E:/p4/sw/physx/PxShared/1.0/trunk/src/foundation/include/PsMutex.h
Go to the documentation of this file.00001 // This code contains NVIDIA Confidential Information and is disclosed to you 00002 // under a form of NVIDIA software license agreement provided separately to you. 00003 // 00004 // Notice 00005 // NVIDIA Corporation and its licensors retain all intellectual property and 00006 // proprietary rights in and to this software and related documentation and 00007 // any modifications thereto. Any use, reproduction, disclosure, or 00008 // distribution of this software and related documentation without an express 00009 // license agreement from NVIDIA Corporation is strictly prohibited. 00010 // 00011 // ALL NVIDIA DESIGN SPECIFICATIONS, CODE ARE PROVIDED "AS IS.". NVIDIA MAKES 00012 // NO WARRANTIES, EXPRESSED, IMPLIED, STATUTORY, OR OTHERWISE WITH RESPECT TO 00013 // THE MATERIALS, AND EXPRESSLY DISCLAIMS ALL IMPLIED WARRANTIES OF NONINFRINGEMENT, 00014 // MERCHANTABILITY, AND FITNESS FOR A PARTICULAR PURPOSE. 00015 // 00016 // Information and code furnished is believed to be accurate and reliable. 00017 // However, NVIDIA Corporation assumes no responsibility for the consequences of use of such 00018 // information or for any infringement of patents or other rights of third parties that may 00019 // result from its use. No license is granted by implication or otherwise under any patent 00020 // or patent rights of NVIDIA Corporation. Details are subject to change without notice. 00021 // This code supersedes and replaces all information previously supplied. 00022 // NVIDIA Corporation products are not authorized for use as critical 00023 // components in life support devices or systems without express written approval of 00024 // NVIDIA Corporation. 00025 // 00026 // Copyright (c) 2008-2014 NVIDIA Corporation. All rights reserved. 00027 // Copyright (c) 2004-2008 AGEIA Technologies, Inc. All rights reserved. 00028 // Copyright (c) 2001-2004 NovodeX AG. All rights reserved. 00029 00030 #ifndef PSFOUNDATION_PSMUTEX_H 00031 #define PSFOUNDATION_PSMUTEX_H 00032 00033 #include "PsAllocator.h" 00034 00035 /* 00036 * This <new> inclusion is a best known fix for gcc 4.4.1 error: 00037 * Creating object file for apex/src/PsAllocator.cpp ... 00038 * In file included from apex/include/PsFoundation.h:30, 00039 * from apex/src/PsAllocator.cpp:26: 00040 * apex/include/PsMutex.h: In constructor 'physx::shdfnd::MutexT<Alloc>::MutexT(const Alloc&)': 00041 * apex/include/PsMutex.h:92: error: no matching function for call to 'operator new(unsigned int, 00042 * physx::shdfnd::MutexImpl*&)' 00043 * <built-in>:0: note: candidates are: void* operator new(unsigned int) 00044 */ 00045 #include <new> 00046 00047 namespace physx 00048 { 00049 namespace shdfnd 00050 { 00051 class PX_FOUNDATION_API MutexImpl 00052 { 00053 public: 00057 MutexImpl(); 00058 00062 ~MutexImpl(); 00063 00069 void lock(); 00070 00075 bool trylock(); 00076 00080 void unlock(); 00081 00085 static const uint32_t& getSize(); 00086 }; 00087 00088 template <typename Alloc = ReflectionAllocator<MutexImpl> > 00089 class MutexT : protected Alloc 00090 { 00091 PX_NOCOPY(MutexT) 00092 public: 00093 class ScopedLock 00094 { 00095 MutexT<Alloc>& mMutex; 00096 PX_NOCOPY(ScopedLock) 00097 public: 00098 PX_INLINE ScopedLock(MutexT<Alloc>& mutex) : mMutex(mutex) 00099 { 00100 mMutex.lock(); 00101 } 00102 PX_INLINE ~ScopedLock() 00103 { 00104 mMutex.unlock(); 00105 } 00106 }; 00107 00111 MutexT(const Alloc& alloc = Alloc()) : Alloc(alloc) 00112 { 00113 mImpl = reinterpret_cast<MutexImpl*>(Alloc::allocate(MutexImpl::getSize(), __FILE__, __LINE__)); 00114 PX_PLACEMENT_NEW(mImpl, MutexImpl)(); 00115 } 00116 00120 ~MutexT() 00121 { 00122 mImpl->~MutexImpl(); 00123 Alloc::deallocate(mImpl); 00124 } 00125 00131 void lock() const 00132 { 00133 mImpl->lock(); 00134 } 00135 00141 bool trylock() const 00142 { 00143 return mImpl->trylock(); 00144 } 00145 00150 void unlock() const 00151 { 00152 mImpl->unlock(); 00153 } 00154 00155 private: 00156 MutexImpl* mImpl; 00157 }; 00158 00159 class PX_FOUNDATION_API ReadWriteLock 00160 { 00161 PX_NOCOPY(ReadWriteLock) 00162 public: 00163 ReadWriteLock(); 00164 ~ReadWriteLock(); 00165 00166 void lockReader(); 00167 void lockWriter(); 00168 00169 void unlockReader(); 00170 void unlockWriter(); 00171 00172 private: 00173 class ReadWriteLockImpl* mImpl; 00174 }; 00175 00176 class ScopedReadLock 00177 { 00178 PX_NOCOPY(ScopedReadLock) 00179 public: 00180 PX_INLINE ScopedReadLock(ReadWriteLock& lock) : mLock(lock) 00181 { 00182 mLock.lockReader(); 00183 } 00184 PX_INLINE ~ScopedReadLock() 00185 { 00186 mLock.unlockReader(); 00187 } 00188 00189 private: 00190 ReadWriteLock& mLock; 00191 }; 00192 00193 class ScopedWriteLock 00194 { 00195 PX_NOCOPY(ScopedWriteLock) 00196 public: 00197 PX_INLINE ScopedWriteLock(ReadWriteLock& lock) : mLock(lock) 00198 { 00199 mLock.lockWriter(); 00200 } 00201 PX_INLINE ~ScopedWriteLock() 00202 { 00203 mLock.unlockWriter(); 00204 } 00205 00206 private: 00207 ReadWriteLock& mLock; 00208 }; 00209 00210 typedef MutexT<> Mutex; 00211 00212 /* 00213 * Use this type of lock for mutex behaviour that must operate on SPU and PPU 00214 * On non-PS3 platforms, it is implemented using Mutex 00215 */ 00216 class AtomicLock 00217 { 00218 Mutex mMutex; 00219 PX_NOCOPY(AtomicLock) 00220 00221 public: 00222 AtomicLock() 00223 { 00224 } 00225 00226 bool lock() 00227 { 00228 mMutex.lock(); 00229 return true; 00230 } 00231 00232 bool trylock() 00233 { 00234 return mMutex.trylock(); 00235 } 00236 00237 bool unlock() 00238 { 00239 mMutex.unlock(); 00240 return true; 00241 } 00242 }; 00243 00244 class AtomicLockCopy 00245 { 00246 AtomicLock* pLock; 00247 00248 public: 00249 AtomicLockCopy() : pLock(NULL) 00250 { 00251 } 00252 00253 AtomicLockCopy& operator=(AtomicLock& lock) 00254 { 00255 pLock = &lock; 00256 return *this; 00257 } 00258 00259 bool lock() 00260 { 00261 return pLock->lock(); 00262 } 00263 00264 bool trylock() 00265 { 00266 return pLock->trylock(); 00267 } 00268 00269 bool unlock() 00270 { 00271 return pLock->unlock(); 00272 } 00273 }; 00274 00275 class AtomicRwLock 00276 { 00277 ReadWriteLock m_Lock; 00278 PX_NOCOPY(AtomicRwLock) 00279 00280 public: 00281 AtomicRwLock() 00282 { 00283 } 00284 00285 void lockReader() 00286 { 00287 m_Lock.lockReader(); 00288 } 00289 void lockWriter() 00290 { 00291 m_Lock.lockWriter(); 00292 } 00293 00294 bool tryLockReader() 00295 { 00296 // Todo - implement this 00297 m_Lock.lockReader(); 00298 return true; 00299 } 00300 00301 void unlockReader() 00302 { 00303 m_Lock.unlockReader(); 00304 } 00305 void unlockWriter() 00306 { 00307 m_Lock.unlockWriter(); 00308 } 00309 }; 00310 00311 class ScopedAtomicLock 00312 { 00313 PX_INLINE ScopedAtomicLock(AtomicLock& lock) : mLock(lock) 00314 { 00315 mLock.lock(); 00316 } 00317 PX_INLINE ~ScopedAtomicLock() 00318 { 00319 mLock.unlock(); 00320 } 00321 00322 PX_NOCOPY(ScopedAtomicLock) 00323 private: 00324 AtomicLock& mLock; 00325 }; 00326 00327 } // namespace shdfnd 00328 } // namespace physx 00329 00330 #endif // #ifndef PSFOUNDATION_PSMUTEX_H
Generated on Tue Jul 28 14:21:55 2015 for NVIDIA(R) PsFoundation Reference by
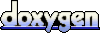