Inshaping
0.1
|
Normal.h
Go to the documentation of this file.
40 WArc(pcl::PointNormal& _s, int is, pcl::PointNormal& _t, int it) :src({ &_s, is }), trg({ &_t,it }), w(0)
55 //����һ���Բ��� void setCoherence(int _coherence)//
{
coherence = _coherence;
}
//����һ���Բ���
int getCoherence()
{
return coherence;
}
pcl::PointCloud<pcl::PointNormal>::Ptr getCoherencyPointNormals();
static void AddNeighboursToHeap(pcl::PointCloud<pcl::PointNormal>::Ptr cloud_with_normals, int index, int K, pcl::search::KdTree<pcl::PointNormal>::Ptr &tree, std::vector<WArc> &heap, std::shared_ptr<bool>& visited);
static void coherencyPass(pcl::PointCloud<pcl::PointNormal>::Ptr cloud_with_normals, int coherence = 8);
};
/*@}*/
}
61 //����һ���Բ��� int getCoherence()
{
return coherence;
}
pcl::PointCloud<pcl::PointNormal>::Ptr getCoherencyPointNormals();
static void AddNeighboursToHeap(pcl::PointCloud<pcl::PointNormal>::Ptr cloud_with_normals, int index, int K, pcl::search::KdTree<pcl::PointNormal>::Ptr &tree, std::vector<WArc> &heap, std::shared_ptr<bool>& visited);
static void coherencyPass(pcl::PointCloud<pcl::PointNormal>::Ptr cloud_with_normals, int coherence = 8);
};
/*@}*/
}
69 static void AddNeighboursToHeap(pcl::PointCloud<pcl::PointNormal>::Ptr cloud_with_normals, int index, int K, pcl::search::KdTree<pcl::PointNormal>::Ptr &tree, std::vector<WArc> &heap, std::shared_ptr<bool>& visited);
71 static void coherencyPass(pcl::PointCloud<pcl::PointNormal>::Ptr cloud_with_normals, int coherence = 8);
void invertNormal()
Definition: Normal.h:32
Eigen::Vector3f normalCopy()
Definition: Normal.h:27
Definition: AfterProcess.h:9
pcl::PointCloud< pcl::PointNormal >::Ptr getCoherencyPointNormals()
static void coherencyPass(pcl::PointCloud< pcl::PointNormal >::Ptr cloud_with_normals, int coherence=8)
Definition: Normal.h:21
Definition: Normal.h:12
static void AddNeighboursToHeap(pcl::PointCloud< pcl::PointNormal >::Ptr cloud_with_normals, int index, int K, pcl::search::KdTree< pcl::PointNormal >::Ptr &tree, std::vector< WArc > &heap, std::shared_ptr< bool > &visited)
Generated by
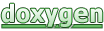