XFaceApp::Task Class Reference
#include <Task.h>
Detailed Description
- Author:
- Koray Balci
Here is a sample of usage from XfaceClient, where we issue a "resume playback" task through TCP/IP;
// we create a task with the client Id and a unique task ID. Task task("RESUME_PLAYBACK", m_clientId, ++m_taskCount + 1000000*m_clientId); // following function translates the Task to XML string std::string msg = XMLUtils::xmlify(task); // Here we send the string through network m_pSocket->Write(msg.c_str(), msg.size() + 1);
On the other hand, if this is a standalone application, or we have direct access to XFaceApp::TaskHandlerBase derived class, we can issue the Task as follows;
Task playtask("RESUME_PLAYBACK"); m_pApp->newTask(playtask); // m_pApp is a pointer to a TaskHandlerBase derived class instance.
Note that, we do not have to (and in fact should not) pass owner id and task id for the Task object, because, it is defaulted to 0, and this way we prevent creating/sending Notification messages. After all, the latter use is only for the thread that has access to the TaskHandlerBase class. A class derived from TaskHandlerBase, tailored for your own purposes can implement event notification mechanism much more efficiently.
Future: In the future versions, we can add plenty of things to this mechanism. Parameters can be in types other than string. ID can be assigned automatically and invisibly, however this can create a set of new problems, not sure.. And finally, we can implement a whole class hierarchy instead of using strings for naming Task types. For the time being, the system is sufficient for our needs.
Public Member Functions | |
void | clearParams () |
Clears the parameters. | |
unsigned short | getParamCount () const |
Returns the number of currently pushed parameters. | |
void | setName (const std::string &name) |
Sets the name, first checks the existence of the Task by calling TaskDictionary::isTask. | |
const std::string & | getName () const |
Returns the name of the Task. | |
void | pushParameter (const std::string ¶m) |
Pushes a new parameter string to the parameter collection. | |
std::string | getParameter (unsigned int id) const |
Returns the parameter indexed by id. | |
unsigned short | getOwnerID () const |
Returns the owner ID. | |
void | setOwnerID (unsigned short id=0) |
Sets the owner ID. | |
unsigned int | getID () const |
Gets Task ID. | |
void | setID (unsigned int id=0) |
Sets Task ID. | |
Task (const std::string &name, unsigned short owner=0, unsigned int id=0) |
Constructor & Destructor Documentation
|
Note that during construction, task name is checked against TaskDictionary, so, if an invalid task name is inserted, the name of the task is empty string. If you are not sure about the validity of the task name, try calling TaskDictionary::isTask beforehand. |
Member Function Documentation
|
Gets the parameter indexed at id. If an invalid (out of bounds) index is passed an empty string is returned. |
The documentation for this class was generated from the following files:
- Task.h
- Task.cpp
Generated on Mon Aug 28 15:39:26 2006 for Xface Core Library by
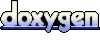