PxActor Class Reference
[Physics]
PxActor is the base class for the main simulation objects in the physics SDK.
More...
#include <PxActor.h>
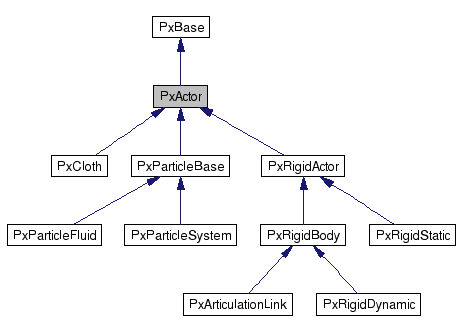
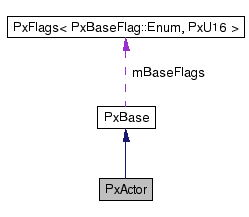
Public Member Functions | |
virtual void | release ()=0 |
Deletes the actor. | |
virtual PxActorType::Enum | getType () const =0 |
Retrieves the type of actor. | |
virtual PxScene * | getScene () const =0 |
Retrieves the scene which this actor belongs to. | |
virtual void | setName (const char *name)=0 |
Sets a name string for the object that can be retrieved with getName(). | |
virtual const char * | getName () const =0 |
Retrieves the name string set with setName(). | |
virtual PxBounds3 | getWorldBounds (float inflation=1.01f) const =0 |
Retrieves the axis aligned bounding box enclosing the actor. | |
virtual void | setActorFlag (PxActorFlag::Enum flag, bool value)=0 |
Raises or clears a particular actor flag. | |
virtual void | setActorFlags (PxActorFlags inFlags)=0 |
sets the actor flags | |
virtual PxActorFlags | getActorFlags () const =0 |
Reads the PxActor flags. | |
virtual void | setDominanceGroup (PxDominanceGroup dominanceGroup)=0 |
Assigns dynamic actors a dominance group identifier. | |
virtual PxDominanceGroup | getDominanceGroup () const =0 |
Retrieves the value set with setDominanceGroup(). | |
virtual void | setOwnerClient (PxClientID inClient)=0 |
Sets the owner client of an actor. | |
virtual PxClientID | getOwnerClient () const =0 |
Returns the owner client that was specified with at creation time. | |
virtual PX_DEPRECATED void | setClientBehaviorFlags (PxActorClientBehaviorFlags)=0 |
Sets the behavior bits of the actor. | |
virtual PX_DEPRECATED PxActorClientBehaviorFlags | getClientBehaviorFlags () const =0 |
Retrieves the behavior bits of the actor. | |
virtual PxAggregate * | getAggregate () const =0 |
Retrieves the aggregate the actor might be a part of. | |
Public Attributes | |
void * | userData |
user can assign this to whatever, usually to create a 1:1 relationship with a user object. | |
Protected Member Functions | |
PX_INLINE | PxActor (PxType concreteType, PxBaseFlags baseFlags) |
PX_INLINE | PxActor (PxBaseFlags baseFlags) |
virtual | ~PxActor () |
virtual bool | isKindOf (const char *name) const |
Returns whether a given type name matches with the type of this instance. |
Detailed Description
PxActor is the base class for the main simulation objects in the physics SDK.The actor is owned by and contained in a PxScene.
Constructor & Destructor Documentation
PX_INLINE PxActor::PxActor | ( | PxType | concreteType, | |
PxBaseFlags | baseFlags | |||
) | [inline, protected] |
PX_INLINE PxActor::PxActor | ( | PxBaseFlags | baseFlags | ) | [inline, protected] |
virtual PxActor::~PxActor | ( | ) | [inline, protected, virtual] |
Member Function Documentation
virtual PxActorFlags PxActor::getActorFlags | ( | ) | const [pure virtual] |
Reads the PxActor flags.
See the list of flags PxActorFlag
- Returns:
- The values of the PxActor flags.
- See also:
- PxActorFlag setActorFlag()
virtual PxAggregate* PxActor::getAggregate | ( | ) | const [pure virtual] |
Retrieves the aggregate the actor might be a part of.
- Returns:
- The aggregate the actor is a part of, or NULL if the actor does not belong to an aggregate.
- See also:
- PxAggregate
virtual PX_DEPRECATED PxActorClientBehaviorFlags PxActor::getClientBehaviorFlags | ( | ) | const [pure virtual] |
Retrieves the behavior bits of the actor.
The behavior bits determine which types of events the actor will broadcast to foreign clients.
- Deprecated:
- PxActorClientBehaviorFlag feature has been deprecated in PhysX version 3.4
virtual PxDominanceGroup PxActor::getDominanceGroup | ( | ) | const [pure virtual] |
virtual const char* PxActor::getName | ( | ) | const [pure virtual] |
virtual PxClientID PxActor::getOwnerClient | ( | ) | const [pure virtual] |
Returns the owner client that was specified with at creation time.
This value cannot be changed once the object is placed into the scene.
- See also:
- PxClientID PxScene::createClient()
virtual PxScene* PxActor::getScene | ( | ) | const [pure virtual] |
Retrieves the scene which this actor belongs to.
- Returns:
- Owner Scene. NULL if not part of a scene.
- See also:
- PxScene
virtual PxActorType::Enum PxActor::getType | ( | ) | const [pure virtual] |
virtual PxBounds3 PxActor::getWorldBounds | ( | float | inflation = 1.01f |
) | const [pure virtual] |
virtual bool PxActor::isKindOf | ( | const char * | superClass | ) | const [inline, protected, virtual] |
Returns whether a given type name matches with the type of this instance.
Reimplemented from PxBase.
Reimplemented in PxArticulationLink, PxRigidActor, PxRigidBody, PxRigidDynamic, PxRigidStatic, PxCloth, PxParticleBase, PxParticleFluid, and PxParticleSystem.
References PxBase::isKindOf().
Referenced by PxRigidActor::isKindOf(), PxParticleBase::isKindOf(), and PxCloth::isKindOf().
virtual void PxActor::release | ( | ) | [pure virtual] |
Deletes the actor.
Do not keep a reference to the deleted instance.
If the actor belongs to a PxAggregate object, it is automatically removed from the aggregate.
- See also:
- PxBase.release(), PxAggregate
Implements PxBase.
Implemented in PxArticulationLink, PxRigidActor, and PxCloth.
virtual void PxActor::setActorFlag | ( | PxActorFlag::Enum | flag, | |
bool | value | |||
) | [pure virtual] |
Raises or clears a particular actor flag.
See the list of flags PxActorFlag
Sleeping: Does NOT wake the actor up automatically.
- Parameters:
-
[in] flag The PxActor flag to raise(set) or clear. See PxActorFlag. [in] value The boolean value to assign to the flag.
- See also:
- PxActorFlag getActorFlags()
virtual void PxActor::setActorFlags | ( | PxActorFlags | inFlags | ) | [pure virtual] |
virtual PX_DEPRECATED void PxActor::setClientBehaviorFlags | ( | PxActorClientBehaviorFlags | ) | [pure virtual] |
Sets the behavior bits of the actor.
The behavior bits determine which types of events the actor will broadcast to foreign clients. The actor will always send notice for all possible events to its own owner client. By default it will not send any events to any other clients. If the user however raises a bit flag for any event type using this function, that event will then be sent also to any other clients which are programmed to listed to foreign actor events of that type.
- Deprecated:
- PxActorClientBehaviorFlag feature has been deprecated in PhysX version 3.4
virtual void PxActor::setDominanceGroup | ( | PxDominanceGroup | dominanceGroup | ) | [pure virtual] |
Assigns dynamic actors a dominance group identifier.
PxDominanceGroup is a 5 bit group identifier (legal range from 0 to 31).
The PxScene::setDominanceGroupPair() lets you set certain behaviors for pairs of dominance groups. By default every dynamic actor is created in group 0.
Default: 0
Sleeping: Changing the dominance group does NOT wake the actor up automatically.
- Parameters:
-
[in] dominanceGroup The dominance group identifier. Range: [0..31]
virtual void PxActor::setName | ( | const char * | name | ) | [pure virtual] |
virtual void PxActor::setOwnerClient | ( | PxClientID | inClient | ) | [pure virtual] |
Sets the owner client of an actor.
This cannot be done once the actor has been placed into a scene.
Default: PX_DEFAULT_CLIENT
- See also:
- PxClientID PxScene::createClient()
Member Data Documentation
void* PxActor::userData |
user can assign this to whatever, usually to create a 1:1 relationship with a user object.
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com