Window that serves as a target for OpenGL rendering. More...
#include <Window.hpp>
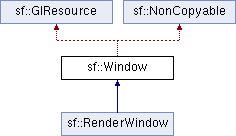
Public Member Functions | |
Window () | |
Default constructor. More... | |
Window (VideoMode mode, const String &title, Uint32 style=Style::Default, const ContextSettings &settings=ContextSettings()) | |
Construct a new window. More... | |
Window (WindowHandle handle, const ContextSettings &settings=ContextSettings()) | |
Construct the window from an existing control. More... | |
virtual | ~Window () |
Destructor. More... | |
void | create (VideoMode mode, const String &title, Uint32 style=Style::Default, const ContextSettings &settings=ContextSettings()) |
Create (or recreate) the window. More... | |
void | create (WindowHandle handle, const ContextSettings &settings=ContextSettings()) |
Create (or recreate) the window from an existing control. More... | |
void | close () |
Close the window and destroy all the attached resources. More... | |
bool | isOpen () const |
Tell whether or not the window is open. More... | |
const ContextSettings & | getSettings () const |
Get the settings of the OpenGL context of the window. More... | |
bool | pollEvent (Event &event) |
Pop the event on top of the event queue, if any, and return it. More... | |
bool | waitEvent (Event &event) |
Wait for an event and return it. More... | |
Vector2i | getPosition () const |
Get the position of the window. More... | |
void | setPosition (const Vector2i &position) |
Change the position of the window on screen. More... | |
Vector2u | getSize () const |
Get the size of the rendering region of the window. More... | |
void | setSize (const Vector2u &size) |
Change the size of the rendering region of the window. More... | |
void | setTitle (const String &title) |
Change the title of the window. More... | |
void | setIcon (unsigned int width, unsigned int height, const Uint8 *pixels) |
Change the window's icon. More... | |
void | setVisible (bool visible) |
Show or hide the window. More... | |
void | setVerticalSyncEnabled (bool enabled) |
Enable or disable vertical synchronization. More... | |
void | setMouseCursorVisible (bool visible) |
Show or hide the mouse cursor. More... | |
void | setKeyRepeatEnabled (bool enabled) |
Enable or disable automatic key-repeat. More... | |
void | setFramerateLimit (unsigned int limit) |
Limit the framerate to a maximum fixed frequency. More... | |
void | setJoystickThreshold (float threshold) |
Change the joystick threshold. More... | |
bool | setActive (bool active=true) const |
Activate or deactivate the window as the current target for OpenGL rendering. More... | |
void | requestFocus () |
Request the current window to be made the active foreground window. More... | |
bool | hasFocus () const |
Check whether the window has the input focus. More... | |
void | display () |
Display on screen what has been rendered to the window so far. More... | |
WindowHandle | getSystemHandle () const |
Get the OS-specific handle of the window. More... | |
Protected Member Functions | |
virtual void | onCreate () |
Function called after the window has been created. More... | |
virtual void | onResize () |
Function called after the window has been resized. More... | |
Static Private Member Functions | |
static void | ensureGlContext () |
Make sure that a valid OpenGL context exists in the current thread. More... | |
Detailed Description
Window that serves as a target for OpenGL rendering.
sf::Window is the main class of the Window module.
It defines an OS window that is able to receive an OpenGL rendering.
A sf::Window can create its own new window, or be embedded into an already existing control using the create(handle) function. This can be useful for embedding an OpenGL rendering area into a view which is part of a bigger GUI with existing windows, controls, etc. It can also serve as embedding an OpenGL rendering area into a window created by another (probably richer) GUI library like Qt or wxWidgets.
The sf::Window class provides a simple interface for manipulating the window: move, resize, show/hide, control mouse cursor, etc. It also provides event handling through its pollEvent() and waitEvent() functions.
Note that OpenGL experts can pass their own parameters (antialiasing level, bits for the depth and stencil buffers, etc.) to the OpenGL context attached to the window, with the sf::ContextSettings structure which is passed as an optional argument when creating the window.
Usage example:
Definition at line 57 of file Window/Window.hpp.
Constructor & Destructor Documentation
sf::Window::Window | ( | ) |
Default constructor.
This constructor doesn't actually create the window, use the other constructors or call create() to do so.
sf::Window::Window | ( | VideoMode | mode, |
const String & | title, | ||
Uint32 | style = Style::Default , |
||
const ContextSettings & | settings = ContextSettings() |
||
) |
Construct a new window.
This constructor creates the window with the size and pixel depth defined in mode. An optional style can be passed to customize the look and behavior of the window (borders, title bar, resizable, closable, ...). If style contains Style::Fullscreen, then mode must be a valid video mode.
The fourth parameter is an optional structure specifying advanced OpenGL context settings such as antialiasing, depth-buffer bits, etc.
- Parameters
-
mode Video mode to use (defines the width, height and depth of the rendering area of the window) title Title of the window style Window style, a bitwise OR combination of sf::Style enumerators settings Additional settings for the underlying OpenGL context
|
explicit |
Construct the window from an existing control.
Use this constructor if you want to create an OpenGL rendering area into an already existing control.
The second parameter is an optional structure specifying advanced OpenGL context settings such as antialiasing, depth-buffer bits, etc.
- Parameters
-
handle Platform-specific handle of the control (HWND on Windows, Window on Linux/FreeBSD, NSWindow on OS X) settings Additional settings for the underlying OpenGL context
|
virtual |
Destructor.
Closes the window and frees all the resources attached to it.
Member Function Documentation
void sf::Window::close | ( | ) |
Close the window and destroy all the attached resources.
After calling this function, the sf::Window instance remains valid and you can call create() to recreate the window. All other functions such as pollEvent() or display() will still work (i.e. you don't have to test isOpen() every time), and will have no effect on closed windows.
void sf::Window::create | ( | VideoMode | mode, |
const String & | title, | ||
Uint32 | style = Style::Default , |
||
const ContextSettings & | settings = ContextSettings() |
||
) |
Create (or recreate) the window.
If the window was already created, it closes it first. If style contains Style::Fullscreen, then mode must be a valid video mode.
The fourth parameter is an optional structure specifying advanced OpenGL context settings such as antialiasing, depth-buffer bits, etc.
- Parameters
-
mode Video mode to use (defines the width, height and depth of the rendering area of the window) title Title of the window style Window style, a bitwise OR combination of sf::Style enumerators settings Additional settings for the underlying OpenGL context
void sf::Window::create | ( | WindowHandle | handle, |
const ContextSettings & | settings = ContextSettings() |
||
) |
Create (or recreate) the window from an existing control.
Use this function if you want to create an OpenGL rendering area into an already existing control. If the window was already created, it closes it first.
The second parameter is an optional structure specifying advanced OpenGL context settings such as antialiasing, depth-buffer bits, etc.
- Parameters
-
handle Platform-specific handle of the control (HWND on Windows, Window on Linux/FreeBSD, NSWindow on OS X) settings Additional settings for the underlying OpenGL context
void sf::Window::display | ( | ) |
Display on screen what has been rendered to the window so far.
This function is typically called after all OpenGL rendering has been done for the current frame, in order to show it on screen.
Vector2i sf::Window::getPosition | ( | ) | const |
const ContextSettings& sf::Window::getSettings | ( | ) | const |
Get the settings of the OpenGL context of the window.
Note that these settings may be different from what was passed to the constructor or the create() function, if one or more settings were not supported. In this case, SFML chose the closest match.
- Returns
- Structure containing the OpenGL context settings
Vector2u sf::Window::getSize | ( | ) | const |
Get the size of the rendering region of the window.
The size doesn't include the titlebar and borders of the window.
- Returns
- Size in pixels
- See also
- setSize
WindowHandle sf::Window::getSystemHandle | ( | ) | const |
Get the OS-specific handle of the window.
The type of the returned handle is sf::WindowHandle, which is a typedef to the handle type defined by the OS. You shouldn't need to use this function, unless you have very specific stuff to implement that SFML doesn't support, or implement a temporary workaround until a bug is fixed. The type is HWND on Windows, Window on Linux/FreeBSD and NSWindow on OS X.
- Returns
- System handle of the window
bool sf::Window::hasFocus | ( | ) | const |
Check whether the window has the input focus.
At any given time, only one window may have the input focus to receive input events such as keystrokes or most mouse events.
- Returns
- True if window has focus, false otherwise
- See also
- requestFocus
bool sf::Window::isOpen | ( | ) | const |
Tell whether or not the window is open.
This function returns whether or not the window exists. Note that a hidden window (setVisible(false)) is open (therefore this function would return true).
- Returns
- True if the window is open, false if it has been closed
|
protectedvirtual |
Function called after the window has been created.
This function is called so that derived classes can perform their own specific initialization as soon as the window is created.
Reimplemented in sf::RenderWindow.
|
protectedvirtual |
Function called after the window has been resized.
This function is called so that derived classes can perform custom actions when the size of the window changes.
Reimplemented in sf::RenderWindow.
bool sf::Window::pollEvent | ( | Event & | event | ) |
Pop the event on top of the event queue, if any, and return it.
This function is not blocking: if there's no pending event then it will return false and leave event unmodified. Note that more than one event may be present in the event queue, thus you should always call this function in a loop to make sure that you process every pending event.
- Parameters
-
event Event to be returned
- Returns
- True if an event was returned, or false if the event queue was empty
- See also
- waitEvent
void sf::Window::requestFocus | ( | ) |
Request the current window to be made the active foreground window.
At any given time, only one window may have the input focus to receive input events such as keystrokes or mouse events. If a window requests focus, it only hints to the operating system, that it would like to be focused. The operating system is free to deny the request. This is not to be confused with setActive().
- See also
- hasFocus
bool sf::Window::setActive | ( | bool | active = true | ) | const |
Activate or deactivate the window as the current target for OpenGL rendering.
A window is active only on the current thread, if you want to make it active on another thread you have to deactivate it on the previous thread first if it was active. Only one window can be active on a thread at a time, thus the window previously active (if any) automatically gets deactivated. This is not to be confused with requestFocus().
- Parameters
-
active True to activate, false to deactivate
- Returns
- True if operation was successful, false otherwise
void sf::Window::setFramerateLimit | ( | unsigned int | limit | ) |
Limit the framerate to a maximum fixed frequency.
If a limit is set, the window will use a small delay after each call to display() to ensure that the current frame lasted long enough to match the framerate limit. SFML will try to match the given limit as much as it can, but since it internally uses sf::sleep, whose precision depends on the underlying OS, the results may be a little unprecise as well (for example, you can get 65 FPS when requesting 60).
- Parameters
-
limit Framerate limit, in frames per seconds (use 0 to disable limit)
void sf::Window::setIcon | ( | unsigned int | width, |
unsigned int | height, | ||
const Uint8 * | pixels | ||
) |
Change the window's icon.
pixels must be an array of width x height pixels in 32-bits RGBA format.
The OS default icon is used by default.
- Parameters
-
width Icon's width, in pixels height Icon's height, in pixels pixels Pointer to the array of pixels in memory. The pixels are copied, so you need not keep the source alive after calling this function.
- See also
- setTitle
void sf::Window::setJoystickThreshold | ( | float | threshold | ) |
Change the joystick threshold.
The joystick threshold is the value below which no JoystickMoved event will be generated.
The threshold value is 0.1 by default.
- Parameters
-
threshold New threshold, in the range [0, 100]
void sf::Window::setKeyRepeatEnabled | ( | bool | enabled | ) |
Enable or disable automatic key-repeat.
If key repeat is enabled, you will receive repeated KeyPressed events while keeping a key pressed. If it is disabled, you will only get a single event when the key is pressed.
Key repeat is enabled by default.
- Parameters
-
enabled True to enable, false to disable
void sf::Window::setMouseCursorVisible | ( | bool | visible | ) |
Show or hide the mouse cursor.
The mouse cursor is visible by default.
- Parameters
-
visible True to show the mouse cursor, false to hide it
void sf::Window::setPosition | ( | const Vector2i & | position | ) |
Change the position of the window on screen.
This function only works for top-level windows (i.e. it will be ignored for windows created from the handle of a child window/control).
- Parameters
-
position New position, in pixels
- See also
- getPosition
void sf::Window::setSize | ( | const Vector2u & | size | ) |
Change the size of the rendering region of the window.
- Parameters
-
size New size, in pixels
- See also
- getSize
void sf::Window::setTitle | ( | const String & | title | ) |
void sf::Window::setVerticalSyncEnabled | ( | bool | enabled | ) |
Enable or disable vertical synchronization.
Activating vertical synchronization will limit the number of frames displayed to the refresh rate of the monitor. This can avoid some visual artifacts, and limit the framerate to a good value (but not constant across different computers).
Vertical synchronization is disabled by default.
- Parameters
-
enabled True to enable v-sync, false to deactivate it
void sf::Window::setVisible | ( | bool | visible | ) |
Show or hide the window.
The window is shown by default.
- Parameters
-
visible True to show the window, false to hide it
bool sf::Window::waitEvent | ( | Event & | event | ) |
Wait for an event and return it.
This function is blocking: if there's no pending event then it will wait until an event is received. After this function returns (and no error occurred), the event object is always valid and filled properly. This function is typically used when you have a thread that is dedicated to events handling: you want to make this thread sleep as long as no new event is received.
- Parameters
-
event Event to be returned
- Returns
- False if any error occurred
- See also
- pollEvent
The documentation for this class was generated from the following file: