physx::shdfnd::Array< T, Alloc > Class Template Reference
#include <PsArray.h>
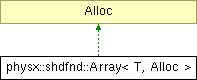
Public Types | |
typedef T * | Iterator |
typedef const T * | ConstIterator |
Public Member Functions | |
Array (const PxEMPTY v) | |
PX_INLINE | Array (const Alloc &alloc=Alloc()) |
PX_INLINE | Array (uint32_t size, const T &a=T(), const Alloc &alloc=Alloc()) |
template<class A > | |
PX_INLINE | Array (const Array< T, A > &other, const Alloc &alloc=Alloc()) |
PX_INLINE | Array (const Array &other, const Alloc &alloc=Alloc()) |
PX_INLINE | Array (const T *first, const T *last, const Alloc &alloc=Alloc()) |
PX_INLINE | ~Array () |
template<class A > | |
PX_INLINE Array & | operator= (const Array< T, A > &rhs) |
PX_INLINE Array & | operator= (const Array &t) |
PX_FORCE_INLINE const T & | operator[] (uint32_t i) const |
PX_FORCE_INLINE T & | operator[] (uint32_t i) |
PX_FORCE_INLINE ConstIterator | begin () const |
PX_FORCE_INLINE Iterator | begin () |
PX_FORCE_INLINE ConstIterator | end () const |
PX_FORCE_INLINE Iterator | end () |
PX_FORCE_INLINE const T & | front () const |
PX_FORCE_INLINE T & | front () |
PX_FORCE_INLINE const T & | back () const |
PX_FORCE_INLINE T & | back () |
PX_FORCE_INLINE uint32_t | size () const |
PX_INLINE void | clear () |
PX_FORCE_INLINE bool | empty () const |
PX_INLINE Iterator | find (const T &a) |
PX_INLINE ConstIterator | find (const T &a) const |
PX_FORCE_INLINE T & | pushBack (const T &a) |
PX_INLINE T | popBack () |
PX_INLINE T & | insert () |
PX_INLINE void | replaceWithLast (uint32_t i) |
PX_INLINE void | replaceWithLast (Iterator i) |
PX_INLINE bool | findAndReplaceWithLast (const T &a) |
PX_INLINE void | remove (uint32_t i) |
PX_INLINE void | removeRange (uint32_t begin, uint32_t count) |
PX_NOINLINE void | resize (const uint32_t size, const T &a=T()) |
PX_NOINLINE void | resizeUninitialized (const uint32_t size) |
PX_INLINE void | shrink () |
PX_INLINE void | reset () |
PX_INLINE void | reserve (const uint32_t capacity) |
PX_FORCE_INLINE uint32_t | capacity () const |
PX_FORCE_INLINE void | forceSize_Unsafe (uint32_t size) |
PX_INLINE void | swap (Array< T, Alloc > &other) |
PX_INLINE void | assign (const T *first, const T *last) |
PX_FORCE_INLINE uint32_t | isInUserMemory () const |
PX_INLINE Alloc & | getAllocator () |
return reference to allocator | |
Static Public Member Functions | |
static PX_FORCE_INLINE bool | isArrayOfPOD () |
Protected Member Functions | |
Array (T *memory, uint32_t size, uint32_t capacity, const Alloc &alloc=Alloc()) | |
template<class A > | |
PX_NOINLINE void | copy (const Array< T, A > &other) |
PX_INLINE T * | allocate (uint32_t size) |
PX_INLINE void | deallocate (void *mem) |
PX_NOINLINE T & | growAndPushBack (const T &a) |
PX_INLINE void | grow (uint32_t capacity) |
PX_NOINLINE void | recreate (uint32_t capacity) |
PX_INLINE uint32_t | capacityIncrement () const |
Static Protected Member Functions | |
static PX_INLINE bool | isZeroInit (const T &object) |
static PX_INLINE void | create (T *first, T *last, const T &a) |
static PX_INLINE void | copy (T *first, T *last, const T *src) |
static PX_INLINE void | destroy (T *first, T *last) |
Protected Attributes | |
T * | mData |
uint32_t | mSize |
uint32_t | mCapacity |
Detailed Description
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
class physx::shdfnd::Array< T, Alloc >
An array is a sequential container.Implementation note entries between 0 and size are valid objects we use inheritance to build this because the array is included inline in a lot of objects and we want the allocator to take no space if it's not stateful, which aggregation doesn't allow. Also, we want the metadata at the front for the inline case where the allocator contains some inline storage space
Member Typedef Documentation
typedef const T* physx::shdfnd::Array< T, Alloc >::ConstIterator |
typedef T* physx::shdfnd::Array< T, Alloc >::Iterator |
Constructor & Destructor Documentation
physx::shdfnd::Array< T, Alloc >::Array | ( | const PxEMPTY | v | ) | [inline, explicit] |
PX_INLINE physx::shdfnd::Array< T, Alloc >::Array | ( | const Alloc & | alloc = Alloc() |
) | [inline, explicit] |
Default array constructor. Initialize an empty array
PX_INLINE physx::shdfnd::Array< T, Alloc >::Array | ( | uint32_t | size, | |
const T & | a = T() , |
|||
const Alloc & | alloc = Alloc() | |||
) | [inline, explicit] |
Initialize array with given capacity
PX_INLINE physx::shdfnd::Array< T, Alloc >::Array | ( | const Array< T, A > & | other, | |
const Alloc & | alloc = Alloc() | |||
) | [inline, explicit] |
Copy-constructor. Copy all entries from other array
PX_INLINE physx::shdfnd::Array< T, Alloc >::Array | ( | const Array< T, Alloc > & | other, | |
const Alloc & | alloc = Alloc() | |||
) | [inline] |
PX_INLINE physx::shdfnd::Array< T, Alloc >::Array | ( | const T * | first, | |
const T * | last, | |||
const Alloc & | alloc = Alloc() | |||
) | [inline, explicit] |
Initialize array with given length
PX_INLINE physx::shdfnd::Array< T, Alloc >::~Array | ( | ) | [inline] |
Destructor
physx::shdfnd::Array< T, Alloc >::Array | ( | T * | memory, | |
uint32_t | size, | |||
uint32_t | capacity, | |||
const Alloc & | alloc = Alloc() | |||
) | [inline, protected] |
Member Function Documentation
PX_INLINE T* physx::shdfnd::Array< T, Alloc >::allocate | ( | uint32_t | size | ) | [inline, protected] |
Mark a specified amount of memory with 0xcd pattern. This is used to check that the meta data definition for serialized classes is complete in checked builds.
PX_INLINE void physx::shdfnd::Array< T, Alloc >::assign | ( | const T * | first, | |
const T * | last | |||
) | [inline] |
Assign a range of values to this vector (resizes to length of range)
PX_FORCE_INLINE T& physx::shdfnd::Array< T, Alloc >::back | ( | ) | [inline] |
PX_FORCE_INLINE const T& physx::shdfnd::Array< T, Alloc >::back | ( | ) | const [inline] |
Returns a reference to the last element of the array. Undefined if the array is empty
- Returns:
- a reference to the last element of the array
PX_FORCE_INLINE Iterator physx::shdfnd::Array< T, Alloc >::begin | ( | ) | [inline] |
PX_FORCE_INLINE ConstIterator physx::shdfnd::Array< T, Alloc >::begin | ( | ) | const [inline] |
Returns a pointer to the initial element of the array.
- Returns:
- a pointer to the initial element of the array.
PX_FORCE_INLINE uint32_t physx::shdfnd::Array< T, Alloc >::capacity | ( | ) | const [inline] |
Query the capacity(allocated mem) for the array.
PX_INLINE uint32_t physx::shdfnd::Array< T, Alloc >::capacityIncrement | ( | ) | const [inline, protected] |
PX_INLINE void physx::shdfnd::Array< T, Alloc >::clear | ( | ) | [inline] |
Clears the array.
static PX_INLINE void physx::shdfnd::Array< T, Alloc >::copy | ( | T * | first, | |
T * | last, | |||
const T * | src | |||
) | [inline, static, protected] |
PX_NOINLINE void physx::shdfnd::Array< T, Alloc >::copy | ( | const Array< T, A > & | other | ) | [inline, protected] |
static PX_INLINE void physx::shdfnd::Array< T, Alloc >::create | ( | T * | first, | |
T * | last, | |||
const T & | a | |||
) | [inline, static, protected] |
PX_INLINE void physx::shdfnd::Array< T, Alloc >::deallocate | ( | void * | mem | ) | [inline, protected] |
static PX_INLINE void physx::shdfnd::Array< T, Alloc >::destroy | ( | T * | first, | |
T * | last | |||
) | [inline, static, protected] |
PX_FORCE_INLINE bool physx::shdfnd::Array< T, Alloc >::empty | ( | ) | const [inline] |
Returns whether the array is empty (i.e. whether its size is 0).
- Returns:
- true if the array is empty
PX_FORCE_INLINE Iterator physx::shdfnd::Array< T, Alloc >::end | ( | ) | [inline] |
PX_FORCE_INLINE ConstIterator physx::shdfnd::Array< T, Alloc >::end | ( | ) | const [inline] |
Returns an iterator beyond the last element of the array. Do not dereference.
- Returns:
- a pointer to the element beyond the last element of the array.
PX_INLINE ConstIterator physx::shdfnd::Array< T, Alloc >::find | ( | const T & | a | ) | const [inline] |
PX_INLINE Iterator physx::shdfnd::Array< T, Alloc >::find | ( | const T & | a | ) | [inline] |
Finds the first occurrence of an element in the array.
- Parameters:
-
a The element to find.
PX_INLINE bool physx::shdfnd::Array< T, Alloc >::findAndReplaceWithLast | ( | const T & | a | ) | [inline] |
Replaces the first occurrence of the element a with the last element Operation is O(n)
- Parameters:
-
a The position of the element that will be subtracted from this array.
- Returns:
- true if the element has been removed.
PX_FORCE_INLINE void physx::shdfnd::Array< T, Alloc >::forceSize_Unsafe | ( | uint32_t | size | ) | [inline] |
Unsafe function to force the size of the array
PX_FORCE_INLINE T& physx::shdfnd::Array< T, Alloc >::front | ( | ) | [inline] |
PX_FORCE_INLINE const T& physx::shdfnd::Array< T, Alloc >::front | ( | ) | const [inline] |
Returns a reference to the first element of the array. Undefined if the array is empty.
- Returns:
- a reference to the first element of the array
PX_INLINE Alloc& physx::shdfnd::Array< T, Alloc >::getAllocator | ( | ) | [inline] |
return reference to allocator
PX_INLINE void physx::shdfnd::Array< T, Alloc >::grow | ( | uint32_t | capacity | ) | [inline, protected] |
Resizes the available memory for the array.
- Parameters:
-
capacity The number of entries that the set should be able to hold.
PX_NOINLINE T & physx::shdfnd::Array< T, Alloc >::growAndPushBack | ( | const T & | a | ) | [inline, protected] |
Called when pushBack() needs to grow the array.
- Parameters:
-
a The element that will be added to this array.
PX_INLINE T& physx::shdfnd::Array< T, Alloc >::insert | ( | ) | [inline] |
Construct one element at the end of the array. Operation is O(1).
static PX_FORCE_INLINE bool physx::shdfnd::Array< T, Alloc >::isArrayOfPOD | ( | ) | [inline, static] |
PX_FORCE_INLINE uint32_t physx::shdfnd::Array< T, Alloc >::isInUserMemory | ( | ) | const [inline] |
static PX_INLINE bool physx::shdfnd::Array< T, Alloc >::isZeroInit | ( | const T & | object | ) | [inline, static, protected] |
PX_INLINE Array& physx::shdfnd::Array< T, Alloc >::operator= | ( | const Array< T, Alloc > & | t | ) | [inline] |
PX_INLINE Array& physx::shdfnd::Array< T, Alloc >::operator= | ( | const Array< T, A > & | rhs | ) | [inline] |
Assignment operator. Copy content (deep-copy)
PX_FORCE_INLINE T& physx::shdfnd::Array< T, Alloc >::operator[] | ( | uint32_t | i | ) | [inline] |
Array indexing operator.
- Parameters:
-
i The index of the element that will be returned.
- Returns:
- The element i in the array.
PX_FORCE_INLINE const T& physx::shdfnd::Array< T, Alloc >::operator[] | ( | uint32_t | i | ) | const [inline] |
Array indexing operator.
- Parameters:
-
i The index of the element that will be returned.
- Returns:
- The element i in the array.
PX_INLINE T physx::shdfnd::Array< T, Alloc >::popBack | ( | ) | [inline] |
Returns the element at the end of the array. Only legal if the array is non-empty.
PX_FORCE_INLINE T& physx::shdfnd::Array< T, Alloc >::pushBack | ( | const T & | a | ) | [inline] |
Adds one element to the end of the array. Operation is O(1).
- Parameters:
-
a The element that will be added to this array.
PX_NOINLINE void physx::shdfnd::Array< T, Alloc >::recreate | ( | uint32_t | capacity | ) | [inline, protected] |
Creates a new memory block, copies all entries to the new block and destroys old entries.
- Parameters:
-
capacity The number of entries that the set should be able to hold.
PX_INLINE void physx::shdfnd::Array< T, Alloc >::remove | ( | uint32_t | i | ) | [inline] |
Subtracts the element on position i from the array. Shift the entire array one step. Operation is O(n)
- Parameters:
-
i The position of the element that will be subtracted from this array.
PX_INLINE void physx::shdfnd::Array< T, Alloc >::removeRange | ( | uint32_t | begin, | |
uint32_t | count | |||
) | [inline] |
Removes a range from the array. Shifts the array so order is maintained. Operation is O(n)
- Parameters:
-
begin The starting position of the element that will be subtracted from this array. count The number of elments that will be subtracted from this array.
PX_INLINE void physx::shdfnd::Array< T, Alloc >::replaceWithLast | ( | Iterator | i | ) | [inline] |
PX_INLINE void physx::shdfnd::Array< T, Alloc >::replaceWithLast | ( | uint32_t | i | ) | [inline] |
Subtracts the element on position i from the array and replace it with the last element. Operation is O(1)
- Parameters:
-
i The position of the element that will be subtracted from this array.
PX_INLINE void physx::shdfnd::Array< T, Alloc >::reserve | ( | const uint32_t | capacity | ) | [inline] |
Ensure that the array has at least size capacity.
PX_INLINE void physx::shdfnd::Array< T, Alloc >::reset | ( | ) | [inline] |
Deletes all array elements and frees memory.
PX_NOINLINE void physx::shdfnd::Array< T, Alloc >::resize | ( | const uint32_t | size, | |
const T & | a = T() | |||
) | [inline] |
Resize array
PX_NOINLINE void physx::shdfnd::Array< T, Alloc >::resizeUninitialized | ( | const uint32_t | size | ) | [inline] |
PX_INLINE void physx::shdfnd::Array< T, Alloc >::shrink | ( | ) | [inline] |
Resize array such that only as much memory is allocated to hold the existing elements
PX_FORCE_INLINE uint32_t physx::shdfnd::Array< T, Alloc >::size | ( | ) | const [inline] |
Returns the number of entries in the array. This can, and probably will, differ from the array capacity.
- Returns:
- The number of of entries in the array.
PX_INLINE void physx::shdfnd::Array< T, Alloc >::swap | ( | Array< T, Alloc > & | other | ) | [inline] |
Swap contents of an array without allocating temporary storage
Member Data Documentation
uint32_t physx::shdfnd::Array< T, Alloc >::mCapacity [protected] |
T* physx::shdfnd::Array< T, Alloc >::mData [protected] |
uint32_t physx::shdfnd::Array< T, Alloc >::mSize [protected] |
The documentation for this class was generated from the following file:
- E:/p4/sw/physx/PxShared/1.0/trunk/src/foundation/include/PsArray.h
Generated on Tue Jul 28 14:21:57 2015 for NVIDIA(R) PsFoundation Reference by
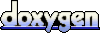