Pointer to a thread-local variable. More...
#include <ThreadLocalPtr.hpp>
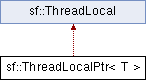
Public Member Functions | |
ThreadLocalPtr (T *value=NULL) | |
Default constructor. More... | |
T & | operator* () const |
Overload of unary operator *. More... | |
T * | operator-> () const |
Overload of operator -> More... | |
operator T * () const | |
Conversion operator to implicitly convert the pointer to its raw pointer type (T*) More... | |
ThreadLocalPtr< T > & | operator= (T *value) |
Assignment operator for a raw pointer parameter. More... | |
ThreadLocalPtr< T > & | operator= (const ThreadLocalPtr< T > &right) |
Assignment operator for a ThreadLocalPtr parameter. More... | |
Private Member Functions | |
void | setValue (void *value) |
Set the thread-specific value of the variable. More... | |
void * | getValue () const |
Retrieve the thread-specific value of the variable. More... | |
Detailed Description
template<typename T>
class sf::ThreadLocalPtr< T >
Pointer to a thread-local variable.
sf::ThreadLocalPtr is a type-safe wrapper for storing pointers to thread-local variables.
A thread-local variable holds a different value for each different thread, unlike normal variables that are shared.
Its usage is completely transparent, so that it is similar to manipulating the raw pointer directly (like any smart pointer).
Usage example:
ThreadLocalPtr is designed for internal use; however you can use it if you feel like it fits well your implementation.
Definition at line 41 of file ThreadLocalPtr.hpp.
Constructor & Destructor Documentation
sf::ThreadLocalPtr< T >::ThreadLocalPtr | ( | T * | value = NULL | ) |
Default constructor.
- Parameters
-
value Optional value to initialize the variable
Member Function Documentation
sf::ThreadLocalPtr< T >::operator T * | ( | ) | const |
Conversion operator to implicitly convert the pointer to its raw pointer type (T*)
- Returns
- Pointer to the actual object
T& sf::ThreadLocalPtr< T >::operator* | ( | ) | const |
Overload of unary operator *.
Like raw pointers, applying the * operator returns a reference to the pointed-to object.
- Returns
- Reference to the thread-local variable
T* sf::ThreadLocalPtr< T >::operator-> | ( | ) | const |
Overload of operator ->
Similarly to raw pointers, applying the -> operator returns the pointed-to object.
- Returns
- Pointer to the thread-local variable
ThreadLocalPtr<T>& sf::ThreadLocalPtr< T >::operator= | ( | T * | value | ) |
Assignment operator for a raw pointer parameter.
- Parameters
-
value Pointer to assign
- Returns
- Reference to self
ThreadLocalPtr<T>& sf::ThreadLocalPtr< T >::operator= | ( | const ThreadLocalPtr< T > & | right | ) |
Assignment operator for a ThreadLocalPtr parameter.
- Parameters
-
right ThreadLocalPtr to assign
- Returns
- Reference to self
The documentation for this class was generated from the following file: