PxDistanceJoint Class Reference
[Extensions]
a joint that maintains an upper or lower bound (or both) on the distance between two points on different objects
More...
#include <PxDistanceJoint.h>
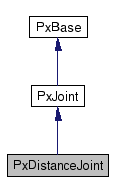
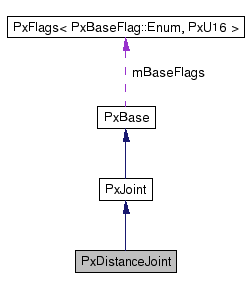
Public Member Functions | |
virtual PxReal | getDistance () const =0 |
Return the current distance of the joint. | |
virtual void | setMinDistance (PxReal distance)=0 |
Set the allowed minimum distance for the joint. | |
virtual PxReal | getMinDistance () const =0 |
Get the allowed minimum distance for the joint. | |
virtual void | setMaxDistance (PxReal distance)=0 |
Set the allowed maximum distance for the joint. | |
virtual PxReal | getMaxDistance () const =0 |
Get the allowed maximum distance for the joint. | |
virtual void | setTolerance (PxReal tolerance)=0 |
Set the error tolerance of the joint. | |
virtual PxReal | getTolerance () const =0 |
Get the error tolerance of the joint. | |
virtual void | setStiffness (PxReal stiffness)=0 |
Set the strength of the joint spring. | |
virtual PxReal | getStiffness () const =0 |
Get the strength of the joint spring. | |
virtual void | setDamping (PxReal damping)=0 |
Set the damping of the joint spring. | |
virtual PxReal | getDamping () const =0 |
Get the damping of the joint spring. | |
virtual void | setDistanceJointFlags (PxDistanceJointFlags flags)=0 |
Set the flags specific to the Distance Joint. | |
virtual void | setDistanceJointFlag (PxDistanceJointFlag::Enum flag, bool value)=0 |
Set a single flag specific to a Distance Joint to true or false. | |
virtual PxDistanceJointFlags | getDistanceJointFlags (void) const =0 |
Get the flags specific to the Distance Joint. | |
virtual const char * | getConcreteTypeName () const |
Returns string name of PxDistanceJoint, used for serialization. | |
Protected Member Functions | |
PX_INLINE | PxDistanceJoint (PxType concreteType, PxBaseFlags baseFlags) |
Constructor. | |
PX_INLINE | PxDistanceJoint (PxBaseFlags baseFlags) |
Deserialization constructor. | |
virtual bool | isKindOf (const char *name) const |
Returns whether a given type name matches with the type of this instance. |
Detailed Description
a joint that maintains an upper or lower bound (or both) on the distance between two points on different objects
- See also:
- PxDistanceJointCreate PxJoint
Constructor & Destructor Documentation
PX_INLINE PxDistanceJoint::PxDistanceJoint | ( | PxType | concreteType, | |
PxBaseFlags | baseFlags | |||
) | [inline, protected] |
Constructor.
PX_INLINE PxDistanceJoint::PxDistanceJoint | ( | PxBaseFlags | baseFlags | ) | [inline, protected] |
Deserialization constructor.
Member Function Documentation
virtual const char* PxDistanceJoint::getConcreteTypeName | ( | ) | const [inline, virtual] |
virtual PxReal PxDistanceJoint::getDamping | ( | ) | const [pure virtual] |
Get the damping of the joint spring.
- Returns:
- the degree of damping of the joint spring of the joint
virtual PxReal PxDistanceJoint::getDistance | ( | ) | const [pure virtual] |
Return the current distance of the joint.
virtual PxDistanceJointFlags PxDistanceJoint::getDistanceJointFlags | ( | void | ) | const [pure virtual] |
Get the flags specific to the Distance Joint.
- Returns:
- the joint flags
- See also:
- PxDistanceJoint::flags, PxDistanceJointFlag setFlag() setFlags()
virtual PxReal PxDistanceJoint::getMaxDistance | ( | ) | const [pure virtual] |
Get the allowed maximum distance for the joint.
- Returns:
- the allowed maximum distance
- See also:
- PxDistanceJoint::maxDistance, PxDistanceJointFlag::eMAX_DISTANCE_ENABLED setMaxDistance()
virtual PxReal PxDistanceJoint::getMinDistance | ( | ) | const [pure virtual] |
Get the allowed minimum distance for the joint.
- Returns:
- the allowed minimum distance
- See also:
- PxDistanceJoint::minDistance, PxDistanceJointFlag::eMIN_DISTANCE_ENABLED setMinDistance()
virtual PxReal PxDistanceJoint::getStiffness | ( | ) | const [pure virtual] |
Get the strength of the joint spring.
- Returns:
- stiffness the spring strength of the joint
virtual PxReal PxDistanceJoint::getTolerance | ( | ) | const [pure virtual] |
Get the error tolerance of the joint.
the distance beyond the joint's [min, max] range before the joint becomes active.
Default 0.25f * PxTolerancesScale::length Range (0, PX_MAX_F32)
This value should be used to ensure that if the minimum distance is zero and the spring function is in use, the rest length of the spring is non-zero.
- See also:
- PxDistanceJoint::tolerance, setTolerance()
virtual bool PxDistanceJoint::isKindOf | ( | const char * | name | ) | const [inline, protected, virtual] |
Returns whether a given type name matches with the type of this instance.
Reimplemented from PxJoint.
References PxJoint::isKindOf().
virtual void PxDistanceJoint::setDamping | ( | PxReal | damping | ) | [pure virtual] |
Set the damping of the joint spring.
The spring is used if enabled, and the distance exceeds the range [min-error, max+error].
Default 0.0f Range [0, PX_MAX_F32)
- Parameters:
-
[in] damping the degree of damping of the joint spring of the joint
virtual void PxDistanceJoint::setDistanceJointFlag | ( | PxDistanceJointFlag::Enum | flag, | |
bool | value | |||
) | [pure virtual] |
Set a single flag specific to a Distance Joint to true or false.
- Parameters:
-
[in] flag The flag to set or clear. [in] value the value to which to set the flag
- See also:
- PxDistanceJointFlag, getFlags() setFlags()
virtual void PxDistanceJoint::setDistanceJointFlags | ( | PxDistanceJointFlags | flags | ) | [pure virtual] |
Set the flags specific to the Distance Joint.
Default PxDistanceJointFlag::eMAX_DISTANCE_ENABLED
- Parameters:
-
[in] flags The joint flags.
- See also:
- PxDistanceJointFlag setFlag() getFlags()
virtual void PxDistanceJoint::setMaxDistance | ( | PxReal | distance | ) | [pure virtual] |
Set the allowed maximum distance for the joint.
The maximum distance must be no less than the minimum distance.
Default 0.0f Range [0, PX_MAX_F32)
- Parameters:
-
[in] distance the maximum distance
- See also:
- PxDistanceJoint::maxDistance, PxDistanceJointFlag::eMAX_DISTANCE_ENABLED getMinDistance()
virtual void PxDistanceJoint::setMinDistance | ( | PxReal | distance | ) | [pure virtual] |
Set the allowed minimum distance for the joint.
The minimum distance must be no more than the maximum distance
Default 0.0f Range [0, PX_MAX_F32)
- Parameters:
-
[in] distance the minimum distance
- See also:
- PxDistanceJoint::minDistance, PxDistanceJointFlag::eMIN_DISTANCE_ENABLED getMinDistance()
virtual void PxDistanceJoint::setStiffness | ( | PxReal | stiffness | ) | [pure virtual] |
Set the strength of the joint spring.
The spring is used if enabled, and the distance exceeds the range [min-error, max+error].
Default 0.0f Range [0, PX_MAX_F32)
- Parameters:
-
[in] stiffness the spring strength of the joint
virtual void PxDistanceJoint::setTolerance | ( | PxReal | tolerance | ) | [pure virtual] |
Set the error tolerance of the joint.
- Parameters:
-
[in] tolerance the distance beyond the allowed range at which the joint becomes active
- See also:
- PxDistanceJoint::tolerance, getTolerance()
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com