PxJoint Class Reference
[Extensions]
a base interface providing common functionality for PhysX joints
More...
#include <PxJoint.h>
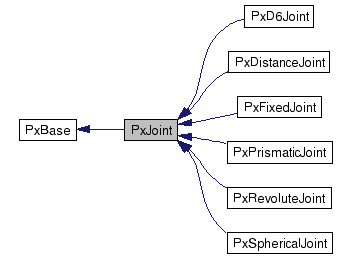
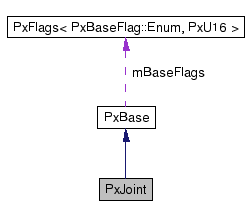
Public Member Functions | |
virtual void | setActors (PxRigidActor *actor0, PxRigidActor *actor1)=0 |
Set the actors for this joint. | |
virtual void | getActors (PxRigidActor *&actor0, PxRigidActor *&actor1) const =0 |
Get the actors for this joint. | |
virtual void | setLocalPose (PxJointActorIndex::Enum actor, const PxTransform &localPose)=0 |
Set the joint local pose for an actor. | |
virtual PxTransform | getLocalPose (PxJointActorIndex::Enum actor) const =0 |
get the joint local pose for an actor. | |
virtual PxTransform | getRelativeTransform () const =0 |
get the relative pose for this joint | |
virtual PxVec3 | getRelativeLinearVelocity () const =0 |
get the relative linear velocity of the joint | |
virtual PxVec3 | getRelativeAngularVelocity () const =0 |
get the relative angular velocity of the joint | |
virtual void | setBreakForce (PxReal force, PxReal torque)=0 |
set the break force for this joint. | |
virtual void | getBreakForce (PxReal &force, PxReal &torque) const =0 |
get the break force for this joint. | |
virtual void | setConstraintFlags (PxConstraintFlags flags)=0 |
set the constraint flags for this joint. | |
virtual void | setConstraintFlag (PxConstraintFlag::Enum flag, bool value)=0 |
set a constraint flags for this joint to a specified value. | |
virtual PxConstraintFlags | getConstraintFlags () const =0 |
get the constraint flags for this joint. | |
virtual void | setInvMassScale0 (PxReal invMassScale)=0 |
set the inverse mass scale for actor0. | |
virtual PxReal | getInvMassScale0 () const =0 |
get the inverse mass scale for actor0. | |
virtual void | setInvInertiaScale0 (PxReal invInertiaScale)=0 |
set the inverse inertia scale for actor0. | |
virtual PxReal | getInvInertiaScale0 () const =0 |
get the inverse inertia scale for actor0. | |
virtual void | setInvMassScale1 (PxReal invMassScale)=0 |
set the inverse mass scale for actor1. | |
virtual PxReal | getInvMassScale1 () const =0 |
get the inverse mass scale for actor1. | |
virtual void | setInvInertiaScale1 (PxReal invInertiaScale)=0 |
set the inverse inertia scale for actor1. | |
virtual PxReal | getInvInertiaScale1 () const =0 |
get the inverse inertia scale for actor1. | |
virtual PxConstraint * | getConstraint () const =0 |
Retrieves the PxConstraint corresponding to this joint. | |
virtual void | setName (const char *name)=0 |
Sets a name string for the object that can be retrieved with getName(). | |
virtual const char * | getName () const =0 |
Retrieves the name string set with setName(). | |
virtual void | release ()=0 |
Deletes the joint. | |
virtual PxScene * | getScene () const =0 |
Retrieves the scene which this joint belongs to. | |
Static Public Member Functions | |
static void | getBinaryMetaData (PxOutputStream &stream) |
Put class meta data in stream, used for serialization. | |
Public Attributes | |
void * | userData |
user can assign this to whatever, usually to create a 1:1 relationship with a user object. | |
Protected Member Functions | |
virtual | ~PxJoint () |
PX_INLINE | PxJoint (PxType concreteType, PxBaseFlags baseFlags) |
Constructor. | |
PX_INLINE | PxJoint (PxBaseFlags baseFlags) |
Deserialization constructor. | |
virtual bool | isKindOf (const char *name) const |
Returns whether a given type name matches with the type of this instance. |
Detailed Description
a base interface providing common functionality for PhysX jointsConstructor & Destructor Documentation
virtual PxJoint::~PxJoint | ( | ) | [inline, protected, virtual] |
PX_INLINE PxJoint::PxJoint | ( | PxType | concreteType, | |
PxBaseFlags | baseFlags | |||
) | [inline, protected] |
Constructor.
PX_INLINE PxJoint::PxJoint | ( | PxBaseFlags | baseFlags | ) | [inline, protected] |
Deserialization constructor.
Member Function Documentation
virtual void PxJoint::getActors | ( | PxRigidActor *& | actor0, | |
PxRigidActor *& | actor1 | |||
) | const [pure virtual] |
Get the actors for this joint.
- Parameters:
-
[out] actor0 the first actor. [out] actor1 the second actor
- See also:
- setActors()
static void PxJoint::getBinaryMetaData | ( | PxOutputStream & | stream | ) | [static] |
Put class meta data in stream, used for serialization.
virtual void PxJoint::getBreakForce | ( | PxReal & | force, | |
PxReal & | torque | |||
) | const [pure virtual] |
get the break force for this joint.
- Parameters:
-
[out] force the maximum force the joint can apply before breaking [out] torque the maximum torque the joint can apply before breaking
- See also:
- setBreakForce()
virtual PxConstraint* PxJoint::getConstraint | ( | ) | const [pure virtual] |
Retrieves the PxConstraint corresponding to this joint.
This can be used to determine, among other things, the force applied at the joint.
- Returns:
- the constraint
virtual PxConstraintFlags PxJoint::getConstraintFlags | ( | ) | const [pure virtual] |
virtual PxReal PxJoint::getInvInertiaScale0 | ( | ) | const [pure virtual] |
get the inverse inertia scale for actor0.
- Returns:
- inverse inertia scale for actor0
- See also:
- setInvInertiaScale0
virtual PxReal PxJoint::getInvInertiaScale1 | ( | ) | const [pure virtual] |
get the inverse inertia scale for actor1.
- Returns:
- inverse inertia scale for actor1
- See also:
- setInvInertiaScale1
virtual PxReal PxJoint::getInvMassScale0 | ( | ) | const [pure virtual] |
get the inverse mass scale for actor0.
- Returns:
- inverse mass scale for actor0
- See also:
- setInvMassScale0
virtual PxReal PxJoint::getInvMassScale1 | ( | ) | const [pure virtual] |
get the inverse mass scale for actor1.
- Returns:
- inverse mass scale for actor1
- See also:
- setInvMassScale1
virtual PxTransform PxJoint::getLocalPose | ( | PxJointActorIndex::Enum | actor | ) | const [pure virtual] |
get the joint local pose for an actor.
- Parameters:
-
[in] actor 0 for the first actor, 1 for the second actor.
- See also:
- setLocalPose()
virtual const char* PxJoint::getName | ( | ) | const [pure virtual] |
virtual PxVec3 PxJoint::getRelativeAngularVelocity | ( | ) | const [pure virtual] |
get the relative angular velocity of the joint
This function returns the angular velocity of actor1 relative to actor0. The value is returned in the constraint frame of actor0
virtual PxVec3 PxJoint::getRelativeLinearVelocity | ( | ) | const [pure virtual] |
get the relative linear velocity of the joint
This function returns the linear velocity of the origin of the constraint frame of actor1, relative to the origin of the constraint frame of actor0. The value is returned in the constraint frame of actor0
virtual PxTransform PxJoint::getRelativeTransform | ( | ) | const [pure virtual] |
get the relative pose for this joint
This function returns the pose of the joint frame of actor1 relative to actor0
virtual PxScene* PxJoint::getScene | ( | ) | const [pure virtual] |
Retrieves the scene which this joint belongs to.
- Returns:
- Owner Scene. NULL if not part of a scene.
- See also:
- PxScene
virtual bool PxJoint::isKindOf | ( | const char * | name | ) | const [inline, protected, virtual] |
Returns whether a given type name matches with the type of this instance.
Reimplemented from PxBase.
Reimplemented in PxD6Joint, PxDistanceJoint, PxFixedJoint, PxPrismaticJoint, PxRevoluteJoint, and PxSphericalJoint.
References PxBase::isKindOf().
Referenced by PxSphericalJoint::isKindOf(), PxRevoluteJoint::isKindOf(), PxPrismaticJoint::isKindOf(), PxFixedJoint::isKindOf(), PxDistanceJoint::isKindOf(), and PxD6Joint::isKindOf().
virtual void PxJoint::release | ( | ) | [pure virtual] |
virtual void PxJoint::setActors | ( | PxRigidActor * | actor0, | |
PxRigidActor * | actor1 | |||
) | [pure virtual] |
Set the actors for this joint.
An actor may be NULL to indicate the world frame. At most one of the actors may be NULL.
- Parameters:
-
[in] actor0 the first actor. [in] actor1 the second actor
- See also:
- getActors()
virtual void PxJoint::setBreakForce | ( | PxReal | force, | |
PxReal | torque | |||
) | [pure virtual] |
set the break force for this joint.
if the constraint force or torque on the joint exceeds the specified values, the joint will break, at which point it will not constrain the two actors and the flag PxConstraintFlag::eBROKEN will be set. The force and torque are measured in the joint frame of the first actor
- Parameters:
-
[in] force the maximum force the joint can apply before breaking [in] torque the maximum torque the joint can apply before breaking
virtual void PxJoint::setConstraintFlag | ( | PxConstraintFlag::Enum | flag, | |
bool | value | |||
) | [pure virtual] |
set a constraint flags for this joint to a specified value.
- Parameters:
-
[in] flag the constraint flag [in] value the value to which to set the flag
- See also:
- PxConstraintFlag
virtual void PxJoint::setConstraintFlags | ( | PxConstraintFlags | flags | ) | [pure virtual] |
set the constraint flags for this joint.
- Parameters:
-
[in] flags the constraint flags
- See also:
- PxConstraintFlag
virtual void PxJoint::setInvInertiaScale0 | ( | PxReal | invInertiaScale | ) | [pure virtual] |
set the inverse inertia scale for actor0.
- Parameters:
-
[in] invInertiaScale the scale to apply to the inverse inertia of actor0 for resolving this constraint
- See also:
- getInvMassScale0
virtual void PxJoint::setInvInertiaScale1 | ( | PxReal | invInertiaScale | ) | [pure virtual] |
set the inverse inertia scale for actor1.
- Parameters:
-
[in] invInertiaScale the scale to apply to the inverse inertia of actor1 for resolving this constraint
- See also:
- getInvInertiaScale1
virtual void PxJoint::setInvMassScale0 | ( | PxReal | invMassScale | ) | [pure virtual] |
set the inverse mass scale for actor0.
- Parameters:
-
[in] invMassScale the scale to apply to the inverse mass of actor 0 for resolving this constraint
- See also:
- getInvMassScale0
virtual void PxJoint::setInvMassScale1 | ( | PxReal | invMassScale | ) | [pure virtual] |
set the inverse mass scale for actor1.
- Parameters:
-
[in] invMassScale the scale to apply to the inverse mass of actor 1 for resolving this constraint
- See also:
- getInvMassScale1
virtual void PxJoint::setLocalPose | ( | PxJointActorIndex::Enum | actor, | |
const PxTransform & | localPose | |||
) | [pure virtual] |
Set the joint local pose for an actor.
This is the relative pose which locates the joint frame relative to the actor.
- Parameters:
-
[in] actor 0 for the first actor, 1 for the second actor. [in] localPose the local pose for the actor this joint
- See also:
- getLocalPose()
virtual void PxJoint::setName | ( | const char * | name | ) | [pure virtual] |
Member Data Documentation
void* PxJoint::userData |
user can assign this to whatever, usually to create a 1:1 relationship with a user object.
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com