b2Body Class Reference
A rigid body. More...
Public Member Functions | |
b2Shape * | CreateShape (b2ShapeDef *shapeDef) |
Creates a shape and attach it to this body. | |
void | DestroyShape (b2Shape *shape) |
Destroy a shape. | |
void | SetMass (const b2MassData *massData) |
Set the mass properties. | |
void | SetMassFromShapes () |
Compute the mass properties from the attached shapes. | |
bool | SetXForm (const b2Vec2 &position, float32 angle) |
Set the position of the body's origin and rotation (radians). | |
const b2XForm & | GetXForm () const |
Get the body transform for the body's origin. | |
const b2Vec2 & | GetPosition () const |
Get the world body origin position. | |
float32 | GetAngle () const |
Get the angle in radians. | |
const b2Vec2 & | GetWorldCenter () const |
Get the world position of the center of mass. | |
const b2Vec2 & | GetLocalCenter () const |
Get the local position of the center of mass. | |
void | SetLinearVelocity (const b2Vec2 &v) |
Set the linear velocity of the center of mass. | |
b2Vec2 | GetLinearVelocity () const |
Get the linear velocity of the center of mass. | |
void | SetAngularVelocity (float32 omega) |
Set the angular velocity. | |
float32 | GetAngularVelocity () const |
Get the angular velocity. | |
void | ApplyForce (const b2Vec2 &force, const b2Vec2 &point) |
Apply a force at a world point. | |
void | ApplyTorque (float32 torque) |
Apply a torque. | |
void | ApplyImpulse (const b2Vec2 &impulse, const b2Vec2 &point) |
Apply an impulse at a point. | |
float32 | GetMass () const |
Get the total mass of the body. | |
float32 | GetInertia () const |
Get the central rotational inertia of the body. | |
b2Vec2 | GetWorldPoint (const b2Vec2 &localPoint) const |
Get the world coordinates of a point given the local coordinates. | |
b2Vec2 | GetWorldVector (const b2Vec2 &localVector) const |
Get the world coordinates of a vector given the local coordinates. | |
b2Vec2 | GetLocalPoint (const b2Vec2 &worldPoint) const |
Gets a local point relative to the body's origin given a world point. | |
b2Vec2 | GetLocalVector (const b2Vec2 &worldVector) const |
Gets a local vector given a world vector. | |
b2Vec2 | GetLinearVelocityFromWorldPoint (const b2Vec2 &worldPoint) const |
Get the world linear velocity of a world point attached to this body. | |
b2Vec2 | GetLinearVelocityFromLocalPoint (const b2Vec2 &localPoint) const |
Get the world velocity of a local point. | |
bool | IsBullet () const |
Is this body treated like a bullet for continuous collision detection? | |
void | SetBullet (bool flag) |
Should this body be treated like a bullet for continuous collision detection? | |
bool | IsStatic () const |
Is this body static (immovable)? | |
bool | IsDynamic () const |
Is this body dynamic (movable)? | |
bool | IsFrozen () const |
Is this body frozen? | |
bool | IsSleeping () const |
Is this body sleeping (not simulating). | |
void | AllowSleeping (bool flag) |
You can disable sleeping on this body. | |
void | WakeUp () |
Wake up this body so it will begin simulating. | |
void | PutToSleep () |
Put this body to sleep so it will stop simulating. | |
b2Shape * | GetShapeList () |
Get the list of all shapes attached to this body. | |
b2JointEdge * | GetJointList () |
Get the list of all joints attached to this body. | |
b2Body * | GetNext () |
Get the next body in the world's body list. | |
void * | GetUserData () |
Get the user data pointer that was provided in the body definition. | |
void | SetUserData (void *data) |
Set the user data. Use this to store your application specific data. | |
b2World * | GetWorld () |
Get the parent world of this body. | |
Friends | |
class | b2World |
class | b2DistanceJoint |
class | b2GearJoint |
class | b2MouseJoint |
class | b2PrismaticJoint |
class | b2PulleyJoint |
class | b2RevoluteJoint |
Detailed Description
A rigid body.Member Function Documentation
b2Shape * b2Body::CreateShape | ( | b2ShapeDef * | shapeDef | ) |
Creates a shape and attach it to this body.
- Parameters:
-
shapeDef the shape definition.
- Warning:
- This function is locked during callbacks.
void b2Body::DestroyShape | ( | b2Shape * | shape | ) |
Destroy a shape.
This removes the shape from the broad-phase and therefore destroys any contacts associated with this shape. All shapes attached to a body are implicitly destroyed when the body is destroyed.
- Parameters:
-
shape the shape to be removed.
- Warning:
- This function is locked during callbacks.
void b2Body::SetMass | ( | const b2MassData * | massData | ) |
Set the mass properties.
Note that this changes the center of mass position. If you are not sure how to compute mass properties, use SetMassFromShapes. The inertia tensor is assumed to be relative to the center of mass.
- Parameters:
-
massData the mass properties.
void b2Body::SetMassFromShapes | ( | ) |
Compute the mass properties from the attached shapes.
You typically call this after adding all the shapes. If you add or remove shapes later, you may want to call this again. Note that this changes the center of mass position.
bool b2Body::SetXForm | ( | const b2Vec2 & | position, | |
float32 | angle | |||
) |
Set the position of the body's origin and rotation (radians).
This breaks any contacts and wakes the other bodies.
- Parameters:
-
position the new world position of the body's origin (not necessarily the center of mass). angle the new world rotation angle of the body in radians.
- Returns:
- false if the movement put a shape outside the world. In this case the body is automatically frozen.
const b2XForm & b2Body::GetXForm | ( | ) | const [inline] |
Get the body transform for the body's origin.
- Returns:
- the world transform of the body's origin.
const b2Vec2 & b2Body::GetPosition | ( | ) | const [inline] |
Get the world body origin position.
- Returns:
- the world position of the body's origin.
float32 b2Body::GetAngle | ( | ) | const [inline] |
Get the angle in radians.
- Returns:
- the current world rotation angle in radians.
void b2Body::SetLinearVelocity | ( | const b2Vec2 & | v | ) | [inline] |
Set the linear velocity of the center of mass.
- Parameters:
-
v the new linear velocity of the center of mass.
b2Vec2 b2Body::GetLinearVelocity | ( | ) | const [inline] |
Get the linear velocity of the center of mass.
- Returns:
- the linear velocity of the center of mass.
void b2Body::SetAngularVelocity | ( | float32 | omega | ) | [inline] |
Set the angular velocity.
- Parameters:
-
omega the new angular velocity in radians/second.
float32 b2Body::GetAngularVelocity | ( | ) | const [inline] |
Get the angular velocity.
- Returns:
- the angular velocity in radians/second.
Apply a force at a world point.
If the force is not applied at the center of mass, it will generate a torque and affect the angular velocity. This wakes up the body.
- Parameters:
-
force the world force vector, usually in Newtons (N). point the world position of the point of application.
void b2Body::ApplyTorque | ( | float32 | torque | ) | [inline] |
Apply a torque.
This affects the angular velocity without affecting the linear velocity of the center of mass. This wakes up the body.
- Parameters:
-
torque about the z-axis (out of the screen), usually in N-m.
Apply an impulse at a point.
This immediately modifies the velocity. It also modifies the angular velocity if the point of application is not at the center of mass. This wakes up the body.
- Parameters:
-
impulse the world impulse vector, usually in N-seconds or kg-m/s. point the world position of the point of application.
float32 b2Body::GetMass | ( | ) | const [inline] |
Get the total mass of the body.
- Returns:
- the mass, usually in kilograms (kg).
float32 b2Body::GetInertia | ( | ) | const [inline] |
Get the central rotational inertia of the body.
- Returns:
- the rotational inertia, usually in kg-m^2.
Get the world coordinates of a point given the local coordinates.
- Parameters:
-
localPoint a point on the body measured relative the the body's origin.
- Returns:
- the same point expressed in world coordinates.
Get the world coordinates of a vector given the local coordinates.
- Parameters:
-
localVector a vector fixed in the body.
- Returns:
- the same vector expressed in world coordinates.
Gets a local point relative to the body's origin given a world point.
- Parameters:
-
a point in world coordinates.
- Returns:
- the corresponding local point relative to the body's origin.
Gets a local vector given a world vector.
- Parameters:
-
a vector in world coordinates.
- Returns:
- the corresponding local vector.
Get the world linear velocity of a world point attached to this body.
- Parameters:
-
a point in world coordinates.
- Returns:
- the world velocity of a point.
Get the world velocity of a local point.
- Parameters:
-
a point in local coordinates.
- Returns:
- the world velocity of a point.
void b2Body::PutToSleep | ( | ) | [inline] |
Put this body to sleep so it will stop simulating.
This also sets the velocity to zero.
The documentation for this class was generated from the following files:
- b2Body.h
- b2Body.cpp
Generated on Sun Apr 13 15:21:27 2008 for Box2D by
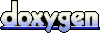