Inshaping
0.1
|
Plane.h
Go to the documentation of this file.
35 //�㷨ʽ��ƽ�淽�� inline Plane(Eigen::Vector3d normal, Eigen::Vector3d point) { pointNormForm(normal, point); }
//�������㣬�����ƽ��
inline Plane(std::vector<Eigen::Vector3d>& ptArr) { FromThreePt(ptArr); }
//�ͷ��������һ��
bool belowPlane(Eigen::Vector3d point, double offset);
//���ö��ַ�������D��ʹmatch���ӽ�minMatch
//bool adjustToTargetMatch(pcl::PointCloud<pcl::PointXYZ>& body,pcl::PointCloud<pcl::PointXYZ>& head,int traget_match,double threshold);
//����ƽ��ָ���� POSITON ����Ҫ����ĵ��Ƶĵ�λ��
void segmentCloud(pcl::PointCloud<pcl::PointXYZ>& cloud, pcl::PointCloud<pcl::PointXYZ>& output, Position position, double offset);
//����ƽ��ָ���� POSITON ����Ҫ����ĵ��Ƶĵ�λ��
void segmentMesh(MyMesh& mesh, MyMesh& output, Position position, double offset = 0);
//�㷨ʽ
bool pointNormForm(Eigen::Vector3d normal, Eigen::Vector3d point);
//����ȷ��һ��ƽ��
bool FromThreePt(std::vector<Eigen::Vector3d>& ptArr);
inline friend std::ostream& operator<<(std::ostream& os, const Plane& plane)
{
os << "A=" << plane.Normal.x() << ",B=" << plane.Normal.y() << ",C=" << plane.Normal.z() << ",D=" << plane.D << std::endl;
return os;
}
};
/*@}*/
}
41 //�ͷ��������һ�� bool belowPlane(Eigen::Vector3d point, double offset);
//���ö��ַ�������D��ʹmatch���ӽ�minMatch
//bool adjustToTargetMatch(pcl::PointCloud<pcl::PointXYZ>& body,pcl::PointCloud<pcl::PointXYZ>& head,int traget_match,double threshold);
//����ƽ��ָ���� POSITON ����Ҫ����ĵ��Ƶĵ�λ��
void segmentCloud(pcl::PointCloud<pcl::PointXYZ>& cloud, pcl::PointCloud<pcl::PointXYZ>& output, Position position, double offset);
//����ƽ��ָ���� POSITON ����Ҫ����ĵ��Ƶĵ�λ��
void segmentMesh(MyMesh& mesh, MyMesh& output, Position position, double offset = 0);
//�㷨ʽ
bool pointNormForm(Eigen::Vector3d normal, Eigen::Vector3d point);
//����ȷ��һ��ƽ��
bool FromThreePt(std::vector<Eigen::Vector3d>& ptArr);
inline friend std::ostream& operator<<(std::ostream& os, const Plane& plane)
{
os << "A=" << plane.Normal.x() << ",B=" << plane.Normal.y() << ",C=" << plane.Normal.z() << ",D=" << plane.D << std::endl;
return os;
}
};
/*@}*/
}
45 //bool adjustToTargetMatch(pcl::PointCloud<pcl::PointXYZ>& body,pcl::PointCloud<pcl::PointXYZ>& head,int traget_match,double threshold);
49 void segmentCloud(pcl::PointCloud<pcl::PointXYZ>& cloud, pcl::PointCloud<pcl::PointXYZ>& output, Position position, double offset);
62 os << "A=" << plane.Normal.x() << ",B=" << plane.Normal.y() << ",C=" << plane.Normal.z() << ",D=" << plane.D << std::endl;
Definition: Plane.h:13
bool pointNormForm(Eigen::Vector3d normal, Eigen::Vector3d point)
friend std::ostream & operator<<(std::ostream &os, const Plane &plane)
Definition: Plane.h:60
Definition: AfterProcess.h:9
void segmentMesh(MyMesh &mesh, MyMesh &output, Position position, double offset=0)
bool belowPlane(Eigen::Vector3d point, double offset)
void segmentCloud(pcl::PointCloud< pcl::PointXYZ > &cloud, pcl::PointCloud< pcl::PointXYZ > &output, Position position, double offset)
Definition: Plane.h:18
bool FromThreePt(std::vector< Eigen::Vector3d > &ptArr)
Definition: Plane.h:18
Generated by
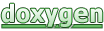