PxTransform Class Reference
[Foundation]
class representing a rigid euclidean transform as a quaternion and a vector
More...
#include <PxTransform.h>
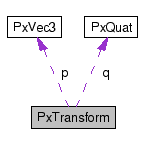
Public Member Functions | |
PX_CUDA_CALLABLE PX_FORCE_INLINE | PxTransform () |
PX_CUDA_CALLABLE PX_FORCE_INLINE | PxTransform (const PxVec3 &position) |
PX_CUDA_CALLABLE PX_FORCE_INLINE | PxTransform (PxIDENTITY r) |
PX_CUDA_CALLABLE PX_FORCE_INLINE | PxTransform (const PxQuat &orientation) |
PX_CUDA_CALLABLE PX_FORCE_INLINE | PxTransform (float x, float y, float z, PxQuat aQ=PxQuat(PxIdentity)) |
PX_CUDA_CALLABLE PX_FORCE_INLINE | PxTransform (const PxVec3 &p0, const PxQuat &q0) |
PX_CUDA_CALLABLE PX_FORCE_INLINE | PxTransform (const PxMat44 &m) |
PX_CUDA_CALLABLE PX_INLINE bool | operator== (const PxTransform &t) const |
returns true if the two transforms are exactly equal | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxTransform | operator* (const PxTransform &x) const |
PX_CUDA_CALLABLE PX_INLINE PxTransform & | operator*= (PxTransform &other) |
Equals matrix multiplication. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxTransform | getInverse () const |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxVec3 | transform (const PxVec3 &input) const |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxVec3 | transformInv (const PxVec3 &input) const |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxVec3 | rotate (const PxVec3 &input) const |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxVec3 | rotateInv (const PxVec3 &input) const |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxTransform | transform (const PxTransform &src) const |
Transform transform to parent (returns compound transform: first src, then *this). | |
PX_CUDA_CALLABLE bool | isValid () const |
returns true if finite and q is a unit quaternion | |
PX_CUDA_CALLABLE bool | isSane () const |
returns true if finite and quat magnitude is reasonably close to unit to allow for some accumulation of error vs isValid | |
PX_CUDA_CALLABLE PX_FORCE_INLINE bool | isFinite () const |
returns true if all elems are finite (not NAN or INF, etc.) | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxTransform | transformInv (const PxTransform &src) const |
Transform transform from parent (returns compound transform: first src, then this->inverse). | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxPlane | transform (const PxPlane &plane) const |
transform plane | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxPlane | inverseTransform (const PxPlane &plane) const |
inverse-transform plane | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxTransform | getNormalized () const |
return a normalized transform (i.e. one in which the quaternion has unit magnitude) | |
Public Attributes | |
PxQuat | q |
PxVec3 | p |
Detailed Description
class representing a rigid euclidean transform as a quaternion and a vectorConstructor & Destructor Documentation
PX_CUDA_CALLABLE PX_FORCE_INLINE PxTransform::PxTransform | ( | ) | [inline] |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxTransform::PxTransform | ( | const PxVec3 & | position | ) | [inline, explicit] |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxTransform::PxTransform | ( | PxIDENTITY | r | ) | [inline, explicit] |
References PX_UNUSED().
PX_CUDA_CALLABLE PX_FORCE_INLINE PxTransform::PxTransform | ( | const PxQuat & | orientation | ) | [inline, explicit] |
References PxQuat::isSane(), and PX_ASSERT.
PX_CUDA_CALLABLE PX_FORCE_INLINE PxTransform::PxTransform | ( | float | x, | |
float | y, | |||
float | z, | |||
PxQuat | aQ = PxQuat(PxIdentity) | |||
) | [inline] |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxTransform::PxTransform | ( | const PxVec3 & | p0, | |
const PxQuat & | q0 | |||
) | [inline] |
References PxQuat::isSane(), and PX_ASSERT.
Member Function Documentation
PX_CUDA_CALLABLE PX_FORCE_INLINE PxTransform PxTransform::getInverse | ( | ) | const [inline] |
References PX_ASSERT.
PX_CUDA_CALLABLE PX_FORCE_INLINE PxTransform PxTransform::getNormalized | ( | ) | const [inline] |
return a normalized transform (i.e. one in which the quaternion has unit magnitude)
PX_CUDA_CALLABLE PX_FORCE_INLINE bool PxTransform::isFinite | ( | ) | const [inline] |
returns true if all elems are finite (not NAN or INF, etc.)
PX_CUDA_CALLABLE bool PxTransform::isSane | ( | ) | const [inline] |
returns true if finite and quat magnitude is reasonably close to unit to allow for some accumulation of error vs isValid
Referenced by operator*(), transform(), and transformInv().
PX_CUDA_CALLABLE bool PxTransform::isValid | ( | ) | const [inline] |
returns true if finite and q is a unit quaternion
PX_CUDA_CALLABLE PX_FORCE_INLINE PxTransform PxTransform::operator* | ( | const PxTransform & | x | ) | const [inline] |
PX_CUDA_CALLABLE PX_INLINE PxTransform& PxTransform::operator*= | ( | PxTransform & | other | ) | [inline] |
Equals matrix multiplication.
PX_CUDA_CALLABLE PX_INLINE bool PxTransform::operator== | ( | const PxTransform & | t | ) | const [inline] |
References PX_ASSERT.
PX_CUDA_CALLABLE PX_FORCE_INLINE PxVec3 PxTransform::rotateInv | ( | const PxVec3 & | input | ) | const [inline] |
References PX_ASSERT.
PX_CUDA_CALLABLE PX_FORCE_INLINE PxTransform PxTransform::transform | ( | const PxTransform & | src | ) | const [inline] |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxVec3 PxTransform::transform | ( | const PxVec3 & | input | ) | const [inline] |
References PX_ASSERT.
Referenced by PxPlaneEquationFromTransform(), PxMassProperties::sum(), and PxBounds3::transformFast().
PX_CUDA_CALLABLE PX_FORCE_INLINE PxTransform PxTransform::transformInv | ( | const PxTransform & | src | ) | const [inline] |
Transform transform from parent (returns compound transform: first src, then this->inverse).
References isSane(), p, PX_ASSERT, q, and PxQuat::rotate().
PX_CUDA_CALLABLE PX_FORCE_INLINE PxVec3 PxTransform::transformInv | ( | const PxVec3 & | input | ) | const [inline] |
References PX_ASSERT.
Member Data Documentation
Referenced by operator==(), PxBounds3::poseExtent(), PxMat44::PxMat44(), PxTransform(), transform(), and transformInv().
Referenced by operator==(), PxBounds3::poseExtent(), PxMat44::PxMat44(), PxTransform(), transform(), PxBounds3::transformFast(), and transformInv().
The documentation for this class was generated from the following files:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com