PxMat33 Class Reference
[Foundation]
3x3 matrix class
More...
#include <PxMat33.h>
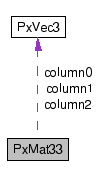
Public Member Functions | |
PX_CUDA_CALLABLE PX_FORCE_INLINE | PxMat33 () |
Default constructor. | |
PX_CUDA_CALLABLE PX_INLINE | PxMat33 (PxIDENTITY r) |
identity constructor | |
PX_CUDA_CALLABLE PX_INLINE | PxMat33 (PxZERO r) |
zero constructor | |
PX_CUDA_CALLABLE | PxMat33 (const PxVec3 &col0, const PxVec3 &col1, const PxVec3 &col2) |
Construct from three base vectors. | |
PX_CUDA_CALLABLE PX_INLINE | PxMat33 (float r) |
constructor from a scalar, which generates a multiple of the identity matrix | |
PX_CUDA_CALLABLE PX_INLINE | PxMat33 (float values[]) |
Construct from float[9]. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE | PxMat33 (const PxQuat &q) |
Construct from a quaternion. | |
PX_CUDA_CALLABLE PX_INLINE | PxMat33 (const PxMat33 &other) |
Copy constructor. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxMat33 & | operator= (const PxMat33 &other) |
Assignment operator. | |
PX_CUDA_CALLABLE PX_INLINE bool | operator== (const PxMat33 &m) const |
returns true if the two matrices are exactly equal | |
PX_CUDA_CALLABLE PX_FORCE_INLINE const PxMat33 | getTranspose () const |
Get transposed matrix. | |
PX_CUDA_CALLABLE PX_INLINE const PxMat33 | getInverse () const |
Get the real inverse. | |
PX_CUDA_CALLABLE PX_INLINE float | getDeterminant () const |
Get determinant. | |
PX_CUDA_CALLABLE PX_INLINE const PxMat33 | operator- () const |
Unary minus. | |
PX_CUDA_CALLABLE PX_INLINE const PxMat33 | operator+ (const PxMat33 &other) const |
Add. | |
PX_CUDA_CALLABLE PX_INLINE const PxMat33 | operator- (const PxMat33 &other) const |
Subtract. | |
PX_CUDA_CALLABLE PX_INLINE const PxMat33 | operator* (float scalar) const |
Scalar multiplication. | |
PX_CUDA_CALLABLE PX_INLINE const PxVec3 | operator* (const PxVec3 &vec) const |
Matrix vector multiplication (returns 'this->transform(vec)'). | |
PX_CUDA_CALLABLE PX_FORCE_INLINE const PxMat33 | operator* (const PxMat33 &other) const |
Matrix multiplication. | |
PX_CUDA_CALLABLE PX_INLINE PxMat33 & | operator+= (const PxMat33 &other) |
Equals-add. | |
PX_CUDA_CALLABLE PX_INLINE PxMat33 & | operator-= (const PxMat33 &other) |
Equals-sub. | |
PX_CUDA_CALLABLE PX_INLINE PxMat33 & | operator*= (float scalar) |
Equals scalar multiplication. | |
PX_CUDA_CALLABLE PX_INLINE PxMat33 & | operator*= (const PxMat33 &other) |
Equals matrix multiplication. | |
PX_DEPRECATED PX_CUDA_CALLABLE PX_FORCE_INLINE float | operator() (unsigned int row, unsigned int col) const |
Element access, mathematical way! | |
PX_DEPRECATED PX_CUDA_CALLABLE PX_FORCE_INLINE float & | operator() (unsigned int row, unsigned int col) |
Element access, mathematical way! | |
PX_CUDA_CALLABLE PX_FORCE_INLINE const PxVec3 | transform (const PxVec3 &other) const |
Transform vector by matrix, equal to v' = M*v. | |
PX_CUDA_CALLABLE PX_INLINE const PxVec3 | transformTranspose (const PxVec3 &other) const |
Transform vector by matrix transpose, v' = M^t*v. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE const float * | front () const |
PX_DEPRECATED PX_CUDA_CALLABLE PX_FORCE_INLINE PxVec3 & | operator[] (unsigned int num) |
PX_DEPRECATED PX_CUDA_CALLABLE PX_FORCE_INLINE const PxVec3 & | operator[] (unsigned int num) const |
Static Public Member Functions | |
PX_CUDA_CALLABLE static PX_INLINE const PxMat33 | createDiagonal (const PxVec3 &d) |
Construct from diagonal, off-diagonals are zero. | |
Public Attributes | |
PxVec3 | column0 |
PxVec3 | column1 |
PxVec3 | column2 |
Friends | |
PxMat33 | operator* (float, const PxMat33 &) |
Detailed Description
3x3 matrix classSome clarifications, as there have been much confusion about matrix formats etc in the past.
Short:
- Matrix have base vectors in columns (vectors are column matrices, 3x1 matrices).
- Matrix is physically stored in column major format
- Matrices are concaternated from left
Long: Given three base vectors a, b and c the matrix is stored as
|a.x b.x c.x| |a.y b.y c.y| |a.z b.z c.z|
Vectors are treated as columns, so the vector v is
|x| |y| |z|
And matrices are applied _before_ the vector (pre-multiplication) v' = M*v
|x'| |a.x b.x c.x| |x| |a.x*x + b.x*y + c.x*z| |y'| = |a.y b.y c.y| * |y| = |a.y*x + b.y*y + c.y*z| |z'| |a.z b.z c.z| |z| |a.z*x + b.z*y + c.z*z|
Physical storage and indexing: To be compatible with popular 3d rendering APIs (read D3d and OpenGL) the physical indexing is
|0 3 6| |1 4 7| |2 5 8|
index = column*3 + row
which in C++ translates to M[column][row]
The mathematical indexing is M_row,column and this is what is used for _-notation so _12 is 1st row, second column and operator(row, column)!
Constructor & Destructor Documentation
PX_CUDA_CALLABLE PX_FORCE_INLINE PxMat33::PxMat33 | ( | ) | [inline] |
Default constructor.
PX_CUDA_CALLABLE PX_INLINE PxMat33::PxMat33 | ( | PxIDENTITY | r | ) | [inline] |
PX_CUDA_CALLABLE PX_INLINE PxMat33::PxMat33 | ( | PxZERO | r | ) | [inline] |
PX_CUDA_CALLABLE PxMat33::PxMat33 | ( | const PxVec3 & | col0, | |
const PxVec3 & | col1, | |||
const PxVec3 & | col2 | |||
) | [inline] |
Construct from three base vectors.
PX_CUDA_CALLABLE PX_INLINE PxMat33::PxMat33 | ( | float | r | ) | [inline, explicit] |
constructor from a scalar, which generates a multiple of the identity matrix
PX_CUDA_CALLABLE PX_INLINE PxMat33::PxMat33 | ( | float | values[] | ) | [inline, explicit] |
Construct from float[9].
PX_CUDA_CALLABLE PX_FORCE_INLINE PxMat33::PxMat33 | ( | const PxQuat & | q | ) | [inline, explicit] |
PX_CUDA_CALLABLE PX_INLINE PxMat33::PxMat33 | ( | const PxMat33 & | other | ) | [inline] |
Copy constructor.
Member Function Documentation
PX_CUDA_CALLABLE static PX_INLINE const PxMat33 PxMat33::createDiagonal | ( | const PxVec3 & | d | ) | [inline, static] |
Construct from diagonal, off-diagonals are zero.
References PxVec3::x, PxVec3::y, and PxVec3::z.
Referenced by PxMassProperties::PxMassProperties().
PX_CUDA_CALLABLE PX_FORCE_INLINE const float* PxMat33::front | ( | ) | const [inline] |
PX_CUDA_CALLABLE PX_INLINE float PxMat33::getDeterminant | ( | ) | const [inline] |
Get determinant.
PX_CUDA_CALLABLE PX_INLINE const PxMat33 PxMat33::getInverse | ( | ) | const [inline] |
PX_CUDA_CALLABLE PX_FORCE_INLINE const PxMat33 PxMat33::getTranspose | ( | ) | const [inline] |
PX_DEPRECATED PX_CUDA_CALLABLE PX_FORCE_INLINE float& PxMat33::operator() | ( | unsigned int | row, | |
unsigned int | col | |||
) | [inline] |
Element access, mathematical way!
PX_DEPRECATED PX_CUDA_CALLABLE PX_FORCE_INLINE float PxMat33::operator() | ( | unsigned int | row, | |
unsigned int | col | |||
) | const [inline] |
Element access, mathematical way!
Matrix vector multiplication (returns 'this->transform(vec)').
PX_CUDA_CALLABLE PX_INLINE const PxMat33 PxMat33::operator* | ( | float | scalar | ) | const [inline] |
Scalar multiplication.
Equals matrix multiplication.
PX_CUDA_CALLABLE PX_INLINE PxMat33& PxMat33::operator*= | ( | float | scalar | ) | [inline] |
Equals scalar multiplication.
PX_CUDA_CALLABLE PX_INLINE const PxMat33 PxMat33::operator- | ( | ) | const [inline] |
Unary minus.
PX_CUDA_CALLABLE PX_INLINE bool PxMat33::operator== | ( | const PxMat33 & | m | ) | const [inline] |
PX_DEPRECATED PX_CUDA_CALLABLE PX_FORCE_INLINE const PxVec3& PxMat33::operator[] | ( | unsigned int | num | ) | const [inline] |
PX_DEPRECATED PX_CUDA_CALLABLE PX_FORCE_INLINE PxVec3& PxMat33::operator[] | ( | unsigned int | num | ) | [inline] |
PX_CUDA_CALLABLE PX_INLINE const PxVec3 PxMat33::transformTranspose | ( | const PxVec3 & | other | ) | const [inline] |
Transform vector by matrix transpose, v' = M^t*v.
Friends And Related Function Documentation
Member Data Documentation
Referenced by PxBounds3::basisExtent(), getInverse(), PxMassProperties::getMassSpaceInertia(), operator*(), operator+(), operator+=(), operator-(), operator-=(), operator=(), operator==(), PxQuat::PxQuat(), PxMassProperties::rotateInertia(), PxMassProperties::scaleInertia(), PxMassProperties::sum(), PxMeshScale::toMat33(), and PxMassProperties::translateInertia().
Referenced by PxBounds3::basisExtent(), getInverse(), PxMassProperties::getMassSpaceInertia(), operator*(), operator+(), operator+=(), operator-(), operator-=(), operator=(), operator==(), PxQuat::PxQuat(), PxMassProperties::rotateInertia(), PxMassProperties::scaleInertia(), PxMassProperties::sum(), PxMeshScale::toMat33(), and PxMassProperties::translateInertia().
Referenced by PxBounds3::basisExtent(), getInverse(), PxMassProperties::getMassSpaceInertia(), operator*(), operator+(), operator+=(), operator-(), operator-=(), operator=(), operator==(), PxQuat::PxQuat(), PxMassProperties::rotateInertia(), PxMassProperties::scaleInertia(), PxMassProperties::sum(), PxMeshScale::toMat33(), and PxMassProperties::translateInertia().
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com