHEELS] [protected] |
Mapping between wheel id and shape id.
Data describing the setup of all the wheels/suspensions/tires.
The documentation for this class was generated from the following file:
Copyright © 2008-2012 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com