Inshaping
0.1
|
Setting.h
Go to the documentation of this file.
22 double theta;//�㵽��IJ��� double lamda_min;//������LAMDA,LAMDAԽ��Խ����
double lamda_max; //������LAMDA,LAMDAԽ��Խ����
double eta;//������ ETA ETAԽ��Խ�ܹ�������
double threshold;//������ ��Ӧ��������� THRESHOLD
std::string toString(int i)
{
return std::to_string(theta) + "_" + std::to_string(lamda_min) + "_" + std::to_string(lamda_max) + "_" + std::to_string(eta) + "_" + std::to_string(threshold) + std::to_string(i);
}
};
struct Param
{
std::vector<ParamItem> paramItems;
ParamItem finalParam;
void clear()
{
paramItems.clear();
}
};
class Setting:public Anyarg
{
public:
std::string headFilename;
std::string faceFilename;
std::string bodyFilename;
std::string outputFilename;
std::string subHead;
std::string subBody;
Param param;
bool preAligned; //�Ƿ�Ԥ�ȶ��� true ����Ӧ�ø�����
bool outputIntermediate;
bool useMatch;
bool readInputTemplate(std::string filename);
bool readMatch(std::string filename);
bool readParam(std::string filename);
Setting();
// parsing command line, collect command line arguments
bool parse_argv(int argc, char** argv);
};
/*@}*/
}
23 double lamda_min;//������LAMDA,LAMDAԽ��Խ���� double lamda_max; //������LAMDA,LAMDAԽ��Խ����
double eta;//������ ETA ETAԽ��Խ�ܹ�������
double threshold;//������ ��Ӧ��������� THRESHOLD
std::string toString(int i)
{
return std::to_string(theta) + "_" + std::to_string(lamda_min) + "_" + std::to_string(lamda_max) + "_" + std::to_string(eta) + "_" + std::to_string(threshold) + std::to_string(i);
}
};
struct Param
{
std::vector<ParamItem> paramItems;
ParamItem finalParam;
void clear()
{
paramItems.clear();
}
};
class Setting:public Anyarg
{
public:
std::string headFilename;
std::string faceFilename;
std::string bodyFilename;
std::string outputFilename;
std::string subHead;
std::string subBody;
Param param;
bool preAligned; //�Ƿ�Ԥ�ȶ��� true ����Ӧ�ø�����
bool outputIntermediate;
bool useMatch;
bool readInputTemplate(std::string filename);
bool readMatch(std::string filename);
bool readParam(std::string filename);
Setting();
// parsing command line, collect command line arguments
bool parse_argv(int argc, char** argv);
};
/*@}*/
}
24 double lamda_max; //������LAMDA,LAMDAԽ��Խ���� double eta;//������ ETA ETAԽ��Խ�ܹ�������
double threshold;//������ ��Ӧ��������� THRESHOLD
std::string toString(int i)
{
return std::to_string(theta) + "_" + std::to_string(lamda_min) + "_" + std::to_string(lamda_max) + "_" + std::to_string(eta) + "_" + std::to_string(threshold) + std::to_string(i);
}
};
struct Param
{
std::vector<ParamItem> paramItems;
ParamItem finalParam;
void clear()
{
paramItems.clear();
}
};
class Setting:public Anyarg
{
public:
std::string headFilename;
std::string faceFilename;
std::string bodyFilename;
std::string outputFilename;
std::string subHead;
std::string subBody;
Param param;
bool preAligned; //�Ƿ�Ԥ�ȶ��� true ����Ӧ�ø�����
bool outputIntermediate;
bool useMatch;
bool readInputTemplate(std::string filename);
bool readMatch(std::string filename);
bool readParam(std::string filename);
Setting();
// parsing command line, collect command line arguments
bool parse_argv(int argc, char** argv);
};
/*@}*/
}
29 return std::to_string(theta) + "_" + std::to_string(lamda_min) + "_" + std::to_string(lamda_max) + "_" + std::to_string(eta) + "_" + std::to_string(threshold) + std::to_string(i);
bool readParam(std::string filename)
Definition: AfterProcess.h:9
Definition: Setting.h:20
bool readMatch(std::string filename)
bool parse_argv(int argc, char **argv)
A simple option parser for C++.
Definition: Setting.h:44
Use this class to define program options and parse command line arguments.
Definition: anyarg.h:97
Setting()
bool readInputTemplate(std::string filename)
Definition: Setting.h:33
Generated by
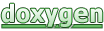