Inshaping
0.1
|
TriSparse.h
Go to the documentation of this file.
49 //���� void setParam(const double _THRESHOLD, const double _THETA, const double _LAMDA_MIN, const double _LAMDA_MAX, const double _ETA, bool _doubleCheck);
void setParam(const ParamItem& _param, bool _doubleCheck);
void printParam();
inline void setInput(
pcl::PointCloud<pcl::PointNormal>::Ptr _body,
MyMeshPtr _head)
{
body = _body;
head = _head;
n = (*_head).n_vertices();
}
void setA(Eigen::SparseMatrix<double>& _A);
void setB(Eigen::VectorXd& _B);
void updateHead(pcl::PointCloud<pcl::PointXYZ>::Ptr& head_cloud);
double getLAMDA(unsigned index);
MyMesh& getHead() { return *head; }
pcl::PointCloud<pcl::PointNormal>& getBody() { return *body; }
std::unordered_map<unsigned, unsigned>& getMap() { return maping; }
bool construct();//���������飬����֮ǰ��Ҫ�ȵ���setParam()
bool solve(Eigen::VectorXd& x);//�ⷽ����
bool solveToCloud(pcl::PointCloud<pcl::PointXYZ>::Ptr& cloud);//�����ֱ�ӱ�ɵ���
~TriSparse() {};
//-------------------��̬����------------------//
bool static solveTriSparse(Eigen::SparseMatrix<double>& A, Eigen::VectorXd& B, Eigen::VectorXd& x);
//˫��У�飬����Ҫhead�����body������������head���Ǹ���
static void confirmNearestNeighbor(pcl::PointCloud<pcl::PointNormal>& body, MyMesh& head_mesh, std::unordered_map<unsigned, unsigned>& maping, double threshold);
//˫��У�飬����Ҫhead�����body������������head���Ǹ���
static void confirmNearestNeighbor(pcl::PointCloud<pcl::PointXYZ>& from, pcl::PointCloud<pcl::PointNormal>& to, std::unordered_map<unsigned, unsigned>& maping, double threshold);
//���� body ���ƺ� head ������,Ȼ����һ��maping,�������ֵ threshold
static void findNearestNeighbor(pcl::PointCloud<pcl::PointNormal>& body, MyMesh& head, std::unordered_map<unsigned, unsigned>& maping, double threshold, bool doubleCheck);
static void findNearestNeighbor2(pcl::PointCloud<pcl::PointXYZ> & body, pcl::PointCloud<pcl::PointXYZ>& head, std::unordered_map<unsigned, unsigned>& maping, double threshold, bool doubleCheck);
static void confirmNearestNeighbor2(pcl::PointCloud<pcl::PointXYZ> & from, pcl::PointCloud<pcl::PointXYZ> & to, std::unordered_map<unsigned, unsigned>& maping, double threshold);
//�õ��Ƶĵ����滻mesh�Ķ���
static void updateMesh(MyMesh& mesh, pcl::PointCloud<pcl::PointXYZ>::Ptr& cloud);
};
/*@}*/
}
50 void setParam(const double _THRESHOLD, const double _THETA, const double _LAMDA_MIN, const double _LAMDA_MAX, const double _ETA, bool _doubleCheck);
82 bool solve(Eigen::VectorXd& x);//�ⷽ����
bool solveToCloud(pcl::PointCloud<pcl::PointXYZ>::Ptr& cloud);//�����ֱ�ӱ�ɵ���
~TriSparse() {};
//-------------------��̬����------------------//
bool static solveTriSparse(Eigen::SparseMatrix<double>& A, Eigen::VectorXd& B, Eigen::VectorXd& x);
//˫��У�飬����Ҫhead�����body������������head���Ǹ���
static void confirmNearestNeighbor(pcl::PointCloud<pcl::PointNormal>& body, MyMesh& head_mesh, std::unordered_map<unsigned, unsigned>& maping, double threshold);
//˫��У�飬����Ҫhead�����body������������head���Ǹ���
static void confirmNearestNeighbor(pcl::PointCloud<pcl::PointXYZ>& from, pcl::PointCloud<pcl::PointNormal>& to, std::unordered_map<unsigned, unsigned>& maping, double threshold);
//���� body ���ƺ� head ������,Ȼ����һ��maping,�������ֵ threshold
static void findNearestNeighbor(pcl::PointCloud<pcl::PointNormal>& body, MyMesh& head, std::unordered_map<unsigned, unsigned>& maping, double threshold, bool doubleCheck);
static void findNearestNeighbor2(pcl::PointCloud<pcl::PointXYZ> & body, pcl::PointCloud<pcl::PointXYZ>& head, std::unordered_map<unsigned, unsigned>& maping, double threshold, bool doubleCheck);
static void confirmNearestNeighbor2(pcl::PointCloud<pcl::PointXYZ> & from, pcl::PointCloud<pcl::PointXYZ> & to, std::unordered_map<unsigned, unsigned>& maping, double threshold);
//�õ��Ƶĵ����滻mesh�Ķ���
static void updateMesh(MyMesh& mesh, pcl::PointCloud<pcl::PointXYZ>::Ptr& cloud);
};
/*@}*/
}
84 bool solveToCloud(pcl::PointCloud<pcl::PointXYZ>::Ptr& cloud);//�����ֱ�ӱ�ɵ���
~TriSparse() {};
//-------------------��̬����------------------//
bool static solveTriSparse(Eigen::SparseMatrix<double>& A, Eigen::VectorXd& B, Eigen::VectorXd& x);
//˫��У�飬����Ҫhead�����body������������head���Ǹ���
static void confirmNearestNeighbor(pcl::PointCloud<pcl::PointNormal>& body, MyMesh& head_mesh, std::unordered_map<unsigned, unsigned>& maping, double threshold);
//˫��У�飬����Ҫhead�����body������������head���Ǹ���
static void confirmNearestNeighbor(pcl::PointCloud<pcl::PointXYZ>& from, pcl::PointCloud<pcl::PointNormal>& to, std::unordered_map<unsigned, unsigned>& maping, double threshold);
//���� body ���ƺ� head ������,Ȼ����һ��maping,�������ֵ threshold
static void findNearestNeighbor(pcl::PointCloud<pcl::PointNormal>& body, MyMesh& head, std::unordered_map<unsigned, unsigned>& maping, double threshold, bool doubleCheck);
static void findNearestNeighbor2(pcl::PointCloud<pcl::PointXYZ> & body, pcl::PointCloud<pcl::PointXYZ>& head, std::unordered_map<unsigned, unsigned>& maping, double threshold, bool doubleCheck);
static void confirmNearestNeighbor2(pcl::PointCloud<pcl::PointXYZ> & from, pcl::PointCloud<pcl::PointXYZ> & to, std::unordered_map<unsigned, unsigned>& maping, double threshold);
//�õ��Ƶĵ����滻mesh�Ķ���
static void updateMesh(MyMesh& mesh, pcl::PointCloud<pcl::PointXYZ>::Ptr& cloud);
};
/*@}*/
}
91 bool static solveTriSparse(Eigen::SparseMatrix<double>& A, Eigen::VectorXd& B, Eigen::VectorXd& x);
95 //˫��У�飬����Ҫhead�����body������������head���Ǹ��� static void confirmNearestNeighbor(pcl::PointCloud<pcl::PointNormal>& body, MyMesh& head_mesh, std::unordered_map<unsigned, unsigned>& maping, double threshold);
//˫��У�飬����Ҫhead�����body������������head���Ǹ���
static void confirmNearestNeighbor(pcl::PointCloud<pcl::PointXYZ>& from, pcl::PointCloud<pcl::PointNormal>& to, std::unordered_map<unsigned, unsigned>& maping, double threshold);
//���� body ���ƺ� head ������,Ȼ����һ��maping,�������ֵ threshold
static void findNearestNeighbor(pcl::PointCloud<pcl::PointNormal>& body, MyMesh& head, std::unordered_map<unsigned, unsigned>& maping, double threshold, bool doubleCheck);
static void findNearestNeighbor2(pcl::PointCloud<pcl::PointXYZ> & body, pcl::PointCloud<pcl::PointXYZ>& head, std::unordered_map<unsigned, unsigned>& maping, double threshold, bool doubleCheck);
static void confirmNearestNeighbor2(pcl::PointCloud<pcl::PointXYZ> & from, pcl::PointCloud<pcl::PointXYZ> & to, std::unordered_map<unsigned, unsigned>& maping, double threshold);
//�õ��Ƶĵ����滻mesh�Ķ���
static void updateMesh(MyMesh& mesh, pcl::PointCloud<pcl::PointXYZ>::Ptr& cloud);
};
/*@}*/
}
96 static void confirmNearestNeighbor(pcl::PointCloud<pcl::PointNormal>& body, MyMesh& head_mesh, std::unordered_map<unsigned, unsigned>& maping, double threshold);
98 //˫��У�飬����Ҫhead�����body������������head���Ǹ��� static void confirmNearestNeighbor(pcl::PointCloud<pcl::PointXYZ>& from, pcl::PointCloud<pcl::PointNormal>& to, std::unordered_map<unsigned, unsigned>& maping, double threshold);
//���� body ���ƺ� head ������,Ȼ����һ��maping,�������ֵ threshold
static void findNearestNeighbor(pcl::PointCloud<pcl::PointNormal>& body, MyMesh& head, std::unordered_map<unsigned, unsigned>& maping, double threshold, bool doubleCheck);
static void findNearestNeighbor2(pcl::PointCloud<pcl::PointXYZ> & body, pcl::PointCloud<pcl::PointXYZ>& head, std::unordered_map<unsigned, unsigned>& maping, double threshold, bool doubleCheck);
static void confirmNearestNeighbor2(pcl::PointCloud<pcl::PointXYZ> & from, pcl::PointCloud<pcl::PointXYZ> & to, std::unordered_map<unsigned, unsigned>& maping, double threshold);
//�õ��Ƶĵ����滻mesh�Ķ���
static void updateMesh(MyMesh& mesh, pcl::PointCloud<pcl::PointXYZ>::Ptr& cloud);
};
/*@}*/
}
99 static void confirmNearestNeighbor(pcl::PointCloud<pcl::PointXYZ>& from, pcl::PointCloud<pcl::PointNormal>& to, std::unordered_map<unsigned, unsigned>& maping, double threshold);
102 static void findNearestNeighbor(pcl::PointCloud<pcl::PointNormal>& body, MyMesh& head, std::unordered_map<unsigned, unsigned>& maping, double threshold, bool doubleCheck);
104 static void findNearestNeighbor2(pcl::PointCloud<pcl::PointXYZ> & body, pcl::PointCloud<pcl::PointXYZ>& head, std::unordered_map<unsigned, unsigned>& maping, double threshold, bool doubleCheck);
106 static void confirmNearestNeighbor2(pcl::PointCloud<pcl::PointXYZ> & from, pcl::PointCloud<pcl::PointXYZ> & to, std::unordered_map<unsigned, unsigned>& maping, double threshold);
108 //�õ��Ƶĵ����滻mesh�Ķ��� static void updateMesh(MyMesh& mesh, pcl::PointCloud<pcl::PointXYZ>::Ptr& cloud);
};
/*@}*/
}
double getLAMDA(unsigned index)
void printParam()
static void findNearestNeighbor(pcl::PointCloud< pcl::PointNormal > &body, MyMesh &head, std::unordered_map< unsigned, unsigned > &maping, double threshold, bool doubleCheck)
bool construct()
bool solve(Eigen::VectorXd &x)
Definition: AfterProcess.h:9
void updateHead(pcl::PointCloud< pcl::PointXYZ >::Ptr &head_cloud)
Definition: Setting.h:20
void setInput(pcl::PointCloud< pcl::PointNormal >::Ptr _body, MyMeshPtr _head)
Definition: TriSparse.h:56
static bool solveTriSparse(Eigen::SparseMatrix< double > &A, Eigen::VectorXd &B, Eigen::VectorXd &x)
static void confirmNearestNeighbor2(pcl::PointCloud< pcl::PointXYZ > &from, pcl::PointCloud< pcl::PointXYZ > &to, std::unordered_map< unsigned, unsigned > &maping, double threshold)
static void findNearestNeighbor2(pcl::PointCloud< pcl::PointXYZ > &body, pcl::PointCloud< pcl::PointXYZ > &head, std::unordered_map< unsigned, unsigned > &maping, double threshold, bool doubleCheck)
Definition: TriSparse.h:17
static void updateMesh(MyMesh &mesh, pcl::PointCloud< pcl::PointXYZ >::Ptr &cloud)
std::unordered_map< unsigned, unsigned > & getMap()
Definition: TriSparse.h:77
bool solveToCloud(pcl::PointCloud< pcl::PointXYZ >::Ptr &cloud)
pcl::PointCloud< pcl::PointNormal > & getBody()
Definition: TriSparse.h:75
static void confirmNearestNeighbor(pcl::PointCloud< pcl::PointNormal > &body, MyMesh &head_mesh, std::unordered_map< unsigned, unsigned > &maping, double threshold)
void setB(Eigen::VectorXd &_B)
TriSparse(pcl::PointCloud< pcl::PointNormal >::Ptr _body, MyMeshPtr _head)
Definition: TriSparse.h:37
void setA(Eigen::SparseMatrix< double > &_A)
void setParam(const double _THRESHOLD, const double _THETA, const double _LAMDA_MIN, const double _LAMDA_MAX, const double _ETA, bool _doubleCheck)
Generated by
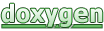