b2World Class Reference
The world class manages all physics entities, dynamic simulation, and asynchronous queries. More...
Public Member Functions | |
b2World (const b2AABB &worldAABB, const b2Vec2 &gravity, bool doSleep) | |
Construct a world object. | |
~b2World () | |
Destruct the world. All physics entities are destroyed and all heap memory is released. | |
void | SetDestructionListener (b2DestructionListener *listener) |
Register a destruction listener. | |
void | SetBoundaryListener (b2BoundaryListener *listener) |
Register a broad-phase boundary listener. | |
void | SetContactFilter (b2ContactFilter *filter) |
Register a contact filter to provide specific control over collision. | |
void | SetContactListener (b2ContactListener *listener) |
Register a contact event listener. | |
void | SetDebugDraw (b2DebugDraw *debugDraw) |
Register a routine for debug drawing. | |
b2Body * | CreateBody (const b2BodyDef *def) |
Create a rigid body given a definition. | |
void | DestroyBody (b2Body *body) |
Destroy a rigid body given a definition. | |
b2Joint * | CreateJoint (const b2JointDef *def) |
Create a joint to constrain bodies together. | |
void | DestroyJoint (b2Joint *joint) |
Destroy a joint. | |
b2Body * | GetGroundBody () |
The world provides a single static ground body with no collision shapes. | |
void | Step (float32 timeStep, int32 iterations) |
Take a time step. | |
int32 | Query (const b2AABB &aabb, b2Shape **shapes, int32 maxCount) |
Query the world for all shapes that potentially overlap the provided AABB. | |
b2Body * | GetBodyList () |
Get the world body list. | |
b2Joint * | GetJointList () |
Get the world joint list. | |
void | Refilter (b2Shape *shape) |
Re-filter a shape. This re-runs contact filtering on a shape. | |
void | SetWarmStarting (bool flag) |
Enable/disable warm starting. For testing. | |
void | SetPositionCorrection (bool flag) |
Enable/disable position correction. For testing. | |
void | SetContinuousPhysics (bool flag) |
Enable/disable continuous physics. For testing. | |
void | Validate () |
Perform validation of internal data structures. | |
int32 | GetProxyCount () const |
Get the number of broad-phase proxies. | |
int32 | GetPairCount () const |
Get the number of broad-phase pairs. | |
int32 | GetBodyCount () const |
Get the number of bodies. | |
int32 | GetJointCount () const |
Get the number joints. | |
int32 | GetContactCount () const |
Get the number of contacts (each may have 0 or more contact points). | |
void | SetGravity (const b2Vec2 &gravity) |
Change the global gravity vector. | |
Friends | |
class | b2Body |
Detailed Description
The world class manages all physics entities, dynamic simulation, and asynchronous queries.The world also contains efficient memory management facilities.
Constructor & Destructor Documentation
Construct a world object.
- Parameters:
-
worldAABB a bounding box that completely encompasses all your shapes. gravity the world gravity vector. doSleep improve performance by not simulating inactive bodies.
Member Function Documentation
void b2World::SetContactFilter | ( | b2ContactFilter * | filter | ) |
Register a contact filter to provide specific control over collision.
Otherwise the default filter is used (b2_defaultFilter).
void b2World::SetDebugDraw | ( | b2DebugDraw * | debugDraw | ) |
Register a routine for debug drawing.
The debug draw functions are called inside the b2World::Step method, so make sure your renderer is ready to consume draw commands when you call Step().
Create a rigid body given a definition.
No reference to the definition is retained.
- Warning:
- This function is locked during callbacks.
void b2World::DestroyBody | ( | b2Body * | body | ) |
Destroy a rigid body given a definition.
No reference to the definition is retained. This function is locked during callbacks.
- Warning:
- This automatically deletes all associated shapes and joints.
This function is locked during callbacks.
b2Joint * b2World::CreateJoint | ( | const b2JointDef * | def | ) |
Create a joint to constrain bodies together.
No reference to the definition is retained. This may cause the connected bodies to cease colliding.
- Warning:
- This function is locked during callbacks.
void b2World::DestroyJoint | ( | b2Joint * | joint | ) |
Destroy a joint.
This may cause the connected bodies to begin colliding.
- Warning:
- This function is locked during callbacks.
b2Body * b2World::GetGroundBody | ( | ) | [inline] |
The world provides a single static ground body with no collision shapes.
You can use this to simplify the creation of joints and static shapes.
void b2World::Step | ( | float32 | timeStep, | |
int32 | iterations | |||
) |
Take a time step.
This performs collision detection, integration, and constraint solution.
- Parameters:
-
timeStep the amount of time to simulate, this should not vary. iterations the number of iterations to be used by the constraint solver.
Query the world for all shapes that potentially overlap the provided AABB.
You provide a shape pointer buffer of specified size. The number of shapes found is returned.
- Parameters:
-
aabb the query box. shapes a user allocated shape pointer array of size maxCount (or greater). maxCount the capacity of the shapes array.
- Returns:
- the number of shapes found in aabb.
b2Body * b2World::GetBodyList | ( | ) | [inline] |
Get the world body list.
With the returned body, use b2Body::GetNext to get the next body in the world list. A NULL body indicates the end of the list.
- Returns:
- the head of the world body list.
b2Joint * b2World::GetJointList | ( | ) | [inline] |
Get the world joint list.
With the returned joint, use b2Joint::GetNext to get the next joint in the world list. A NULL joint indicates the end of the list.
- Returns:
- the head of the world joint list.
The documentation for this class was generated from the following files:
- b2World.h
- b2World.cpp
Generated on Sun Apr 13 15:21:27 2008 for Box2D by
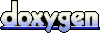