E:/p4/sw/physx/PxShared/1.0/trunk/src/foundation/include/PsHash.h
Go to the documentation of this file.00001 // This code contains NVIDIA Confidential Information and is disclosed to you 00002 // under a form of NVIDIA software license agreement provided separately to you. 00003 // 00004 // Notice 00005 // NVIDIA Corporation and its licensors retain all intellectual property and 00006 // proprietary rights in and to this software and related documentation and 00007 // any modifications thereto. Any use, reproduction, disclosure, or 00008 // distribution of this software and related documentation without an express 00009 // license agreement from NVIDIA Corporation is strictly prohibited. 00010 // 00011 // ALL NVIDIA DESIGN SPECIFICATIONS, CODE ARE PROVIDED "AS IS.". NVIDIA MAKES 00012 // NO WARRANTIES, EXPRESSED, IMPLIED, STATUTORY, OR OTHERWISE WITH RESPECT TO 00013 // THE MATERIALS, AND EXPRESSLY DISCLAIMS ALL IMPLIED WARRANTIES OF NONINFRINGEMENT, 00014 // MERCHANTABILITY, AND FITNESS FOR A PARTICULAR PURPOSE. 00015 // 00016 // Information and code furnished is believed to be accurate and reliable. 00017 // However, NVIDIA Corporation assumes no responsibility for the consequences of use of such 00018 // information or for any infringement of patents or other rights of third parties that may 00019 // result from its use. No license is granted by implication or otherwise under any patent 00020 // or patent rights of NVIDIA Corporation. Details are subject to change without notice. 00021 // This code supersedes and replaces all information previously supplied. 00022 // NVIDIA Corporation products are not authorized for use as critical 00023 // components in life support devices or systems without express written approval of 00024 // NVIDIA Corporation. 00025 // 00026 // Copyright (c) 2008-2014 NVIDIA Corporation. All rights reserved. 00027 // Copyright (c) 2004-2008 AGEIA Technologies, Inc. All rights reserved. 00028 // Copyright (c) 2001-2004 NovodeX AG. All rights reserved. 00029 00030 #ifndef PSFOUNDATION_PSHASH_H 00031 #define PSFOUNDATION_PSHASH_H 00032 00033 #include "Ps.h" 00034 #include "PsBasicTemplates.h" 00035 00036 #if PX_VC 00037 #pragma warning(push) 00038 #pragma warning(disable : 4302) 00039 #endif 00040 00041 #if PX_LINUX 00042 #include "PxSimpleTypes.h" 00043 #endif 00044 00049 namespace physx 00050 { 00051 namespace shdfnd 00052 { 00053 // Hash functions 00054 00055 // Thomas Wang's 32 bit mix 00056 // http://www.cris.com/~Ttwang/tech/inthash.htm 00057 PX_FORCE_INLINE uint32_t hash(const uint32_t key) 00058 { 00059 uint32_t k = key; 00060 k += ~(k << 15); 00061 k ^= (k >> 10); 00062 k += (k << 3); 00063 k ^= (k >> 6); 00064 k += ~(k << 11); 00065 k ^= (k >> 16); 00066 return uint32_t(k); 00067 } 00068 00069 PX_FORCE_INLINE uint32_t hash(const int32_t key) 00070 { 00071 return hash(uint32_t(key)); 00072 } 00073 00074 // Thomas Wang's 64 bit mix 00075 // http://www.cris.com/~Ttwang/tech/inthash.htm 00076 PX_FORCE_INLINE uint32_t hash(const uint64_t key) 00077 { 00078 uint64_t k = key; 00079 k += ~(k << 32); 00080 k ^= (k >> 22); 00081 k += ~(k << 13); 00082 k ^= (k >> 8); 00083 k += (k << 3); 00084 k ^= (k >> 15); 00085 k += ~(k << 27); 00086 k ^= (k >> 31); 00087 return uint32_t(UINT32_MAX & k); 00088 } 00089 00090 #if PX_APPLE_FAMILY 00091 // hash for size_t, to make gcc happy 00092 PX_INLINE uint32_t hash(const size_t key) 00093 { 00094 #if PX_P64_FAMILY 00095 return hash(uint64_t(key)); 00096 #else 00097 return hash(uint32_t(key)); 00098 #endif 00099 } 00100 #endif 00101 00102 // Hash function for pointers 00103 PX_INLINE uint32_t hash(const void* ptr) 00104 { 00105 #if PX_P64_FAMILY 00106 return hash(uint64_t(ptr)); 00107 #else 00108 return hash(uint32_t(UINT32_MAX & size_t(ptr))); 00109 #endif 00110 } 00111 00112 // Hash function for pairs 00113 template <typename F, typename S> 00114 PX_INLINE uint32_t hash(const Pair<F, S>& p) 00115 { 00116 uint32_t seed = 0x876543; 00117 uint32_t m = 1000007; 00118 return hash(p.second) ^ (m * (hash(p.first) ^ (m * seed))); 00119 } 00120 00121 // hash object for hash map template parameter 00122 template <class Key> 00123 struct Hash 00124 { 00125 uint32_t operator()(const Key& k) const 00126 { 00127 return hash(k); 00128 } 00129 bool equal(const Key& k0, const Key& k1) const 00130 { 00131 return k0 == k1; 00132 } 00133 }; 00134 00135 // specialization for strings 00136 template <> 00137 struct Hash<const char*> 00138 { 00139 public: 00140 uint32_t operator()(const char* _string) const 00141 { 00142 // "DJB" string hash 00143 const uint8_t* string = reinterpret_cast<const uint8_t*>(_string); 00144 uint32_t h = 5381; 00145 for(const uint8_t* ptr = string; *ptr; ptr++) 00146 h = ((h << 5) + h) ^ uint32_t(*ptr); 00147 return h; 00148 } 00149 bool equal(const char* string0, const char* string1) const 00150 { 00151 return !strcmp(string0, string1); 00152 } 00153 }; 00154 00155 } // namespace shdfnd 00156 } // namespace physx 00157 00158 #if PX_VC 00159 #pragma warning(pop) 00160 #endif 00161 00162 #endif // #ifndef PSFOUNDATION_PSHASH_H
Generated on Tue Jul 28 14:21:55 2015 for NVIDIA(R) PsFoundation Reference by
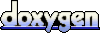