PxD6Joint Class Reference
[Extensions]
A D6 joint is a general constraint between two actors.
More...
#include <PxD6Joint.h>
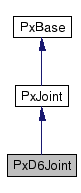
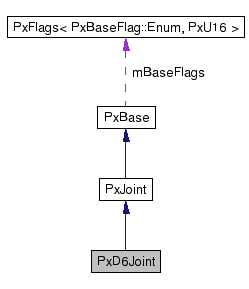
Public Member Functions | |
virtual void | setMotion (PxD6Axis::Enum axis, PxD6Motion::Enum type)=0 |
Set the motion type around the specified axis. | |
virtual PxD6Motion::Enum | getMotion (PxD6Axis::Enum axis) const =0 |
Get the motion type around the specified axis. | |
virtual PxReal | getTwist () const =0 |
get the twist angle of the joint | |
virtual PxReal | getSwingYAngle () const =0 |
get the swing angle of the joint from the Y axis | |
virtual PxReal | getSwingZAngle () const =0 |
get the swing angle of the joint from the Z axis | |
virtual void | setLinearLimit (const PxJointLinearLimit &limit)=0 |
Set the linear limit for the joint. | |
virtual PxJointLinearLimit | getLinearLimit () const =0 |
Get the linear limit for the joint. | |
virtual void | setTwistLimit (const PxJointAngularLimitPair &limit)=0 |
Set the twist limit for the joint. | |
virtual PxJointAngularLimitPair | getTwistLimit () const =0 |
Get the twist limit for the joint. | |
virtual void | setSwingLimit (const PxJointLimitCone &limit)=0 |
Set the swing cone limit for the joint. | |
virtual PxJointLimitCone | getSwingLimit () const =0 |
Get the cone limit for the joint. | |
virtual void | setDrive (PxD6Drive::Enum index, const PxD6JointDrive &drive)=0 |
Set the drive parameters for the specified drive type. | |
virtual PxD6JointDrive | getDrive (PxD6Drive::Enum index) const =0 |
Get the drive parameters for the specified drive type. | |
virtual void | setDrivePosition (const PxTransform &pose)=0 |
Set the drive goal pose. | |
virtual PxTransform | getDrivePosition () const =0 |
Get the drive goal pose. | |
virtual void | setDriveVelocity (const PxVec3 &linear, const PxVec3 &angular)=0 |
Set the target goal velocity for drive. | |
virtual void | getDriveVelocity (PxVec3 &linear, PxVec3 &angular) const =0 |
Get the target goal velocity for joint drive. | |
virtual void | setProjectionLinearTolerance (PxReal tolerance)=0 |
Set the linear tolerance threshold for projection. Projection is enabled if PxConstraintFlag::ePROJECTION is set for the joint. | |
virtual PxReal | getProjectionLinearTolerance () const =0 |
Get the linear tolerance threshold for projection. | |
virtual void | setProjectionAngularTolerance (PxReal tolerance)=0 |
Set the angular tolerance threshold for projection. Projection is enabled if PxConstraintFlag::ePROJECTION is set for the joint. | |
virtual PxReal | getProjectionAngularTolerance () const =0 |
Get the angular tolerance threshold for projection. | |
virtual const char * | getConcreteTypeName () const |
Returns string name of PxD6Joint, used for serialization. | |
Protected Member Functions | |
PX_INLINE | PxD6Joint (PxType concreteType, PxBaseFlags baseFlags) |
Constructor. | |
PX_INLINE | PxD6Joint (PxBaseFlags baseFlags) |
Deserialization constructor. | |
virtual bool | isKindOf (const char *name) const |
Returns whether a given type name matches with the type of this instance. |
Detailed Description
A D6 joint is a general constraint between two actors.It allows the application to individually define the linear and rotational degrees of freedom, and also to configure a variety of limits and driven degrees of freedom.
By default all degrees of freedom are locked. So to create a prismatic joint with free motion along the x-axis:
... joint->setMotion(PxD6Axis::eX, PxD6JointMotion::eFREE); ...
Or a Revolute joint with motion free allowed around the x-axis:
... joint->setMotion(PxD6Axis::eTWIST, PxD6JointMotion::eFREE); ...
Degrees of freedom may also be set to limited instead of locked. There is a single limit value for all linear degrees of freedom, which may act as a linear, circular, or spherical limit depending on which degrees of freedom are limited.
If the twist degree of freedom is limited, is supports upper and lower limits. The two swing degrees of freedom are limited with a cone limit.
- See also:
- PxD6JointCreate() PxJoint
Constructor & Destructor Documentation
PX_INLINE PxD6Joint::PxD6Joint | ( | PxType | concreteType, | |
PxBaseFlags | baseFlags | |||
) | [inline, protected] |
Constructor.
PX_INLINE PxD6Joint::PxD6Joint | ( | PxBaseFlags | baseFlags | ) | [inline, protected] |
Deserialization constructor.
Member Function Documentation
virtual const char* PxD6Joint::getConcreteTypeName | ( | ) | const [inline, virtual] |
virtual PxD6JointDrive PxD6Joint::getDrive | ( | PxD6Drive::Enum | index | ) | const [pure virtual] |
Get the drive parameters for the specified drive type.
- Parameters:
-
[in] index the specified drive type
- See also:
- setDrive() PxD6JointDrive
virtual PxTransform PxD6Joint::getDrivePosition | ( | ) | const [pure virtual] |
Get the target goal velocity for joint drive.
- Parameters:
-
[in] linear The goal velocity for linear drive [in] angular The goal velocity for angular drive
- See also:
- setDriveVelocity()
virtual PxJointLinearLimit PxD6Joint::getLinearLimit | ( | ) | const [pure virtual] |
Get the linear limit for the joint.
- Returns:
- the linear limit structure
- See also:
- setLinearLimit() PxJointLinearLimit
virtual PxD6Motion::Enum PxD6Joint::getMotion | ( | PxD6Axis::Enum | axis | ) | const [pure virtual] |
Get the motion type around the specified axis.
- See also:
- setMotion() PxD6Axis PxD6Motion
- Parameters:
-
[in] axis the degree of freedom around which the motion type is specified
- Returns:
- the motion type around the specified axis
virtual PxReal PxD6Joint::getProjectionAngularTolerance | ( | ) | const [pure virtual] |
Get the angular tolerance threshold for projection.
- Returns:
- tolerance the angular tolerance threshold in radians
- See also:
- setProjectionAngularTolerance()
virtual PxReal PxD6Joint::getProjectionLinearTolerance | ( | ) | const [pure virtual] |
Get the linear tolerance threshold for projection.
- Returns:
- the linear tolerance threshold
- See also:
- setProjectionLinearTolerance()
virtual PxJointLimitCone PxD6Joint::getSwingLimit | ( | ) | const [pure virtual] |
Get the cone limit for the joint.
- Returns:
- the swing limit structure
- See also:
- setLimitCone() PxJointLimitCone
virtual PxReal PxD6Joint::getSwingYAngle | ( | ) | const [pure virtual] |
get the swing angle of the joint from the Y axis
virtual PxReal PxD6Joint::getSwingZAngle | ( | ) | const [pure virtual] |
get the swing angle of the joint from the Z axis
virtual PxReal PxD6Joint::getTwist | ( | ) | const [pure virtual] |
get the twist angle of the joint
virtual PxJointAngularLimitPair PxD6Joint::getTwistLimit | ( | ) | const [pure virtual] |
Get the twist limit for the joint.
- Returns:
- the twist limit structure
- See also:
- setTwistLimit() PxJointAngularLimitPair
virtual bool PxD6Joint::isKindOf | ( | const char * | name | ) | const [inline, protected, virtual] |
Returns whether a given type name matches with the type of this instance.
Reimplemented from PxJoint.
References PxJoint::isKindOf().
virtual void PxD6Joint::setDrive | ( | PxD6Drive::Enum | index, | |
const PxD6JointDrive & | drive | |||
) | [pure virtual] |
Set the drive parameters for the specified drive type.
- Parameters:
-
[in] index the type of drive being specified [in] drive the drive parameters
- See also:
- getDrive() PxD6JointDrive
virtual void PxD6Joint::setDrivePosition | ( | const PxTransform & | pose | ) | [pure virtual] |
Set the drive goal pose.
The goal is relative to the constraint frame of actor[0]
Default the identity transform
- Parameters:
-
[in] pose The goal drive pose if positional drive is in use.
- See also:
- setDrivePosition()
virtual void PxD6Joint::setDriveVelocity | ( | const PxVec3 & | linear, | |
const PxVec3 & | angular | |||
) | [pure virtual] |
Set the target goal velocity for drive.
The velocity is measured in the constraint frame of actor[0]
- Parameters:
-
[in] linear The goal velocity for linear drive [in] angular The goal velocity for angular drive
- See also:
- getDriveVelocity()
virtual void PxD6Joint::setLinearLimit | ( | const PxJointLinearLimit & | limit | ) | [pure virtual] |
Set the linear limit for the joint.
A single limit constraints all linear limited degrees of freedom, forming a linear, circular or spherical constraint on motion depending on the number of limited degrees.
- Parameters:
-
[in] limit the linear limit structure
- See also:
- getLinearLimit()
virtual void PxD6Joint::setMotion | ( | PxD6Axis::Enum | axis, | |
PxD6Motion::Enum | type | |||
) | [pure virtual] |
Set the motion type around the specified axis.
Each axis may independently specify that the degree of freedom is locked (blocking relative movement along or around this axis), limited by the corresponding limit, or free.
- Parameters:
-
[in] axis the axis around which motion is specified [in] type the motion type around the specified axis
- See also:
- getMotion() PxD6Axis PxD6Motion
virtual void PxD6Joint::setProjectionAngularTolerance | ( | PxReal | tolerance | ) | [pure virtual] |
Set the angular tolerance threshold for projection. Projection is enabled if PxConstraintFlag::ePROJECTION is set for the joint.
If the joint deviates by more than this angle around its locked angular degrees of freedom, the solver will move the bodies to close the angle.
Setting a very small tolerance may result in simulation jitter or other artifacts.
Sometimes it is not possible to project (for example when the joints form a cycle).
Range: [0,Pi]
Default: Pi
- Parameters:
-
[in] tolerance the angular tolerance threshold in radians
- Note:
- Angular projection is implemented only for the case of two or three locked angular degrees of freedom.
virtual void PxD6Joint::setProjectionLinearTolerance | ( | PxReal | tolerance | ) | [pure virtual] |
Set the linear tolerance threshold for projection. Projection is enabled if PxConstraintFlag::ePROJECTION is set for the joint.
If the joint separates by more than this distance along its locked degrees of freedom, the solver will move the bodies to close the distance.
Setting a very small tolerance may result in simulation jitter or other artifacts.
Sometimes it is not possible to project (for example when the joints form a cycle).
Range: [0, PX_MAX_F32)
Default: 1e10f
- Parameters:
-
[in] tolerance the linear tolerance threshold
virtual void PxD6Joint::setSwingLimit | ( | const PxJointLimitCone & | limit | ) | [pure virtual] |
Set the swing cone limit for the joint.
The cone limit is used if either or both swing axes are limited. The extents are symmetrical and measured in the frame of the parent. If only one swing degree of freedom is limited, the corresponding value from the cone limit defines the limit range.
- Parameters:
-
[in] limit the cone limit structure
- See also:
- getLimitCone() PxJointLimitCone
virtual void PxD6Joint::setTwistLimit | ( | const PxJointAngularLimitPair & | limit | ) | [pure virtual] |
Set the twist limit for the joint.
The twist limit controls the range of motion around the twist axis.
The limit angle range is (-2*PI, 2*PI) and the extent of the limit must be strictly less than 2*PI
- Parameters:
-
[in] limit the twist limit structure
- See also:
- getTwistLimit() PxJointAngularLimitPair
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com