PxTriangleMesh Class Reference
[Geomutils]
A triangle mesh, also called a 'polygon soup'.
More...
#include <PxTriangleMesh.h>
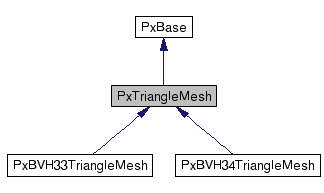
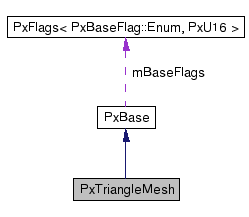
Public Member Functions | |
virtual PxU32 | getNbVertices () const =0 |
Returns the number of vertices. | |
virtual const PxVec3 * | getVertices () const =0 |
Returns the vertices. | |
virtual PxVec3 * | getVerticesForModification ()=0 |
Returns all mesh vertices for modification. | |
virtual PxBounds3 | refitBVH ()=0 |
Refits BVH for mesh vertices. | |
virtual PxU32 | getNbTriangles () const =0 |
Returns the number of triangles. | |
virtual const void * | getTriangles () const =0 |
Returns the triangle indices. | |
virtual PxTriangleMeshFlags | getTriangleMeshFlags () const =0 |
Reads the PxTriangleMesh flags. | |
virtual const PxU32 * | getTrianglesRemap () const =0 |
Returns the triangle remapping table. | |
virtual void | release ()=0 |
Decrements the reference count of a triangle mesh and releases it if the new reference count is zero. | |
virtual PxMaterialTableIndex | getTriangleMaterialIndex (PxTriangleID triangleIndex) const =0 |
Returns material table index of given triangle. | |
virtual PxBounds3 | getLocalBounds () const =0 |
Returns the local-space (vertex space) AABB from the triangle mesh. | |
virtual PxU32 | getReferenceCount () const =0 |
Returns the reference count for shared meshes. | |
virtual void | acquireReference ()=0 |
Acquires a counted reference to a triangle mesh. | |
Protected Member Functions | |
PX_INLINE | PxTriangleMesh (PxType concreteType, PxBaseFlags baseFlags) |
PX_INLINE | PxTriangleMesh (PxBaseFlags baseFlags) |
virtual | ~PxTriangleMesh () |
virtual bool | isKindOf (const char *name) const |
Returns whether a given type name matches with the type of this instance. |
Detailed Description
A triangle mesh, also called a 'polygon soup'.It is represented as an indexed triangle list. There are no restrictions on the triangle data.
To avoid duplicating data when you have several instances of a particular mesh positioned differently, you do not use this class to represent a mesh object directly. Instead, you create an instance of this mesh via the PxTriangleMeshGeometry and PxShape classes.
Creation
To create an instance of this class call PxPhysics::createTriangleMesh(), and release() to delete it. This is only possible once you have released all of its PxShape instances.
Visualizations:
- PxVisualizationParameter::eCOLLISION_AABBS
- PxVisualizationParameter::eCOLLISION_SHAPES
- PxVisualizationParameter::eCOLLISION_AXES
- PxVisualizationParameter::eCOLLISION_FNORMALS
- PxVisualizationParameter::eCOLLISION_EDGES
Constructor & Destructor Documentation
PX_INLINE PxTriangleMesh::PxTriangleMesh | ( | PxType | concreteType, | |
PxBaseFlags | baseFlags | |||
) | [inline, protected] |
PX_INLINE PxTriangleMesh::PxTriangleMesh | ( | PxBaseFlags | baseFlags | ) | [inline, protected] |
virtual PxTriangleMesh::~PxTriangleMesh | ( | ) | [inline, protected, virtual] |
Member Function Documentation
virtual void PxTriangleMesh::acquireReference | ( | ) | [pure virtual] |
Acquires a counted reference to a triangle mesh.
This method increases the reference count of the triangle mesh by 1. Decrement the reference count by calling release()
virtual PxBounds3 PxTriangleMesh::getLocalBounds | ( | ) | const [pure virtual] |
Returns the local-space (vertex space) AABB from the triangle mesh.
- Returns:
- local-space bounds
virtual PxU32 PxTriangleMesh::getNbTriangles | ( | ) | const [pure virtual] |
Returns the number of triangles.
- Returns:
- number of triangles
- See also:
- getTriangles() getTrianglesRemap()
virtual PxU32 PxTriangleMesh::getNbVertices | ( | ) | const [pure virtual] |
virtual PxU32 PxTriangleMesh::getReferenceCount | ( | ) | const [pure virtual] |
Returns the reference count for shared meshes.
At creation, the reference count of the mesh is 1. Every shape referencing this mesh increments the count by 1. When the reference count reaches 0, and only then, the mesh gets destroyed automatically.
- Returns:
- the current reference count.
virtual PxMaterialTableIndex PxTriangleMesh::getTriangleMaterialIndex | ( | PxTriangleID | triangleIndex | ) | const [pure virtual] |
Returns material table index of given triangle.
This function takes a post cooking triangle index.
- Parameters:
-
[in] triangleIndex (internal) index of desired triangle
- Returns:
- Material table index, or 0xffff if no per-triangle materials are used
virtual PxTriangleMeshFlags PxTriangleMesh::getTriangleMeshFlags | ( | ) | const [pure virtual] |
Reads the PxTriangleMesh flags.
See the list of flags PxTriangleMeshFlag
- Returns:
- The values of the PxTriangleMesh flags.
- See also:
- PxTriangleMesh
virtual const void* PxTriangleMesh::getTriangles | ( | ) | const [pure virtual] |
Returns the triangle indices.
The indices can be 16 or 32bit depending on the number of triangles in the mesh. Call getTriangleMeshFlags() to know if the indices are 16 or 32 bits.
The number of indices is the number of triangles * 3.
- Returns:
- array of triangles
virtual const PxU32* PxTriangleMesh::getTrianglesRemap | ( | ) | const [pure virtual] |
Returns the triangle remapping table.
The triangles are internally sorted according to various criteria. Hence the internal triangle order does not always match the original (user-defined) order. The remapping table helps finding the old indices knowing the new ones:
remapTable[ internalTriangleIndex ] = originalTriangleIndex
- Returns:
- the remapping table (or NULL if 'PxCookingParamssuppressTriangleMeshRemapTable' has been used)
virtual const PxVec3* PxTriangleMesh::getVertices | ( | ) | const [pure virtual] |
virtual PxVec3* PxTriangleMesh::getVerticesForModification | ( | ) | [pure virtual] |
Returns all mesh vertices for modification.
This function will return the vertices of the mesh so that their positions can be changed in place. After modifying the vertices you must call refitBVH for the refitting to actually take place. This function maintains the old mesh topology (triangle indices).
- Returns:
- inplace vertex coordinates for each existing mesh vertex.
- Note:
- works only for PxMeshMidPhase::eBVH33
Size of array returned is equal to the number returned by getNbVertices().
This function operates on cooked vertex indices.
This means the index mapping and vertex count can be different from what was provided as an input to the cooking routine.
To achieve unchanged 1-to-1 index mapping with orignal mesh data (before cooking) please use the following cooking flags:
eWELD_VERTICES = 0, eDISABLE_CLEAN_MESH = 1.
It is also recommended to make sure that a call to validateTriangleMesh returns true if mesh cleaning is disabled.
- See also:
- getNbVertices()
virtual bool PxTriangleMesh::isKindOf | ( | const char * | superClass | ) | const [inline, protected, virtual] |
Returns whether a given type name matches with the type of this instance.
Reimplemented from PxBase.
Reimplemented in PxBVH33TriangleMesh, and PxBVH34TriangleMesh.
References PxBase::isKindOf().
Referenced by PxBVH34TriangleMesh::isKindOf(), and PxBVH33TriangleMesh::isKindOf().
virtual PxBounds3 PxTriangleMesh::refitBVH | ( | ) | [pure virtual] |
Refits BVH for mesh vertices.
This function will refit the mesh BVH to correctly enclose the new positions updated by getVerticesForModification. Mesh BVH will not be reoptimized by this function so significantly different new positions will cause significantly reduced performance.
- Returns:
- New bounds for the entire mesh.
- Note:
- works only for PxMeshMidPhase::eBVH33
PhysX does not keep a mapping from the mesh to mesh shapes that reference it.
Call PxShape::setGeometry on each shape which references the mesh, to ensure that internal data structures are updated to reflect the new geometry.
PxShape::setGeometry does not guarantee correct/continuous behavior when objects are resting on top of old or new geometry.
It is also recommended to make sure that a call to validateTriangleMesh returns true if mesh cleaning is disabled.
Active edges information will be lost during refit, the rigid body mesh contact generation might not perform as expected.
virtual void PxTriangleMesh::release | ( | ) | [pure virtual] |
Decrements the reference count of a triangle mesh and releases it if the new reference count is zero.
- See also:
- PxPhysics.createTriangleMesh()
Implements PxBase.
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com