PxConstraint Class Reference
[Physics]
A plugin class for implementing constraints.
More...
#include <PxConstraint.h>
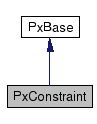
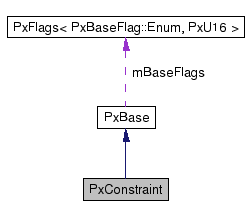
Public Member Functions | |
virtual void | release ()=0 |
Releases a PxConstraint instance. | |
virtual PxScene * | getScene () const =0 |
Retrieves the scene which this constraint belongs to. | |
virtual void | getActors (PxRigidActor *&actor0, PxRigidActor *&actor1) const =0 |
Retrieves the actors for this constraint. | |
virtual void | setActors (PxRigidActor *actor0, PxRigidActor *actor1)=0 |
Sets the actors for this constraint. | |
virtual void | markDirty ()=0 |
Notify the scene that the constraint shader data has been updated by the application. | |
virtual void | setFlags (PxConstraintFlags flags)=0 |
Set the flags for this constraint. | |
virtual PxConstraintFlags | getFlags () const =0 |
Retrieve the flags for this constraint. | |
virtual void | setFlag (PxConstraintFlag::Enum flag, bool value)=0 |
Set a flag for this constraint. | |
virtual void | getForce (PxVec3 &linear, PxVec3 &angular) const =0 |
Retrieve the constraint force most recently applied to maintain this constraint. | |
virtual bool | isValid () const =0 |
whether the constraint is valid. | |
virtual void | setBreakForce (PxReal linear, PxReal angular)=0 |
Set the break force and torque thresholds for this constraint. | |
virtual void | getBreakForce (PxReal &linear, PxReal &angular) const =0 |
Retrieve the constraint break force and torque thresholds. | |
virtual void | setMinResponseThreshold (PxReal threshold)=0 |
Set the minimum response threshold for a constraint row. | |
virtual PxReal | getMinResponseThreshold () const =0 |
Retrieve the constraint break force and torque thresholds. | |
virtual void * | getExternalReference (PxU32 &typeID)=0 |
Fetch external owner of the constraint. | |
virtual void | setConstraintFunctions (PxConstraintConnector &connector, const PxConstraintShaderTable &shaders)=0 |
Set the constraint functions for this constraint. | |
virtual const char * | getConcreteTypeName () const |
Returns string name of dynamic type. | |
Protected Member Functions | |
PX_INLINE | PxConstraint (PxType concreteType, PxBaseFlags baseFlags) |
PX_INLINE | PxConstraint (PxBaseFlags baseFlags) |
virtual | ~PxConstraint () |
virtual bool | isKindOf (const char *name) const |
Returns whether a given type name matches with the type of this instance. |
Detailed Description
A plugin class for implementing constraints.
- See also:
- PxPhysics.createConstraint
Constructor & Destructor Documentation
PX_INLINE PxConstraint::PxConstraint | ( | PxType | concreteType, | |
PxBaseFlags | baseFlags | |||
) | [inline, protected] |
PX_INLINE PxConstraint::PxConstraint | ( | PxBaseFlags | baseFlags | ) | [inline, protected] |
virtual PxConstraint::~PxConstraint | ( | ) | [inline, protected, virtual] |
Member Function Documentation
virtual void PxConstraint::getActors | ( | PxRigidActor *& | actor0, | |
PxRigidActor *& | actor1 | |||
) | const [pure virtual] |
Retrieves the actors for this constraint.
- Parameters:
-
[out] actor0 a reference to the pointer for the first actor [out] actor1 a reference to the pointer for the second actor
- See also:
- PxActor
virtual void PxConstraint::getBreakForce | ( | PxReal & | linear, | |
PxReal & | angular | |||
) | const [pure virtual] |
Retrieve the constraint break force and torque thresholds.
- Parameters:
-
[out] linear the linear break threshold [out] angular the angular break threshold
virtual const char* PxConstraint::getConcreteTypeName | ( | ) | const [inline, virtual] |
Returns string name of dynamic type.
- Returns:
- Class name of most derived type of this object.
Implements PxBase.
virtual void* PxConstraint::getExternalReference | ( | PxU32 & | typeID | ) | [pure virtual] |
Fetch external owner of the constraint.
Provides a reference to the external owner of a constraint and a unique owner type ID.
- Parameters:
-
[out] typeID Unique type identifier of the external object.
- Returns:
- Reference to the external object which owns the constraint.
virtual PxConstraintFlags PxConstraint::getFlags | ( | ) | const [pure virtual] |
Retrieve the constraint force most recently applied to maintain this constraint.
- Parameters:
-
[out] linear the constraint force [out] angular the constraint torque
virtual PxReal PxConstraint::getMinResponseThreshold | ( | ) | const [pure virtual] |
Retrieve the constraint break force and torque thresholds.
- Returns:
- the minimum response threshold for a constraint row
virtual PxScene* PxConstraint::getScene | ( | ) | const [pure virtual] |
Retrieves the scene which this constraint belongs to.
- Returns:
- Owner Scene. NULL if not part of a scene.
- See also:
- PxScene
virtual bool PxConstraint::isKindOf | ( | const char * | superClass | ) | const [inline, protected, virtual] |
Returns whether a given type name matches with the type of this instance.
Reimplemented from PxBase.
References PxBase::isKindOf().
virtual bool PxConstraint::isValid | ( | ) | const [pure virtual] |
whether the constraint is valid.
A constraint is valid if it has at least one dynamic rigid body or articulation link. A constraint that is not valid may not be inserted into a scene, and therefore a static actor to which an invalid constraint is attached may not be inserted into a scene.
Invalid constraints arise only when an actor to which the constraint is attached has been deleted.
virtual void PxConstraint::markDirty | ( | ) | [pure virtual] |
Notify the scene that the constraint shader data has been updated by the application.
virtual void PxConstraint::release | ( | ) | [pure virtual] |
Releases a PxConstraint instance.
- Note:
- This call does not wake up the connected rigid bodies.
- See also:
- PxPhysics.createConstraint, PxBase.release()
Implements PxBase.
virtual void PxConstraint::setActors | ( | PxRigidActor * | actor0, | |
PxRigidActor * | actor1 | |||
) | [pure virtual] |
Sets the actors for this constraint.
- Parameters:
-
[in] actor0 a reference to the pointer for the first actor [in] actor1 a reference to the pointer for the second actor
- See also:
- PxActor
virtual void PxConstraint::setBreakForce | ( | PxReal | linear, | |
PxReal | angular | |||
) | [pure virtual] |
Set the break force and torque thresholds for this constraint.
If either the force or torque measured at the constraint exceed these thresholds the constraint will break.
- Parameters:
-
[in] linear the linear break threshold [in] angular the angular break threshold
virtual void PxConstraint::setConstraintFunctions | ( | PxConstraintConnector & | connector, | |
const PxConstraintShaderTable & | shaders | |||
) | [pure virtual] |
Set the constraint functions for this constraint.
- Parameters:
-
[in] connector the constraint connector object by which the SDK communicates with the constraint. [in] shaders the shader table for the constraint
virtual void PxConstraint::setFlag | ( | PxConstraintFlag::Enum | flag, | |
bool | value | |||
) | [pure virtual] |
Set a flag for this constraint.
- Parameters:
-
[in] flag the constraint flag [in] value the new value of the flag
- See also:
- PxConstraintFlags
virtual void PxConstraint::setFlags | ( | PxConstraintFlags | flags | ) | [pure virtual] |
Set the flags for this constraint.
- Parameters:
-
[in] flags the new constraint flags
- See also:
- PxConstraintFlags
virtual void PxConstraint::setMinResponseThreshold | ( | PxReal | threshold | ) | [pure virtual] |
Set the minimum response threshold for a constraint row.
When using mass modification for a joint or infinite inertia for a jointed body, very stiff solver constraints can be generated which can destabilize simulation. Setting this value to a small positive value (e.g. 1e-8) will cause constraint rows to be ignored if very large changes in impulses will generate only small changes in velocity. When setting this value, also set PxConstraintFlag::eDISABLE_PREPROCESSING. The solver accuracy for this joint may be reduced.
- Parameters:
-
[in] threshold the minimum response threshold
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com