E:/p4/sw/physx/PxShared/1.0/trunk/src/foundation/include/PsAllocator.h
Go to the documentation of this file.00001 // This code contains NVIDIA Confidential Information and is disclosed to you 00002 // under a form of NVIDIA software license agreement provided separately to you. 00003 // 00004 // Notice 00005 // NVIDIA Corporation and its licensors retain all intellectual property and 00006 // proprietary rights in and to this software and related documentation and 00007 // any modifications thereto. Any use, reproduction, disclosure, or 00008 // distribution of this software and related documentation without an express 00009 // license agreement from NVIDIA Corporation is strictly prohibited. 00010 // 00011 // ALL NVIDIA DESIGN SPECIFICATIONS, CODE ARE PROVIDED "AS IS.". NVIDIA MAKES 00012 // NO WARRANTIES, EXPRESSED, IMPLIED, STATUTORY, OR OTHERWISE WITH RESPECT TO 00013 // THE MATERIALS, AND EXPRESSLY DISCLAIMS ALL IMPLIED WARRANTIES OF NONINFRINGEMENT, 00014 // MERCHANTABILITY, AND FITNESS FOR A PARTICULAR PURPOSE. 00015 // 00016 // Information and code furnished is believed to be accurate and reliable. 00017 // However, NVIDIA Corporation assumes no responsibility for the consequences of use of such 00018 // information or for any infringement of patents or other rights of third parties that may 00019 // result from its use. No license is granted by implication or otherwise under any patent 00020 // or patent rights of NVIDIA Corporation. Details are subject to change without notice. 00021 // This code supersedes and replaces all information previously supplied. 00022 // NVIDIA Corporation products are not authorized for use as critical 00023 // components in life support devices or systems without express written approval of 00024 // NVIDIA Corporation. 00025 // 00026 // Copyright (c) 2008-2014 NVIDIA Corporation. All rights reserved. 00027 // Copyright (c) 2004-2008 AGEIA Technologies, Inc. All rights reserved. 00028 // Copyright (c) 2001-2004 NovodeX AG. All rights reserved. 00029 00030 #ifndef PSFOUNDATION_PSALLOCATOR_H 00031 #define PSFOUNDATION_PSALLOCATOR_H 00032 00033 #include "foundation/PxAllocatorCallback.h" 00034 #include "Ps.h" 00035 #include "PsGlobals.h" 00036 00037 #if(PX_WINDOWS_FAMILY || PX_WINRT || PX_XBOXONE) 00038 #include <exception> 00039 #include <typeinfo.h> 00040 #endif 00041 #if(PX_APPLE_FAMILY) 00042 #include <typeinfo> 00043 #endif 00044 00045 #include <new> 00046 00047 // Allocation macros going through user allocator 00048 #if PX_CHECKED 00049 #define PX_ALLOC(n, name) physx::shdfnd::NamedAllocator(name).allocate(n, __FILE__, __LINE__) 00050 #else 00051 #define PX_ALLOC(n, name) physx::shdfnd::NonTrackingAllocator().allocate(n, __FILE__, __LINE__) 00052 #endif 00053 #define PX_ALLOC_TEMP(n, name) PX_ALLOC(n, name) 00054 #define PX_FREE(x) physx::shdfnd::NonTrackingAllocator().deallocate(x) 00055 #define PX_FREE_AND_RESET(x) \ 00056 { \ 00057 PX_FREE(x); \ 00058 x = 0; \ 00059 } 00060 00061 // The following macros support plain-old-types and classes derived from UserAllocated. 00062 #define PX_NEW(T) new (physx::shdfnd::ReflectionAllocator<T>(), __FILE__, __LINE__) T 00063 #define PX_NEW_TEMP(T) PX_NEW(T) 00064 #define PX_DELETE(x) delete x 00065 #define PX_DELETE_AND_RESET(x) \ 00066 { \ 00067 PX_DELETE(x); \ 00068 x = 0; \ 00069 } 00070 #define PX_DELETE_POD(x) \ 00071 { \ 00072 PX_FREE(x); \ 00073 x = 0; \ 00074 } 00075 #define PX_DELETE_ARRAY(x) \ 00076 { \ 00077 PX_DELETE([] x); \ 00078 x = 0; \ 00079 } 00080 00081 // aligned allocation 00082 #define PX_ALIGNED16_ALLOC(n) physx::shdfnd::AlignedAllocator<16>().allocate(n, __FILE__, __LINE__) 00083 #define PX_ALIGNED16_FREE(x) physx::shdfnd::AlignedAllocator<16>().deallocate(x) 00084 00086 #define PX_PLACEMENT_NEW(p, T) new (p) T 00087 00088 #if PX_DEBUG || PX_CHECKED 00089 #define PX_USE_NAMED_ALLOCATOR 1 00090 #else 00091 #define PX_USE_NAMED_ALLOCATOR 0 00092 #endif 00093 00094 // Don't use inline for alloca !!! 00095 #if PX_WINDOWS_FAMILY || PX_WINRT 00096 #include <malloc.h> 00097 #define PxAlloca(x) _alloca(x) 00098 #elif PX_LINUX || PX_ANDROID 00099 #include <malloc.h> 00100 #define PxAlloca(x) alloca(x) 00101 #elif PX_APPLE_FAMILY 00102 #include <alloca.h> 00103 #define PxAlloca(x) alloca(x) 00104 #elif PX_PS4 00105 #include <memory.h> 00106 #define PxAlloca(x) alloca(x) 00107 #elif PX_XBOXONE 00108 #include <malloc.h> 00109 #define PxAlloca(x) alloca(x) 00110 #endif 00111 00112 #define PxAllocaAligned(x, alignment) ((size_t(PxAlloca(x + alignment)) + (alignment - 1)) & ~size_t(alignment - 1)) 00113 00114 namespace physx 00115 { 00116 namespace shdfnd 00117 { 00118 /* 00119 * Bootstrap allocator using malloc/free. 00120 * Don't use unless your objects get allocated before foundation is initialized. 00121 */ 00122 class RawAllocator 00123 { 00124 public: 00125 RawAllocator(const char* = 0) 00126 { 00127 } 00128 void* allocate(size_t size, const char*, int) 00129 { 00130 // malloc returns valid pointer for size==0, no need to check 00131 return ::malloc(size); 00132 } 00133 void deallocate(void* ptr) 00134 { 00135 // free(0) is guaranteed to have no side effect, no need to check 00136 ::free(ptr); 00137 } 00138 }; 00139 00140 /* 00141 * Allocator that simply calls straight back to the application without tracking. 00142 * This is used by the heap (Foundation::mNamedAllocMap) that tracks allocations 00143 * because it needs to be able to grow as a result of an allocation. 00144 * Making the hash table re-entrant to deal with this may not make sense. 00145 */ 00146 class NonTrackingAllocator 00147 { 00148 public: 00149 PX_FORCE_INLINE NonTrackingAllocator(const char* = 0) 00150 { 00151 } 00152 PX_FORCE_INLINE void* allocate(size_t size, const char* file, int line) 00153 { 00154 return !size ? 0 : getAllocator().allocate(size, "NonTrackedAlloc", file, line); 00155 } 00156 PX_FORCE_INLINE void deallocate(void* ptr) 00157 { 00158 if(ptr) 00159 getAllocator().deallocate(ptr); 00160 } 00161 }; 00162 00166 void initializeNamedAllocatorGlobals(); 00167 void terminateNamedAllocatorGlobals(); 00168 00169 #if PX_USE_NAMED_ALLOCATOR // can be slow, so only use in debug/checked 00170 class PX_FOUNDATION_API NamedAllocator 00171 { 00172 public: 00173 NamedAllocator(const PxEMPTY); 00174 NamedAllocator(const char* name = 0); // todo: should not have default argument! 00175 NamedAllocator(const NamedAllocator&); 00176 ~NamedAllocator(); 00177 NamedAllocator& operator=(const NamedAllocator&); 00178 void* allocate(size_t size, const char* filename, int line); 00179 void deallocate(void* ptr); 00180 }; 00181 #else 00182 class NamedAllocator; 00183 #endif // PX_DEBUG 00184 00189 template <typename T> 00190 class ReflectionAllocator 00191 { 00192 static const char* getName() 00193 { 00194 if(!getReflectionAllocatorReportsNames()) 00195 return "<allocation names disabled>"; 00196 #if PX_GCC_FAMILY 00197 return __PRETTY_FUNCTION__; 00198 #else 00199 // name() calls malloc(), raw_name() wouldn't 00200 return typeid(T).name(); 00201 #endif 00202 } 00203 00204 public: 00205 ReflectionAllocator(const PxEMPTY) 00206 { 00207 } 00208 ReflectionAllocator(const char* = 0) 00209 { 00210 } 00211 inline ReflectionAllocator(const ReflectionAllocator&) 00212 { 00213 } 00214 void* allocate(size_t size, const char* filename, int line) 00215 { 00216 return size ? getAllocator().allocate(size, getName(), filename, line) : 0; 00217 } 00218 void deallocate(void* ptr) 00219 { 00220 if(ptr) 00221 getAllocator().deallocate(ptr); 00222 } 00223 }; 00224 00225 template <typename T> 00226 struct AllocatorTraits 00227 { 00228 #if PX_USE_NAMED_ALLOCATOR 00229 typedef NamedAllocator Type; 00230 #else 00231 typedef ReflectionAllocator<T> Type; 00232 #endif 00233 }; 00234 00235 // if you get a build error here, you are trying to PX_NEW a class 00236 // that is neither plain-old-type nor derived from UserAllocated 00237 template <typename T, typename X> 00238 union EnableIfPod 00239 { 00240 int i; 00241 T t; 00242 typedef X Type; 00243 }; 00244 00245 } // namespace shdfnd 00246 } // namespace physx 00247 00248 // Global placement new for ReflectionAllocator templated by 00249 // plain-old-type. Allows using PX_NEW for pointers and built-in-types. 00250 // 00251 // ATTENTION: You need to use PX_DELETE_POD or PX_FREE to deallocate 00252 // memory, not PX_DELETE. PX_DELETE_POD redirects to PX_FREE. 00253 // 00254 // Rationale: PX_DELETE uses global operator delete(void*), which we dont' want to overload. 00255 // Any other definition of PX_DELETE couldn't support array syntax 'PX_DELETE([]a);'. 00256 // PX_DELETE_POD was preferred over PX_DELETE_ARRAY because it is used 00257 // less often and applies to both single instances and arrays. 00258 template <typename T> 00259 PX_INLINE void* operator new(size_t size, physx::shdfnd::ReflectionAllocator<T> alloc, const char* fileName, 00260 typename physx::shdfnd::EnableIfPod<T, int>::Type line) 00261 { 00262 return alloc.allocate(size, fileName, line); 00263 } 00264 00265 template <typename T> 00266 PX_INLINE void* operator new [](size_t size, physx::shdfnd::ReflectionAllocator<T> alloc, const char* fileName, 00267 typename physx::shdfnd::EnableIfPod<T, int>::Type line) 00268 { return alloc.allocate(size, fileName, line); } 00269 00270 // If construction after placement new throws, this placement delete is being called. 00271 template <typename T> 00272 PX_INLINE void operator delete(void* ptr, physx::shdfnd::ReflectionAllocator<T> alloc, const char* fileName, 00273 typename physx::shdfnd::EnableIfPod<T, int>::Type line) 00274 { 00275 PX_UNUSED(fileName); 00276 PX_UNUSED(line); 00277 00278 alloc.deallocate(ptr); 00279 } 00280 00281 // If construction after placement new throws, this placement delete is being called. 00282 template <typename T> 00283 PX_INLINE void operator delete [](void* ptr, physx::shdfnd::ReflectionAllocator<T> alloc, const char* fileName, 00284 typename physx::shdfnd::EnableIfPod<T, int>::Type line) 00285 { 00286 PX_UNUSED(fileName); 00287 PX_UNUSED(line); 00288 00289 alloc.deallocate(ptr); 00290 } 00291 00292 #endif // #ifndef PSFOUNDATION_PSALLOCATOR_H
Generated on Tue Jul 28 14:21:55 2015 for NVIDIA(R) PsFoundation Reference by
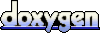