PxVehicleWheelsSimData Class Reference
[Vehicle]
Data structure describing configuration data of a vehicle with up to 20 wheels,.
More...
#include <PxVehicleWheels.h>
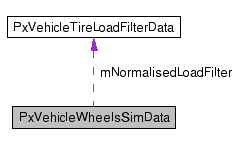
Public Member Functions | |
void | setChassisMass (const PxF32 chassisMass) |
Setup with mass information that can be applied to the default values of the suspensions, wheels, and tires set in their respective constructors. This function assumes that the suspensions equally share the load of the chassis mass. It also assumes that the suspension will have a particular natural frequency and damping ratio that is typical of a standard car. If either of these assumptions is broken then each suspension will need to be individually configured with custom strength, damping rate, and sprung mass. | |
void | free () |
Free a PxVehicleWheelsSimData instance. | |
PxVehicleWheelsSimData & | operator= (const PxVehicleWheelsSimData &src) |
Copy wheel simulation data. | |
void | copy (const PxVehicleWheelsSimData &src, const PxU32 srcWheel, const PxU32 trgWheel) |
Copy the data of a single wheel unit (wheel, suspension, tire) from srcWheel of src to trgWheel. | |
PxU32 | getNumWheels () const |
Return the number of wheels. | |
const PxVehicleSuspensionData & | getSuspensionData (const PxU32 id) const |
Return the suspension data of the idth wheel. | |
const PxVehicleWheelData & | getWheelData (const PxU32 id) const |
Return the wheel data of the idth wheel. | |
const PxVehicleTireData & | getTireData (const PxU32 id) const |
Return the tire data of the idth wheel. | |
const PxVec3 & | getSuspTravelDirection (const PxU32 id) const |
Return the direction of travel of the suspension of the idth wheel. | |
const PxVec3 & | getSuspForceAppPointOffset (const PxU32 id) const |
Return the application point of the suspension force of the suspension of the idth wheel. | |
const PxVec3 & | getTireForceAppPointOffset (const PxU32 id) const |
Return the application point of the tire force of the tire of the idth wheel. | |
const PxVec3 & | getWheelCentreOffset (const PxU32 id) const |
Return the offset from the rigid body centre of mass to the centre of the idth wheel. | |
PX_FORCE_INLINE const PxVehicleTireLoadFilterData & | getTireLoadFilterData () const |
Return the data that describes the filtering of the tire load to produce smoother handling at large timesteps. | |
void | setSuspensionData (const PxU32 id, const PxVehicleSuspensionData &susp) |
Set the suspension data of the idth wheel. | |
void | setWheelData (const PxU32 id, const PxVehicleWheelData &wheel) |
Set the wheel data of the idth wheel. | |
void | setTireData (const PxU32 id, const PxVehicleTireData &tire) |
Set the tire data of the idth wheel. | |
void | setSuspTravelDirection (const PxU32 id, const PxVec3 &dir) |
Set the direction of travel of the suspension of the idth wheel. | |
void | setSuspForceAppPointOffset (const PxU32 id, const PxVec3 &offset) |
Set the application point of the suspension force of the suspension of the idth wheel. | |
void | setTireForceAppPointOffset (const PxU32 id, const PxVec3 &offset) |
Set the application point of the tire force of the tire of the idth wheel. | |
void | setWheelCentreOffset (const PxU32 id, const PxVec3 &offset) |
Set the offset from the rigid body centre of mass to the centre of the idth wheel. | |
void | setTireLoadFilterData (const PxVehicleTireLoadFilterData &tireLoadFilter) |
Set the data that describes the filtering of the tire load to produce smoother handling at large timesteps. | |
void | disableWheel (const PxU32 wheel) |
Disable a wheel so that zero suspension forces and zero tire forces are applied to the rigid body from this wheel. | |
bool | getIsWheelDisabled (const PxU32 wheel) const |
Test if a wheel has been disabled. | |
Static Public Member Functions | |
static PxVehicleWheelsSimData * | allocate (const PxU32 numWheels) |
Allocate a PxVehicleWheelsSimData instance for with numWheels. | |
Private Member Functions | |
PxVehicleWheelsSimData () | |
~PxVehicleWheelsSimData () | |
bool | isValid () const |
Test if wheel simulation data has been setup with legal values. | |
Private Attributes | |
PxVehicleTireLoadFilterData | mNormalisedLoadFilter |
Graph to filter normalised load. | |
PxVehicleWheels4SimData * | mWheels4SimData |
Wheels data organised in blocks of 4 wheels. | |
PxU32 | mNumWheels4 |
Number of blocks of 4 wheels. | |
PxU32 | mNumActiveWheels |
Number of actual wheels (<=(mNumWheels4*4)). | |
PxU32 | mPad [1] |
Friends | |
class | PxVehicleWheels |
class | PxVehicleDrive4W |
class | PxVehicleDriveTank |
class | PxVehicleUpdate |
Detailed Description
Data structure describing configuration data of a vehicle with up to 20 wheels,.Constructor & Destructor Documentation
PxVehicleWheelsSimData::PxVehicleWheelsSimData | ( | ) | [inline, private] |
PxVehicleWheelsSimData::~PxVehicleWheelsSimData | ( | ) | [inline, private] |
Member Function Documentation
static PxVehicleWheelsSimData* PxVehicleWheelsSimData::allocate | ( | const PxU32 | numWheels | ) | [static] |
void PxVehicleWheelsSimData::copy | ( | const PxVehicleWheelsSimData & | src, | |
const PxU32 | srcWheel, | |||
const PxU32 | trgWheel | |||
) |
Copy the data of a single wheel unit (wheel, suspension, tire) from srcWheel of src to trgWheel.
void PxVehicleWheelsSimData::disableWheel | ( | const PxU32 | wheel | ) |
Disable a wheel so that zero suspension forces and zero tire forces are applied to the rigid body from this wheel.
void PxVehicleWheelsSimData::free | ( | ) |
bool PxVehicleWheelsSimData::getIsWheelDisabled | ( | const PxU32 | wheel | ) | const |
Test if a wheel has been disabled.
PxU32 PxVehicleWheelsSimData::getNumWheels | ( | ) | const [inline] |
const PxVehicleSuspensionData& PxVehicleWheelsSimData::getSuspensionData | ( | const PxU32 | id | ) | const |
Return the suspension data of the idth wheel.
Return the application point of the suspension force of the suspension of the idth wheel.
Specified relative to the centre of mass of the rigid body
Return the direction of travel of the suspension of the idth wheel.
const PxVehicleTireData& PxVehicleWheelsSimData::getTireData | ( | const PxU32 | id | ) | const |
Return the tire data of the idth wheel.
Return the application point of the tire force of the tire of the idth wheel.
Specified relative to the centre of mass of the rigid body
PX_FORCE_INLINE const PxVehicleTireLoadFilterData& PxVehicleWheelsSimData::getTireLoadFilterData | ( | ) | const [inline] |
Return the data that describes the filtering of the tire load to produce smoother handling at large timesteps.
Return the offset from the rigid body centre of mass to the centre of the idth wheel.
const PxVehicleWheelData& PxVehicleWheelsSimData::getWheelData | ( | const PxU32 | id | ) | const |
Return the wheel data of the idth wheel.
bool PxVehicleWheelsSimData::isValid | ( | ) | const [private] |
Test if wheel simulation data has been setup with legal values.
PxVehicleWheelsSimData& PxVehicleWheelsSimData::operator= | ( | const PxVehicleWheelsSimData & | src | ) |
Copy wheel simulation data.
void PxVehicleWheelsSimData::setChassisMass | ( | const PxF32 | chassisMass | ) |
Setup with mass information that can be applied to the default values of the suspensions, wheels, and tires set in their respective constructors. This function assumes that the suspensions equally share the load of the chassis mass. It also assumes that the suspension will have a particular natural frequency and damping ratio that is typical of a standard car. If either of these assumptions is broken then each suspension will need to be individually configured with custom strength, damping rate, and sprung mass.
- See also:
- allocate
void PxVehicleWheelsSimData::setSuspensionData | ( | const PxU32 | id, | |
const PxVehicleSuspensionData & | susp | |||
) |
Set the suspension data of the idth wheel.
Set the application point of the suspension force of the suspension of the idth wheel.
Specified relative to the centre of mass of the rigid body
Set the direction of travel of the suspension of the idth wheel.
void PxVehicleWheelsSimData::setTireData | ( | const PxU32 | id, | |
const PxVehicleTireData & | tire | |||
) |
Set the tire data of the idth wheel.
Set the application point of the tire force of the tire of the idth wheel.
Specified relative to the centre of mass of the rigid body
void PxVehicleWheelsSimData::setTireLoadFilterData | ( | const PxVehicleTireLoadFilterData & | tireLoadFilter | ) |
Set the data that describes the filtering of the tire load to produce smoother handling at large timesteps.
Set the offset from the rigid body centre of mass to the centre of the idth wheel.
void PxVehicleWheelsSimData::setWheelData | ( | const PxU32 | id, | |
const PxVehicleWheelData & | wheel | |||
) |
Set the wheel data of the idth wheel.
Friends And Related Function Documentation
friend class PxVehicleDrive4W [friend] |
friend class PxVehicleDriveTank [friend] |
friend class PxVehicleUpdate [friend] |
friend class PxVehicleWheels [friend] |
Member Data Documentation
Number of actual wheels (<=(mNumWheels4*4)).
PxU32 PxVehicleWheelsSimData::mNumWheels4 [private] |
Number of blocks of 4 wheels.
PxU32 PxVehicleWheelsSimData::mPad[1] [private] |
PxVehicleWheels4SimData* PxVehicleWheelsSimData::mWheels4SimData [private] |
Wheels data organised in blocks of 4 wheels.
The documentation for this class was generated from the following file:
Copyright © 2008-2012 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com