Message
The Message function shows a message.
int WINAPI Message( int PluginNumber, DWORD Flags, const char *HelpTopic, const char * const *Items, int ItemsNumber, int ButtonsNumber );
Parameters
PluginNumber
Number of the plugin module. It is passed to the plugin in the
SetStartupInfo function.
Flags
Can be a combination of the following values (FARMESSAGEFLAGS enum):
Flag | Description |
---|---|
FMSG_WARNING | Warning message colors are used (white text on red background by default). |
FMSG_ERRORTYPE | If error type returned by
GetLastError
|
FMSG_KEEPBACKGROUND | Do not redraw the message background. |
FMSG_DOWN | Display the message two lines lower than usual. |
FMSG_LEFTALIGN | Left align the message lines instead of centering them. |
FMSG_ALLINONE |
In this case the Items parameter is not an array of string pointers.
Instead it points to a single string in which the lines of the
message are separated by the newline character '\n' .
Minimal number of lines is - 2 - a title and one message line. If this flag is specified the ItemsNumber parameter is ignored and the number of lines shown is calculated automatically (taking into account the button flags -FMSG_MB_*). To suppress title output when this flag is specified, start the line with a
|
FMSG_MB_OK | Additional button: <Ok> |
FMSG_MB_OKCANCEL | Additional buttons: <Ok> and <Cancel> |
FMSG_MB_ABORTRETRYIGNORE | Additional buttons: <Abort>, <Retry> and <Ignore> |
FMSG_MB_YESNO | Additional buttons: <Yes> and <No> |
FMSG_MB_YESNOCANCEL | Additional buttons: <Yes>, <No> and <Cancel> |
FMSG_MB_RETRYCANCEL | Additional buttons: <Retry> and <Cancel> |
HelpTopic
The help topic
associated with the message.Set to
NULL
if help is not used.
Items
Address of an array of pointers to null-terminated text strings. The first
string is the message title, the last ButtonsNumber strings are buttons, and all other strings
belong to the message body.
To draw a single border line start the string with a character with code 1 (\x001).
To draw a double border line start the string with a character with code 2 (\x002).
See also the description of the flag FMSG_ALLINONE
To draw a single border line start the string with a character with code 1 (\x001).
To draw a double border line start the string with a character with code 2 (\x002).
See also the description of the flag FMSG_ALLINONE
ItemsNumber
Number of strings in the array passed in the Items parameter.
Minimal values - 2 lines.
ButtonsNumber
Number of strings which are shown as buttons. If one of the FMSG_MB_* flags
is set, this value is ignored.
Return value
This function returns either -1, if the user cancelled the message (or the sysrem could not
allocate enough memory for internal buffers), or the number of the selected button (for the first
button 0 is returned, for the second 1 is returned, and so on).
Remarks
- In FAR Manager versions up to (and including) 1.70 beta 4 the maximum number of items in a message (including the buttons) was limited to 13.
- If ButtonsNumber is zero and none of the FMSG_MB_* flags is set the plugin should restore the screen either by using RestoreScreen or in any other way when the message output is no longer necessary
- If ButtonsNumber is not equal to zero, the screen will be restored by FAR.
- If Items is
NULL
or the total number of items is less than 2, the message is not shown. - When FMSG_MB_* button flags are specified the ButtonsNumber parameter is ignored.
- It is possible to specify hotkeys for buttons.
- When using the FMSG_ALLINONE flag you need to do an explicit typecast to achieve error free
compilation:
Info.Message(Info.ModuleNumber, FMSG_ALLINONE|FMSG_MB_OKCANCEL, "HelpTopic", (const char * const *)"Title\nItem1\nItem2\nItem3", 0,0);
orconst char *Msg="Title\nItem1\nItem2\nItem3\nOk\nCancel"; Info.Message(Info.ModuleNumber, FMSG_ALLINONE, "HelpTopic", (const char * const *)Msg, 0,2);
Example
The following function displays a file deletion confirmation dialog:
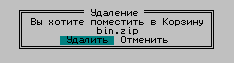
BOOL IsDeleted(char *filename) { const char *Msg[5]; Msg[0]=GetMsg(MTitle); // message title Msg[1]=GetMsg(MIsDeleted); // message body Msg[2]=filename; Msg[3]=GetMsg(MDelete); // last ButtonsNumber (2) strings are buttons Msg[4]=GetMsg(MCancel); return Info.Message(Info.ModuleNumber, 0, "DeleteFile", Msg, sizeof(Msg)/sizeof(Msg[0]), 2) == 0; }Info is defined as a global variable:
struct PluginStartupInfo Info;...and is initialized in the SetStartupInfo function:
void WINAPI _export SetStartupInfo(struct PluginStartupInfo *Info) { ... ::Info=*Info; ... }
See also: