E:/p4/sw/physx/PxShared/1.0/trunk/src/foundation/include/PsBasicTemplates.h
Go to the documentation of this file.00001 // This code contains NVIDIA Confidential Information and is disclosed to you 00002 // under a form of NVIDIA software license agreement provided separately to you. 00003 // 00004 // Notice 00005 // NVIDIA Corporation and its licensors retain all intellectual property and 00006 // proprietary rights in and to this software and related documentation and 00007 // any modifications thereto. Any use, reproduction, disclosure, or 00008 // distribution of this software and related documentation without an express 00009 // license agreement from NVIDIA Corporation is strictly prohibited. 00010 // 00011 // ALL NVIDIA DESIGN SPECIFICATIONS, CODE ARE PROVIDED "AS IS.". NVIDIA MAKES 00012 // NO WARRANTIES, EXPRESSED, IMPLIED, STATUTORY, OR OTHERWISE WITH RESPECT TO 00013 // THE MATERIALS, AND EXPRESSLY DISCLAIMS ALL IMPLIED WARRANTIES OF NONINFRINGEMENT, 00014 // MERCHANTABILITY, AND FITNESS FOR A PARTICULAR PURPOSE. 00015 // 00016 // Information and code furnished is believed to be accurate and reliable. 00017 // However, NVIDIA Corporation assumes no responsibility for the consequences of use of such 00018 // information or for any infringement of patents or other rights of third parties that may 00019 // result from its use. No license is granted by implication or otherwise under any patent 00020 // or patent rights of NVIDIA Corporation. Details are subject to change without notice. 00021 // This code supersedes and replaces all information previously supplied. 00022 // NVIDIA Corporation products are not authorized for use as critical 00023 // components in life support devices or systems without express written approval of 00024 // NVIDIA Corporation. 00025 // 00026 // Copyright (c) 2008-2014 NVIDIA Corporation. All rights reserved. 00027 // Copyright (c) 2004-2008 AGEIA Technologies, Inc. All rights reserved. 00028 // Copyright (c) 2001-2004 NovodeX AG. All rights reserved. 00029 00030 #ifndef PSFOUNDATION_PSBASICTEMPLATES_H 00031 #define PSFOUNDATION_PSBASICTEMPLATES_H 00032 00033 #include "Ps.h" 00034 00035 namespace physx 00036 { 00037 namespace shdfnd 00038 { 00039 template <typename A> 00040 struct Equal 00041 { 00042 bool operator()(const A& a, const A& b) const 00043 { 00044 return a == b; 00045 } 00046 }; 00047 00048 template <typename A> 00049 struct Less 00050 { 00051 bool operator()(const A& a, const A& b) const 00052 { 00053 return a < b; 00054 } 00055 }; 00056 00057 template <typename A> 00058 struct Greater 00059 { 00060 bool operator()(const A& a, const A& b) const 00061 { 00062 return a > b; 00063 } 00064 }; 00065 00066 template <class F, class S> 00067 class Pair 00068 { 00069 public: 00070 F first; 00071 S second; 00072 Pair() : first(F()), second(S()) 00073 { 00074 } 00075 Pair(const F& f, const S& s) : first(f), second(s) 00076 { 00077 } 00078 Pair(const Pair& p) : first(p.first), second(p.second) 00079 { 00080 } 00081 // CN - fix for /.../PsBasicTemplates.h(61) : warning C4512: 'physx::shdfnd::Pair<F,S>' : assignment operator could 00082 // not be generated 00083 Pair& operator=(const Pair& p) 00084 { 00085 first = p.first; 00086 second = p.second; 00087 return *this; 00088 } 00089 bool operator==(const Pair& p) const 00090 { 00091 return first == p.first && second == p.second; 00092 } 00093 bool operator<(const Pair& p) const 00094 { 00095 if(first < p.first) 00096 return true; 00097 else 00098 return !(p.first < first) && (second < p.second); 00099 } 00100 }; 00101 00102 template <unsigned int A> 00103 struct LogTwo 00104 { 00105 static const unsigned int value = LogTwo<(A >> 1)>::value + 1; 00106 }; 00107 template <> 00108 struct LogTwo<1> 00109 { 00110 static const unsigned int value = 0; 00111 }; 00112 00113 template <typename T> 00114 struct UnConst 00115 { 00116 typedef T Type; 00117 }; 00118 template <typename T> 00119 struct UnConst<const T> 00120 { 00121 typedef T Type; 00122 }; 00123 00124 template <typename T> 00125 T pointerOffset(void* p, ptrdiff_t offset) 00126 { 00127 return reinterpret_cast<T>(reinterpret_cast<char*>(p) + offset); 00128 } 00129 template <typename T> 00130 T pointerOffset(const void* p, ptrdiff_t offset) 00131 { 00132 return reinterpret_cast<T>(reinterpret_cast<const char*>(p) + offset); 00133 } 00134 00135 template <class T> 00136 PX_CUDA_CALLABLE PX_INLINE void swap(T& x, T& y) 00137 { 00138 const T tmp = x; 00139 x = y; 00140 y = tmp; 00141 } 00142 00143 } // namespace shdfnd 00144 } // namespace physx 00145 00146 #endif // #ifndef PSFOUNDATION_PSBASICTEMPLATES_H
Generated on Tue Jul 28 14:21:55 2015 for NVIDIA(R) PsFoundation Reference by
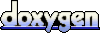