PxContactSet Class Reference
[Physics]
An array of contact points, as passed to contact modification.
More...
#include <PxContactModifyCallback.h>
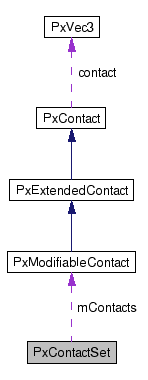
Public Member Functions | |
PX_FORCE_INLINE const PxVec3 & | getPoint (PxU32 i) const |
Get the position of a specific contact point in the set. | |
PX_FORCE_INLINE void | setPoint (PxU32 i, const PxVec3 &p) |
Alter the position of a specific contact point in the set. | |
PX_FORCE_INLINE const PxVec3 & | getNormal (PxU32 i) const |
Get the contact normal of a specific contact point in the set. | |
PX_FORCE_INLINE void | setNormal (PxU32 i, const PxVec3 &n) |
Alter the contact normal of a specific contact point in the set. | |
PX_FORCE_INLINE PxReal | getSeparation (PxU32 i) const |
Get the separation of a specific contact point in the set. | |
PX_FORCE_INLINE void | setSeparation (PxU32 i, PxReal s) |
Alter the separation of a specific contact point in the set. | |
PX_FORCE_INLINE const PxVec3 & | getTargetVelocity (PxU32 i) const |
Get the target velocity of a specific contact point in the set. | |
PX_FORCE_INLINE void | setTargetVelocity (PxU32 i, const PxVec3 &v) |
Alter the target velocity of a specific contact point in the set. | |
PX_FORCE_INLINE PxU32 | getInternalFaceIndex0 (PxU32 i) |
Get the face index with respect to the first shape of the pair for a specific contact point in the set. | |
PX_FORCE_INLINE PxU32 | getInternalFaceIndex1 (PxU32 i) |
Get the face index with respect to the second shape of the pair for a specific contact point in the set. | |
PX_FORCE_INLINE PxReal | getMaxImpulse (PxU32 i) const |
Get the maximum impulse for a specific contact point in the set. | |
PX_FORCE_INLINE void | setMaxImpulse (PxU32 i, PxReal s) |
Alter the maximum impulse for a specific contact point in the set. | |
PX_FORCE_INLINE PxReal | getRestitution (PxU32 i) const |
Get the restitution coefficient for a specific contact point in the set. | |
PX_FORCE_INLINE void | setRestitution (PxU32 i, PxReal r) |
Alter the restitution coefficient for a specific contact point in the set. | |
PX_FORCE_INLINE PxReal | getStaticFriction (PxU32 i) const |
Get the static friction coefficient for a specific contact point in the set. | |
PX_FORCE_INLINE void | setStaticFriction (PxU32 i, PxReal f) |
Alter the static friction coefficient for a specific contact point in the set. | |
PX_FORCE_INLINE PxReal | getDynamicFriction (PxU32 i) const |
Get the static friction coefficient for a specific contact point in the set. | |
PX_FORCE_INLINE void | setDynamicFriction (PxU32 i, PxReal f) |
Alter the static dynamic coefficient for a specific contact point in the set. | |
PX_FORCE_INLINE void | ignore (PxU32 i) |
Ignore the contact point. | |
PX_FORCE_INLINE PxU32 | size () const |
The number of contact points in the set. | |
PX_FORCE_INLINE PxReal | getInvMassScale0 () const |
Returns the invMassScale of body 0. | |
PX_FORCE_INLINE PxReal | getInvMassScale1 () const |
Returns the invMassScale of body 1. | |
PX_FORCE_INLINE PxReal | getInvInertiaScale0 () const |
Returns the invInertiaScale of body 0. | |
PX_FORCE_INLINE PxReal | getInvInertiaScale1 () const |
Returns the invInertiaScale of body 1. | |
PX_FORCE_INLINE void | setInvMassScale0 (const PxReal scale) |
Sets the invMassScale of body 0. | |
PX_FORCE_INLINE void | setInvMassScale1 (const PxReal scale) |
Sets the invMassScale of body 1. | |
PX_FORCE_INLINE void | setInvInertiaScale0 (const PxReal scale) |
Sets the invInertiaScale of body 0. | |
PX_FORCE_INLINE void | setInvInertiaScale1 (const PxReal scale) |
Sets the invInertiaScale of body 1. | |
Protected Member Functions | |
PX_FORCE_INLINE PxContactPatch * | getPatch () const |
Protected Attributes | |
PxU32 | mCount |
Number of contact points in the set. | |
PxModifiableContact * | mContacts |
The contact points of the set. |
Detailed Description
An array of contact points, as passed to contact modification.The word 'set' in the name does not imply that duplicates are filtered in any way. This initial set of contacts does potentially get reduced to a smaller set before being passed to the solver.
You can use the accessors to read and write contact properties. The number of contacts is immutable, other than being able to disable contacts using ignore().
- See also:
- PxContactModifyCallback, PxModifiableContact
Member Function Documentation
PX_FORCE_INLINE PxReal PxContactSet::getDynamicFriction | ( | PxU32 | i | ) | const [inline] |
Get the static friction coefficient for a specific contact point in the set.
- See also:
- PxModifiableContact.dynamicFriction
Get the face index with respect to the first shape of the pair for a specific contact point in the set.
- See also:
- PxModifiableContact.internalFaceIndex0
References PX_UNUSED(), and PXC_CONTACT_NO_FACE_INDEX.
Get the face index with respect to the second shape of the pair for a specific contact point in the set.
- See also:
- PxModifiableContact.internalFaceIndex1
References PxContactPatch::eHAS_FACE_INDICES, PxContactPatch::internalFlags, and PXC_CONTACT_NO_FACE_INDEX.
PX_FORCE_INLINE PxReal PxContactSet::getInvInertiaScale0 | ( | ) | const [inline] |
Returns the invInertiaScale of body 0.
A value < 1.0 makes this contact treat the body as if it had larger inertia. A value of 0.f makes this contact treat the body as if it had infinite inertia. Any value > 1.f makes this contact treat the body as if it had smaller inertia.
PX_FORCE_INLINE PxReal PxContactSet::getInvInertiaScale1 | ( | ) | const [inline] |
Returns the invInertiaScale of body 1.
A value < 1.0 makes this contact treat the body as if it had larger inertia. A value of 0.f makes this contact treat the body as if it had infinite inertia. Any value > 1.f makes this contact treat the body as if it had smaller inertia.
PX_FORCE_INLINE PxReal PxContactSet::getInvMassScale0 | ( | ) | const [inline] |
Returns the invMassScale of body 0.
A value < 1.0 makes this contact treat the body as if it had larger mass. A value of 0.f makes this contact treat the body as if it had infinite mass. Any value > 1.f makes this contact treat the body as if it had smaller mass.
PX_FORCE_INLINE PxReal PxContactSet::getInvMassScale1 | ( | ) | const [inline] |
Returns the invMassScale of body 1.
A value < 1.0 makes this contact treat the body as if it had larger mass. A value of 0.f makes this contact treat the body as if it had infinite mass. Any value > 1.f makes this contact treat the body as if it had smaller mass.
PX_FORCE_INLINE PxReal PxContactSet::getMaxImpulse | ( | PxU32 | i | ) | const [inline] |
Get the maximum impulse for a specific contact point in the set.
- See also:
- PxModifiableContact.maxImpulse
Get the contact normal of a specific contact point in the set.
- See also:
- PxModifiableContact.normal
PX_FORCE_INLINE PxContactPatch* PxContactSet::getPatch | ( | ) | const [inline, protected] |
Get the position of a specific contact point in the set.
- See also:
- PxModifiableContact.point
PX_FORCE_INLINE PxReal PxContactSet::getRestitution | ( | PxU32 | i | ) | const [inline] |
Get the restitution coefficient for a specific contact point in the set.
- See also:
- PxModifiableContact.restitution
PX_FORCE_INLINE PxReal PxContactSet::getSeparation | ( | PxU32 | i | ) | const [inline] |
PX_FORCE_INLINE PxReal PxContactSet::getStaticFriction | ( | PxU32 | i | ) | const [inline] |
Get the static friction coefficient for a specific contact point in the set.
- See also:
- PxModifiableContact.staticFriction
Get the target velocity of a specific contact point in the set.
- See also:
- PxModifiableContact.targetVelocity
PX_FORCE_INLINE void PxContactSet::ignore | ( | PxU32 | i | ) | [inline] |
Ignore the contact point.
If a contact point is ignored then no force will get applied at this point. This can be used to disable collision in certain areas of a shape, for example.
PX_FORCE_INLINE void PxContactSet::setDynamicFriction | ( | PxU32 | i, | |
PxReal | f | |||
) | [inline] |
Alter the static dynamic coefficient for a specific contact point in the set.
- See also:
- PxModifiableContact.dynamic
References PxContactPatch::eREGENERATE_PATCHES, and PxContactPatch::internalFlags.
PX_FORCE_INLINE void PxContactSet::setInvInertiaScale0 | ( | const PxReal | scale | ) | [inline] |
Sets the invInertiaScale of body 0.
This can be set to any value in the range [0, PX_MAX_F32). A value < 1.0 makes this contact treat the body as if it had larger inertia. A value of 0.f makes this contact treat the body as if it had infinite inertia. Any value > 1.f makes this contact treat the body as if it had smaller inertia.
References PxContactPatch::eHAS_MODIFIED_MASS_RATIOS, and PxContactPatch::internalFlags.
PX_FORCE_INLINE void PxContactSet::setInvInertiaScale1 | ( | const PxReal | scale | ) | [inline] |
Sets the invInertiaScale of body 1.
This can be set to any value in the range [0, PX_MAX_F32). A value < 1.0 makes this contact treat the body as if it had larger inertia. A value of 0.f makes this contact treat the body as if it had infinite inertia. Any value > 1.f makes this contact treat the body as if it had smaller inertia.
References PxContactPatch::eHAS_MODIFIED_MASS_RATIOS, and PxContactPatch::internalFlags.
PX_FORCE_INLINE void PxContactSet::setInvMassScale0 | ( | const PxReal | scale | ) | [inline] |
Sets the invMassScale of body 0.
This can be set to any value in the range [0, PX_MAX_F32). A value < 1.0 makes this contact treat the body as if it had larger mass. A value of 0.f makes this contact treat the body as if it had infinite mass. Any value > 1.f makes this contact treat the body as if it had smaller mass.
References PxContactPatch::eHAS_MODIFIED_MASS_RATIOS, and PxContactPatch::internalFlags.
PX_FORCE_INLINE void PxContactSet::setInvMassScale1 | ( | const PxReal | scale | ) | [inline] |
Sets the invMassScale of body 1.
This can be set to any value in the range [0, PX_MAX_F32). A value < 1.0 makes this contact treat the body as if it had larger mass. A value of 0.f makes this contact treat the body as if it had infinite mass. Any value > 1.f makes this contact treat the body as if it had smaller mass.
References PxContactPatch::eHAS_MODIFIED_MASS_RATIOS, and PxContactPatch::internalFlags.
PX_FORCE_INLINE void PxContactSet::setMaxImpulse | ( | PxU32 | i, | |
PxReal | s | |||
) | [inline] |
Alter the maximum impulse for a specific contact point in the set.
- Note:
- Must be nonnegative. If set to zero, the contact point will be ignored
- See also:
- PxModifiableContact.maxImpulse
References PxContactPatch::eHAS_MAX_IMPULSE, and PxContactPatch::internalFlags.
Alter the contact normal of a specific contact point in the set.
- Note:
- Changing the normal can cause contact points to be ignored.
- See also:
- PxModifiableContact.normal
References PxContactPatch::eREGENERATE_PATCHES, and PxContactPatch::internalFlags.
Alter the position of a specific contact point in the set.
- See also:
- PxModifiableContact.point
PX_FORCE_INLINE void PxContactSet::setRestitution | ( | PxU32 | i, | |
PxReal | r | |||
) | [inline] |
Alter the restitution coefficient for a specific contact point in the set.
- Note:
- Valid ranges [0,1]
- See also:
- PxModifiableContact.restitution
References PxContactPatch::eREGENERATE_PATCHES, and PxContactPatch::internalFlags.
PX_FORCE_INLINE void PxContactSet::setSeparation | ( | PxU32 | i, | |
PxReal | s | |||
) | [inline] |
Alter the separation of a specific contact point in the set.
- See also:
- PxModifiableContact.separation
PX_FORCE_INLINE void PxContactSet::setStaticFriction | ( | PxU32 | i, | |
PxReal | f | |||
) | [inline] |
Alter the static friction coefficient for a specific contact point in the set.
- See also:
- PxModifiableContact.staticFriction
References PxContactPatch::eREGENERATE_PATCHES, and PxContactPatch::internalFlags.
Alter the target velocity of a specific contact point in the set.
- See also:
- PxModifiableContact.targetVelocity
References PxContactPatch::eHAS_TARGET_VELOCITY, and PxContactPatch::internalFlags.
PX_FORCE_INLINE PxU32 PxContactSet::size | ( | ) | const [inline] |
The number of contact points in the set.
Member Data Documentation
PxModifiableContact* PxContactSet::mContacts [protected] |
The contact points of the set.
PxU32 PxContactSet::mCount [protected] |
Number of contact points in the set.
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com