E:/p4/sw/physx/PxShared/1.0/trunk/src/foundation/include/PsThread.h
Go to the documentation of this file.00001 // This code contains NVIDIA Confidential Information and is disclosed to you 00002 // under a form of NVIDIA software license agreement provided separately to you. 00003 // 00004 // Notice 00005 // NVIDIA Corporation and its licensors retain all intellectual property and 00006 // proprietary rights in and to this software and related documentation and 00007 // any modifications thereto. Any use, reproduction, disclosure, or 00008 // distribution of this software and related documentation without an express 00009 // license agreement from NVIDIA Corporation is strictly prohibited. 00010 // 00011 // ALL NVIDIA DESIGN SPECIFICATIONS, CODE ARE PROVIDED "AS IS.". NVIDIA MAKES 00012 // NO WARRANTIES, EXPRESSED, IMPLIED, STATUTORY, OR OTHERWISE WITH RESPECT TO 00013 // THE MATERIALS, AND EXPRESSLY DISCLAIMS ALL IMPLIED WARRANTIES OF NONINFRINGEMENT, 00014 // MERCHANTABILITY, AND FITNESS FOR A PARTICULAR PURPOSE. 00015 // 00016 // Information and code furnished is believed to be accurate and reliable. 00017 // However, NVIDIA Corporation assumes no responsibility for the consequences of use of such 00018 // information or for any infringement of patents or other rights of third parties that may 00019 // result from its use. No license is granted by implication or otherwise under any patent 00020 // or patent rights of NVIDIA Corporation. Details are subject to change without notice. 00021 // This code supersedes and replaces all information previously supplied. 00022 // NVIDIA Corporation products are not authorized for use as critical 00023 // components in life support devices or systems without express written approval of 00024 // NVIDIA Corporation. 00025 // 00026 // Copyright (c) 2008-2014 NVIDIA Corporation. All rights reserved. 00027 // Copyright (c) 2004-2008 AGEIA Technologies, Inc. All rights reserved. 00028 // Copyright (c) 2001-2004 NovodeX AG. All rights reserved. 00029 00030 #ifndef PSFOUNDATION_PSTHREAD_H 00031 #define PSFOUNDATION_PSTHREAD_H 00032 00033 #include "PsUserAllocated.h" 00034 00035 // dsequeira: according to existing comment here (David Black would be my guess) 00036 // "This is useful to reduce bus contention on tight spin locks. And it needs 00037 // to be a macro as the xenon compiler often ignores even __forceinline." What's not 00038 // clear is why a pause function needs inlining...? (TODO: check with XBox team) 00039 00040 // todo: these need to go somewhere else 00041 00042 #if PX_WINDOWS_FAMILY || PX_WINRT || PX_XBOXONE 00043 #define PxSpinLockPause() __asm pause 00044 #if PX_WINRT 00045 #define PX_TLS_MAX_SLOTS 64 00046 #endif 00047 #elif PX_LINUX || PX_ANDROID || PX_PS4 || PX_APPLE_FAMILY 00048 #define PxSpinLockPause() asm("nop") 00049 #else 00050 #error "Platform not supported!" 00051 #endif 00052 00053 namespace physx 00054 { 00055 namespace shdfnd 00056 { 00057 struct ThreadPriority // todo: put in some other header file 00058 { 00059 enum Enum 00060 { 00064 eHIGH = 0, 00065 00069 eABOVE_NORMAL = 1, 00070 00074 eNORMAL = 2, 00075 00079 eBELOW_NORMAL = 3, 00080 00084 eLOW = 4, 00085 eFORCE_DWORD = 0xffFFffFF 00086 }; 00087 }; 00088 00089 class Runnable 00090 { 00091 public: 00092 Runnable() 00093 { 00094 } 00095 virtual ~Runnable() 00096 { 00097 } 00098 virtual void execute(void) 00099 { 00100 } 00101 }; 00102 00103 class PX_FOUNDATION_API ThreadImpl 00104 { 00105 public: 00106 typedef size_t Id; // space for a pointer or an integer 00107 typedef void* (*ExecuteFn)(void*); 00108 00109 static uint32_t getDefaultStackSize(); 00110 static Id getId(); 00111 00118 ThreadImpl(); 00119 00124 ThreadImpl(ExecuteFn fn, void* arg); 00125 00131 ~ThreadImpl(); 00132 00139 void start(uint32_t stackSize, Runnable* r); 00140 00147 void kill(); 00148 00154 void signalQuit(); 00155 00161 bool waitForQuit(); 00162 00168 bool quitIsSignalled(); 00169 00173 void quit(); 00174 00192 uint32_t setAffinityMask(uint32_t mask); 00193 00194 static ThreadPriority::Enum getPriority(Id threadId); 00195 00197 void setPriority(ThreadPriority::Enum prio); 00198 00200 void setName(const char* name); 00201 00203 static void sleep(uint32_t ms); 00204 00206 static void yield(); 00207 00209 static uint32_t getNbPhysicalCores(); 00210 00214 static const uint32_t& getSize(); 00215 }; 00216 00220 template <typename Alloc = ReflectionAllocator<ThreadImpl> > 00221 class ThreadT : protected Alloc, public UserAllocated, public Runnable 00222 { 00223 public: 00224 typedef ThreadImpl::Id Id; // space for a pointer or an integer 00225 00230 ThreadT(const Alloc& alloc = Alloc()) : Alloc(alloc) 00231 { 00232 mImpl = reinterpret_cast<ThreadImpl*>(Alloc::allocate(ThreadImpl::getSize(), __FILE__, __LINE__)); 00233 PX_PLACEMENT_NEW(mImpl, ThreadImpl)(); 00234 } 00235 00239 ThreadT(ThreadImpl::ExecuteFn fn, void* arg, const Alloc& alloc = Alloc()) : Alloc(alloc) 00240 { 00241 mImpl = reinterpret_cast<ThreadImpl*>(Alloc::allocate(ThreadImpl::getSize(), __FILE__, __LINE__)); 00242 PX_PLACEMENT_NEW(mImpl, ThreadImpl)(fn, arg); 00243 } 00244 00249 virtual ~ThreadT() 00250 { 00251 mImpl->~ThreadImpl(); 00252 Alloc::deallocate(mImpl); 00253 } 00254 00259 void start(uint32_t stackSize = ThreadImpl::getDefaultStackSize()) 00260 { 00261 mImpl->start(stackSize, this); 00262 } 00263 00270 void kill() 00271 { 00272 mImpl->kill(); 00273 } 00274 00280 virtual void execute(void) 00281 { 00282 } 00283 00289 void signalQuit() 00290 { 00291 mImpl->signalQuit(); 00292 } 00293 00299 bool waitForQuit() 00300 { 00301 return mImpl->waitForQuit(); 00302 } 00303 00309 bool quitIsSignalled() 00310 { 00311 return mImpl->quitIsSignalled(); 00312 } 00313 00317 void quit() 00318 { 00319 mImpl->quit(); 00320 } 00321 00322 uint32_t setAffinityMask(uint32_t mask) 00323 { 00324 return mImpl->setAffinityMask(mask); 00325 } 00326 00327 static ThreadPriority::Enum getPriority(ThreadImpl::Id threadId) 00328 { 00329 return ThreadImpl::getPriority(threadId); 00330 } 00331 00333 void setPriority(ThreadPriority::Enum prio) 00334 { 00335 mImpl->setPriority(prio); 00336 } 00337 00339 void setName(const char* name) 00340 { 00341 mImpl->setName(name); 00342 } 00343 00345 static void sleep(uint32_t ms) 00346 { 00347 ThreadImpl::sleep(ms); 00348 } 00349 00351 static void yield() 00352 { 00353 ThreadImpl::yield(); 00354 } 00355 00356 static uint32_t getDefaultStackSize() 00357 { 00358 return ThreadImpl::getDefaultStackSize(); 00359 } 00360 00361 static ThreadImpl::Id getId() 00362 { 00363 return ThreadImpl::getId(); 00364 } 00365 00366 static uint32_t getNbPhysicalCores() 00367 { 00368 return ThreadImpl::getNbPhysicalCores(); 00369 } 00370 00371 private: 00372 class ThreadImpl* mImpl; 00373 }; 00374 00375 typedef ThreadT<> Thread; 00376 00377 PX_FOUNDATION_API uint32_t TlsAlloc(); 00378 PX_FOUNDATION_API void TlsFree(uint32_t index); 00379 PX_FOUNDATION_API void* TlsGet(uint32_t index); 00380 PX_FOUNDATION_API uint32_t TlsSet(uint32_t index, void* value); 00381 00382 } // namespace shdfnd 00383 } // namespace physx 00384 00385 #endif // #ifndef PSFOUNDATION_PSTHREAD_H
Generated on Tue Jul 28 14:21:55 2015 for NVIDIA(R) PsFoundation Reference by
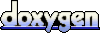