PxClothFabric Class Reference
[Cloth]
A cloth fabric is a structure that contains all the internal solver constraints of a clothing mesh.
More...
#include <PxClothFabric.h>
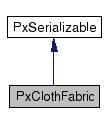
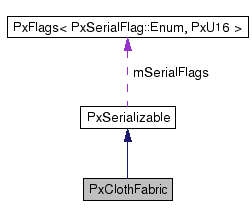
Public Member Functions | |
virtual void | release ()=0 |
Release the cloth fabric. | |
virtual PxU32 | getNbParticles () const =0 |
Returns number of particles. | |
virtual PxU32 | getNbPhases () const =0 |
Returns number of phases. | |
virtual PxU32 | getNbRestvalues () const =0 |
Returns number of rest values. | |
virtual PxU32 | getNbSets () const =0 |
Returns number of sets. | |
virtual PxU32 | getNbFibers () const =0 |
Returns number of fibers. | |
virtual PxU32 | getNbParticleIndices () const =0 |
Get number of particle indices. | |
virtual PxU32 | getPhases (PxU32 *userPhaseBuffer, PxU32 bufferSize) const =0 |
Copies the phase array to a user specified buffer. | |
virtual PxU32 | getSets (PxU32 *userSetBuffer, PxU32 bufferSize) const =0 |
Copies the set array to a user specified buffer. | |
virtual PxU32 | getFibers (PxU32 *userFiberBuffer, PxU32 bufferSize) const =0 |
Copies the fibers array to a user specified buffer. | |
virtual PxU32 | getParticleIndices (PxU32 *userParticleIndexBuffer, PxU32 bufferSize) const =0 |
Copies the particle indices array to a user specified buffer. | |
virtual PxU32 | getRestvalues (PxReal *userRestvalueBuffer, PxU32 bufferSize) const =0 |
Copies the rest values array to a user specified buffer. | |
virtual PxClothFabricPhaseType::Enum | getPhaseType (PxU32 phaseIndex) const =0 |
Returns the type of a phase. | |
virtual void | scaleRestvalues (PxClothFabricPhaseType::Enum phaseType, PxReal scale)=0 |
Scale all rest values of a phase type. | |
virtual PxU32 | getReferenceCount () const =0 |
Reference count of the cloth instance. | |
virtual const char * | getConcreteTypeName () const |
returns string name of dynamic type. | |
Protected Member Functions | |
PxClothFabric () | |
PxClothFabric (PxRefResolver &v) | |
virtual | ~PxClothFabric () |
virtual bool | isKindOf (const char *name) const |
Detailed Description
A cloth fabric is a structure that contains all the internal solver constraints of a clothing mesh.
A fabric consists of phases
that represent a group of internal constraints of the same type. Each phase references an array of rest-values
and a set
of particle indices, grouped into fibers
. A fiber is a linear array of particle indices that are connected by constraints, and fibers of a set are guaranteed to be disconnected so they can be solved in parallel.
The data representation for the fabric has layers of indirect indices:
- All particle indices of the fabric are stored in one linear array and reference by the fibers.
- The fiber array holds the prefix sum of the number of indices per fiber and is referenced by the sets.
- The set array holds the prefix sum of the number of fibers per set and is referenced by the phases.
- A phase consists of the type of constraints, and the index of the set referencing fibers and indices.
- The rest-value are packed in a linear array in the order they are referenced by the phases.
phase types: [ eVERTICAL, eBENDING] phases: [ 0, 1] sets: [ 3, 5] |----------- set 0 -----------|------ set 1 ------| fibers: [ 3, 5, 7, 10, 13] | fiber 0 | fiber 1 | fiber 2 | fiber 3 | fiber 4 | indices: [2, 0, 3, 6, 4, 5, 1, 4, 2, 0, 1, 3, 6] restvalues: [2.0, 2.0, 2.0 , 2.0 , 1.0 , 1.0 ]
- See also:
- The fabric structure can be created from a mesh using PxCooking.cookClothFabric() and saved to a PxStream. Instances of PxClothFabric can then be created from the stream using PxPhysics.createClothFabric().
Constructor & Destructor Documentation
PxClothFabric::PxClothFabric | ( | ) | [inline, protected] |
PxClothFabric::PxClothFabric | ( | PxRefResolver & | v | ) | [inline, protected] |
virtual PxClothFabric::~PxClothFabric | ( | ) | [inline, protected, virtual] |
Member Function Documentation
virtual const char* PxClothFabric::getConcreteTypeName | ( | ) | const [inline, virtual] |
returns string name of dynamic type.
- Returns:
- class name of most derived type of this object.
Reimplemented from PxSerializable.
virtual PxU32 PxClothFabric::getFibers | ( | PxU32 * | userFiberBuffer, | |
PxU32 | bufferSize | |||
) | const [pure virtual] |
Copies the fibers array to a user specified buffer.
The fibers array is the inclusive prefix sum of the number of particle indices per set. It has the same length as getNbFibers().
- Parameters:
-
[in] userFiberBuffer Destination buffer to copy the set data to. [in] bufferSize Size of userFiberBuffer, should be at least getNbFibers().
- Returns:
- getNbFibers() if the copy was successful, 0 otherwise.
- Note:
- Particle indices of the i-th fiber are stored at indices [i?fiber[i-1]:0, fiber[i]) in the particle indices array.
virtual PxU32 PxClothFabric::getNbFibers | ( | ) | const [pure virtual] |
Returns number of fibers.
- Returns:
- The size of the fiber array.
virtual PxU32 PxClothFabric::getNbParticleIndices | ( | ) | const [pure virtual] |
Get number of particle indices.
- Returns:
- The size of the particle indices array.
virtual PxU32 PxClothFabric::getNbParticles | ( | ) | const [pure virtual] |
Returns number of particles.
- Returns:
- The number of particles needed when creating a PxCloth instance from the fabric.
virtual PxU32 PxClothFabric::getNbPhases | ( | ) | const [pure virtual] |
Returns number of phases.
- Returns:
- The number of solver phases.
virtual PxU32 PxClothFabric::getNbRestvalues | ( | ) | const [pure virtual] |
Returns number of rest values.
- Returns:
- The size of the rest values array.
virtual PxU32 PxClothFabric::getNbSets | ( | ) | const [pure virtual] |
Returns number of sets.
- Returns:
- The size of the set array.
virtual PxU32 PxClothFabric::getParticleIndices | ( | PxU32 * | userParticleIndexBuffer, | |
PxU32 | bufferSize | |||
) | const [pure virtual] |
Copies the particle indices array to a user specified buffer.
The particle indices array determines which particles are affected by each constraint. It has the same length as getNbParticleIndices().
- Parameters:
-
[in] userParticleIndexBuffer Destination buffer to copy the set data to. [in] bufferSize Size of userParticleIndexBuffer, should be at least getNbParticleIndices().
- Returns:
- getNbParticleIndices() if the copy was successful, 0 otherwise.
virtual PxU32 PxClothFabric::getPhases | ( | PxU32 * | userPhaseBuffer, | |
PxU32 | bufferSize | |||
) | const [pure virtual] |
Copies the phase array to a user specified buffer.
The phase array is a mapping of the phase index to the corresponding set index. A set index can occur multiple times. The array has the same length as getNbPhases().
- Parameters:
-
[in] userPhaseBuffer Destination buffer to copy the phase data to. [in] bufferSize Size of userPhaseBuffer, should be at least getNbPhases().
- Returns:
- getNbPhases() if the copy was successful, 0 otherwise.
virtual PxClothFabricPhaseType::Enum PxClothFabric::getPhaseType | ( | PxU32 | phaseIndex | ) | const [pure virtual] |
Returns the type of a phase.
- Parameters:
-
[in] phaseIndex The index of the phase to return the type for.
- Returns:
- The phase type as PxClothFabricPhaseType::Enum.
- Note:
- If phase index is invalid, PxClothFabricPhaseType::eINVALID is returned.
virtual PxU32 PxClothFabric::getReferenceCount | ( | ) | const [pure virtual] |
Reference count of the cloth instance.
At creation, the reference count of the fabric is 1. Every cloth instance referencing this fabric increments the count by 1. When the reference count reaches 0, and only then, the fabric gets released automatically.
- See also:
- PxCloth
virtual PxU32 PxClothFabric::getRestvalues | ( | PxReal * | userRestvalueBuffer, | |
PxU32 | bufferSize | |||
) | const [pure virtual] |
Copies the rest values array to a user specified buffer.
The rest values array holds the target value of the constraint in rest state, for example edge length for stretch constraints or bending angle for bending constraints. It has the same length as getNbRestvalues(). The rest-values are stored in the order they are (indirectly) referenced by the phases. There is one less rest value than particle indices in a stretch fiber, and two less in a bending fiber.
- Parameters:
-
[in] userRestvalueBuffer Destination buffer to copy the set data to. [in] bufferSize Size of userRestvalueBuffer, should be at least getNbRestvalues().
- Returns:
- getNbRestvalues() if the copy was successful, 0 otherwise.
virtual PxU32 PxClothFabric::getSets | ( | PxU32 * | userSetBuffer, | |
PxU32 | bufferSize | |||
) | const [pure virtual] |
Copies the set array to a user specified buffer.
The set array is the inclusive prefix sum of the number of fibers per set. It has the same length as getNbSets().
- Parameters:
-
[in] userSetBuffer Destination buffer to copy the set data to. [in] bufferSize Size of userSetBuffer, should be at least getNbSets().
- Returns:
- getNbSets() if the copy was successful, 0 otherwise.
- Note:
- Fibers of the i-th set are stored at indices [i?set[i-1]:0, set[i]) in the fibers array.
virtual bool PxClothFabric::isKindOf | ( | const char * | name | ) | const [inline, protected, virtual] |
virtual void PxClothFabric::release | ( | ) | [pure virtual] |
Release the cloth fabric.
Releases the application's reference to the cloth fabric. The fabric is destroyed when the application's reference is released and all cloth instances referencing the fabric are destroyed.
- See also:
- PxPhysics.createClothFabric()
virtual void PxClothFabric::scaleRestvalues | ( | PxClothFabricPhaseType::Enum | phaseType, | |
PxReal | scale | |||
) | [pure virtual] |
Scale all rest values of a phase type.
- Parameters:
-
[in] phaseType Type of phases to scale rest values for. [in] scale The scale factor to multiply each rest value with.
- Note:
- Only call this function when no PxCloth instance has been created for this fabric yet.
Scaling rest values of type PxClothFabricPhaseType::eBENDING results in undefined behavior.
The documentation for this class was generated from the following file:
Copyright © 2008-2012 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com