Inshaping
0.1
|
HyperCloud.h
Go to the documentation of this file.
59 //���ص��Eigen::Vector3d ���ͣ����Ƕ���������IJ����Dz���Ӱ�쵽Դ���Ƶ� inline virtual const Eigen::Vector3d getEigenVec(unsigned index);
inline virtual const pcl::PointXYZ getPointXYZ(unsigned index);
//��������
Eigen::Vector3d getBaryCenter();
void addCloud(CCLib::HyperCloud& cloud);
virtual void getPoint(unsigned index, CCVector3& P) const;
//**** inherited form GenericIndexedCloudPersist ****//
virtual const CCVector3* getPointPersistentPtr(unsigned index);
//! Clears cloud
void clear();
//! Point insertion mechanism
/** The point data will be duplicated in memory.
\param P the point to insert
**/
virtual void addPoint(const CCVector3 &P);
virtual void addPoint(const Eigen::Vector3d& p);
//! Point insertion mechanism
/** The point data will be duplicated in memory.
\param P the point to insert (as a 3-size array)
**/
virtual void addPoint(const PointCoordinateType P[]);
//! Reserves some memory for hosting the points
/** \param n the number of points
**/
virtual bool reserve(unsigned n);
//! Presets the size of the vector used to store the points
/** \param n the number of points
**/
virtual bool resize(unsigned n);
//! Applies a rigid transformation to the cloud
/** WARNING: THIS METHOD IS NOT COMPATIBLE WITH PARALLEL STRATEGIES
\param trans transformation (scale * rotation matrix + translation vector)
**/
virtual void applyTransformation(PointProjectionTools::Transformation& trans);
//! Returns associated scalar field (if any)
ScalarField* getScalarField() { return m_scalarField; }
//! Returns associated scalar field (if any) (const version)
const ScalarField* getScalarField() const { return m_scalarField; }
protected:
//! Point container
typedef GenericChunkedArray<3, PointCoordinateType> PointsContainer;
//! 3D Points container
PointsContainer* m_points;
//! The points distances
ScalarField* m_scalarField;
//! Iterator on the points container
unsigned globalIterator;
//! Bounding-box validity
bool m_validBB;
};
/*@}*/
}
#endif //HYPER_CLOUD_HEADER
64 //�������� Eigen::Vector3d getBaryCenter();
void addCloud(CCLib::HyperCloud& cloud);
virtual void getPoint(unsigned index, CCVector3& P) const;
//**** inherited form GenericIndexedCloudPersist ****//
virtual const CCVector3* getPointPersistentPtr(unsigned index);
//! Clears cloud
void clear();
//! Point insertion mechanism
/** The point data will be duplicated in memory.
\param P the point to insert
**/
virtual void addPoint(const CCVector3 &P);
virtual void addPoint(const Eigen::Vector3d& p);
//! Point insertion mechanism
/** The point data will be duplicated in memory.
\param P the point to insert (as a 3-size array)
**/
virtual void addPoint(const PointCoordinateType P[]);
//! Reserves some memory for hosting the points
/** \param n the number of points
**/
virtual bool reserve(unsigned n);
//! Presets the size of the vector used to store the points
/** \param n the number of points
**/
virtual bool resize(unsigned n);
//! Applies a rigid transformation to the cloud
/** WARNING: THIS METHOD IS NOT COMPATIBLE WITH PARALLEL STRATEGIES
\param trans transformation (scale * rotation matrix + translation vector)
**/
virtual void applyTransformation(PointProjectionTools::Transformation& trans);
//! Returns associated scalar field (if any)
ScalarField* getScalarField() { return m_scalarField; }
//! Returns associated scalar field (if any) (const version)
const ScalarField* getScalarField() const { return m_scalarField; }
protected:
//! Point container
typedef GenericChunkedArray<3, PointCoordinateType> PointsContainer;
//! 3D Points container
PointsContainer* m_points;
//! The points distances
ScalarField* m_scalarField;
//! Iterator on the points container
unsigned globalIterator;
//! Bounding-box validity
bool m_validBB;
};
/*@}*/
}
#endif //HYPER_CLOUD_HEADER
const ScalarField * getScalarField() const
Returns associated scalar field (if any) (const version)
Definition: HyperCloud.h:114
ScalarField * m_scalarField
The points distances.
Definition: HyperCloud.h:126
GenericChunkedArray< 3, PointCoordinateType > PointsContainer
Point container.
Definition: HyperCloud.h:119
Definition: HyperCloud.h:17
PointsContainer * m_points
3D Points container
Definition: HyperCloud.h:122
virtual const CCVector3 * getPoint(unsigned index)
Definition: HyperCloud.h:54
An Extended point cloud (with point duplication mechanism)
Definition: HyperCloud.h:29
ScalarField * getScalarField()
Returns associated scalar field (if any)
Definition: HyperCloud.h:111
unsigned globalIterator
Iterator on the points container.
Definition: HyperCloud.h:129
Generated by
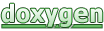