PxContactStreamIterator Struct Reference
A class to iterate over a compressed contact stream. This supports read-only access to the various contact formats. More...
#include <PxContact.h>
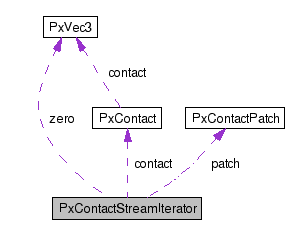
Public Types | |
enum | StreamFormat { eSIMPLE_STREAM, eMODIFIABLE_STREAM, eCOMPRESSED_MODIFIABLE_STREAM } |
Public Member Functions | |
PX_CUDA_CALLABLE PX_FORCE_INLINE | PxContactStreamIterator (const PxU8 *contactPatches, const PxU8 *contactPoints, const PxU32 *contactFaceIndices, PxU32 nbPatches, PxU32 nbContacts) |
Constructor. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE bool | hasNextPatch () const |
Returns whether there are more patches in this stream. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxU32 | getTotalContactCount () const |
Returns the total contact count. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxU32 | getTotalPatchCount () const |
PX_CUDA_CALLABLE PX_INLINE void | nextPatch () |
Advances iterator to next contact patch. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE bool | hasNextContact () const |
Returns if the current patch has more contacts. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE void | nextContact () |
Advances to the next contact in the patch. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE const PxVec3 & | getContactNormal () const |
Gets the current contact's normal. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal | getInvMassScale0 () const |
Gets the inverse mass scale for body 0. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal | getInvMassScale1 () const |
Gets the inverse mass scale for body 1. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal | getInvInertiaScale0 () const |
Gets the inverse inertia scale for body 0. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal | getInvInertiaScale1 () const |
Gets the inverse inertia scale for body 1. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal | getMaxImpulse () const |
Gets the contact's max impulse. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE const PxVec3 & | getTargetVel () const |
Gets the contact's target velocity. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE const PxVec3 & | getContactPoint () const |
Gets the contact's contact point. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal | getSeparation () const |
Gets the contact's separation. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxU32 | getFaceIndex0 () const |
Gets the contact's face index for shape 0. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxU32 | getFaceIndex1 () const |
Gets the contact's face index for shape 1. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal | getStaticFriction () const |
Gets the contact's static friction coefficient. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal | getDynamicFriction () const |
Gets the contact's static dynamic coefficient. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal | getRestitution () const |
Gets the contact's restitution coefficient. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxU32 | getMaterialFlags () const |
Gets the contact's material flags. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxU16 | getMaterialIndex0 () const |
Gets the contact's material index for shape 0. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxU16 | getMaterialIndex1 () const |
Gets the contact's material index for shape 1. | |
bool | advanceToIndex (const PxU32 initialIndex) |
Advances the contact stream iterator to a specific contact index. | |
Public Attributes | |
PxVec3 | zero |
Utility zero vector to optimize functions returning zero vectors when a certain flag isn't set. | |
const PxContactPatch * | patch |
The patch headers. | |
const PxContact * | contact |
The contacts. | |
const PxU32 * | faceIndice |
The contact triangle face index. | |
PxU32 | totalPatches |
The total number of patches in this contact stream. | |
PxU32 | totalContacts |
The total number of contact points in this stream. | |
PxU32 | nextContactIndex |
The current contact index. | |
PxU32 | nextPatchIndex |
The current patch Index. | |
PxU32 | contactPatchHeaderSize |
PxU32 | contactPointSize |
Contact point size. | |
StreamFormat | mStreamFormat |
The stream format. | |
PxU32 | forceNoResponse |
Indicates whether this stream is notify-only or not. | |
bool | pointStepped |
PxU32 | hasFaceIndices |
Private Member Functions | |
PX_CUDA_CALLABLE PX_FORCE_INLINE const PxContactPatch & | getContactPatch () const |
Internal helper. | |
PX_CUDA_CALLABLE PX_FORCE_INLINE const PxExtendedContact & | getExtendedContact () const |
Detailed Description
A class to iterate over a compressed contact stream. This supports read-only access to the various contact formats.Member Enumeration Documentation
Constructor & Destructor Documentation
Member Function Documentation
bool PxContactStreamIterator::advanceToIndex | ( | const PxU32 | initialIndex | ) | [inline] |
PX_CUDA_CALLABLE PX_FORCE_INLINE const PxVec3& PxContactStreamIterator::getContactNormal | ( | ) | const [inline] |
Gets the current contact's normal.
- Returns:
- The current contact's normal.
Referenced by PxContactPair::extractContacts().
PX_CUDA_CALLABLE PX_FORCE_INLINE const PxContactPatch& PxContactStreamIterator::getContactPatch | ( | ) | const [inline, private] |
Internal helper.
PX_CUDA_CALLABLE PX_FORCE_INLINE const PxVec3& PxContactStreamIterator::getContactPoint | ( | ) | const [inline] |
Gets the contact's contact point.
- Returns:
- The contact's contact point.
Referenced by PxContactPair::extractContacts().
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal PxContactStreamIterator::getDynamicFriction | ( | ) | const [inline] |
Gets the contact's static dynamic coefficient.
- Returns:
- The contact's static dynamic coefficient.
PX_CUDA_CALLABLE PX_FORCE_INLINE const PxExtendedContact& PxContactStreamIterator::getExtendedContact | ( | ) | const [inline, private] |
References PX_ASSERT.
PX_CUDA_CALLABLE PX_FORCE_INLINE PxU32 PxContactStreamIterator::getFaceIndex0 | ( | ) | const [inline] |
Gets the contact's face index for shape 0.
- Returns:
- The contact's face index for shape 0.
References PXC_CONTACT_NO_FACE_INDEX.
Referenced by PxContactPair::extractContacts().
PX_CUDA_CALLABLE PX_FORCE_INLINE PxU32 PxContactStreamIterator::getFaceIndex1 | ( | ) | const [inline] |
Gets the contact's face index for shape 1.
- Returns:
- The contact's face index for shape 1.
References PXC_CONTACT_NO_FACE_INDEX.
Referenced by PxContactPair::extractContacts().
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal PxContactStreamIterator::getInvInertiaScale0 | ( | ) | const [inline] |
Gets the inverse inertia scale for body 0.
- Returns:
- The inverse inertia scale for body 0.
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal PxContactStreamIterator::getInvInertiaScale1 | ( | ) | const [inline] |
Gets the inverse inertia scale for body 1.
- Returns:
- The inverse inertia scale for body 1.
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal PxContactStreamIterator::getInvMassScale0 | ( | ) | const [inline] |
Gets the inverse mass scale for body 0.
- Returns:
- The inverse mass scale for body 0.
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal PxContactStreamIterator::getInvMassScale1 | ( | ) | const [inline] |
Gets the inverse mass scale for body 1.
- Returns:
- The inverse mass scale for body 1.
PX_CUDA_CALLABLE PX_FORCE_INLINE PxU32 PxContactStreamIterator::getMaterialFlags | ( | ) | const [inline] |
Gets the contact's material flags.
- Returns:
- The contact's material flags.
PX_CUDA_CALLABLE PX_FORCE_INLINE PxU16 PxContactStreamIterator::getMaterialIndex0 | ( | ) | const [inline] |
Gets the contact's material index for shape 0.
- Returns:
- The contact's material index for shape 0.
PX_CUDA_CALLABLE PX_FORCE_INLINE PxU16 PxContactStreamIterator::getMaterialIndex1 | ( | ) | const [inline] |
Gets the contact's material index for shape 1.
- Returns:
- The contact's material index for shape 1.
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal PxContactStreamIterator::getMaxImpulse | ( | ) | const [inline] |
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal PxContactStreamIterator::getRestitution | ( | ) | const [inline] |
Gets the contact's restitution coefficient.
- Returns:
- The contact's restitution coefficient.
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal PxContactStreamIterator::getSeparation | ( | ) | const [inline] |
Gets the contact's separation.
- Returns:
- The contact's separation.
Referenced by PxContactPair::extractContacts().
PX_CUDA_CALLABLE PX_FORCE_INLINE PxReal PxContactStreamIterator::getStaticFriction | ( | ) | const [inline] |
Gets the contact's static friction coefficient.
- Returns:
- The contact's static friction coefficient.
PX_CUDA_CALLABLE PX_FORCE_INLINE const PxVec3& PxContactStreamIterator::getTargetVel | ( | ) | const [inline] |
Gets the contact's target velocity.
- Returns:
- The contact's target velocity.
PX_CUDA_CALLABLE PX_FORCE_INLINE PxU32 PxContactStreamIterator::getTotalContactCount | ( | ) | const [inline] |
Returns the total contact count.
- Returns:
- Total contact count.
PX_CUDA_CALLABLE PX_FORCE_INLINE PxU32 PxContactStreamIterator::getTotalPatchCount | ( | ) | const [inline] |
PX_CUDA_CALLABLE PX_FORCE_INLINE bool PxContactStreamIterator::hasNextContact | ( | ) | const [inline] |
Returns if the current patch has more contacts.
- Returns:
- If there are more contacts in the current patch.
Referenced by PxContactPair::extractContacts().
PX_CUDA_CALLABLE PX_FORCE_INLINE bool PxContactStreamIterator::hasNextPatch | ( | ) | const [inline] |
Returns whether there are more patches in this stream.
- Returns:
- Whether there are more patches in this stream.
Referenced by PxContactPair::extractContacts().
PX_CUDA_CALLABLE PX_FORCE_INLINE void PxContactStreamIterator::nextContact | ( | ) | [inline] |
Advances to the next contact in the patch.
References PX_ASSERT.
Referenced by PxContactPair::extractContacts().
PX_CUDA_CALLABLE PX_INLINE void PxContactStreamIterator::nextPatch | ( | ) | [inline] |
Advances iterator to next contact patch.
References PX_ASSERT.
Referenced by PxContactPair::extractContacts().
Member Data Documentation
The contacts.
Contact point size.
- Note:
- This varies whether the patch has feature indices or is modifiable.
The contact triangle face index.
Indicates whether this stream is notify-only or not.
The stream format.
The current contact index.
The current patch Index.
The patch headers.
The total number of contact points in this stream.
The total number of patches in this contact stream.
Utility zero vector to optimize functions returning zero vectors when a certain flag isn't set.
- Note:
- This allows us to return by reference instead of having to return by value. Returning by value will go via memory (registers -> stack -> registers), which can cause performance issues on certain platforms.
The documentation for this struct was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com