PxRevoluteJoint Class Reference
[Extensions]
A joint which behaves in a similar way to a hinge or axle.
More...
#include <PxRevoluteJoint.h>
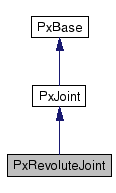
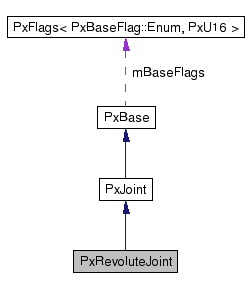
Public Member Functions | |
virtual PxReal | getAngle () const =0 |
return the angle of the joint, in the range (-Pi, Pi] | |
virtual PxReal | getVelocity () const =0 |
return the velocity of the joint | |
virtual void | setLimit (const PxJointAngularLimitPair &limits)=0 |
set the joint limit parameters. | |
virtual PxJointAngularLimitPair | getLimit () const =0 |
get the joint limit parameters. | |
virtual void | setDriveVelocity (PxReal velocity)=0 |
set the target velocity for the drive model. | |
virtual PxReal | getDriveVelocity () const =0 |
gets the target velocity for the drive model. | |
virtual void | setDriveForceLimit (PxReal limit)=0 |
sets the maximum torque the drive can exert. | |
virtual PxReal | getDriveForceLimit () const =0 |
gets the maximum torque the drive can exert. | |
virtual void | setDriveGearRatio (PxReal ratio)=0 |
sets the gear ratio for the drive. | |
virtual PxReal | getDriveGearRatio () const =0 |
gets the gear ratio. | |
virtual void | setRevoluteJointFlags (PxRevoluteJointFlags flags)=0 |
sets the flags specific to the Revolute Joint. | |
virtual void | setRevoluteJointFlag (PxRevoluteJointFlag::Enum flag, bool value)=0 |
sets a single flag specific to a Revolute Joint. | |
virtual PxRevoluteJointFlags | getRevoluteJointFlags (void) const =0 |
gets the flags specific to the Revolute Joint. | |
virtual void | setProjectionLinearTolerance (PxReal tolerance)=0 |
Set the linear tolerance threshold for projection. Projection is enabled if PxConstraintFlag::ePROJECTION is set for the joint. | |
virtual PxReal | getProjectionLinearTolerance () const =0 |
Get the linear tolerance threshold for projection. | |
virtual void | setProjectionAngularTolerance (PxReal tolerance)=0 |
Set the angular tolerance threshold for projection. Projection is enabled if PxConstraintFlag::ePROJECTION is set for the joint. | |
virtual PxReal | getProjectionAngularTolerance () const =0 |
gets the angular tolerance threshold for projection. | |
virtual const char * | getConcreteTypeName () const |
Returns string name of PxRevoluteJoint, used for serialization. | |
Protected Member Functions | |
PX_INLINE | PxRevoluteJoint (PxType concreteType, PxBaseFlags baseFlags) |
Constructor. | |
PX_INLINE | PxRevoluteJoint (PxBaseFlags baseFlags) |
Deserialization constructor. | |
virtual bool | isKindOf (const char *name) const |
Returns whether a given type name matches with the type of this instance. |
Detailed Description
A joint which behaves in a similar way to a hinge or axle.A hinge joint removes all but a single rotational degree of freedom from two objects. The axis along which the two bodies may rotate is specified with a point and a direction vector.
The position of the hinge on each body is specified by the origin of the body's joint frame. The axis of the hinge is specified as the direction of the x-axis in the body's joint frame.
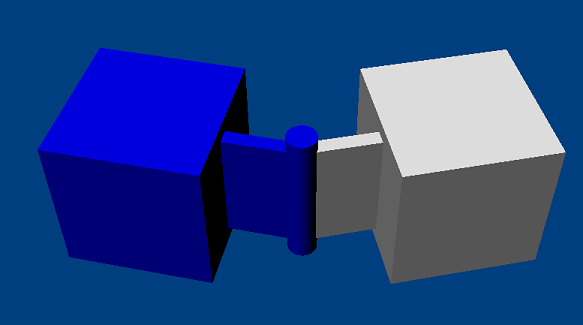
A revolute joint can be given a motor, so that it can apply a force to rotate the attached actors. It may also be given a limit, to restrict the revolute motion to within a certain range. In addition, the bodies may be projected together if the distance or angle between them exceeds a given threshold.
Projection, drive and limits are activated by setting the appropriate flags on the joint.
- See also:
- PxRevoluteJointCreate() PxJoint
Constructor & Destructor Documentation
PX_INLINE PxRevoluteJoint::PxRevoluteJoint | ( | PxType | concreteType, | |
PxBaseFlags | baseFlags | |||
) | [inline, protected] |
Constructor.
PX_INLINE PxRevoluteJoint::PxRevoluteJoint | ( | PxBaseFlags | baseFlags | ) | [inline, protected] |
Deserialization constructor.
Member Function Documentation
virtual PxReal PxRevoluteJoint::getAngle | ( | ) | const [pure virtual] |
return the angle of the joint, in the range (-Pi, Pi]
virtual const char* PxRevoluteJoint::getConcreteTypeName | ( | ) | const [inline, virtual] |
virtual PxReal PxRevoluteJoint::getDriveForceLimit | ( | ) | const [pure virtual] |
virtual PxReal PxRevoluteJoint::getDriveGearRatio | ( | ) | const [pure virtual] |
virtual PxReal PxRevoluteJoint::getDriveVelocity | ( | ) | const [pure virtual] |
gets the target velocity for the drive model.
- Returns:
- the drive target velocity
- See also:
- setDriveVelocity()
virtual PxJointAngularLimitPair PxRevoluteJoint::getLimit | ( | ) | const [pure virtual] |
get the joint limit parameters.
- Returns:
- the joint limit parameters
- See also:
- PxJointAngularLimitPair setLimit()
virtual PxReal PxRevoluteJoint::getProjectionAngularTolerance | ( | ) | const [pure virtual] |
gets the angular tolerance threshold for projection.
- Returns:
- the angular tolerance threshold in radians
- See also:
- setProjectionAngularTolerance()
virtual PxReal PxRevoluteJoint::getProjectionLinearTolerance | ( | ) | const [pure virtual] |
Get the linear tolerance threshold for projection.
- Returns:
- the linear tolerance threshold
- See also:
- setProjectionLinearTolerance()
virtual PxRevoluteJointFlags PxRevoluteJoint::getRevoluteJointFlags | ( | void | ) | const [pure virtual] |
gets the flags specific to the Revolute Joint.
- Returns:
- the joint flags
- See also:
- PxRevoluteJoint::flags, PxRevoluteJointFlag setFlag() setFlags()
virtual PxReal PxRevoluteJoint::getVelocity | ( | ) | const [pure virtual] |
return the velocity of the joint
virtual bool PxRevoluteJoint::isKindOf | ( | const char * | name | ) | const [inline, protected, virtual] |
Returns whether a given type name matches with the type of this instance.
Reimplemented from PxJoint.
References PxJoint::isKindOf().
virtual void PxRevoluteJoint::setDriveForceLimit | ( | PxReal | limit | ) | [pure virtual] |
sets the maximum torque the drive can exert.
Setting this to a very large value if velTarget is also very large may cause unexpected results.
The value set here may be used either as an impulse limit or a force limit, depending on the flag PxConstraintFlag::eDRIVE_LIMITS_ARE_FORCES
Range: [0, PX_MAX_F32)
Default: PX_MAX_F32
- See also:
- setDriveVelocity()
virtual void PxRevoluteJoint::setDriveGearRatio | ( | PxReal | ratio | ) | [pure virtual] |
sets the gear ratio for the drive.
When setting up the drive constraint, the velocity of the first actor is scaled by this value, and its response to drive torque is scaled down. So if the drive target velocity is zero, the second actor will be driven to the velocity of the first scaled by the gear ratio
Range: [0, PX_MAX_F32)
Default: 1.0
- Parameters:
-
[in] ratio the drive gear ratio
- See also:
- getDriveGearRatio()
virtual void PxRevoluteJoint::setDriveVelocity | ( | PxReal | velocity | ) | [pure virtual] |
set the target velocity for the drive model.
The motor will only be able to reach this velocity if the maxForce is sufficiently large. If the joint is spinning faster than this velocity, the motor will actually try to brake (see PxRevoluteJointFlag::eDRIVE_FREESPIN.)
If you set this to infinity then the motor will keep speeding up, unless there is some sort of resistance on the attached bodies. The sign of this variable determines the rotation direction, with positive values going the same way as positive joint angles.
- Parameters:
-
[in] velocity the drive target velocity
Default: 0.0
- See also:
- PxRevoluteFlags::eDRIVE_FREESPIN
virtual void PxRevoluteJoint::setLimit | ( | const PxJointAngularLimitPair & | limits | ) | [pure virtual] |
set the joint limit parameters.
The limit is activated using the flag PxRevoluteJointFlag::eLIMIT_ENABLED
The limit angle range is (-2*PI, 2*PI) and the extent of the limit must be strictly less than 2*PI
- Parameters:
-
[in] limits The joint limit parameters.
- See also:
- PxJointAngularLimitPair getLimit()
virtual void PxRevoluteJoint::setProjectionAngularTolerance | ( | PxReal | tolerance | ) | [pure virtual] |
Set the angular tolerance threshold for projection. Projection is enabled if PxConstraintFlag::ePROJECTION is set for the joint.
If the joint deviates by more than this angle around its locked angular degrees of freedom, the solver will move the bodies to close the angle.
Setting a very small tolerance may result in simulation jitter or other artifacts.
Sometimes it is not possible to project (for example when the joints form a cycle).
Range: [0,Pi]
Default: Pi
- Parameters:
-
[in] tolerance the angular tolerance threshold in radians
virtual void PxRevoluteJoint::setProjectionLinearTolerance | ( | PxReal | tolerance | ) | [pure virtual] |
Set the linear tolerance threshold for projection. Projection is enabled if PxConstraintFlag::ePROJECTION is set for the joint.
If the joint separates by more than this distance along its locked degrees of freedom, the solver will move the bodies to close the distance.
Setting a very small tolerance may result in simulation jitter or other artifacts.
Sometimes it is not possible to project (for example when the joints form a cycle).
Range: [0, PX_MAX_F32)
Default: 1e10f
- Parameters:
-
[in] tolerance the linear tolerance threshold
virtual void PxRevoluteJoint::setRevoluteJointFlag | ( | PxRevoluteJointFlag::Enum | flag, | |
bool | value | |||
) | [pure virtual] |
sets a single flag specific to a Revolute Joint.
- Parameters:
-
[in] flag The flag to set or clear. [in] value the value to which to set the flag
- See also:
- PxRevoluteJointFlag, getFlags() setFlags()
virtual void PxRevoluteJoint::setRevoluteJointFlags | ( | PxRevoluteJointFlags | flags | ) | [pure virtual] |
sets the flags specific to the Revolute Joint.
Default PxRevoluteJointFlags(0)
- Parameters:
-
[in] flags The joint flags.
- See also:
- PxRevoluteJointFlag setFlag() getFlags()
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com