STSW-STLKT01
|
SensorTile_gyro.h
Go to the documentation of this file.
DrvStatusTypeDef BSP_GYRO_Sensor_Enable(void *handle)
Enable gyroscope sensor.
Definition: SensorTile_gyro.c:236
DrvStatusTypeDef BSP_GYRO_Set_ODR_Value(void *handle, float odr)
Set the gyroscope sensor output data rate.
Definition: SensorTile_gyro.c:671
DrvStatusTypeDef BSP_GYRO_IsCombo(void *handle, uint8_t *status)
Check if the gyroscope sensor is combo.
Definition: SensorTile_gyro.c:358
DrvStatusTypeDef BSP_GYRO_IsEnabled(void *handle, uint8_t *status)
Check if the gyroscope sensor is enabled.
Definition: SensorTile_gyro.c:331
DrvStatusTypeDef BSP_GYRO_Init(GYRO_ID_t id, void **handle)
Initialize a gyroscope sensor.
Definition: SensorTile_gyro.c:88
DrvStatusTypeDef BSP_GYRO_Set_FS_Value(void *handle, float fullScale)
Set the gyroscope sensor full scale.
Definition: SensorTile_gyro.c:777
DrvStatusTypeDef BSP_GYRO_Get_FS(void *handle, float *fullScale)
Get the gyroscope sensor full scale.
Definition: SensorTile_gyro.c:705
DrvStatusTypeDef BSP_GYRO_Get_AxesRaw(void *handle, SensorAxesRaw_t *value)
Get the gyroscope sensor raw axes.
Definition: SensorTile_gyro.c:522
DrvStatusTypeDef BSP_GYRO_Check_WhoAmI(void *handle)
Check the WHO_AM_I ID of the gyroscope sensor.
Definition: SensorTile_gyro.c:450
DrvStatusTypeDef BSP_GYRO_Get_WhoAmI(void *handle, uint8_t *who_am_i)
Get the WHO_AM_I ID of the gyroscope sensor.
Definition: SensorTile_gyro.c:413
DrvStatusTypeDef BSP_GYRO_Write_Reg(void *handle, uint8_t reg, uint8_t data)
Write the data to register.
Definition: SensorTile_gyro.c:889
DrvStatusTypeDef BSP_GYRO_Read_Reg(void *handle, uint8_t reg, uint8_t *data)
Read the data from register.
Definition: SensorTile_gyro.c:849
DrvStatusTypeDef BSP_GYRO_Set_Axes_Status(void *handle, uint8_t *enable_xyz)
Set the enabled/disabled status of the gyroscope sensor axes.
Definition: SensorTile_gyro.c:957
DrvStatusTypeDef BSP_GYRO_DeInit(void **handle)
Deinitialize a gyroscope sensor.
Definition: SensorTile_gyro.c:198
DrvStatusTypeDef BSP_GYRO_Set_FS(void *handle, SensorFs_t fullScale)
Set the gyroscope sensor full scale.
Definition: SensorTile_gyro.c:743
This file contains definitions for SensorTile.c file.
DrvStatusTypeDef BSP_GYRO_Get_Sensitivity(void *handle, float *sensitivity)
Get the gyroscope sensor sensitivity.
Definition: SensorTile_gyro.c:561
DrvStatusTypeDef BSP_GYRO_Get_Instance(void *handle, uint8_t *instance)
Get the gyroscope sensor instance.
Definition: SensorTile_gyro.c:385
DrvStatusTypeDef BSP_GYRO_Sensor_Disable(void *handle)
Disable gyroscope sensor.
Definition: SensorTile_gyro.c:270
DrvStatusTypeDef BSP_GYRO_Get_DRDY_Status(void *handle, uint8_t *status)
Get gyroscope data ready status.
Definition: SensorTile_gyro.c:923
DrvStatusTypeDef BSP_GYRO_Get_Axes_Status(void *handle, uint8_t *xyz_enabled)
Get the gyroscope sensor axes status.
Definition: SensorTile_gyro.c:811
DrvStatusTypeDef BSP_GYRO_Set_ODR(void *handle, SensorOdr_t odr)
Set the gyroscope sensor output data rate.
Definition: SensorTile_gyro.c:637
DrvStatusTypeDef BSP_GYRO_IsInitialized(void *handle, uint8_t *status)
Check if the gyroscope sensor is initialized.
Definition: SensorTile_gyro.c:304
DrvStatusTypeDef BSP_GYRO_Get_ODR(void *handle, float *odr)
Get the gyroscope sensor output data rate.
Definition: SensorTile_gyro.c:599
DrvStatusTypeDef BSP_GYRO_Get_Axes(void *handle, SensorAxes_t *angular_velocity)
Get the gyroscope sensor axes.
Definition: SensorTile_gyro.c:484
Generated by
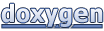