physx::shdfnd Namespace Reference
Namespaces | |
namespace | internal |
Classes | |
class | AlignedAllocator |
class | ScopedPointer |
class | RawAllocator |
class | NonTrackingAllocator |
class | ReflectionAllocator |
struct | AllocatorTraits |
union | EnableIfPod |
class | Array |
struct | Equal |
struct | Less |
struct | Greater |
class | Pair |
struct | LogTwo |
struct | LogTwo< 1 > |
struct | UnConst |
struct | UnConst< const T > |
class | Cpu |
class | FPUGuard |
class | SIMDGuard |
struct | Hash |
struct | Hash< const char * > |
class | HashMap |
class | CoalescedHashMap |
class | HashSet |
class | CoalescedHashSet |
class | InlineAllocator |
class | InlineArray |
class | MutexImpl |
class | MutexT |
class | ReadWriteLock |
class | ScopedReadLock |
class | ScopedWriteLock |
class | AtomicLock |
class | AtomicLockCopy |
class | AtomicRwLock |
class | ScopedAtomicLock |
class | PoolBase |
class | Pool |
class | Pool2 |
struct | SListImpl |
class | SListT |
class | Socket |
class | SyncImpl |
class | SyncT |
union | TempAllocatorChunk |
class | TempAllocator |
struct | ThreadPriority |
class | Runnable |
class | ThreadImpl |
class | ThreadT |
struct | CounterFrequencyToTensOfNanos |
class | Time |
class | UserAllocated |
class | ReadWriteLockImpl |
class | SocketImpl |
class | BufferedSocketImpl |
Typedefs | |
typedef int | IntBool |
typedef MutexT | Mutex |
typedef SListT | SList |
typedef SyncT | Sync |
typedef ThreadT | Thread |
typedef BOOL(WINAPI * | LPFN_GLPI )(PSYSTEM_LOGICAL_PROCESSOR_INFORMATION, PDWORD) |
Functions | |
void | initializeNamedAllocatorGlobals () |
void | terminateNamedAllocatorGlobals () |
template<class Serializer > | |
void | exportArray (Serializer &stream, const void *data, uint32_t size, uint32_t sizeOfElement, uint32_t capacity) |
char * | importArray (char *address, void **data, uint32_t size, uint32_t sizeOfElement, uint32_t capacity) |
template<class T , class Alloc > | |
PX_INLINE void | swap (Array< T, Alloc > &x, Array< T, Alloc > &y) |
PX_FOUNDATION_API int32_t | atomicExchange (volatile int32_t *dest, int32_t val) |
PX_FOUNDATION_API int32_t | atomicCompareExchange (volatile int32_t *dest, int32_t exch, int32_t comp) |
PX_FOUNDATION_API void * | atomicCompareExchangePointer (volatile void **dest, void *exch, void *comp) |
PX_FOUNDATION_API int32_t | atomicIncrement (volatile int32_t *val) |
PX_FOUNDATION_API int32_t | atomicDecrement (volatile int32_t *val) |
PX_FOUNDATION_API int32_t | atomicAdd (volatile int32_t *val, int32_t delta) |
PX_FOUNDATION_API int32_t | atomicMax (volatile int32_t *val, int32_t val2) |
template<typename T > | |
T | pointerOffset (void *p, ptrdiff_t offset) |
template<typename T > | |
T | pointerOffset (const void *p, ptrdiff_t offset) |
template<class T > | |
PX_CUDA_CALLABLE PX_INLINE void | swap (T &x, T &y) |
PX_INLINE uint32_t | bitCount (uint32_t v) |
PX_INLINE bool | isPowerOfTwo (uint32_t x) |
PX_INLINE uint32_t | nextPowerOfTwo (uint32_t x) |
PX_INLINE uint32_t | lowestSetBit (uint32_t x) |
PX_INLINE uint32_t | highestSetBit (uint32_t x) |
PX_INLINE uint32_t | ilog2 (uint32_t num) |
PX_FOUNDATION_API void | enableFPExceptions () |
Enables floating point exceptions for the scalar and SIMD unit. | |
PX_FOUNDATION_API void | disableFPExceptions () |
Disables floating point exceptions for the scalar and SIMD unit. | |
PX_FOUNDATION_API void | initializeSharedFoundation (uint32_t version, PxAllocatorCallback &, PxErrorCallback &) |
PX_FOUNDATION_API bool | sharedFoundationIsInitialized () |
PX_FOUNDATION_API void | terminateSharedFoundation () |
PX_FOUNDATION_API uint32_t | getInitializationCount () |
PX_FOUNDATION_API PxAllocatorCallback & | getAllocator () |
PX_FOUNDATION_API PxErrorCallback & | getErrorCallback () |
PX_FOUNDATION_API void | setReflectionAllocatorReportsNames (bool val) |
PX_FOUNDATION_API bool | getReflectionAllocatorReportsNames () |
PX_FOUNDATION_API PxProfilerCallback * | getProfilerCallback () |
PX_FOUNDATION_API void | setProfilerCallback (PxProfilerCallback *profiler) |
PX_FORCE_INLINE uint32_t | hash (const uint32_t key) |
PX_FORCE_INLINE uint32_t | hash (const int32_t key) |
PX_FORCE_INLINE uint32_t | hash (const uint64_t key) |
PX_INLINE uint32_t | hash (const void *ptr) |
template<typename F , typename S > | |
PX_INLINE uint32_t | hash (const Pair< F, S > &p) |
__declspec (align(8)) class SListEntry | |
template<class T , class Predicate , class Allocator > | |
void | sort (T *elements, uint32_t count, const Predicate &compare, const Allocator &inAllocator, const uint32_t initialStackSize=32) |
template<class T , class Predicate > | |
void | sort (T *elements, uint32_t count, const Predicate &compare) |
template<class T > | |
void | sort (T *elements, uint32_t count) |
PX_FOUNDATION_API int32_t | sscanf (const char *buffer, const char *format,...) |
PX_FOUNDATION_API int32_t | strcmp (const char *str1, const char *str2) |
PX_FOUNDATION_API int32_t | strncmp (const char *str1, const char *str2, size_t count) |
PX_FOUNDATION_API int32_t | snprintf (char *dst, size_t dstSize, const char *format,...) |
PX_FOUNDATION_API int32_t | vsnprintf (char *dst, size_t dstSize, const char *src, va_list arg) |
PX_FOUNDATION_API size_t | strlcat (char *dst, size_t dstSize, const char *src) |
PX_FOUNDATION_API size_t | strlcpy (char *dst, size_t dstSize, const char *src) |
PX_FOUNDATION_API int32_t | stricmp (const char *str1, const char *str2) |
PX_FOUNDATION_API int32_t | strnicmp (const char *str1, const char *str2, size_t count) |
PX_FOUNDATION_API void | strlwr (char *str) |
PX_FOUNDATION_API void | strupr (char *str) |
PX_FOUNDATION_API void | printFormatted (const char *,...) |
Prints the formatted data, trying to make sure it's visible to the app programmer. | |
PX_FOUNDATION_API void | printString (const char *) |
Prints the string literally (does not consume % specifier), trying to make sure it's visible to the app programmer. | |
void | initializeTempAllocatorGlobals () |
void | terminateTempAllocatorGlobals () |
PX_FOUNDATION_API uint32_t | TlsAlloc () |
PX_FOUNDATION_API void | TlsFree (uint32_t index) |
PX_FOUNDATION_API void * | TlsGet (uint32_t index) |
PX_FOUNDATION_API uint32_t | TlsSet (uint32_t index, void *value) |
PX_FORCE_INLINE void | memoryBarrier () |
PX_FORCE_INLINE uint32_t | highestSetBitUnsafe (uint32_t v) |
PX_FORCE_INLINE uint32_t | lowestSetBitUnsafe (uint32_t v) |
PX_FORCE_INLINE uint32_t | countLeadingZeros (uint32_t v) |
PX_FORCE_INLINE void | prefetchLine (const void *ptr, uint32_t offset=0) |
PX_FORCE_INLINE void | prefetch (const void *ptr, uint32_t count=1) |
PX_CUDA_CALLABLE PX_FORCE_INLINE float | recipFast (float a) |
platform-specific reciprocal | |
PX_CUDA_CALLABLE PX_FORCE_INLINE float | recipSqrtFast (float a) |
platform-specific fast reciprocal square root | |
PX_CUDA_CALLABLE PX_FORCE_INLINE float | floatFloor (float x) |
platform-specific floor | |
Variables | |
PxProfilerCallback * | gProfilerCallback = NULL |
PxAllocatorCallback * | gAllocatorCallback = NULL |
PxErrorCallback * | gErrorCallback = NULL |
uint32_t | gInitializationCount = 0 |
Typedef Documentation
typedef int physx::shdfnd::IntBool |
typedef BOOL(WINAPI* physx::shdfnd::LPFN_GLPI)(PSYSTEM_LOGICAL_PROCESSOR_INFORMATION, PDWORD) |
typedef MutexT physx::shdfnd::Mutex |
typedef SListT physx::shdfnd::SList |
typedef SyncT physx::shdfnd::Sync |
typedef ThreadT physx::shdfnd::Thread |
Function Documentation
physx::shdfnd::__declspec | ( | align(8) | ) |
int32_t physx::shdfnd::atomicAdd | ( | volatile int32_t * | val, | |
int32_t | delta | |||
) |
int32_t physx::shdfnd::atomicCompareExchange | ( | volatile int32_t * | dest, | |
int32_t | exch, | |||
int32_t | comp | |||
) |
void * physx::shdfnd::atomicCompareExchangePointer | ( | volatile void ** | dest, | |
void * | exch, | |||
void * | comp | |||
) |
int32_t physx::shdfnd::atomicDecrement | ( | volatile int32_t * | val | ) |
int32_t physx::shdfnd::atomicExchange | ( | volatile int32_t * | dest, | |
int32_t | val | |||
) |
int32_t physx::shdfnd::atomicIncrement | ( | volatile int32_t * | val | ) |
int32_t physx::shdfnd::atomicMax | ( | volatile int32_t * | val, | |
int32_t | val2 | |||
) |
PX_INLINE uint32_t physx::shdfnd::bitCount | ( | uint32_t | v | ) |
PX_FORCE_INLINE uint32_t physx::shdfnd::countLeadingZeros | ( | uint32_t | v | ) |
Returns the number of leading zeros in v. Returns 32 for v=0.
void physx::shdfnd::disableFPExceptions | ( | ) |
Disables floating point exceptions for the scalar and SIMD unit.
void physx::shdfnd::enableFPExceptions | ( | ) |
Enables floating point exceptions for the scalar and SIMD unit.
void physx::shdfnd::exportArray | ( | Serializer & | stream, | |
const void * | data, | |||
uint32_t | size, | |||
uint32_t | sizeOfElement, | |||
uint32_t | capacity | |||
) | [inline] |
PX_CUDA_CALLABLE PX_FORCE_INLINE float physx::shdfnd::floatFloor | ( | float | x | ) |
platform-specific floor
PxAllocatorCallback & physx::shdfnd::getAllocator | ( | ) |
PxErrorCallback & physx::shdfnd::getErrorCallback | ( | ) |
uint32_t physx::shdfnd::getInitializationCount | ( | ) |
PxProfilerCallback * physx::shdfnd::getProfilerCallback | ( | void | ) |
bool physx::shdfnd::getReflectionAllocatorReportsNames | ( | ) |
PX_INLINE uint32_t physx::shdfnd::hash | ( | const Pair< F, S > & | p | ) | [inline] |
PX_INLINE uint32_t physx::shdfnd::hash | ( | const void * | ptr | ) |
PX_FORCE_INLINE uint32_t physx::shdfnd::hash | ( | const uint64_t | key | ) |
PX_FORCE_INLINE uint32_t physx::shdfnd::hash | ( | const int32_t | key | ) |
PX_FORCE_INLINE uint32_t physx::shdfnd::hash | ( | const uint32_t | key | ) |
PX_INLINE uint32_t physx::shdfnd::highestSetBit | ( | uint32_t | x | ) |
Return the index of the highest set bit. Not valid for zero arg.
PX_FORCE_INLINE uint32_t physx::shdfnd::highestSetBitUnsafe | ( | uint32_t | v | ) |
Returns the index of the highest set bit. Not valid for zero arg.
PX_INLINE uint32_t physx::shdfnd::ilog2 | ( | uint32_t | num | ) |
char* physx::shdfnd::importArray | ( | char * | address, | |
void ** | data, | |||
uint32_t | size, | |||
uint32_t | sizeOfElement, | |||
uint32_t | capacity | |||
) |
void physx::shdfnd::initializeNamedAllocatorGlobals | ( | ) |
Allocator used to access the global PxAllocatorCallback instance using a dynamic name.
void physx::shdfnd::initializeSharedFoundation | ( | uint32_t | version, | |
PxAllocatorCallback & | allocator, | |||
PxErrorCallback & | err | |||
) |
void physx::shdfnd::initializeTempAllocatorGlobals | ( | ) |
PX_INLINE bool physx::shdfnd::isPowerOfTwo | ( | uint32_t | x | ) |
PX_INLINE uint32_t physx::shdfnd::lowestSetBit | ( | uint32_t | x | ) |
Return the index of the highest set bit. Not valid for zero arg.
PX_FORCE_INLINE uint32_t physx::shdfnd::lowestSetBitUnsafe | ( | uint32_t | v | ) |
Returns the index of the highest set bit. Undefined for zero arg.
PX_FORCE_INLINE void physx::shdfnd::memoryBarrier | ( | ) |
PX_INLINE uint32_t physx::shdfnd::nextPowerOfTwo | ( | uint32_t | x | ) |
T physx::shdfnd::pointerOffset | ( | const void * | p, | |
ptrdiff_t | offset | |||
) | [inline] |
T physx::shdfnd::pointerOffset | ( | void * | p, | |
ptrdiff_t | offset | |||
) | [inline] |
PX_FORCE_INLINE void physx::shdfnd::prefetch | ( | const void * | ptr, | |
uint32_t | count = 1 | |||
) |
Prefetch count
bytes starting at ptr
.
PX_FORCE_INLINE void physx::shdfnd::prefetchLine | ( | const void * | ptr, | |
uint32_t | offset = 0 | |||
) |
Prefetch aligned cache size around ptr+offset
.
void physx::shdfnd::printFormatted | ( | const char * | format, | |
... | ||||
) |
Prints the formatted data, trying to make sure it's visible to the app programmer.
- See also:
- NS_MAX_PRINTFORMATTED_LENGTH
void physx::shdfnd::printString | ( | const char * | str | ) |
Prints the string literally (does not consume % specifier), trying to make sure it's visible to the app programmer.
PX_CUDA_CALLABLE PX_FORCE_INLINE float physx::shdfnd::recipFast | ( | float | a | ) |
platform-specific reciprocal
PX_CUDA_CALLABLE PX_FORCE_INLINE float physx::shdfnd::recipSqrtFast | ( | float | a | ) |
platform-specific fast reciprocal square root
void physx::shdfnd::setProfilerCallback | ( | PxProfilerCallback * | profiler | ) |
void physx::shdfnd::setReflectionAllocatorReportsNames | ( | bool | val | ) |
bool physx::shdfnd::sharedFoundationIsInitialized | ( | ) |
int32_t physx::shdfnd::snprintf | ( | char * | dst, | |
size_t | dstSize, | |||
const char * | format, | |||
... | ||||
) |
void physx::shdfnd::sort | ( | T * | elements, | |
uint32_t | count, | |||
const Predicate & | compare, | |||
const Allocator & | inAllocator, | |||
const uint32_t | initialStackSize = 32 | |||
) | [inline] |
int32_t physx::shdfnd::sscanf | ( | const char * | buffer, | |
const char * | format, | |||
... | ||||
) |
int32_t physx::shdfnd::strcmp | ( | const char * | str1, | |
const char * | str2 | |||
) |
int32_t physx::shdfnd::stricmp | ( | const char * | str1, | |
const char * | str2 | |||
) |
size_t physx::shdfnd::strlcat | ( | char * | dst, | |
size_t | dstSize, | |||
const char * | src | |||
) |
size_t physx::shdfnd::strlcpy | ( | char * | dst, | |
size_t | dstSize, | |||
const char * | src | |||
) |
void physx::shdfnd::strlwr | ( | char * | str | ) |
int32_t physx::shdfnd::strncmp | ( | const char * | str1, | |
const char * | str2, | |||
size_t | count | |||
) |
int32_t physx::shdfnd::strnicmp | ( | const char * | str1, | |
const char * | str2, | |||
size_t | count | |||
) |
void physx::shdfnd::strupr | ( | char * | str | ) |
PX_CUDA_CALLABLE PX_INLINE void physx::shdfnd::swap | ( | T & | x, | |
T & | y | |||
) | [inline] |
PX_INLINE void physx::shdfnd::swap | ( | Array< T, Alloc > & | x, | |
Array< T, Alloc > & | y | |||
) | [inline] |
void physx::shdfnd::terminateNamedAllocatorGlobals | ( | ) |
void physx::shdfnd::terminateSharedFoundation | ( | ) |
void physx::shdfnd::terminateTempAllocatorGlobals | ( | ) |
uint32_t physx::shdfnd::TlsAlloc | ( | ) |
void physx::shdfnd::TlsFree | ( | uint32_t | index | ) |
void * physx::shdfnd::TlsGet | ( | uint32_t | index | ) |
uint32_t physx::shdfnd::TlsSet | ( | uint32_t | index, | |
void * | value | |||
) |
int32_t physx::shdfnd::vsnprintf | ( | char * | dst, | |
size_t | dstSize, | |||
const char * | src, | |||
va_list | arg | |||
) |
Variable Documentation
PxAllocatorCallback* physx::shdfnd::gAllocatorCallback = NULL |
PxErrorCallback* physx::shdfnd::gErrorCallback = NULL |
uint32_t physx::shdfnd::gInitializationCount = 0 |
PxProfilerCallback* physx::shdfnd::gProfilerCallback = NULL |
Generated on Tue Jul 28 14:21:56 2015 for NVIDIA(R) PsFoundation Reference by
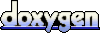