PxMat44 Class Reference
[Foundation]
4x4 matrix class
More...
#include <PxMat44.h>
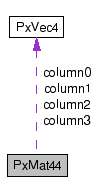
Public Member Functions | |
PX_CUDA_CALLABLE PX_INLINE | PxMat44 () |
Default constructor. | |
PX_CUDA_CALLABLE PX_INLINE | PxMat44 (PxIDENTITY r) |
identity constructor | |
PX_CUDA_CALLABLE PX_INLINE | PxMat44 (PxZERO r) |
zero constructor | |
PX_CUDA_CALLABLE | PxMat44 (const PxVec4 &col0, const PxVec4 &col1, const PxVec4 &col2, const PxVec4 &col3) |
Construct from four 4-vectors. | |
PX_CUDA_CALLABLE PX_INLINE | PxMat44 (float r) |
constructor that generates a multiple of the identity matrix | |
PX_CUDA_CALLABLE | PxMat44 (const PxVec3 &col0, const PxVec3 &col1, const PxVec3 &col2, const PxVec3 &col3) |
Construct from three base vectors and a translation. | |
PX_CUDA_CALLABLE PX_INLINE | PxMat44 (float values[]) |
Construct from float[16]. | |
PX_CUDA_CALLABLE PX_INLINE | PxMat44 (const PxQuat &q) |
Construct from a quaternion. | |
PX_CUDA_CALLABLE PX_INLINE | PxMat44 (const PxVec4 &diagonal) |
Construct from a diagonal vector. | |
PX_CUDA_CALLABLE | PxMat44 (const PxMat33 &axes, const PxVec3 &position) |
Construct from Mat33 and a translation. | |
PX_CUDA_CALLABLE | PxMat44 (const PxTransform &t) |
PX_CUDA_CALLABLE PX_INLINE bool | operator== (const PxMat44 &m) const |
returns true if the two matrices are exactly equal | |
PX_CUDA_CALLABLE PX_INLINE | PxMat44 (const PxMat44 &other) |
Copy constructor. | |
PX_CUDA_CALLABLE PX_INLINE PxMat44 & | operator= (const PxMat44 &other) |
Assignment operator. | |
PX_CUDA_CALLABLE PX_INLINE const PxMat44 | getTranspose () const |
Get transposed matrix. | |
PX_CUDA_CALLABLE PX_INLINE const PxMat44 | operator- () const |
Unary minus. | |
PX_CUDA_CALLABLE PX_INLINE const PxMat44 | operator+ (const PxMat44 &other) const |
Add. | |
PX_CUDA_CALLABLE PX_INLINE const PxMat44 | operator- (const PxMat44 &other) const |
Subtract. | |
PX_CUDA_CALLABLE PX_INLINE const PxMat44 | operator* (float scalar) const |
Scalar multiplication. | |
PX_CUDA_CALLABLE PX_INLINE const PxMat44 | operator* (const PxMat44 &other) const |
Matrix multiplication. | |
PX_CUDA_CALLABLE PX_INLINE PxMat44 & | operator+= (const PxMat44 &other) |
Equals-add. | |
PX_CUDA_CALLABLE PX_INLINE PxMat44 & | operator-= (const PxMat44 &other) |
Equals-sub. | |
PX_CUDA_CALLABLE PX_INLINE PxMat44 & | operator*= (float scalar) |
Equals scalar multiplication. | |
PX_CUDA_CALLABLE PX_INLINE PxMat44 & | operator*= (const PxMat44 &other) |
Equals matrix multiplication. | |
PX_DEPRECATED PX_CUDA_CALLABLE PX_FORCE_INLINE float | operator() (unsigned int row, unsigned int col) const |
Element access, mathematical way! | |
PX_DEPRECATED PX_CUDA_CALLABLE PX_FORCE_INLINE float & | operator() (unsigned int row, unsigned int col) |
Element access, mathematical way! | |
PX_CUDA_CALLABLE PX_INLINE const PxVec4 | transform (const PxVec4 &other) const |
Transform vector by matrix, equal to v' = M*v. | |
PX_CUDA_CALLABLE PX_INLINE const PxVec3 | transform (const PxVec3 &other) const |
Transform vector by matrix, equal to v' = M*v. | |
PX_CUDA_CALLABLE PX_INLINE const PxVec4 | rotate (const PxVec4 &other) const |
Rotate vector by matrix, equal to v' = M*v. | |
PX_CUDA_CALLABLE PX_INLINE const PxVec3 | rotate (const PxVec3 &other) const |
Rotate vector by matrix, equal to v' = M*v. | |
PX_CUDA_CALLABLE PX_INLINE const PxVec3 | getBasis (int num) const |
PX_CUDA_CALLABLE PX_INLINE const PxVec3 | getPosition () const |
PX_CUDA_CALLABLE PX_INLINE void | setPosition (const PxVec3 &position) |
PX_CUDA_CALLABLE PX_FORCE_INLINE const float * | front () const |
PX_DEPRECATED PX_CUDA_CALLABLE PX_FORCE_INLINE PxVec4 & | operator[] (unsigned int num) |
PX_DEPRECATED PX_CUDA_CALLABLE PX_FORCE_INLINE const PxVec4 & | operator[] (unsigned int num) const |
PX_CUDA_CALLABLE PX_INLINE void | scale (const PxVec4 &p) |
PX_CUDA_CALLABLE PX_INLINE const PxMat44 | inverseRT (void) const |
PX_CUDA_CALLABLE PX_INLINE bool | isFinite () const |
Public Attributes | |
PxVec4 | column0 |
PxVec4 | column1 |
PxVec4 | column2 |
PxVec4 | column3 |
Friends | |
PxMat44 | operator* (float, const PxMat44 &) |
Detailed Description
4x4 matrix classThis class is layout-compatible with D3D and OpenGL matrices. More notes on layout are given in the PxMat33
- See also:
- PxMat33 PxTransform
Constructor & Destructor Documentation
PX_CUDA_CALLABLE PX_INLINE PxMat44::PxMat44 | ( | ) | [inline] |
Default constructor.
PX_CUDA_CALLABLE PX_INLINE PxMat44::PxMat44 | ( | PxIDENTITY | r | ) | [inline] |
PX_CUDA_CALLABLE PX_INLINE PxMat44::PxMat44 | ( | PxZERO | r | ) | [inline] |
PX_CUDA_CALLABLE PxMat44::PxMat44 | ( | const PxVec4 & | col0, | |
const PxVec4 & | col1, | |||
const PxVec4 & | col2, | |||
const PxVec4 & | col3 | |||
) | [inline] |
Construct from four 4-vectors.
PX_CUDA_CALLABLE PX_INLINE PxMat44::PxMat44 | ( | float | r | ) | [inline, explicit] |
constructor that generates a multiple of the identity matrix
PX_CUDA_CALLABLE PxMat44::PxMat44 | ( | const PxVec3 & | col0, | |
const PxVec3 & | col1, | |||
const PxVec3 & | col2, | |||
const PxVec3 & | col3 | |||
) | [inline] |
Construct from three base vectors and a translation.
PX_CUDA_CALLABLE PX_INLINE PxMat44::PxMat44 | ( | float | values[] | ) | [inline, explicit] |
Construct from float[16].
PX_CUDA_CALLABLE PX_INLINE PxMat44::PxMat44 | ( | const PxQuat & | q | ) | [inline, explicit] |
PX_CUDA_CALLABLE PX_INLINE PxMat44::PxMat44 | ( | const PxVec4 & | diagonal | ) | [inline, explicit] |
Construct from a diagonal vector.
Construct from Mat33 and a translation.
PX_CUDA_CALLABLE PxMat44::PxMat44 | ( | const PxTransform & | t | ) | [inline] |
References PxTransform::p, and PxTransform::q.
PX_CUDA_CALLABLE PX_INLINE PxMat44::PxMat44 | ( | const PxMat44 & | other | ) | [inline] |
Copy constructor.
Member Function Documentation
PX_CUDA_CALLABLE PX_FORCE_INLINE const float* PxMat44::front | ( | ) | const [inline] |
PX_CUDA_CALLABLE PX_INLINE const PxVec3 PxMat44::getBasis | ( | int | num | ) | const [inline] |
References PX_ASSERT.
PX_CUDA_CALLABLE PX_INLINE const PxVec3 PxMat44::getPosition | ( | ) | const [inline] |
PX_CUDA_CALLABLE PX_INLINE const PxMat44 PxMat44::getTranspose | ( | ) | const [inline] |
Get transposed matrix.
PX_CUDA_CALLABLE PX_INLINE const PxMat44 PxMat44::inverseRT | ( | void | ) | const [inline] |
References PxVec3::z.
PX_CUDA_CALLABLE PX_INLINE bool PxMat44::isFinite | ( | ) | const [inline] |
PX_DEPRECATED PX_CUDA_CALLABLE PX_FORCE_INLINE float& PxMat44::operator() | ( | unsigned int | row, | |
unsigned int | col | |||
) | [inline] |
Element access, mathematical way!
PX_DEPRECATED PX_CUDA_CALLABLE PX_FORCE_INLINE float PxMat44::operator() | ( | unsigned int | row, | |
unsigned int | col | |||
) | const [inline] |
Element access, mathematical way!
PX_CUDA_CALLABLE PX_INLINE const PxMat44 PxMat44::operator* | ( | float | scalar | ) | const [inline] |
Scalar multiplication.
Equals matrix multiplication.
PX_CUDA_CALLABLE PX_INLINE PxMat44& PxMat44::operator*= | ( | float | scalar | ) | [inline] |
Equals scalar multiplication.
PX_CUDA_CALLABLE PX_INLINE const PxMat44 PxMat44::operator- | ( | ) | const [inline] |
Unary minus.
PX_CUDA_CALLABLE PX_INLINE bool PxMat44::operator== | ( | const PxMat44 & | m | ) | const [inline] |
PX_DEPRECATED PX_CUDA_CALLABLE PX_FORCE_INLINE const PxVec4& PxMat44::operator[] | ( | unsigned int | num | ) | const [inline] |
PX_DEPRECATED PX_CUDA_CALLABLE PX_FORCE_INLINE PxVec4& PxMat44::operator[] | ( | unsigned int | num | ) | [inline] |
Rotate vector by matrix, equal to v' = M*v.
PX_CUDA_CALLABLE PX_INLINE void PxMat44::scale | ( | const PxVec4 & | p | ) | [inline] |
PX_CUDA_CALLABLE PX_INLINE void PxMat44::setPosition | ( | const PxVec3 & | position | ) | [inline] |
Transform vector by matrix, equal to v' = M*v.
Friends And Related Function Documentation
Member Data Documentation
Referenced by operator*(), operator+(), operator+=(), operator-(), operator-=(), operator=(), operator==(), and PxTransform::PxTransform().
Referenced by operator*(), operator+(), operator+=(), operator-(), operator-=(), operator=(), operator==(), and PxTransform::PxTransform().
Referenced by operator*(), operator+(), operator+=(), operator-(), operator-=(), operator=(), operator==(), and PxTransform::PxTransform().
Referenced by operator*(), operator+(), operator+=(), operator-(), operator-=(), operator=(), operator==(), and PxTransform::PxTransform().
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com