PxCloth Class Reference
[Cloth]
Set of connected particles tailored towards simulating character cloth. A cloth object consists of the following components:.
More...
#include <PxCloth.h>
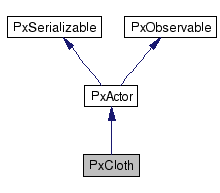
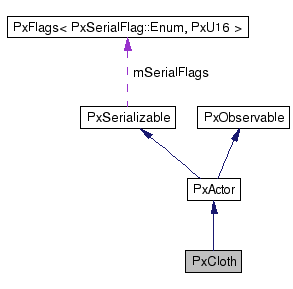
Public Member Functions | |
virtual void | release ()=0 |
Deletes the actor. | |
virtual PxClothFabric * | getFabric () const =0 |
Returns a pointer to the corresponding cloth fabric. | |
virtual void | setParticles (const PxClothParticle *currentParticles, const PxClothParticle *previousParticles)=0 |
Updates cloth particle location or inverse weight for current and previous particle state. | |
virtual PxU32 | getNbParticles () const =0 |
Returns the number of particles. | |
virtual void | setMotionConstraints (const PxClothParticleMotionConstraint *motionConstraints)=0 |
Updates motion constraints (position and radius of the constraint sphere). | |
virtual bool | getMotionConstraints (PxClothParticleMotionConstraint *motionConstraintBuffer) const =0 |
Copies motion constraints to the user provided buffer. | |
virtual PxU32 | getNbMotionConstraints () const =0 |
Returns the number of motion constraints. | |
virtual void | setMotionConstraintScaleBias (PxReal scale, PxReal bias)=0 |
Specifies motion constraint scale and bias. | |
virtual void | getMotionConstraintScaleBias (PxReal &scale, PxReal &bias) const =0 |
Reads back scale and bias factor for motion constraints. | |
virtual void | setSeparationConstraints (const PxClothParticleSeparationConstraint *separationConstraints)=0 |
Updates separation constraints (position and radius of the constraint sphere). | |
virtual bool | getSeparationConstraints (PxClothParticleSeparationConstraint *separationConstraintBuffer) const =0 |
Copies separation constraints to the user provided buffer. | |
virtual PxU32 | getNbSeparationConstraints () const =0 |
Returns the number of separation constraints. | |
virtual void | clearInterpolation ()=0 |
Assign current to previous positions for collision shapes, motion constraints, and separation constraints. | |
virtual void | setParticleAccelerations (const PxVec4 *particleAccelerations)=0 |
Updates particle accelerations, w component is ignored. | |
virtual bool | getParticleAccelerations (PxVec4 *particleAccelerationsBuffer) const =0 |
Copies particle accelerations to the user provided buffer. | |
virtual PxU32 | getNbParticleAccelerations () const =0 |
Returns the number of particle accelerations. | |
virtual void | setCollisionSpheres (const PxClothCollisionSphere *sphereBuffer)=0 |
Updates location and radii of collision spheres. | |
virtual void | getCollisionData (PxClothCollisionSphere *sphereBuffer, PxU32 *pairIndexBuffer, PxClothCollisionPlane *planesBuffer, PxU32 *convexMaskBuffer) const =0 |
Retrieves the collision shapes. | |
virtual PxU32 | getNbCollisionSpheres () const =0 |
Returns the number of collision spheres. | |
virtual PxU32 | getNbCollisionSpherePairs () const =0 |
Returns the number of collision capsules. | |
virtual void | addCollisionPlane (const PxClothCollisionPlane &plane)=0 |
Adds a collision plane. | |
virtual void | removeCollisionPlane (PxU32 index)=0 |
Removes a collision plane. | |
virtual void | setCollisionPlanes (const PxClothCollisionPlane *planesBuffer)=0 |
Updates positions of collision planes. | |
virtual void | addCollisionConvex (PxU32 mask)=0 |
Adds a new collision convex. | |
virtual void | removeCollisionConvex (PxU32 index)=0 |
Removes a collision convex. | |
virtual PxU32 | getNbCollisionPlanes () const =0 |
Returns the number of collision planes. | |
virtual PxU32 | getNbCollisionConvexes () const =0 |
Returns the number of collision convexes. | |
virtual void | setVirtualParticles (PxU32 numParticles, const PxU32 *triangleVertexAndWeightIndices, PxU32 weightTableSize, const PxVec3 *triangleVertexWeightTable)=0 |
Assigns virtual particles. | |
virtual PxU32 | getNbVirtualParticles () const =0 |
Returns the number of virtual particles. | |
virtual void | getVirtualParticles (PxU32 *userTriangleVertexAndWeightIndices) const =0 |
Copies index array of virtual particles to the user provided buffer. | |
virtual PxU32 | getNbVirtualParticleWeights () const =0 |
Returns the number of the virtual particle weights. | |
virtual void | getVirtualParticleWeights (PxVec3 *userTriangleVertexWeightTable) const =0 |
Copies weight table of virtual particles to the user provided buffer. | |
virtual void | setGlobalPose (const PxTransform &pose)=0 |
Sets global pose. | |
virtual PxTransform | getGlobalPose () const =0 |
Returns global pose. | |
virtual void | setTargetPose (const PxTransform &pose)=0 |
Sets target pose. | |
virtual void | setInertiaScale (PxReal scale)=0 |
Sets the acceleration scale factor to adjust inertia effect from global pose changes. | |
virtual PxReal | getInertiaScale () const =0 |
Returns acceleration scale parameter. | |
virtual void | setExternalAcceleration (PxVec3 acceleration)=0 |
Sets external particle accelerations. | |
virtual PxVec3 | getExternalAcceleration () const =0 |
Returns external acceleration. | |
virtual void | setDampingCoefficient (PxReal dampingCoefficient)=0 |
Sets the damping coefficient. | |
virtual PxReal | getDampingCoefficient () const =0 |
Returns the damping coefficient. | |
virtual void | setFrictionCoefficient (PxReal frictionCoefficient)=0 |
Sets the collision friction coefficient. | |
virtual PxReal | getFrictionCoefficient () const =0 |
Returns the friction coefficient. | |
virtual void | setDragCoefficient (PxReal dragCoefficient)=0 |
Sets the drag coefficient. | |
virtual PxReal | getDragCoefficient () const =0 |
Returns the drag coefficient. | |
virtual void | setCollisionMassScale (PxReal scalingCoefficient)=0 |
Sets the collision mass scaling coefficient. | |
virtual PxReal | getCollisionMassScale () const =0 |
Returns the mass-scaling coefficient. | |
virtual void | setSolverFrequency (PxReal frequency)=0 |
Sets the solver frequency parameter. | |
virtual PxReal | getSolverFrequency () const =0 |
Returns solver frequency. | |
virtual void | setPhaseSolverConfig (PxClothFabricPhaseType::Enum phaseType, const PxClothPhaseSolverConfig &config)=0 |
Sets solver configuration per phase type. | |
virtual PxClothPhaseSolverConfig | getPhaseSolverConfig (PxClothFabricPhaseType::Enum phaseType) const =0 |
Reads solver configuration for specified phase type. | |
virtual void | setClothFlag (PxClothFlag::Enum flag, bool value)=0 |
Sets cloth flags (e.g. use GPU or not, use CCD or not). | |
virtual PxClothFlags | getClothFlags () const =0 |
Returns cloth flags. | |
virtual bool | isSleeping () const =0 |
Returns true if cloth is in sleep state. | |
virtual PxReal | getSleepLinearVelocity () const =0 |
Returns the velocity threshold for putting cloth in sleep state. | |
virtual void | setSleepLinearVelocity (PxReal threshold)=0 |
Sets the velocity threshold for putting cloth in sleep state. | |
virtual void | wakeUp (PxReal wakeCounterValue=PX_SLEEP_INTERVAL)=0 |
Forces cloth to wake up from sleep state. | |
virtual void | putToSleep ()=0 |
Forces cloth to be put in sleep state. | |
virtual PxClothReadData * | lockClothReadData () const =0 |
Locks the cloth solver so that external applications can safely read back particle data. | |
virtual PxReal | getPreviousTimeStep () const =0 |
Returns previous time step size. | |
virtual PxBounds3 | getWorldBounds () const =0 |
Returns world space bounding box. | |
virtual const char * | getConcreteTypeName () const |
returns string name of dynamic type. | |
Protected Member Functions | |
PxCloth (PxRefResolver &v) | |
PX_INLINE | PxCloth () |
virtual | ~PxCloth () |
virtual bool | isKindOf (const char *name) const |
Detailed Description
Set of connected particles tailored towards simulating character cloth. A cloth object consists of the following components:.
- A set of particles that sample that cloth to simulate. The sampling does not need to be regular. Particles are simulated in local space, which allows tuning the effect of changes to the global pose on the particles.
- Distance, bending, and shearing constraints between particles. These are stored in a PxClothFabric instance which can be shared across cloth instances.
- Spheres, capsules, planes, and convexes collision shapes. These shapes are all treated separately to the main PhysX rigid body scene.
- Virtual particles can be used to improve collision at a finer scale than the cloth sampling.
- Motion and separation constraints are used to limit the particle movement within or outside of a sphere.
Constructor & Destructor Documentation
PxCloth::PxCloth | ( | PxRefResolver & | v | ) | [inline, protected] |
PX_INLINE PxCloth::PxCloth | ( | ) | [inline, protected] |
virtual PxCloth::~PxCloth | ( | ) | [inline, protected, virtual] |
Member Function Documentation
virtual void PxCloth::addCollisionConvex | ( | PxU32 | mask | ) | [pure virtual] |
Adds a new collision convex.
A collision convex is defined as the intersection of planes.
- Parameters:
-
[in] mask The bitmask of the planes that make up the convex.
virtual void PxCloth::addCollisionPlane | ( | const PxClothCollisionPlane & | plane | ) | [pure virtual] |
Adds a collision plane.
- Parameters:
-
[in] plane New collision plane.
- Note:
- Planes are not used for collision until they are added to a convex object, see addCollisionConvex().
A maximum of 32 planes are supported.
virtual void PxCloth::clearInterpolation | ( | ) | [pure virtual] |
Assign current to previous positions for collision shapes, motion constraints, and separation constraints.
This allows to prevent false interpolation after leaping to an animation frame, for example. After calling clearInterpolation(), the current positions will be used without interpolation. New positions can be set afterwards to interpolate to by the end of the next frame.
virtual PxClothFlags PxCloth::getClothFlags | ( | ) | const [pure virtual] |
Returns cloth flags.
- Returns:
- Cloth flags.
virtual void PxCloth::getCollisionData | ( | PxClothCollisionSphere * | sphereBuffer, | |
PxU32 * | pairIndexBuffer, | |||
PxClothCollisionPlane * | planesBuffer, | |||
PxU32 * | convexMaskBuffer | |||
) | const [pure virtual] |
Retrieves the collision shapes.
Returns collision spheres, capsules, convexes, and triangles that were added through the addCollision*() methods and modified through the setCollision*() methods.
- Parameters:
-
[out] sphereBuffer Spheres destination buffer, must be the same length as getNbCollisionSpheres(). [out] pairIndexBuffer Capsules destination buffer, must be the same length as 2*getNbCollisionSpherePairs(). [out] planesBuffer Planes destination buffer, must be the same length as getNbCollisionPlanes(). [out] convexMaskBuffer Convexes destination buffer, must be the same length as getNbCollisionConvexes().
- Note:
- Returns the positions at the end of the next simulate() call as specified by the setCollision*() methods.
virtual PxReal PxCloth::getCollisionMassScale | ( | ) | const [pure virtual] |
Returns the mass-scaling coefficient.
- Returns:
- Mass-scaling coefficient.
virtual const char* PxCloth::getConcreteTypeName | ( | ) | const [inline, virtual] |
returns string name of dynamic type.
- Returns:
- class name of most derived type of this object.
Reimplemented from PxSerializable.
virtual PxReal PxCloth::getDampingCoefficient | ( | ) | const [pure virtual] |
Returns the damping coefficient.
- Returns:
- Damping coefficient.
virtual PxReal PxCloth::getDragCoefficient | ( | ) | const [pure virtual] |
Returns the drag coefficient.
- Returns:
- Drag coefficient.
virtual PxVec3 PxCloth::getExternalAcceleration | ( | ) | const [pure virtual] |
Returns external acceleration.
- Returns:
- External acceleration in global coordinates.
virtual PxClothFabric* PxCloth::getFabric | ( | ) | const [pure virtual] |
Returns a pointer to the corresponding cloth fabric.
- Returns:
- The associated cloth fabric.
virtual PxReal PxCloth::getFrictionCoefficient | ( | ) | const [pure virtual] |
Returns the friction coefficient.
- Returns:
- Friction coefficient.
virtual PxTransform PxCloth::getGlobalPose | ( | ) | const [pure virtual] |
Returns global pose.
- Returns:
- Global pose as specified by the last setGlobalPose() or setTargetPose() call.
virtual PxReal PxCloth::getInertiaScale | ( | ) | const [pure virtual] |
Returns acceleration scale parameter.
- Returns:
- Acceleration scale parameter.
virtual bool PxCloth::getMotionConstraints | ( | PxClothParticleMotionConstraint * | motionConstraintBuffer | ) | const [pure virtual] |
Copies motion constraints to the user provided buffer.
- Parameters:
-
[out] motionConstraintBuffer Destination buffer, must be at least getNbMotionConstraints().
- Returns:
- True if the copy was successful.
virtual PxU32 PxCloth::getNbCollisionConvexes | ( | ) | const [pure virtual] |
Returns the number of collision convexes.
- Returns:
- Number of collision convexes.
virtual PxU32 PxCloth::getNbCollisionPlanes | ( | ) | const [pure virtual] |
Returns the number of collision planes.
- Returns:
- Number of collision planes.
virtual PxU32 PxCloth::getNbCollisionSpherePairs | ( | ) | const [pure virtual] |
Returns the number of collision capsules.
- Returns:
- Number of collision capsules.
virtual PxU32 PxCloth::getNbCollisionSpheres | ( | ) | const [pure virtual] |
Returns the number of collision spheres.
- Returns:
- Number of collision spheres.
virtual PxU32 PxCloth::getNbMotionConstraints | ( | ) | const [pure virtual] |
Returns the number of motion constraints.
- Returns:
- Number of motion constraints (same as getNbPartices() if enabled, 0 otherwise).
virtual PxU32 PxCloth::getNbParticleAccelerations | ( | ) | const [pure virtual] |
Returns the number of particle accelerations.
- Returns:
- Number of particle accelerations (same as getNbPartices() if enabled, 0 otherwise).
virtual PxU32 PxCloth::getNbParticles | ( | ) | const [pure virtual] |
Returns the number of particles.
- Returns:
- Number of particles.
virtual PxU32 PxCloth::getNbSeparationConstraints | ( | ) | const [pure virtual] |
Returns the number of separation constraints.
- Returns:
- Number of separation constraints (same as getNbPartices() if enabled, 0 otherwise).
virtual PxU32 PxCloth::getNbVirtualParticles | ( | ) | const [pure virtual] |
Returns the number of virtual particles.
- Returns:
- Number of virtual particles.
virtual PxU32 PxCloth::getNbVirtualParticleWeights | ( | ) | const [pure virtual] |
Returns the number of the virtual particle weights.
- Returns:
- Number of virtual particle weights.
virtual bool PxCloth::getParticleAccelerations | ( | PxVec4 * | particleAccelerationsBuffer | ) | const [pure virtual] |
Copies particle accelerations to the user provided buffer.
- Parameters:
-
[out] particleAccelerationsBuffer Destination buffer, must be at least getNbParticleAccelerations().
- Returns:
- true if the copy was successful.
virtual PxClothPhaseSolverConfig PxCloth::getPhaseSolverConfig | ( | PxClothFabricPhaseType::Enum | phaseType | ) | const [pure virtual] |
Reads solver configuration for specified phase type.
- Parameters:
-
[in] phaseType Which phase to return the configuration for.
- Returns:
- solver configuration (see PxClothPhaseSolverConfig)
- Note:
- If phaseType is invalid, the returned solver configuration's solverType member will become eINVALID.
virtual PxReal PxCloth::getPreviousTimeStep | ( | ) | const [pure virtual] |
Returns previous time step size.
Time between sampling of previous and current particle positions for computing particle velocity.
- Returns:
- Previous time step size.
virtual bool PxCloth::getSeparationConstraints | ( | PxClothParticleSeparationConstraint * | separationConstraintBuffer | ) | const [pure virtual] |
Copies separation constraints to the user provided buffer.
- Parameters:
-
[out] separationConstraintBuffer Destination buffer, must be at least getNbSeparationConstraints().
- Returns:
- True if the copy was successful.
virtual PxReal PxCloth::getSleepLinearVelocity | ( | ) | const [pure virtual] |
Returns the velocity threshold for putting cloth in sleep state.
- Returns:
- Velocity threshold for putting cloth in sleep state.
virtual PxReal PxCloth::getSolverFrequency | ( | ) | const [pure virtual] |
Returns solver frequency.
- Returns:
- Solver frequency.
virtual void PxCloth::getVirtualParticles | ( | PxU32 * | userTriangleVertexAndWeightIndices | ) | const [pure virtual] |
Copies index array of virtual particles to the user provided buffer.
- Parameters:
-
[out] userTriangleVertexAndWeightIndices Destination buffer, must be at least 4*getNbVirtualParticles().
- See also:
- setVirtualParticles()
virtual void PxCloth::getVirtualParticleWeights | ( | PxVec3 * | userTriangleVertexWeightTable | ) | const [pure virtual] |
Copies weight table of virtual particles to the user provided buffer.
- Parameters:
-
[out] userTriangleVertexWeightTable Destination buffer, must be at least getNbVirtualParticleWeights().
virtual PxBounds3 PxCloth::getWorldBounds | ( | ) | const [pure virtual] |
Returns world space bounding box.
- Returns:
- Particle bounds in global coordinates.
Implements PxActor.
virtual bool PxCloth::isKindOf | ( | const char * | name | ) | const [inline, protected, virtual] |
virtual bool PxCloth::isSleeping | ( | ) | const [pure virtual] |
Returns true if cloth is in sleep state.
- Returns:
- True if cloth is in sleep state.
virtual PxClothReadData* PxCloth::lockClothReadData | ( | ) | const [pure virtual] |
Locks the cloth solver so that external applications can safely read back particle data.
- Returns:
- See PxClothReadData for available user data for read back.
virtual void PxCloth::putToSleep | ( | ) | [pure virtual] |
Forces cloth to be put in sleep state.
virtual void PxCloth::release | ( | ) | [pure virtual] |
Deletes the actor.
Do not keep a reference to the deleted instance.
Sleeping: If this is a PxRigidDynamic, this call will awaken any sleeping actors contacting the deleted actor (directly or indirectly).
If the actor belongs to a PxAggregate object, it is automatically removed from the aggregate.
- See also:
- PxScene::createRigidStatic(), PxScene::createRigidDynamic(), PxScene::createParticleSystem(), PxScene::createParticleFluid(), PxAggregate
Implements PxActor.
virtual void PxCloth::removeCollisionConvex | ( | PxU32 | index | ) | [pure virtual] |
Removes a collision convex.
- Parameters:
-
[in] index Index of convex to remove.
- Note:
- The indices of convexes added after
index
are decremented by 1.Planes referenced by this convex will not be removed.
virtual void PxCloth::removeCollisionPlane | ( | PxU32 | index | ) | [pure virtual] |
Removes a collision plane.
- Parameters:
-
[in] index Index of plane to remove.
- Note:
- The indices of planes added after
index
are decremented by 1.Convexes that reference the plane will have the plane removed from their mask. If after removal a convex consists of zero planes, it will also be removed.
virtual void PxCloth::setClothFlag | ( | PxClothFlag::Enum | flag, | |
bool | value | |||
) | [pure virtual] |
Sets cloth flags (e.g. use GPU or not, use CCD or not).
- Parameters:
-
[in] flag Mask of which flags to set. [in] value Value to set flags to.
virtual void PxCloth::setCollisionMassScale | ( | PxReal | scalingCoefficient | ) | [pure virtual] |
Sets the collision mass scaling coefficient.
During collision it is possible to artificially increase the mass of a colliding particle, this has an effect comparable to making constraints attached to the particle stiffer and can help reduce stretching and interpenetration around collision shapes.
- Parameters:
-
[in] scalingCoefficient Unitless multiplier that can take on values > 1 (default: 0.0).
virtual void PxCloth::setCollisionPlanes | ( | const PxClothCollisionPlane * | planesBuffer | ) | [pure virtual] |
Updates positions of collision planes.
- Parameters:
-
[in] planesBuffer New plane positions by the end of the next simulate() call.
- See also:
- clearInterpolation()
virtual void PxCloth::setCollisionSpheres | ( | const PxClothCollisionSphere * | sphereBuffer | ) | [pure virtual] |
Updates location and radii of collision spheres.
- Parameters:
-
[in] sphereBuffer New sphere positions and radii by the end of the next simulate() call.
- Note:
- A maximum of 32 spheres are supported.
- See also:
- clearInterpolation()
virtual void PxCloth::setDampingCoefficient | ( | PxReal | dampingCoefficient | ) | [pure virtual] |
Sets the damping coefficient.
The damping coefficient is the portion of local particle velocity that is canceled in 1/10 sec.
- Parameters:
-
[in] dampingCoefficient New damping coefficient between 0.0 and 1.0 (default: 0.0).
virtual void PxCloth::setDragCoefficient | ( | PxReal | dragCoefficient | ) | [pure virtual] |
Sets the drag coefficient.
The drag coefficient is the portion of the pose velocity that is applied to each particle in 1/10 sec.
- Parameters:
-
[in] dragCoefficient New drag coefficient between 0.0f and 1.0 (default: 0.0).
- Note:
- The drag coefficient shouldn't be set higher than the damping coefficient.
virtual void PxCloth::setExternalAcceleration | ( | PxVec3 | acceleration | ) | [pure virtual] |
Sets external particle accelerations.
- Parameters:
-
[in] acceleration New acceleration in global coordinates (default: 0.0).
- Note:
- Use this to implement simple wind etc.
virtual void PxCloth::setFrictionCoefficient | ( | PxReal | frictionCoefficient | ) | [pure virtual] |
Sets the collision friction coefficient.
- Parameters:
-
[in] frictionCoefficient New friction coefficient between 0.0 and 1.0 (default: 0.0).
- Note:
- Currently only spheres and capsules impose friction on the colliding particles.
virtual void PxCloth::setGlobalPose | ( | const PxTransform & | pose | ) | [pure virtual] |
Sets global pose.
Use this to reset the pose (e.g. teleporting).
- Parameters:
-
[in] pose New global pose.
- Note:
- No pose interpolation is performed.
Inertia is not preserved.
- See also:
- setTargetPose() for inertia preserving method.
virtual void PxCloth::setInertiaScale | ( | PxReal | scale | ) | [pure virtual] |
Sets the acceleration scale factor to adjust inertia effect from global pose changes.
- Parameters:
-
[in] scale New scale factor between 0.0 (no inertia) and 1.0 (full inertia) (default: 1.0).
- Note:
- A value of 0.0 disables all inertia effects of accelerations applied through setTargetPos().
- See also:
- setTargetPose()
virtual void PxCloth::setMotionConstraints | ( | const PxClothParticleMotionConstraint * | motionConstraints | ) | [pure virtual] |
Updates motion constraints (position and radius of the constraint sphere).
- Parameters:
-
[in] motionConstraints motion constraints at the end of the next simulate() call.
- Note:
- The motionConstraints must either be null to disable motion constraints, or be the same length as the number of particles, see getNbParticles().
- See also:
- clearInterpolation()
Specifies motion constraint scale and bias.
- Parameters:
-
[in] scale Global scale multiplied to the radius of every motion constraint sphere (default = 1.0). [in] bias Global bias added to the radius of every motion constraint sphere (default = 0.0).
virtual void PxCloth::setParticleAccelerations | ( | const PxVec4 * | particleAccelerations | ) | [pure virtual] |
Updates particle accelerations, w component is ignored.
- Parameters:
-
[in] particleAccelerations New particle accelerations.
- Note:
- The particleAccelerations must either be null to disable accelerations, or be the same length as the number of particles, see getNbParticles().
virtual void PxCloth::setParticles | ( | const PxClothParticle * | currentParticles, | |
const PxClothParticle * | previousParticles | |||
) | [pure virtual] |
Updates cloth particle location or inverse weight for current and previous particle state.
- Parameters:
-
[in] currentParticles The particle data for the current particle state or NULL if the state should not be changed. [in] previousParticles The particle data for the previous particle state or NULL if the state should not be changed.
- Note:
- The invWeight stored in currentParticles is the new particle inverse mass, or zero for a static particle. However, the invWeight stored in previousParticles is still used once for the next particle integration and fabric solve.
If currentParticles or previousParticles are non-NULL then they must be the length specified by getNbParticles().
This can be used to teleport particles (use same positions for current and previous).
- See also:
- PxClothParticle
virtual void PxCloth::setPhaseSolverConfig | ( | PxClothFabricPhaseType::Enum | phaseType, | |
const PxClothPhaseSolverConfig & | config | |||
) | [pure virtual] |
Sets solver configuration per phase type.
Users can assign different solver configuration (solver type, stiffness, etc.) per each phase type (see PxClothFabricPhaseType).
- Parameters:
-
[in] phaseType Which phases to change the configuration for. [in] config What configuration to change it to.
virtual void PxCloth::setSeparationConstraints | ( | const PxClothParticleSeparationConstraint * | separationConstraints | ) | [pure virtual] |
Updates separation constraints (position and radius of the constraint sphere).
- Parameters:
-
[in] separationConstraints separation constraints at the end of the next simulate() call.
- Note:
- The separationConstraints must either be null to disable separation constraints, or be the same length as the number of particles, see getNbParticles().
- See also:
- clearInterpolation()
virtual void PxCloth::setSleepLinearVelocity | ( | PxReal | threshold | ) | [pure virtual] |
Sets the velocity threshold for putting cloth in sleep state.
If none of the particles moves faster (in local space) than the threshold for a while, the cloth will be put in sleep state and simulation will be skipped.
- Parameters:
-
[in] threshold Velocity threshold (default: 0.0f)
virtual void PxCloth::setSolverFrequency | ( | PxReal | frequency | ) | [pure virtual] |
Sets the solver frequency parameter.
Solver frequency specifies how often the simulation step is computed per second. For example, a value of 60 represents one simulation step per frame in a 60fps scene. A value of 120 will represent two simulation steps per frame, etc.
- Parameters:
-
[in] frequency Solver frequency per second.
virtual void PxCloth::setTargetPose | ( | const PxTransform & | pose | ) | [pure virtual] |
Sets target pose.
This function will move the cloth in world space. The resulting simulation may reflect inertia effect as a result of pose acceleration.
- Parameters:
-
[in] pose Target pose at the end of the next simulate() call.
- See also:
- setGlobalPose() to move Cloth without inertia effect.
virtual void PxCloth::setVirtualParticles | ( | PxU32 | numParticles, | |
const PxU32 * | triangleVertexAndWeightIndices, | |||
PxU32 | weightTableSize, | |||
const PxVec3 * | triangleVertexWeightTable | |||
) | [pure virtual] |
Assigns virtual particles.
Virtual particles provide more robust and accurate collision handling against collision spheres and capsules. More virtual particles will generally increase the accuracy of collision handling, and thus a sufficient number of virtual particles can mimic triangle-based collision handling.
Virtual particles are specified as barycentric interpolation of real particles: The position of a virtual particle is w0 * P0 + w1 * P1 + w2 * P2, where P1, P2, P3 real particle positions. The barycentric weights w0, w1, w2 are stored in a separate table so they can be shared across multiple virtual particles.
- Parameters:
-
[in] numParticles total number of virtual particles. [in] triangleVertexAndWeightIndices Each virtual particle has four indices, the first three for real particle indices, and the last for the weight table index. Thus, the length of indices needs to be 4*numVirtualParticles. [in] weightTableSize total number of unique weights triples. [in] triangleVertexWeightTable array for barycentric weights.
- Note:
- Virtual particles only incur a runtime cost during the collision stage. Still, it is advisable to only use virtual particles for areas where high collision accuracy is desired. (e.g. sleeve around very thin wrist).
virtual void PxCloth::wakeUp | ( | PxReal | wakeCounterValue = PX_SLEEP_INTERVAL |
) | [pure virtual] |
Forces cloth to wake up from sleep state.
The wakeCounterValue determines how long all particles need to move less than the velocity threshold until the cloth is put to sleep.
- Parameters:
-
[in] wakeCounterValue New wake counter value (range: [0, inf]).
The documentation for this class was generated from the following file:
Copyright © 2008-2012 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com