Inshaping
0.1
|
anyarg.h
Go to the documentation of this file.
39 A single letter option begins with a hyphen '-'. The parsing of single letter options follows POSIX conventions.
47 --buffer-size, -n), you can turn on flag a and v, set option s to 100 and n to 50, and pass another two
bool add_option_int(char letter, int v0, const char *desc)
bool add_option_str(const char *name, char letter, const char *v0, const char *desc)
double get_value_double(const char *name) const
int get_value_int(const char *name) const
bool add_flag(const char *name, char letter, const char *desc)
int get_argc() const
const char * auto_usage()
bool set_value(const char *opt_value)
bool add_option_double(char letter, double v0, const char *desc)
bool parse_argv(int argc, char **argv)
const char * get_arg(int i) const
Use this class to define program options and parse command line arguments.
Definition: anyarg.h:97
bool set_desc_meta(const char *opt_desc)
bool is_true(const char *name) const
const char * get_value_str(const char *name) const
Generated by
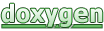