E:/p4/sw/physx/PxShared/1.0/trunk/src/foundation/include/PsString.h
Go to the documentation of this file.00001 // This code contains NVIDIA Confidential Information and is disclosed to you 00002 // under a form of NVIDIA software license agreement provided separately to you. 00003 // 00004 // Notice 00005 // NVIDIA Corporation and its licensors retain all intellectual property and 00006 // proprietary rights in and to this software and related documentation and 00007 // any modifications thereto. Any use, reproduction, disclosure, or 00008 // distribution of this software and related documentation without an express 00009 // license agreement from NVIDIA Corporation is strictly prohibited. 00010 // 00011 // ALL NVIDIA DESIGN SPECIFICATIONS, CODE ARE PROVIDED "AS IS.". NVIDIA MAKES 00012 // NO WARRANTIES, EXPRESSED, IMPLIED, STATUTORY, OR OTHERWISE WITH RESPECT TO 00013 // THE MATERIALS, AND EXPRESSLY DISCLAIMS ALL IMPLIED WARRANTIES OF NONINFRINGEMENT, 00014 // MERCHANTABILITY, AND FITNESS FOR A PARTICULAR PURPOSE. 00015 // 00016 // Information and code furnished is believed to be accurate and reliable. 00017 // However, NVIDIA Corporation assumes no responsibility for the consequences of use of such 00018 // information or for any infringement of patents or other rights of third parties that may 00019 // result from its use. No license is granted by implication or otherwise under any patent 00020 // or patent rights of NVIDIA Corporation. Details are subject to change without notice. 00021 // This code supersedes and replaces all information previously supplied. 00022 // NVIDIA Corporation products are not authorized for use as critical 00023 // components in life support devices or systems without express written approval of 00024 // NVIDIA Corporation. 00025 // 00026 // Copyright (c) 2008-2014 NVIDIA Corporation. All rights reserved. 00027 // Copyright (c) 2004-2008 AGEIA Technologies, Inc. All rights reserved. 00028 // Copyright (c) 2001-2004 NovodeX AG. All rights reserved. 00029 00030 #ifndef PSFOUNDATION_PSSTRING_H 00031 #define PSFOUNDATION_PSSTRING_H 00032 00033 #include "foundation/PxPreprocessor.h" 00034 #include "foundation/PxSimpleTypes.h" 00035 #include <stdarg.h> 00036 00037 namespace physx 00038 { 00039 namespace shdfnd 00040 { 00041 00042 // the following functions have C99 semantics. Note that C99 requires for snprintf and vsnprintf: 00043 // * the resulting string is always NULL-terminated regardless of truncation. 00044 // * in the case of truncation the return value is the number of characters that would have been created. 00045 00046 PX_FOUNDATION_API int32_t sscanf(const char* buffer, const char* format, ...); 00047 PX_FOUNDATION_API int32_t strcmp(const char* str1, const char* str2); 00048 PX_FOUNDATION_API int32_t strncmp(const char* str1, const char* str2, size_t count); 00049 PX_FOUNDATION_API int32_t snprintf(char* dst, size_t dstSize, const char* format, ...); 00050 PX_FOUNDATION_API int32_t vsnprintf(char* dst, size_t dstSize, const char* src, va_list arg); 00051 00052 // strlcat and strlcpy have BSD semantics: 00053 // * dstSize is always the size of the destination buffer 00054 // * the resulting string is always NULL-terminated regardless of truncation 00055 // * in the case of truncation the return value is the length of the string that would have been created 00056 00057 PX_FOUNDATION_API size_t strlcat(char* dst, size_t dstSize, const char* src); 00058 PX_FOUNDATION_API size_t strlcpy(char* dst, size_t dstSize, const char* src); 00059 00060 // case-insensitive string comparison 00061 PX_FOUNDATION_API int32_t stricmp(const char* str1, const char* str2); 00062 PX_FOUNDATION_API int32_t strnicmp(const char* str1, const char* str2, size_t count); 00063 00064 // in-place string case conversion 00065 PX_FOUNDATION_API void strlwr(char* str); 00066 PX_FOUNDATION_API void strupr(char* str); 00067 00074 static const size_t MAX_PRINTFORMATTED_LENGTH = 1024; 00075 00081 PX_FOUNDATION_API void printFormatted(const char*, ...); 00082 00087 PX_FOUNDATION_API void printString(const char*); 00088 } 00089 } 00090 #endif // #ifndef PSFOUNDATION_PSSTRING_H
Generated on Tue Jul 28 14:21:55 2015 for NVIDIA(R) PsFoundation Reference by
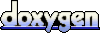