physx::shdfnd::Socket Class Reference
#include <PsSocket.h>
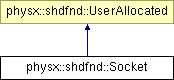
Public Member Functions | |
Socket (bool inEnableBuffering=true, bool blocking=true) | |
virtual | ~Socket () |
bool | connect (const char *host, uint16_t port, uint32_t timeout=1000) |
bool | listen (uint16_t port) |
bool | accept (bool block) |
void | disconnect () |
bool | isConnected () const |
const char * | getHost () const |
uint16_t | getPort () const |
bool | flush () |
uint32_t | write (const uint8_t *data, uint32_t length) |
uint32_t | read (uint8_t *data, uint32_t length) |
void | setBlocking (bool blocking) |
bool | isBlocking () const |
Static Public Attributes | |
static const uint32_t | DEFAULT_BUFFER_SIZE = 32768 |
Detailed Description
Socket abstraction APIConstructor & Destructor Documentation
physx::shdfnd::Socket::Socket | ( | bool | inEnableBuffering = true , |
|
bool | blocking = true | |||
) |
physx::shdfnd::Socket::~Socket | ( | ) | [virtual] |
Member Function Documentation
bool physx::shdfnd::Socket::accept | ( | bool | block | ) |
Accept a connection on a socket that is in listening mode
- Note:
- This method only supports a single connection client. Additional clients that connect to the listening port will overwrite the existing socket handle.
This method is not implemented on Windows Modern.
- Parameters:
-
block whether or not the call should block
- Returns:
- whether a connection was established
bool physx::shdfnd::Socket::connect | ( | const char * | host, | |
uint16_t | port, | |||
uint32_t | timeout = 1000 | |||
) |
Opens a network socket for input and/or output
- Parameters:
-
host Name of the host to connect to. This can be an IP, URL, etc port The port to connect to on the remote host timeout Timeout in ms until the connection must be established.
- Returns:
- True if the connection was successful, false otherwise
void physx::shdfnd::Socket::disconnect | ( | ) |
Disconnects an open socket
bool physx::shdfnd::Socket::flush | ( | ) |
Flushes the output stream. Until the stream is flushed, there is no guarantee that the written data has actually reached the destination storage. Flush forces all buffered data to be sent to the output.
- Note:
- flush always blocks. If the socket is in non-blocking mode, this will result the thread spinning.
- Returns:
- True if the flush was successful, false otherwise
const char * physx::shdfnd::Socket::getHost | ( | ) | const |
Returns the name of the connected host. This is the same as the string that was supplied to the connect call.
- Returns:
- The name of the connected host
uint16_t physx::shdfnd::Socket::getPort | ( | ) | const |
Returns the port of the connected host. This is the same as the port that was supplied to the connect call.
- Returns:
- The port of the connected host
bool physx::shdfnd::Socket::isBlocking | ( | ) | const |
Returns whether read/write/flush calls to the socket are blocking.
- Returns:
- True if the socket is blocking.
bool physx::shdfnd::Socket::isConnected | ( | ) | const |
Returns whether the socket is currently open (connected) or not.
- Returns:
- True if the socket is connected, false otherwise
bool physx::shdfnd::Socket::listen | ( | uint16_t | port | ) |
Opens a network socket for input and/or output as a server. Put the connection in listening mode
- Parameters:
-
port The port on which the socket listens
- Note:
- This method is not implemented on Windows Modern.
uint32_t physx::shdfnd::Socket::read | ( | uint8_t * | data, | |
uint32_t | length | |||
) |
Reads data from the output stream.
- Parameters:
-
data Pointer to a buffer where the read data will be stored. length Amount of data to read, in bytes.
- Returns:
- Number of bytes actually read. This could be lower than length if the stream end is encountered or the socket is non-blocking.
void physx::shdfnd::Socket::setBlocking | ( | bool | blocking | ) |
Sets blocking mode of the socket. Socket must be connected, otherwise calling this method won't take any effect.
uint32_t physx::shdfnd::Socket::write | ( | const uint8_t * | data, | |
uint32_t | length | |||
) |
Writes data to the output stream.
- Parameters:
-
data Pointer to a block of data to write to the stream length Amount of data to write, in bytes
- Returns:
- Number of bytes actually written. This could be lower than length if the socket is non-blocking.
Member Data Documentation
const uint32_t physx::shdfnd::Socket::DEFAULT_BUFFER_SIZE = 32768 [static] |
The documentation for this class was generated from the following files:
- E:/p4/sw/physx/PxShared/1.0/trunk/src/foundation/include/PsSocket.h
- E:/p4/sw/physx/PxShared/1.0/trunk/src/foundation/src/windows/PsWindowsSocket.cpp
Generated on Tue Jul 28 14:22:00 2015 for NVIDIA(R) PsFoundation Reference by
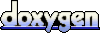