XFaceApp::ApplicationBase Class Reference
#include <ApplicationBase.h>
Inheritance diagram for XFaceApp::ApplicationBase:
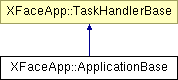
Detailed Description
- Author:
- Koray Balci
Public Types | |
enum | QUERY |
Public Member Functions | |
virtual void | muteAudio (bool bSnd) |
bool | query (const QUERY &q) |
virtual bool | init () |
Protected Member Functions | |
virtual bool | yield ()=0 |
virtual bool | createScriptProcessors ()=0 |
Creates an object implementing IScriptProcessor interface. | |
virtual boost::shared_ptr< ITimer > | createTimer () const =0 |
Creates an object implementing ITimer interface. | |
virtual boost::shared_ptr< ISound > | createSound () const =0 |
Creates an object implementing ISound interface. | |
virtual boost::shared_ptr< FaceBase > | createFace () const =0 |
Creates a FaceBase object (or a derived one). | |
virtual boost::shared_ptr< IRenderer > | createRenderer () const =0 |
Creates an object implementing XFace::IRenderer interface. | |
virtual boost::shared_ptr< IFapStream > | createFapStream () const =0 |
Creates an object implementing IFapStream interface. | |
virtual void | fireNotification (const Notification ¬e) const =0 |
virtual void | renderBegin () const |
virtual void | renderEnd () const |
unsigned long | synchronize (bool) |
virtual void | onRenderFrame () const |
Task Handler. | |
virtual void | onAdvanceFrame () |
virtual bool | onResumePlayback () |
virtual void | onStopPlayback () |
virtual void | onRewindPlayback () const |
virtual bool | onLoadFDP (const std::string ¶m1, const std::string ¶m2) |
virtual bool | onLoadFAP (const std::string ¶m) |
virtual bool | onLoadPHO (const std::string ¶m1, const std::string ¶m2) |
virtual bool | onLoadANIM (const std::string ¶m1) |
virtual bool | onLoadWAV (const std::string ¶m) |
virtual bool | onUpLoadFAP (const std::string ¶m) |
virtual bool | onUpLoadPHO (const std::string ¶m1, const std::string ¶m2, const std::string ¶m3) |
virtual bool | onUpLoadANIM (const std::string ¶m1) |
virtual bool | onUpLoadScript (const std::string ¶m1, const std::string ¶m2) |
Member Enumeration Documentation
|
Basic query mechanism. To be changed.. |
Member Function Documentation
|
Fires the Notification, implementation is probably TCP/IP related, hence left out of core library and implemented in derived class in XfacePlayer. |
|
Pure virtual create* methods are called here to create necessary objects and stored in interface pointers. This way, we provide the mechanism but developers might be interested/need using platform dependent or external library dependent classes, or just improve the already supplied classes in Xface. Sound, Xface::FaceBase, timer, renderer, fap stream, script processor objects are created. |
|
Mutes the audio. Good for testing purposes during development! |
|
Advances the FAP frame, and updates the deformation. No Notification is fired. |
|
Loads the ANIM file from the server (XfacePlayer) side.
Reimplemented from XFaceApp::TaskHandlerBase. |
|
Loads the FAP file from the server (XfacePlayer) side.
Reimplemented from XFaceApp::TaskHandlerBase. |
|
Loads the FDP file from the server (XfacePlayer) harddisk.
Reimplemented from XFaceApp::TaskHandlerBase. |
|
Loads the PHO file from the server (XfacePlayer) side.
Reimplemented from XFaceApp::TaskHandlerBase. |
|
Loads the WAV file from the server (XfacePlayer) disk.
Reimplemented from XFaceApp::TaskHandlerBase. |
|
Renders the current frame. No Notification is fired. Reimplemented from XFaceApp::TaskHandlerBase. |
|
Starts/Resumes the playback of FAP stream and the WAV file and sends back Notification::kStarted in the beginning and Notification::kFinished in the end to the client. On error, Notification::kError is returned. Also yields the message pump for checking if there is a pause or stop Task arrived or not. If by chance, another resume playback Task is in the task queue, it is not executed until tht current task finishes. Reimplemented from XFaceApp::TaskHandlerBase. |
|
Rewinds the FAP stream and the WAV file to beginning and fires Notification::kFinished. Reimplemented from XFaceApp::TaskHandlerBase. |
|
Stops and rewinds the FAP stream and the WAV file to beginning and fires Notification::kFinished. Reimplemented from XFaceApp::TaskHandlerBase. |
|
Reimplemented from XFaceApp::TaskHandlerBase. |
|
Reimplemented from XFaceApp::TaskHandlerBase. |
|
Reimplemented from XFaceApp::TaskHandlerBase. |
|
Processes the script string passed (from remote client possibly). Current IScriptProcessor implementation (Expl2fapProcessor class) also loads the FAP and WAV file produced automatically by issuing proper Tasks.
Reimplemented from XFaceApp::TaskHandlerBase. |
|
Provides basic query mechanism. |
|
Some GUI libraries (wxWidgets, SDL, MFC) for OpenGL windowing operation require pre and especially post processing for double buffering. Derived classes should re-implement these empty methods properly |
|
Some GUI libraries (wxWidgets, SDL, MFC) for OpenGL windowing operation require pre and especially post processing for double buffering. Derived classes should re-implement these empty methods properly |
|
Synchronizes the animation to frames per seconds required by current FAP being played back.
|
|
You should provide an application specific (probably external library dependent) yield functionality which enables processing the task queue, while a task is already being done. We use wxWidgets yield method to achieve this in XfacePlayer implementation (see wxFace class). |
The documentation for this class was generated from the following files:
- ApplicationBase.h
- ApplicationBase.cpp
Generated on Mon Aug 28 15:39:26 2006 for Xface Core Library by
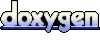