PxController Class Reference
[Character]
Base class for character controllers.
More...
#include <PxController.h>
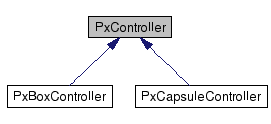
Public Member Functions | |
virtual PxControllerShapeType::Enum | getType () const =0 |
Return the type of controller. | |
virtual void | release ()=0 |
Releases the controller. | |
virtual PxControllerCollisionFlags | move (const PxVec3 &disp, PxF32 minDist, PxF32 elapsedTime, const PxControllerFilters &filters, const PxObstacleContext *obstacles=NULL)=0 |
Moves the character using a "collide-and-slide" algorithm. | |
virtual bool | setPosition (const PxExtendedVec3 &position)=0 |
Sets controller's position. | |
virtual const PxExtendedVec3 & | getPosition () const =0 |
Retrieve the raw position of the controller. | |
virtual bool | setFootPosition (const PxExtendedVec3 &position)=0 |
Set controller's foot position. | |
virtual PxExtendedVec3 | getFootPosition () const =0 |
Retrieve the "foot" position of the controller, i.e. the position of the bottom of the CCT's shape. | |
virtual PxRigidDynamic * | getActor () const =0 |
Get the rigid body actor associated with this controller (see PhysX documentation). The behavior upon manually altering this actor is undefined, you should primarily use it for reading const properties. | |
virtual void | setStepOffset (const PxF32 offset)=0 |
The step height. | |
virtual PxF32 | getStepOffset () const =0 |
Retrieve the step height. | |
virtual void | setNonWalkableMode (PxControllerNonWalkableMode::Enum flag)=0 |
Sets the non-walkable mode for the CCT. | |
virtual PxControllerNonWalkableMode::Enum | getNonWalkableMode () const =0 |
Retrieves the non-walkable mode for the CCT. | |
virtual PxF32 | getContactOffset () const =0 |
Retrieve the contact offset. | |
virtual void | setContactOffset (PxF32 offset)=0 |
Sets the contact offset. | |
virtual PxVec3 | getUpDirection () const =0 |
Retrieve the 'up' direction. | |
virtual void | setUpDirection (const PxVec3 &up)=0 |
Sets the 'up' direction. | |
virtual PxF32 | getSlopeLimit () const =0 |
Retrieve the slope limit. | |
virtual void | setSlopeLimit (PxF32 slopeLimit)=0 |
Sets the slope limit. | |
virtual void | invalidateCache ()=0 |
Flushes internal geometry cache. | |
virtual PxScene * | getScene ()=0 |
Retrieve the scene associated with the controller. | |
virtual void * | getUserData () const =0 |
Returns the user data associated with this controller. | |
virtual void | setUserData (void *userData)=0 |
Sets the user data associated with this controller. | |
virtual void | getState (PxControllerState &state) const =0 |
Returns information about the controller's internal state. | |
virtual void | getStats (PxControllerStats &stats) const =0 |
Returns the controller's internal statistics. | |
virtual void | resize (PxReal height)=0 |
Resizes the controller. | |
Protected Member Functions | |
PX_INLINE | PxController () |
virtual | ~PxController () |
Detailed Description
Base class for character controllers.
- See also:
- PxCapsuleController PxBoxController
Constructor & Destructor Documentation
PX_INLINE PxController::PxController | ( | ) | [inline, protected] |
virtual PxController::~PxController | ( | ) | [inline, protected, virtual] |
Member Function Documentation
virtual PxRigidDynamic* PxController::getActor | ( | ) | const [pure virtual] |
Get the rigid body actor associated with this controller (see PhysX documentation). The behavior upon manually altering this actor is undefined, you should primarily use it for reading const properties.
- Returns:
- the actor associated with the controller.
virtual PxF32 PxController::getContactOffset | ( | ) | const [pure virtual] |
Retrieve the contact offset.
- Returns:
- The contact offset for the controller.
- See also:
- PxControllerDesc.contactOffset
virtual PxExtendedVec3 PxController::getFootPosition | ( | ) | const [pure virtual] |
Retrieve the "foot" position of the controller, i.e. the position of the bottom of the CCT's shape.
- Note:
- The foot position takes the contact offset into account
- Returns:
- The controller's foot position
virtual PxControllerNonWalkableMode::Enum PxController::getNonWalkableMode | ( | ) | const [pure virtual] |
Retrieves the non-walkable mode for the CCT.
- Returns:
- The current non-walkable mode.
- See also:
- PxControllerNonWalkableMode
virtual const PxExtendedVec3& PxController::getPosition | ( | ) | const [pure virtual] |
Retrieve the raw position of the controller.
The position retrieved by this function is the center of the collision shape. To retrieve the bottom position of the shape, a.k.a. the foot position, use the getFootPosition() function.
The position is updated by calls to move(). Calling this method without calling move() will return the last position or the initial position of the controller.
- Returns:
- The controller's center position
virtual PxScene* PxController::getScene | ( | ) | [pure virtual] |
Retrieve the scene associated with the controller.
- Returns:
- The physics scene
virtual PxF32 PxController::getSlopeLimit | ( | ) | const [pure virtual] |
Retrieve the slope limit.
- Returns:
- The slope limit for the controller.
- See also:
- PxControllerDesc.slopeLimit
virtual void PxController::getState | ( | PxControllerState & | state | ) | const [pure virtual] |
Returns information about the controller's internal state.
- Parameters:
-
[out] state The controller's internal state
- See also:
- PxControllerState
virtual void PxController::getStats | ( | PxControllerStats & | stats | ) | const [pure virtual] |
Returns the controller's internal statistics.
- Parameters:
-
[out] stats The controller's internal statistics
- See also:
- PxControllerStats
virtual PxF32 PxController::getStepOffset | ( | ) | const [pure virtual] |
virtual PxControllerShapeType::Enum PxController::getType | ( | ) | const [pure virtual] |
Return the type of controller.
- See also:
- PxControllerType
virtual PxVec3 PxController::getUpDirection | ( | ) | const [pure virtual] |
Retrieve the 'up' direction.
- Returns:
- The up direction for the controller.
- See also:
- PxControllerDesc.upDirection
virtual void* PxController::getUserData | ( | ) | const [pure virtual] |
Returns the user data associated with this controller.
- Returns:
- The user pointer associated with the controller.
- See also:
- PxControllerDesc.userData
virtual void PxController::invalidateCache | ( | ) | [pure virtual] |
Flushes internal geometry cache.
The character controller uses caching in order to speed up collision testing. The cache is automatically flushed when a change to static objects is detected in the scene. For example when a static shape is added, updated, or removed from the scene, the cache is automatically invalidated.
However there may be situations that cannot be automatically detected, and those require manual invalidation of the cache. Currently the user must call this when the filtering behavior changes (the PxControllerFilters parameter of the PxController::move call). While the controller in principle could detect a change in these parameters, it cannot detect a change in the behavior of the filtering function.
- See also:
- PxController.move
virtual PxControllerCollisionFlags PxController::move | ( | const PxVec3 & | disp, | |
PxF32 | minDist, | |||
PxF32 | elapsedTime, | |||
const PxControllerFilters & | filters, | |||
const PxObstacleContext * | obstacles = NULL | |||
) | [pure virtual] |
Moves the character using a "collide-and-slide" algorithm.
- Parameters:
-
[in] disp Displacement vector [in] minDist The minimum travelled distance to consider. If travelled distance is smaller, the character doesn't move. This is used to stop the recursive motion algorithm when remaining distance to travel is small. [in] elapsedTime Time elapsed since last call [in] filters User-defined filters for this move [in] obstacles Potential additional obstacles the CCT should collide with.
- Returns:
- Collision flags, collection of PxControllerCollisionFlags
virtual void PxController::release | ( | ) | [pure virtual] |
Releases the controller.
virtual void PxController::resize | ( | PxReal | height | ) | [pure virtual] |
Resizes the controller.
This function attempts to resize the controller to a given size, while making sure the bottom position of the controller remains constant. In other words the function modifies both the height and the (center) position of the controller. This is a helper function that can be used to implement a 'crouch' functionality for example.
- Parameters:
-
[in] height Desired controller's height
virtual void PxController::setContactOffset | ( | PxF32 | offset | ) | [pure virtual] |
Sets the contact offset.
- Parameters:
-
[in] offset The contact offset for the controller.
- See also:
- PxControllerDesc.contactOffset
virtual bool PxController::setFootPosition | ( | const PxExtendedVec3 & | position | ) | [pure virtual] |
Set controller's foot position.
The position controlled by this function is the bottom of the collision shape, a.k.a. the foot position.
- Note:
- The foot position takes the contact offset into account
- Warning:
- This is a 'teleport' function, it doesn't check for collisions.
- Parameters:
-
[in] position The new (bottom) positon for the controller.
- Returns:
- Currently always returns true.
virtual void PxController::setNonWalkableMode | ( | PxControllerNonWalkableMode::Enum | flag | ) | [pure virtual] |
Sets the non-walkable mode for the CCT.
- Parameters:
-
[in] flag The new value of the non-walkable mode.
- See also:
- PxControllerNonWalkableMode
virtual bool PxController::setPosition | ( | const PxExtendedVec3 & | position | ) | [pure virtual] |
Sets controller's position.
The position controlled by this function is the center of the collision shape.
- Warning:
- This is a 'teleport' function, it doesn't check for collisions.
The character's position must be such that it does not overlap the static geometry.
- Parameters:
-
[in] position The new (center) positon for the controller.
- Returns:
- Currently always returns true.
virtual void PxController::setSlopeLimit | ( | PxF32 | slopeLimit | ) | [pure virtual] |
Sets the slope limit.
- Note:
- This feature can not be enabled at runtime, i.e. if the slope limit is zero when creating the CCT (which disables the feature) then changing the slope limit at runtime will not have any effect, and the call will be ignored.
- Parameters:
-
[in] slopeLimit The slope limit for the controller.
- See also:
- PxControllerDesc.slopeLimit
virtual void PxController::setStepOffset | ( | const PxF32 | offset | ) | [pure virtual] |
The step height.
- Parameters:
-
[in] offset The new step offset for the controller.
- See also:
- PxControllerDesc.stepOffset
virtual void PxController::setUpDirection | ( | const PxVec3 & | up | ) | [pure virtual] |
Sets the 'up' direction.
- Parameters:
-
[in] up The up direction for the controller.
- See also:
- PxControllerDesc.upDirection
virtual void PxController::setUserData | ( | void * | userData | ) | [pure virtual] |
Sets the user data associated with this controller.
- Parameters:
-
[in] userData The user pointer associated with the controller.
- See also:
- PxControllerDesc.userData
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com