b2Shape Class Reference
A shape is used for collision detection. More...
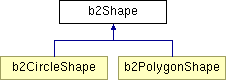
Public Member Functions | |
b2ShapeType | GetType () const |
Get the type of this shape. | |
bool | IsSensor () const |
Is this shape a sensor (non-solid)? | |
void | SetFilterData (const b2FilterData &filter) |
Set the contact filtering data. | |
const b2FilterData & | GetFilterData () const |
Get the contact filtering data. | |
b2Body * | GetBody () |
Get the parent body of this shape. | |
b2Shape * | GetNext () |
Get the next shape in the parent body's shape list. | |
void * | GetUserData () |
Get the user data that was assigned in the shape definition. | |
void | SetUserData (void *data) |
Set the user data. Use this to store your application specific data. | |
virtual bool | TestPoint (const b2XForm &xf, const b2Vec2 &p) const =0 |
Test a point for containment in this shape. | |
virtual bool | TestSegment (const b2XForm &xf, float32 *lambda, b2Vec2 *normal, const b2Segment &segment, float32 maxLambda) const =0 |
Perform a ray cast against this shape. | |
virtual void | ComputeAABB (b2AABB *aabb, const b2XForm &xf) const =0 |
Given a transform, compute the associated axis aligned bounding box for this shape. | |
virtual void | ComputeSweptAABB (b2AABB *aabb, const b2XForm &xf1, const b2XForm &xf2) const =0 |
Given two transforms, compute the associated swept axis aligned bounding box for this shape. | |
virtual void | ComputeMass (b2MassData *massData) const =0 |
Compute the mass properties of this shape using its dimensions and density. | |
float32 | GetSweepRadius () const |
Get the maximum radius about the parent body's center of mass. | |
float32 | GetFriction () const |
Get the coefficient of friction. | |
float32 | GetRestitution () const |
Get the coefficient of restitution. | |
Friends | |
class | b2Body |
class | b2World |
Detailed Description
A shape is used for collision detection.Shapes are created in b2World. You can use shape for collision detection before they are attached to the world.
- Warning:
- you cannot reuse shapes.
Member Function Documentation
b2ShapeType b2Shape::GetType | ( | ) | const [inline] |
Get the type of this shape.
You can use this to down cast to the concrete shape.
- Returns:
- the shape type.
bool b2Shape::IsSensor | ( | ) | const [inline] |
Is this shape a sensor (non-solid)?
- Returns:
- the true if the shape is a sensor.
void b2Shape::SetFilterData | ( | const b2FilterData & | filter | ) | [inline] |
Set the contact filtering data.
You must call b2World::Refilter to correct existing contacts/non-contacts.
b2Body * b2Shape::GetBody | ( | ) | [inline] |
Get the parent body of this shape.
This is NULL if the shape is not attached.
- Returns:
- the parent body.
b2Shape * b2Shape::GetNext | ( | ) | [inline] |
Get the next shape in the parent body's shape list.
- Returns:
- the next shape.
void * b2Shape::GetUserData | ( | ) | [inline] |
Get the user data that was assigned in the shape definition.
Use this to store your application specific data.
Test a point for containment in this shape.
This only works for convex shapes.
- Parameters:
-
xf the shape world transform. p a point in world coordinates.
Implemented in b2CircleShape, and b2PolygonShape.
virtual bool b2Shape::TestSegment | ( | const b2XForm & | xf, | |
float32 * | lambda, | |||
b2Vec2 * | normal, | |||
const b2Segment & | segment, | |||
float32 | maxLambda | |||
) | const [pure virtual] |
Perform a ray cast against this shape.
- Parameters:
-
xf the shape world transform. lambda returns the hit fraction. You can use this to compute the contact point p = (1 - lambda) * segment.p1 + lambda * segment.p2. normal returns the normal at the contact point. If there is no intersection, the normal is not set. segment defines the begin and end point of the ray cast. maxLambda a number typically in the range [0,1].
- Returns:
- true if there was an intersection.
Implemented in b2CircleShape, and b2PolygonShape.
Given a transform, compute the associated axis aligned bounding box for this shape.
- Parameters:
-
aabb returns the axis aligned box. xf the world transform of the shape.
Implemented in b2CircleShape, and b2PolygonShape.
virtual void b2Shape::ComputeSweptAABB | ( | b2AABB * | aabb, | |
const b2XForm & | xf1, | |||
const b2XForm & | xf2 | |||
) | const [pure virtual] |
Given two transforms, compute the associated swept axis aligned bounding box for this shape.
- Parameters:
-
aabb returns the axis aligned box. xf1 the starting shape world transform. xf2 the ending shape world transform.
Implemented in b2CircleShape, and b2PolygonShape.
virtual void b2Shape::ComputeMass | ( | b2MassData * | massData | ) | const [pure virtual] |
Compute the mass properties of this shape using its dimensions and density.
The inertia tensor is computed about the local origin, not the centroid.
- Parameters:
-
massData returns the mass data for this shape.
Implemented in b2CircleShape, and b2PolygonShape.
The documentation for this class was generated from the following files:
- b2Shape.h
- b2Shape.cpp
Generated on Sun Apr 13 15:21:27 2008 for Box2D by
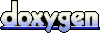