Shader class (vertex and fragment) More...
#include <Shader.hpp>
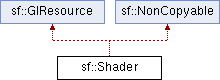
Classes | |
struct | CurrentTextureType |
Special type that can be passed to setParameter, and that represents the texture of the object being drawn. More... | |
Public Types | |
enum | Type { Vertex, Fragment } |
Types of shaders. More... | |
Public Member Functions | |
Shader () | |
Default constructor. More... | |
~Shader () | |
Destructor. More... | |
bool | loadFromFile (const std::string &filename, Type type) |
Load either the vertex or fragment shader from a file. More... | |
bool | loadFromFile (const std::string &vertexShaderFilename, const std::string &fragmentShaderFilename) |
Load both the vertex and fragment shaders from files. More... | |
bool | loadFromMemory (const std::string &shader, Type type) |
Load either the vertex or fragment shader from a source code in memory. More... | |
bool | loadFromMemory (const std::string &vertexShader, const std::string &fragmentShader) |
Load both the vertex and fragment shaders from source codes in memory. More... | |
bool | loadFromStream (InputStream &stream, Type type) |
Load either the vertex or fragment shader from a custom stream. More... | |
bool | loadFromStream (InputStream &vertexShaderStream, InputStream &fragmentShaderStream) |
Load both the vertex and fragment shaders from custom streams. More... | |
void | setParameter (const std::string &name, float x) |
Change a float parameter of the shader. More... | |
void | setParameter (const std::string &name, float x, float y) |
Change a 2-components vector parameter of the shader. More... | |
void | setParameter (const std::string &name, float x, float y, float z) |
Change a 3-components vector parameter of the shader. More... | |
void | setParameter (const std::string &name, float x, float y, float z, float w) |
Change a 4-components vector parameter of the shader. More... | |
void | setParameter (const std::string &name, const Vector2f &vector) |
Change a 2-components vector parameter of the shader. More... | |
void | setParameter (const std::string &name, const Vector3f &vector) |
Change a 3-components vector parameter of the shader. More... | |
void | setParameter (const std::string &name, const Color &color) |
Change a color parameter of the shader. More... | |
void | setParameter (const std::string &name, const Transform &transform) |
Change a matrix parameter of the shader. More... | |
void | setParameter (const std::string &name, const Texture &texture) |
Change a texture parameter of the shader. More... | |
void | setParameter (const std::string &name, CurrentTextureType) |
Change a texture parameter of the shader. More... | |
unsigned int | getNativeHandle () const |
Get the underlying OpenGL handle of the shader. More... | |
Static Public Member Functions | |
static void | bind (const Shader *shader) |
Bind a shader for rendering. More... | |
static bool | isAvailable () |
Tell whether or not the system supports shaders. More... | |
Static Public Attributes | |
static CurrentTextureType | CurrentTexture |
Represents the texture of the object being drawn. More... | |
Static Private Member Functions | |
static void | ensureGlContext () |
Make sure that a valid OpenGL context exists in the current thread. More... | |
Detailed Description
Shader class (vertex and fragment)
Shaders are programs written using a specific language, executed directly by the graphics card and allowing to apply real-time operations to the rendered entities.
There are two kinds of shaders:
- Vertex shaders, that process vertices
- Fragment (pixel) shaders, that process pixels
A sf::Shader can be composed of either a vertex shader alone, a fragment shader alone, or both combined (see the variants of the load functions).
Shaders are written in GLSL, which is a C-like language dedicated to OpenGL shaders. You'll probably need to learn its basics before writing your own shaders for SFML.
Like any C/C++ program, a shader has its own variables that you can set from your C++ application. sf::Shader handles 5 different types of variables:
- floats
- vectors (2, 3 or 4 components)
- colors
- textures
- transforms (matrices)
The value of the variables can be changed at any time with the various overloads of the setParameter function:
The special Shader::CurrentTexture argument maps the given texture variable to the current texture of the object being drawn (which cannot be known in advance).
To apply a shader to a drawable, you must pass it as an additional parameter to the Draw function:
... which is in fact just a shortcut for this:
In the code above we pass a pointer to the shader, because it may be null (which means "no shader").
Shaders can be used on any drawable, but some combinations are not interesting. For example, using a vertex shader on a sf::Sprite is limited because there are only 4 vertices, the sprite would have to be subdivided in order to apply wave effects. Another bad example is a fragment shader with sf::Text: the texture of the text is not the actual text that you see on screen, it is a big texture containing all the characters of the font in an arbitrary order; thus, texture lookups on pixels other than the current one may not give you the expected result.
Shaders can also be used to apply global post-effects to the current contents of the target (like the old sf::PostFx class in SFML 1). This can be done in two different ways:
- draw everything to a sf::RenderTexture, then draw it to the main target using the shader
- draw everything directly to the main target, then use sf::Texture::update(Window&) to copy its contents to a texture and draw it to the main target using the shader
The first technique is more optimized because it doesn't involve retrieving the target's pixels to system memory, but the second one doesn't impact the rendering process and can be easily inserted anywhere without impacting all the code.
Like sf::Texture that can be used as a raw OpenGL texture, sf::Shader can also be used directly as a raw shader for custom OpenGL geometry.
Definition at line 51 of file Shader.hpp.
Member Enumeration Documentation
enum sf::Shader::Type |
Types of shaders.
Enumerator | |
---|---|
Vertex |
Vertex shader. |
Fragment |
Fragment (pixel) shader. |
Definition at line 59 of file Shader.hpp.
Constructor & Destructor Documentation
sf::Shader::Shader | ( | ) |
Default constructor.
This constructor creates an invalid shader.
sf::Shader::~Shader | ( | ) |
Destructor.
Member Function Documentation
|
static |
Bind a shader for rendering.
This function is not part of the graphics API, it mustn't be used when drawing SFML entities. It must be used only if you mix sf::Shader with OpenGL code.
- Parameters
-
shader Shader to bind, can be null to use no shader
unsigned int sf::Shader::getNativeHandle | ( | ) | const |
Get the underlying OpenGL handle of the shader.
You shouldn't need to use this function, unless you have very specific stuff to implement that SFML doesn't support, or implement a temporary workaround until a bug is fixed.
- Returns
- OpenGL handle of the shader or 0 if not yet loaded
|
static |
Tell whether or not the system supports shaders.
This function should always be called before using the shader features. If it returns false, then any attempt to use sf::Shader will fail.
Note: The first call to this function, whether by your code or SFML will result in a context switch.
- Returns
- True if shaders are supported, false otherwise
bool sf::Shader::loadFromFile | ( | const std::string & | filename, |
Type | type | ||
) |
Load either the vertex or fragment shader from a file.
This function loads a single shader, either vertex or fragment, identified by the second argument. The source must be a text file containing a valid shader in GLSL language. GLSL is a C-like language dedicated to OpenGL shaders; you'll probably need to read a good documentation for it before writing your own shaders.
- Parameters
-
filename Path of the vertex or fragment shader file to load type Type of shader (vertex or fragment)
- Returns
- True if loading succeeded, false if it failed
- See also
- loadFromMemory, loadFromStream
bool sf::Shader::loadFromFile | ( | const std::string & | vertexShaderFilename, |
const std::string & | fragmentShaderFilename | ||
) |
Load both the vertex and fragment shaders from files.
This function loads both the vertex and the fragment shaders. If one of them fails to load, the shader is left empty (the valid shader is unloaded). The sources must be text files containing valid shaders in GLSL language. GLSL is a C-like language dedicated to OpenGL shaders; you'll probably need to read a good documentation for it before writing your own shaders.
- Parameters
-
vertexShaderFilename Path of the vertex shader file to load fragmentShaderFilename Path of the fragment shader file to load
- Returns
- True if loading succeeded, false if it failed
- See also
- loadFromMemory, loadFromStream
bool sf::Shader::loadFromMemory | ( | const std::string & | shader, |
Type | type | ||
) |
Load either the vertex or fragment shader from a source code in memory.
This function loads a single shader, either vertex or fragment, identified by the second argument. The source code must be a valid shader in GLSL language. GLSL is a C-like language dedicated to OpenGL shaders; you'll probably need to read a good documentation for it before writing your own shaders.
- Parameters
-
shader String containing the source code of the shader type Type of shader (vertex or fragment)
- Returns
- True if loading succeeded, false if it failed
- See also
- loadFromFile, loadFromStream
bool sf::Shader::loadFromMemory | ( | const std::string & | vertexShader, |
const std::string & | fragmentShader | ||
) |
Load both the vertex and fragment shaders from source codes in memory.
This function loads both the vertex and the fragment shaders. If one of them fails to load, the shader is left empty (the valid shader is unloaded). The sources must be valid shaders in GLSL language. GLSL is a C-like language dedicated to OpenGL shaders; you'll probably need to read a good documentation for it before writing your own shaders.
- Parameters
-
vertexShader String containing the source code of the vertex shader fragmentShader String containing the source code of the fragment shader
- Returns
- True if loading succeeded, false if it failed
- See also
- loadFromFile, loadFromStream
bool sf::Shader::loadFromStream | ( | InputStream & | stream, |
Type | type | ||
) |
Load either the vertex or fragment shader from a custom stream.
This function loads a single shader, either vertex or fragment, identified by the second argument. The source code must be a valid shader in GLSL language. GLSL is a C-like language dedicated to OpenGL shaders; you'll probably need to read a good documentation for it before writing your own shaders.
- Parameters
-
stream Source stream to read from type Type of shader (vertex or fragment)
- Returns
- True if loading succeeded, false if it failed
- See also
- loadFromFile, loadFromMemory
bool sf::Shader::loadFromStream | ( | InputStream & | vertexShaderStream, |
InputStream & | fragmentShaderStream | ||
) |
Load both the vertex and fragment shaders from custom streams.
This function loads both the vertex and the fragment shaders. If one of them fails to load, the shader is left empty (the valid shader is unloaded). The source codes must be valid shaders in GLSL language. GLSL is a C-like language dedicated to OpenGL shaders; you'll probably need to read a good documentation for it before writing your own shaders.
- Parameters
-
vertexShaderStream Source stream to read the vertex shader from fragmentShaderStream Source stream to read the fragment shader from
- Returns
- True if loading succeeded, false if it failed
- See also
- loadFromFile, loadFromMemory
void sf::Shader::setParameter | ( | const std::string & | name, |
float | x | ||
) |
Change a float parameter of the shader.
name is the name of the variable to change in the shader. The corresponding parameter in the shader must be a float (float GLSL type).
Example:
- Parameters
-
name Name of the parameter in the shader x Value to assign
void sf::Shader::setParameter | ( | const std::string & | name, |
float | x, | ||
float | y | ||
) |
Change a 2-components vector parameter of the shader.
name is the name of the variable to change in the shader. The corresponding parameter in the shader must be a 2x1 vector (vec2 GLSL type).
Example:
- Parameters
-
name Name of the parameter in the shader x First component of the value to assign y Second component of the value to assign
void sf::Shader::setParameter | ( | const std::string & | name, |
float | x, | ||
float | y, | ||
float | z | ||
) |
Change a 3-components vector parameter of the shader.
name is the name of the variable to change in the shader. The corresponding parameter in the shader must be a 3x1 vector (vec3 GLSL type).
Example:
- Parameters
-
name Name of the parameter in the shader x First component of the value to assign y Second component of the value to assign z Third component of the value to assign
void sf::Shader::setParameter | ( | const std::string & | name, |
float | x, | ||
float | y, | ||
float | z, | ||
float | w | ||
) |
Change a 4-components vector parameter of the shader.
name is the name of the variable to change in the shader. The corresponding parameter in the shader must be a 4x1 vector (vec4 GLSL type).
Example:
- Parameters
-
name Name of the parameter in the shader x First component of the value to assign y Second component of the value to assign z Third component of the value to assign w Fourth component of the value to assign
void sf::Shader::setParameter | ( | const std::string & | name, |
const Vector2f & | vector | ||
) |
Change a 2-components vector parameter of the shader.
name is the name of the variable to change in the shader. The corresponding parameter in the shader must be a 2x1 vector (vec2 GLSL type).
Example:
- Parameters
-
name Name of the parameter in the shader vector Vector to assign
void sf::Shader::setParameter | ( | const std::string & | name, |
const Vector3f & | vector | ||
) |
Change a 3-components vector parameter of the shader.
name is the name of the variable to change in the shader. The corresponding parameter in the shader must be a 3x1 vector (vec3 GLSL type).
Example:
- Parameters
-
name Name of the parameter in the shader vector Vector to assign
void sf::Shader::setParameter | ( | const std::string & | name, |
const Color & | color | ||
) |
Change a color parameter of the shader.
name is the name of the variable to change in the shader. The corresponding parameter in the shader must be a 4x1 vector (vec4 GLSL type).
It is important to note that the components of the color are normalized before being passed to the shader. Therefore, they are converted from range [0 .. 255] to range [0 .. 1]. For example, a sf::Color(255, 125, 0, 255) will be transformed to a vec4(1.0, 0.5, 0.0, 1.0) in the shader.
Example:
- Parameters
-
name Name of the parameter in the shader color Color to assign
void sf::Shader::setParameter | ( | const std::string & | name, |
const Transform & | transform | ||
) |
Change a matrix parameter of the shader.
name is the name of the variable to change in the shader. The corresponding parameter in the shader must be a 4x4 matrix (mat4 GLSL type).
Example:
- Parameters
-
name Name of the parameter in the shader transform Transform to assign
void sf::Shader::setParameter | ( | const std::string & | name, |
const Texture & | texture | ||
) |
Change a texture parameter of the shader.
name is the name of the variable to change in the shader. The corresponding parameter in the shader must be a 2D texture (sampler2D GLSL type).
Example:
It is important to note that texture must remain alive as long as the shader uses it, no copy is made internally.
To use the texture of the object being draw, which cannot be known in advance, you can pass the special value sf::Shader::CurrentTexture:
- Parameters
-
name Name of the texture in the shader texture Texture to assign
void sf::Shader::setParameter | ( | const std::string & | name, |
CurrentTextureType | |||
) |
Change a texture parameter of the shader.
This overload maps a shader texture variable to the texture of the object being drawn, which cannot be known in advance. The second argument must be sf::Shader::CurrentTexture. The corresponding parameter in the shader must be a 2D texture (sampler2D GLSL type).
Example:
- Parameters
-
name Name of the texture in the shader
Member Data Documentation
|
static |
Represents the texture of the object being drawn.
Definition at line 80 of file Shader.hpp.
The documentation for this class was generated from the following file: