physx::shdfnd::PoolBase< T, Alloc > Class Template Reference
#include <PsPool.h>
Inheritance diagram for physx::shdfnd::PoolBase< T, Alloc >:

Classes | |
struct | FreeList |
Public Member Functions | |
~PoolBase () | |
PX_INLINE T * | allocate () |
PX_INLINE void | deallocate (T *p) |
PX_INLINE T * | construct () |
template<class A1 > | |
PX_INLINE T * | construct (A1 &a) |
template<class A1 , class A2 > | |
PX_INLINE T * | construct (A1 &a, A2 &b) |
template<class A1 , class A2 , class A3 > | |
PX_INLINE T * | construct (A1 &a, A2 &b, A3 &c) |
template<class A1 , class A2 , class A3 > | |
PX_INLINE T * | construct (A1 *a, A2 &b, A3 &c) |
template<class A1 , class A2 , class A3 , class A4 > | |
PX_INLINE T * | construct (A1 &a, A2 &b, A3 &c, A4 &d) |
template<class A1 , class A2 , class A3 , class A4 , class A5 > | |
PX_INLINE T * | construct (A1 &a, A2 &b, A3 &c, A4 &d, A5 &e) |
PX_INLINE void | destroy (T *const p) |
Protected Member Functions | |
PoolBase (const Alloc &alloc, uint32_t elementsPerSlab, uint32_t slabSize) | |
void | push (FreeList *p) |
void | allocateSlab () |
void | disposeElements () |
void | releaseEmptySlabs () |
Protected Attributes | |
InlineArray< void *, 64, Alloc > | mSlabs |
uint32_t | mElementsPerSlab |
uint32_t | mUsed |
uint32_t | mSlabSize |
FreeList * | mFreeElement |
Detailed Description
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
class physx::shdfnd::PoolBase< T, Alloc >
Simple allocation pool Constructor & Destructor Documentation
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
physx::shdfnd::PoolBase< T, Alloc >::PoolBase | ( | const Alloc & | alloc, | |
uint32_t | elementsPerSlab, | |||
uint32_t | slabSize | |||
) | [inline, protected] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
physx::shdfnd::PoolBase< T, Alloc >::~PoolBase | ( | ) | [inline] |
Member Function Documentation
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
PX_INLINE T* physx::shdfnd::PoolBase< T, Alloc >::allocate | ( | ) | [inline] |
Mark a specified amount of memory with 0xcd pattern. This is used to check that the meta data definition for serialized classes is complete in checked builds.
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
void physx::shdfnd::PoolBase< T, Alloc >::allocateSlab | ( | ) | [inline, protected] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
template<class A1 , class A2 , class A3 , class A4 , class A5 >
PX_INLINE T* physx::shdfnd::PoolBase< T, Alloc >::construct | ( | A1 & | a, | |
A2 & | b, | |||
A3 & | c, | |||
A4 & | d, | |||
A5 & | e | |||
) | [inline] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
template<class A1 , class A2 , class A3 , class A4 >
PX_INLINE T* physx::shdfnd::PoolBase< T, Alloc >::construct | ( | A1 & | a, | |
A2 & | b, | |||
A3 & | c, | |||
A4 & | d | |||
) | [inline] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
template<class A1 , class A2 , class A3 >
PX_INLINE T* physx::shdfnd::PoolBase< T, Alloc >::construct | ( | A1 * | a, | |
A2 & | b, | |||
A3 & | c | |||
) | [inline] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
template<class A1 , class A2 , class A3 >
PX_INLINE T* physx::shdfnd::PoolBase< T, Alloc >::construct | ( | A1 & | a, | |
A2 & | b, | |||
A3 & | c | |||
) | [inline] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
template<class A1 , class A2 >
PX_INLINE T* physx::shdfnd::PoolBase< T, Alloc >::construct | ( | A1 & | a, | |
A2 & | b | |||
) | [inline] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
template<class A1 >
PX_INLINE T* physx::shdfnd::PoolBase< T, Alloc >::construct | ( | A1 & | a | ) | [inline] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
PX_INLINE T* physx::shdfnd::PoolBase< T, Alloc >::construct | ( | ) | [inline] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
PX_INLINE void physx::shdfnd::PoolBase< T, Alloc >::deallocate | ( | T * | p | ) | [inline] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
PX_INLINE void physx::shdfnd::PoolBase< T, Alloc >::destroy | ( | T *const | p | ) | [inline] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
void physx::shdfnd::PoolBase< T, Alloc >::disposeElements | ( | ) | [inline, protected] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
void physx::shdfnd::PoolBase< T, Alloc >::push | ( | FreeList * | p | ) | [inline, protected] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
void physx::shdfnd::PoolBase< T, Alloc >::releaseEmptySlabs | ( | ) | [inline, protected] |
Member Data Documentation
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
uint32_t physx::shdfnd::PoolBase< T, Alloc >::mElementsPerSlab [protected] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
FreeList* physx::shdfnd::PoolBase< T, Alloc >::mFreeElement [protected] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
InlineArray<void*, 64, Alloc> physx::shdfnd::PoolBase< T, Alloc >::mSlabs [protected] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
uint32_t physx::shdfnd::PoolBase< T, Alloc >::mSlabSize [protected] |
template<class T, class Alloc = typename AllocatorTraits<T>::Type>
uint32_t physx::shdfnd::PoolBase< T, Alloc >::mUsed [protected] |
The documentation for this class was generated from the following file:
- E:/p4/sw/physx/PxShared/1.0/trunk/src/foundation/include/PsPool.h
Generated on Tue Jul 28 14:21:59 2015 for NVIDIA(R) PsFoundation Reference by
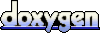