E:/p4/sw/physx/PxShared/1.0/trunk/src/foundation/include/PsBitUtils.h
Go to the documentation of this file.00001 // This code contains NVIDIA Confidential Information and is disclosed to you 00002 // under a form of NVIDIA software license agreement provided separately to you. 00003 // 00004 // Notice 00005 // NVIDIA Corporation and its licensors retain all intellectual property and 00006 // proprietary rights in and to this software and related documentation and 00007 // any modifications thereto. Any use, reproduction, disclosure, or 00008 // distribution of this software and related documentation without an express 00009 // license agreement from NVIDIA Corporation is strictly prohibited. 00010 // 00011 // ALL NVIDIA DESIGN SPECIFICATIONS, CODE ARE PROVIDED "AS IS.". NVIDIA MAKES 00012 // NO WARRANTIES, EXPRESSED, IMPLIED, STATUTORY, OR OTHERWISE WITH RESPECT TO 00013 // THE MATERIALS, AND EXPRESSLY DISCLAIMS ALL IMPLIED WARRANTIES OF NONINFRINGEMENT, 00014 // MERCHANTABILITY, AND FITNESS FOR A PARTICULAR PURPOSE. 00015 // 00016 // Information and code furnished is believed to be accurate and reliable. 00017 // However, NVIDIA Corporation assumes no responsibility for the consequences of use of such 00018 // information or for any infringement of patents or other rights of third parties that may 00019 // result from its use. No license is granted by implication or otherwise under any patent 00020 // or patent rights of NVIDIA Corporation. Details are subject to change without notice. 00021 // This code supersedes and replaces all information previously supplied. 00022 // NVIDIA Corporation products are not authorized for use as critical 00023 // components in life support devices or systems without express written approval of 00024 // NVIDIA Corporation. 00025 // 00026 // Copyright (c) 2008-2014 NVIDIA Corporation. All rights reserved. 00027 // Copyright (c) 2004-2008 AGEIA Technologies, Inc. All rights reserved. 00028 // Copyright (c) 2001-2004 NovodeX AG. All rights reserved. 00029 00030 #ifndef PSFOUNDATION_PSBITUTILS_H 00031 #define PSFOUNDATION_PSBITUTILS_H 00032 00033 #include "foundation/PxIntrinsics.h" 00034 #include "foundation/PxAssert.h" 00035 #include "PsIntrinsics.h" 00036 #include "Ps.h" 00037 00038 namespace physx 00039 { 00040 namespace shdfnd 00041 { 00042 PX_INLINE uint32_t bitCount(uint32_t v) 00043 { 00044 // from http://graphics.stanford.edu/~seander/bithacks.html#CountBitsSetParallel 00045 uint32_t const w = v - ((v >> 1) & 0x55555555); 00046 uint32_t const x = (w & 0x33333333) + ((w >> 2) & 0x33333333); 00047 return (((x + (x >> 4)) & 0xF0F0F0F) * 0x1010101) >> 24; 00048 } 00049 00050 PX_INLINE bool isPowerOfTwo(uint32_t x) 00051 { 00052 return x != 0 && (x & (x - 1)) == 0; 00053 } 00054 00055 // "Next Largest Power of 2 00056 // Given a binary integer value x, the next largest power of 2 can be computed by a SWAR algorithm 00057 // that recursively "folds" the upper bits into the lower bits. This process yields a bit vector with 00058 // the same most significant 1 as x, but all 1's below it. Adding 1 to that value yields the next 00059 // largest power of 2. For a 32-bit value:" 00060 PX_INLINE uint32_t nextPowerOfTwo(uint32_t x) 00061 { 00062 x |= (x >> 1); 00063 x |= (x >> 2); 00064 x |= (x >> 4); 00065 x |= (x >> 8); 00066 x |= (x >> 16); 00067 return x + 1; 00068 } 00069 00074 PX_INLINE uint32_t lowestSetBit(uint32_t x) 00075 { 00076 PX_ASSERT(x); 00077 return lowestSetBitUnsafe(x); 00078 } 00079 00084 PX_INLINE uint32_t highestSetBit(uint32_t x) 00085 { 00086 PX_ASSERT(x); 00087 return highestSetBitUnsafe(x); 00088 } 00089 00090 // Helper function to approximate log2 of an integer value 00091 // assumes that the input is actually power of two. 00092 // todo: replace 2 usages with 'highestSetBit' 00093 PX_INLINE uint32_t ilog2(uint32_t num) 00094 { 00095 for(uint32_t i = 0; i < 32; i++) 00096 { 00097 num >>= 1; 00098 if(num == 0) 00099 return i; 00100 } 00101 00102 PX_ASSERT(0); 00103 return uint32_t(-1); 00104 } 00105 00106 } // namespace shdfnd 00107 } // namespace physx 00108 00109 #endif // #ifndef PSFOUNDATION_PSBITUTILS_H
Generated on Tue Jul 28 14:21:55 2015 for NVIDIA(R) PsFoundation Reference by
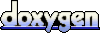