PxRigidBody Class Reference
[Physics]
PxRigidBody is a base class shared between dynamic rigid body objects.
More...
#include <PxRigidBody.h>
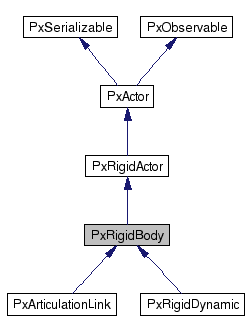
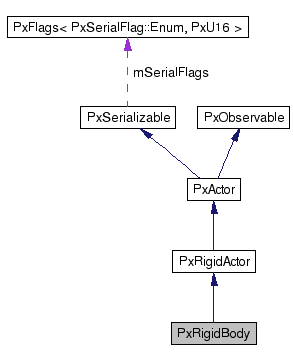
Public Member Functions | |
Mass Manipulation | |
virtual void | setCMassLocalPose (const PxTransform &pose)=0 |
Sets the pose of the center of mass relative to the actor. | |
virtual PxTransform | getCMassLocalPose () const =0 |
Retrieves the center of mass pose relative to the actor frame. | |
virtual void | setMass (PxReal mass)=0 |
Sets the mass of a dynamic actor. | |
virtual PxReal | getMass () const =0 |
Retrieves the mass of the actor. | |
virtual void | setMassSpaceInertiaTensor (const PxVec3 &m)=0 |
Sets the inertia tensor, using a parameter specified in mass space coordinates. | |
virtual PxVec3 | getMassSpaceInertiaTensor () const =0 |
Retrieves the diagonal inertia tensor of the actor relative to the mass coordinate frame. | |
Velocity | |
virtual PxVec3 | getLinearVelocity () const =0 |
Retrieves the linear velocity of an actor. | |
virtual void | setLinearVelocity (const PxVec3 &linVel, bool autowake=true)=0 |
Sets the linear velocity of the actor. | |
virtual PxVec3 | getAngularVelocity () const =0 |
Retrieves the angular velocity of the actor. | |
virtual void | setAngularVelocity (const PxVec3 &angVel, bool autowake=true)=0 |
Sets the angular velocity of the actor. | |
Forces | |
virtual void | addForce (const PxVec3 &force, PxForceMode::Enum mode=PxForceMode::eFORCE, bool autowake=true)=0 |
Applies a force (or impulse) defined in the global coordinate frame to the actor. | |
virtual void | addTorque (const PxVec3 &torque, PxForceMode::Enum mode=PxForceMode::eFORCE, bool autowake=true)=0 |
Applies an impulsive torque defined in the global coordinate frame to the actor. | |
virtual void | clearForce (PxForceMode::Enum mode=PxForceMode::eFORCE, bool autowake=true)=0 |
Clears the accumulated forces (sets the accumulated force back to zero). | |
virtual void | clearTorque (PxForceMode::Enum mode=PxForceMode::eFORCE, bool autowake=true)=0 |
Clears the impulsive torque defined in the global coordinate frame to the actor. | |
PxRigidBody (PxRefResolver &v) | |
PX_INLINE | PxRigidBody () |
virtual | ~PxRigidBody () |
virtual bool | isKindOf (const char *name) const |
Detailed Description
PxRigidBody is a base class shared between dynamic rigid body objects.
- See also:
- PxRigidActor
Constructor & Destructor Documentation
PxRigidBody::PxRigidBody | ( | PxRefResolver & | v | ) | [inline, protected] |
PX_INLINE PxRigidBody::PxRigidBody | ( | ) | [inline, protected] |
virtual PxRigidBody::~PxRigidBody | ( | ) | [inline, protected, virtual] |
Member Function Documentation
virtual void PxRigidBody::addForce | ( | const PxVec3 & | force, | |
PxForceMode::Enum | mode = PxForceMode::eFORCE , |
|||
bool | autowake = true | |||
) | [pure virtual] |
Applies a force (or impulse) defined in the global coordinate frame to the actor.
This will not induce a torque.
PxForceMode determines if the force is to be conventional or impulsive.
- Note:
- The force modes PxForceMode::eIMPULSE and PxForceMode::eVELOCITY_CHANGE can not be applied to articulation links
- Parameters:
-
[in] force Force/Impulse to apply defined in the global frame. Range: force vector [in] mode The mode to use when applying the force/impulse(see PxForceMode) [in] autowake Specify if the call should wake up the actor if it is currently asleep.
- See also:
- PxForceMode addTorque
virtual void PxRigidBody::addTorque | ( | const PxVec3 & | torque, | |
PxForceMode::Enum | mode = PxForceMode::eFORCE , |
|||
bool | autowake = true | |||
) | [pure virtual] |
Applies an impulsive torque defined in the global coordinate frame to the actor.
PxForceMode determines if the torque is to be conventional or impulsive.
- Note:
- The force modes PxForceMode::eIMPULSE and PxForceMode::eVELOCITY_CHANGE can not be applied to articulation links
- Parameters:
-
[in] torque Torque to apply defined in the global frame. Range: torque vector [in] mode The mode to use when applying the force/impulse(see PxForceMode). [in] autowake whether to wake up the object if it is asleep
- See also:
- PxForceMode addForce()
virtual void PxRigidBody::clearForce | ( | PxForceMode::Enum | mode = PxForceMode::eFORCE , |
|
bool | autowake = true | |||
) | [pure virtual] |
Clears the accumulated forces (sets the accumulated force back to zero).
PxForceMode determines if the cleared force is to be conventional or impulsive.
- Note:
- The force modes PxForceMode::eIMPULSE and PxForceMode::eVELOCITY_CHANGE can not be applied to articulation links
- Parameters:
-
[in] mode The mode to use when clearing the force/impulse(see PxForceMode) [in] autowake Specify if the call should wake up the actor if it is currently asleep.
- See also:
- PxForceMode addForce
virtual void PxRigidBody::clearTorque | ( | PxForceMode::Enum | mode = PxForceMode::eFORCE , |
|
bool | autowake = true | |||
) | [pure virtual] |
Clears the impulsive torque defined in the global coordinate frame to the actor.
PxForceMode determines if the cleared torque is to be conventional or impulsive.
- Note:
- The force modes PxForceMode::eIMPULSE and PxForceMode::eVELOCITY_CHANGE can not be applied to articulation links
- Parameters:
-
[in] mode The mode to use when clearing the force/impulse(see PxForceMode). [in] autowake whether to wake up the object if it is asleep
- See also:
- PxForceMode addTorque
virtual PxVec3 PxRigidBody::getAngularVelocity | ( | ) | const [pure virtual] |
Retrieves the angular velocity of the actor.
- Returns:
- The angular velocity of the actor.
virtual PxTransform PxRigidBody::getCMassLocalPose | ( | ) | const [pure virtual] |
Retrieves the center of mass pose relative to the actor frame.
- Returns:
- The center of mass pose relative to the actor frame.
- See also:
- setCMassLocalPose() PxRigidBodyDesc.massLocalPose
virtual PxVec3 PxRigidBody::getLinearVelocity | ( | ) | const [pure virtual] |
Retrieves the linear velocity of an actor.
- Returns:
- The linear velocity of the actor.
virtual PxReal PxRigidBody::getMass | ( | ) | const [pure virtual] |
Retrieves the mass of the actor.
- Returns:
- The mass of this actor.
- See also:
- setMass() PxRigidBodyDesc.mass setMassSpaceInertiaTensor()
virtual PxVec3 PxRigidBody::getMassSpaceInertiaTensor | ( | ) | const [pure virtual] |
Retrieves the diagonal inertia tensor of the actor relative to the mass coordinate frame.
This method retrieves a mass frame inertia vector.
- Returns:
- The mass space inertia tensor of this actor.
- See also:
- PxRigidBodyDesc.massSpaceInertia setMassSpaceInertiaTensor() setMass() setCMassLocalPose()
virtual bool PxRigidBody::isKindOf | ( | const char * | name | ) | const [inline, protected, virtual] |
Reimplemented from PxRigidActor.
Reimplemented in PxArticulationLink, and PxRigidDynamic.
References PxRigidActor::isKindOf().
Referenced by PxRigidDynamic::isKindOf(), and PxArticulationLink::isKindOf().
virtual void PxRigidBody::setAngularVelocity | ( | const PxVec3 & | angVel, | |
bool | autowake = true | |||
) | [pure virtual] |
Sets the angular velocity of the actor.
Note that if you continuously set the angular velocity of an actor yourself, forces such as friction will not be able to rotate the actor, because forces directly influence only the velocity/momentum.
Default: (0.0, 0.0, 0.0)
Sleeping: This call wakes the actor if it is sleeping, the autowake parameter is true (default), and the new velocity is non-zero
- Parameters:
-
[in] angVel New angular velocity of actor. Range: angular velocity vector [in] autowake Whether to wake the object up if it is asleep and the velocity is non-zero
- See also:
- getAngularVelocity() setLinearVelocity()
virtual void PxRigidBody::setCMassLocalPose | ( | const PxTransform & | pose | ) | [pure virtual] |
Sets the pose of the center of mass relative to the actor.
- Note:
- Changing this transform will not move the actor in the world!
Setting an unrealistic center of mass which is a long way from the body can make it difficult for the SDK to solve constraints. Perhaps leading to instability and jittering bodies.
Sleeping: This call wakes the actor if it is sleeping.
- Parameters:
-
[in] pose Mass frame offset transform relative to the actor frame. Range: rigid body transform.
- See also:
- getCMassLocalPose() PxRigidBodyDesc.massLocalPose
virtual void PxRigidBody::setLinearVelocity | ( | const PxVec3 & | linVel, | |
bool | autowake = true | |||
) | [pure virtual] |
Sets the linear velocity of the actor.
Note that if you continuously set the velocity of an actor yourself, forces such as gravity or friction will not be able to manifest themselves, because forces directly influence only the velocity/momentum of an actor.
Default: (0.0, 0.0, 0.0)
Sleeping: This call wakes the actor if it is sleeping, the autowake parameter is true (default), and the new velocity is non-zero
- Parameters:
-
[in] linVel New linear velocity of actor. Range: velocity vector [in] autowake Whether to wake the object up if it is asleep and the velocity is non-zero
- See also:
- getLinearVelocity() setAngularVelocity()
virtual void PxRigidBody::setMass | ( | PxReal | mass | ) | [pure virtual] |
Sets the mass of a dynamic actor.
The mass must be positive.
setMass() does not update the inertial properties of the body, to change the inertia tensor use setMassSpaceInertiaTensor() or the PhysX extensions method PxRigidBodyExt::updateMassAndInertia().
Default: 1.0
Sleeping: Does NOT wake the actor up automatically.
- Parameters:
-
[in] mass New mass value for the actor. Range: (0,inf)
- See also:
- getMass() PxRigidBodyDesc.mass setMassSpaceInertiaTensor()
virtual void PxRigidBody::setMassSpaceInertiaTensor | ( | const PxVec3 & | m | ) | [pure virtual] |
Sets the inertia tensor, using a parameter specified in mass space coordinates.
Note that such matrices are diagonal -- the passed vector is the diagonal.
If you have a non diagonal world/actor space inertia tensor(3x3 matrix). Then you need to diagonalize it and set an appropriate mass space transform. See setCMassLocalPose().
Default: (1.0, 1.0, 1.0)
Sleeping: Does NOT wake the actor up automatically.
- Parameters:
-
[in] m New mass space inertia tensor for the actor. Range: inertia vector
- See also:
- PxRigidBodyDesc.massSpaceInertia getMassSpaceInertia() setMass() setCMassLocalPose()
The documentation for this class was generated from the following file:
Copyright © 2008-2012 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com