PxConvexMesh Class Reference
[Geomutils]
A convex mesh.
More...
#include <PxConvexMesh.h>
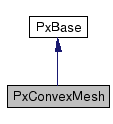
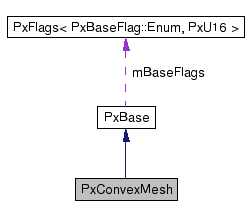
Public Member Functions | |
virtual PX_PHYSX_COMMON_API PxU32 | getNbVertices () const =0 |
Returns the number of vertices. | |
virtual PX_PHYSX_COMMON_API const PxVec3 * | getVertices () const =0 |
Returns the vertices. | |
virtual PX_PHYSX_COMMON_API const PxU8 * | getIndexBuffer () const =0 |
Returns the index buffer. | |
virtual PX_PHYSX_COMMON_API PxU32 | getNbPolygons () const =0 |
Returns the number of polygons. | |
virtual PX_PHYSX_COMMON_API bool | getPolygonData (PxU32 index, PxHullPolygon &data) const =0 |
Returns the polygon data. | |
virtual PX_PHYSX_COMMON_API void | release ()=0 |
Decrements the reference count of a convex mesh and releases it if the new reference count is zero. | |
virtual PX_PHYSX_COMMON_API PxU32 | getReferenceCount () const =0 |
Returns the reference count of a convex mesh. | |
virtual PX_PHYSX_COMMON_API void | acquireReference ()=0 |
Acquires a counted reference to a convex mesh. | |
virtual PX_PHYSX_COMMON_API void | getMassInformation (PxReal &mass, PxMat33 &localInertia, PxVec3 &localCenterOfMass) const =0 |
Returns the mass properties of the mesh assuming unit density. | |
virtual PX_PHYSX_COMMON_API PxBounds3 | getLocalBounds () const =0 |
Returns the local-space (vertex space) AABB from the convex mesh. | |
virtual PX_PHYSX_COMMON_API const char * | getConcreteTypeName () const |
Returns string name of dynamic type. | |
virtual PX_PHYSX_COMMON_API bool | isGpuCompatible () const =0 |
This method decides whether a convex mesh is gpu compatible. If the total number of vertices are more than 64 or any number of vertices in a polygon is more than 32, or convex hull data was not cooked with GPU data enabled during cooking or was loaded from a serialized collection, the convex hull is incompatible with GPU collision detection. Otherwise it is compatible. | |
Protected Member Functions | |
PX_INLINE | PxConvexMesh (PxType concreteType, PxBaseFlags baseFlags) |
PX_INLINE | PxConvexMesh (PxBaseFlags baseFlags) |
virtual PX_PHYSX_COMMON_API | ~PxConvexMesh () |
virtual PX_PHYSX_COMMON_API bool | isKindOf (const char *name) const |
Returns whether a given type name matches with the type of this instance. |
Detailed Description
A convex mesh.Internally represented as a list of convex polygons. The number of polygons is limited to 256.
To avoid duplicating data when you have several instances of a particular mesh positioned differently, you do not use this class to represent a convex object directly. Instead, you create an instance of this mesh via the PxConvexMeshGeometry and PxShape classes.
Creation
To create an instance of this class call PxPhysics::createConvexMesh(), and PxConvexMesh::release() to delete it. This is only possible once you have released all of its PxShape instances.
Visualizations:
- PxVisualizationParameter::eCOLLISION_AABBS
- PxVisualizationParameter::eCOLLISION_SHAPES
- PxVisualizationParameter::eCOLLISION_AXES
- PxVisualizationParameter::eCOLLISION_FNORMALS
- PxVisualizationParameter::eCOLLISION_EDGES
Constructor & Destructor Documentation
PX_INLINE PxConvexMesh::PxConvexMesh | ( | PxType | concreteType, | |
PxBaseFlags | baseFlags | |||
) | [inline, protected] |
PX_INLINE PxConvexMesh::PxConvexMesh | ( | PxBaseFlags | baseFlags | ) | [inline, protected] |
virtual PX_PHYSX_COMMON_API PxConvexMesh::~PxConvexMesh | ( | ) | [inline, protected, virtual] |
Member Function Documentation
virtual PX_PHYSX_COMMON_API void PxConvexMesh::acquireReference | ( | ) | [pure virtual] |
Acquires a counted reference to a convex mesh.
This method increases the reference count of the convex mesh by 1. Decrement the reference count by calling release()
virtual PX_PHYSX_COMMON_API const char* PxConvexMesh::getConcreteTypeName | ( | ) | const [inline, virtual] |
Returns string name of dynamic type.
- Returns:
- Class name of most derived type of this object.
Implements PxBase.
virtual PX_PHYSX_COMMON_API const PxU8* PxConvexMesh::getIndexBuffer | ( | ) | const [pure virtual] |
virtual PX_PHYSX_COMMON_API PxBounds3 PxConvexMesh::getLocalBounds | ( | ) | const [pure virtual] |
Returns the local-space (vertex space) AABB from the convex mesh.
- Returns:
- local-space bounds
virtual PX_PHYSX_COMMON_API void PxConvexMesh::getMassInformation | ( | PxReal & | mass, | |
PxMat33 & | localInertia, | |||
PxVec3 & | localCenterOfMass | |||
) | const [pure virtual] |
Returns the mass properties of the mesh assuming unit density.
The following relationship holds between mass and volume:
mass = volume * density
The mass of a unit density mesh is equal to its volume, so this function returns the volume of the mesh.
Similarly, to obtain the localInertia of an identically shaped object with a uniform density of d, simply multiply the localInertia of the unit density mesh by d.
- Parameters:
-
[out] mass The mass of the mesh assuming unit density. [out] localInertia The inertia tensor in mesh local space assuming unit density. [out] localCenterOfMass Position of center of mass (or centroid) in mesh local space.
Referenced by PxMassProperties::PxMassProperties().
virtual PX_PHYSX_COMMON_API PxU32 PxConvexMesh::getNbPolygons | ( | ) | const [pure virtual] |
Returns the number of polygons.
- Returns:
- Number of polygons.
- See also:
- getIndexBuffer() getPolygonData()
virtual PX_PHYSX_COMMON_API PxU32 PxConvexMesh::getNbVertices | ( | ) | const [pure virtual] |
virtual PX_PHYSX_COMMON_API bool PxConvexMesh::getPolygonData | ( | PxU32 | index, | |
PxHullPolygon & | data | |||
) | const [pure virtual] |
Returns the polygon data.
- Parameters:
-
[in] index Polygon index in [0 ; getNbPolygons()[. [out] data Polygon data.
- Returns:
- True if success.
- See also:
- getIndexBuffer() getNbPolygons()
virtual PX_PHYSX_COMMON_API PxU32 PxConvexMesh::getReferenceCount | ( | ) | const [pure virtual] |
Returns the reference count of a convex mesh.
At creation, the reference count of the convex mesh is 1. Every shape referencing this convex mesh increments the count by 1. When the reference count reaches 0, and only then, the convex mesh gets destroyed automatically.
- Returns:
- the current reference count.
virtual PX_PHYSX_COMMON_API const PxVec3* PxConvexMesh::getVertices | ( | ) | const [pure virtual] |
virtual PX_PHYSX_COMMON_API bool PxConvexMesh::isGpuCompatible | ( | ) | const [pure virtual] |
This method decides whether a convex mesh is gpu compatible. If the total number of vertices are more than 64 or any number of vertices in a polygon is more than 32, or convex hull data was not cooked with GPU data enabled during cooking or was loaded from a serialized collection, the convex hull is incompatible with GPU collision detection. Otherwise it is compatible.
- Returns:
- True if the convex hull is gpu compatible
virtual PX_PHYSX_COMMON_API bool PxConvexMesh::isKindOf | ( | const char * | superClass | ) | const [inline, protected, virtual] |
Returns whether a given type name matches with the type of this instance.
Reimplemented from PxBase.
References PxBase::isKindOf().
virtual PX_PHYSX_COMMON_API void PxConvexMesh::release | ( | ) | [pure virtual] |
Decrements the reference count of a convex mesh and releases it if the new reference count is zero.
Implements PxBase.
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com